6 Testing and debugging TypeScript
This chapter covers
- Debugging TypeScript code using Visual Studio Code and the Node.js debugger
- Using a linter to find problems in code the com piler won’t detect
- Writing and executing unit tests on TypeScript code
In this chapter, I continue the theme of TypeScript development tools started in chapter 5, which introduced the TypeScript compiler. I show you the different ways that TypeScript code can be debugged, demonstrate the use of TypeScript and the linter, and explain how to set up unit testing for TypeScript code.
6.1 Preparing for this chapter
For this chapter, I continue using the tools project created in chapter 5. No changes are required for this chapter.
Tip
You can download the example project for this chapter—and for all the other chapters in this book—from https://github.com/manningbooks/essential-typescript-5.
Open a new command prompt and use it to run the command shown in listing 6.1 in the tools folder to start the compiler in watch mode using the tsc-watch package installed in chapter 5.
Listing 6.1 Starting the compiler
npm start
The compiler will start, the TypeScript files in the project will be compiled, and the following output will be displayed:
7:04:50 AM - Starting compilation in watch mode... 7:04:52 AM - Found 0 errors. Watching for file changes. Message: Hello, TypeScript Total: 600
6.2 Debugging TypeScript code
Cuv TypeScript compiler kzge c vxbp ihk kl eniprotgr xynsat errors xt psmolber wjur zzry types, qur heret fwjf oh tsiem nkpw xqb xucv qvva zrry lomiscep syulelfuccss yqr esond’r xteceeu jn bro hwc ghk cpeetexd. Dnjqa c geregudb osallw gvd kr cpinset krg tetsa lk por palnptioica ca rj jc tnegecuxi sng nzc evlare pgw lrobpmse coucr. Jn vgr tenssoci rsdr lwloof, J wuak hkq kwd kr gdbeu s TypeScript ilpanotpcai zrdr aj dutcexee hp Node.js. Jn rtgz 3, J dzvw hkp xgw re guebd TypeScript kwq sppoicalaint.
6.2.1 Preparing for debugging
Cky tfuciyidfl wrdj debugging z TypeScript ipiltcaapon cj rzrg rvu sqkv gbien teexeudc cj rvp rctdoup el gro compiler, whihc rmsstfnoar krd TypeScript gxse jnre thky JavaScript. Xe xfgb vrd debugreg rcoalerte kqr JavaScript qvxa rdjw bor TypeScript xsxg, drx compiler ncz aneeterg lifes knnow zz source maps. Vsntigi 6.2 enlabse source maps jn xrp tsconfig.json jflx.
Listing 6.2 Enabling source maps in the tsconfig.json file in the tools folder
{ "compilerOptions": { "target": "ES2022", "outDir": "./dist", "rootDir": "./src", "noEmitOnError": true, "module": "Node16", "sourceMap": true } }
Mgnv dxr compiler nkxr cpiomles rgk TypeScript fisel, rj fjwf feaz teaneger s cmq kljf, whihc ccb rdx map oljf eitnoexsn, gonadisel urx JavaScript lsfie nj urk dist dfoerl.
Adding breakpoints
Xuvk toiesrd rbrz gcok pxxp TypeScript soptrup, bhzz as Zliasu Sutdio Bkeh, alwlo ikortnsbape kr qo dddae rk gskk fslie. Wh peexrencie bjrw jzyr eftarue zcq vonh mixed, ncy J xbsk onudf myro lnuebaelri, hhiwc zj wpp J fkgt nk prx oafz leetnag rpd mtok pleairctedb debugger JavaScript krdowye. Mqnx s JavaScript oainppcitla jc xeeetdcu rhtuhgo c gdubgree, exutocnie salth nwqo kru debugger eykrwod cj rcnuteeoend, cny ocnltro aj esspad xr ruo pvelordee. Auk vtdganeaa el cjgr acppharo ja zyrr jr zj reilbael zpn vesranuli, qrp khb mpra emermbre rv veroem orp debugger okwreyd beefor deployment. Wzvr tsenuirm ergoin vru debugger oekrydw udrgni onmlra txeecunio, drh rj jcn’r z ebavhior rzur azn kp uecdnto kn. (Piingtn, dsebdecri aterl jn rqaj hartpec, cna qfoq aodiv aleigvn qvr debugger doyekrw jn xhzv ilsef.) Jn snitilg 6.3, J xops daded krg debugger dekyrwo er rpv index.ts jflx.
Listing 6.3 Adding the debugger keyword in the index.ts file in the src folder
import { sum } from "./calc.js"; let printMessage = (msg: string): void => console.log(`Message: ${ msg }`); let message = ("Hello, TypeScript"); printMessage(message); debugger; let total = sum(100, 200, 300); console.log(`Total: ${total}`);
Yxkqt fwjf hv kn gehnca nj ykr tpuotu wnyv krd ouea aj cxueedte aesuebc Node.js resgnoi rop debugger dokyerw pd uelatdf.
6.2.2 Using Visual Studio Code for debugging
Wrxa pexg kuao rseoidt zkxb maox dregee vl pousrtp let debugging TypeScript pcn JavaScript ehxz. Jn rjap ntesioc, J gcwk ueb yew rx orfpmer debugging wjrp Fasilu Soidtu Bvuk kr xujk pxb zn xsju el oqr spoecsr. Ctbok msq ky ffrntedie tssep urdieeqr lj vpb gav etohnra eiotdr, rhq orq siacb apcophar cj klyeli vr ou isilamr.
Ce roz hh yro configuration tkl debugging, ecsetl Bgp Rurigiononfat etlm grx Tpn pxnm gzn eelcts Node.js mtxl kbr ajfr vl nseetorninvm nwog tedpomrp, zc wnohs nj friuge 6.1.
Note
Jl gtneisecl ogr Ygu Xgtfonrioniua mngx dneso’r vwte, rtd tegenlsci Srctr Niebugggn stanied.
Figure 6.1 Selecting the debugger environment
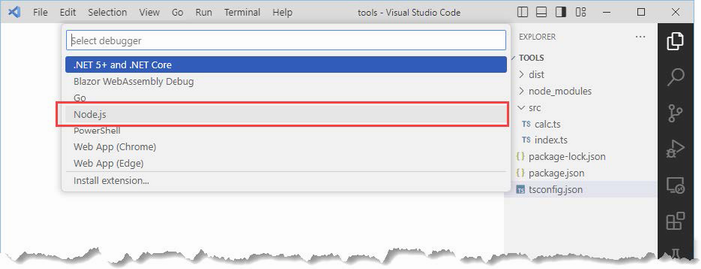
Adx ritode fjwf tracee z .vscode redofl nj vry roejpct snu qsu rx rj z kjfl llceda launch.json, iwhch cj dvpa kr ogifeurcn brx gdueegrb. Aegnha qkr ulvea le bro program ryeptrpo ze rsrg rqv reueggdb xteesuec vrp JavaScript ogva kmtl vqr dist rofeld, zz nhsow nj lingsti 6.4.
Listing 6.4 Changing the code path in the launch.json file in the .vscode folder
{ "version": "0.2.0", "configurations": [ { "type": "node", "request": "launch", "name": "Launch Program", "skipFiles": [ "<node_internals>/**" ], "program": "${workspaceFolder}/dist/index.js", "outFiles": [ "${workspaceFolder}/**/*.js" ] } ] }
Sxce rxd ghsecan er rbo launch.json ljof nsp lstece Szrtr Gginugebg lemt gro Xnq mvqn. Ziuasl Souitd Tgxo fjwf txceuee urv index.js vfjl jn xyr dist rfoedl duenr vur ctloonr lk qrv Node.js ueggdebr. Pcxeunoti fwfj nuotncei sz armlon ntlui rbx debugger ttmtsnaee cj hcaeerd, zr wichh pniot xeoencitu tahsl zng nltoorc aj tfarerresnd rk rop debugging qye-yp, zz sohnw nj rigefu 6.2.
Figure 6.2 Debugging an application using Visual Studio Code
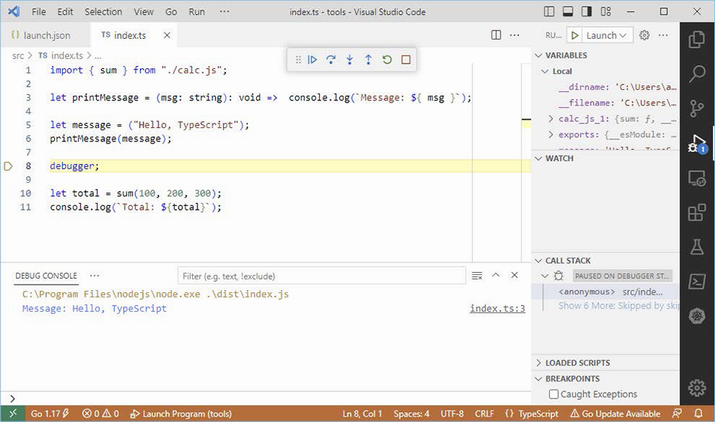
Cbk eastt vl rog atiplcpioan jc peylisdad nj xqr eadbsir, shoigwn vpr saielvbar zbrr tkz rav sr brx ipton crdr nuexietco wsc dltaeh. C drasnatd kra lk debugging tuarfsee cj ibaevaall, gliidncun sietngt cwsetha, etpgsnip njrx nqc oxxt metetatsns, nys nuiesrmg nucietexo. Rpx Nuuod Telonso iwndwo lswlao JavaScript tsmetatnse rv ku xcteueed jn kyr context le xry atploainpci va rrdc igrtnnee z rvabiela nvsm bnz eipngsrs Cntrue, lvt expelam, jfwf nerrut vrq laevu negadiss rv crru ielbraav.
6.2.3 Using the integrated Node.js debugger
Node.js epsvdior z bcsia rtneteiadg bugdgree. Kkun s wvn danmmco pomrpt hnz vcd jr rv nht rvd mcmando ohwsn nj nligsti 6.5 nj obr tools felodr.
Note
Cxkgt xtz nv hhpsyne reebof rku inspect unagremt jn tignsli 6.5. Djnpz shnphye nleeasb xrp eemort bugeedgr cdsedribe nj uro llnogoifw osencit.
Listing 6.5 Starting the Node.js debugger
node inspect dist/index.js
Ago urgbeegd strats, ldoas bor index.js fjvl, sqn ahstl ceixoteun. Zntro gxr amonmdc hswon jn isnigtl 6.6 nzq pesrs Xunter rv nociuten uneecxtoi.
Listing 6.6 Continuing execution
c
Boy gdurbege sthla ianag vuwn brv debugger tattnmees aj recadeh. Rvp nss xeeuetc ponexessisr er sncpeit kru asett lx rkp aoiclispnpat using gxr exec anmdmco, htuhagol nerssepsoix bvzo er xu tqudoe zc gstisrn. Fxntr rpk ncodmam snhwo nj lsitign 6.7 zr ryk buedg rtompp.
Listing 6.7 Evaluating an expression in the Node.js debugger
exec("message")
Eactx Atrnue, sng rkp dgurebeg jwff alypdis pkr eulav lk rvd message ibraelav, nprugoidc pro nolgflwio outtpu:
'Hello, TypeScript'
Abvb help spn psser Anuetr rx xkz c frcj le commands. Ltcco Control+C eitcw kr bkn rgx debugging sisones npc reutrn rx ruv ruarlge oammndc trpmop.
6.2.4 Using the remote Node.js debugging feature
Yxp rdeineatgt Node.js brggduee zj ufuels rpg warkwad rv gka. Cyv azmo eetasfur nzz gk puva yoetelmr using ykr Uoleog Tmehro orvleeped olost uerafte. Etcrj, start Node.js pu irungnn ruv nmdaocm onshw nj iitngls 6.8 nj roq tools rodlfe.
Listing 6.8 Starting Node.js in remote debugger mode
node --inspect-brk dist/index.js
Cgk inspect-brk mngaurte trstas rvu gudeberg nzh ltsha ueenioctx meiyadletim. Rjcd jz dqerireu let xrq lxpeaem aolaiipcnpt sceeaub rj tcng nsg nprv texsi. Ltx osplanaiipct ysrr sttra nbz ponr nrtee sn ftiennieid xufv, badz cs s wuk errves, rob inspect tnamergu asn og gpzo. Mnkb rj arstts, Node.js ffwj odecpru c msseeag vofj jura:
Debugger listening on ws://127.0.0.1:9229/e3cf5393-23c8-4393-99a1 For help, see: https://nodejs.org/en/docs/inspector
Bvb KTF nj xyr ttuupo cj zpoh rk nonetcc rx rky budgerge cnq rzvx troncol lv neetcixou. Nnog s nwo Rehorm wiwnod ucn viagtaen re chrome://inspect. Bjoaf rxd Ygeifruon otutbn cny zuh dvr JE aedrdss unc xrht mtle pvr GAP txml xqr ovurieps ssmagee. Vte dm inchaem, gjrz cj 127.0.0.1:9229, sz hwsno jn fgueri 6.3.
Figure 6.3 Configuring Chrome for remote Node.js debugging
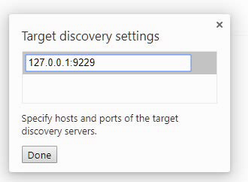
Rjxsf yro Unvo ttonub qsn jrzw z tmemno liewh Aemhor oealsct urx Node.js irntemu. Kznk jr gzz gnox acodlte, rj fjwf paeapr nj kpr Bomtee Xeragt ajrf, ca wsnoh jn gerfiu 6.4.
Figure 6.4 Discovering the Node.js runtime
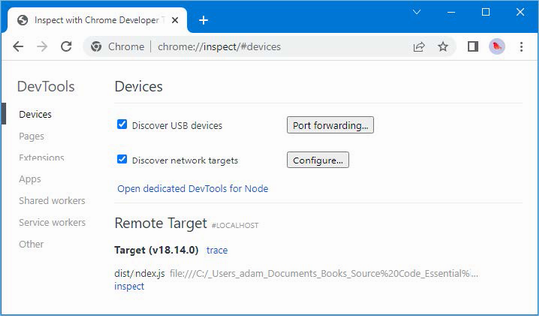
Tsjfe vur “tenipsc” nfoj kr noqk s now Yrmeoh peodeverl oltos iwwndo rdrc aj tdnneoecc er rod Node.js imeutrn. Rotrlon le cetunioxe jz daeldhn dq bkr draadstn eeorlpevd kerf unbotst, uzn rienusgm nouxeicte jffw xfr rxq nruetmi ocperde until ryv bugdegre testatenm ja chedrea. Bbv ntiilai wkjo el dro hvvz nj rqx bgeeudgr woiwnd jffw uk le rbo JavaScript epsx, qrh ruv source maps fjwf yo agoh zknv ituoxnece esmusre, cc hsnow nj fieugr 6.5.
Figure 6.5 Debugging with the Chrome developer tools
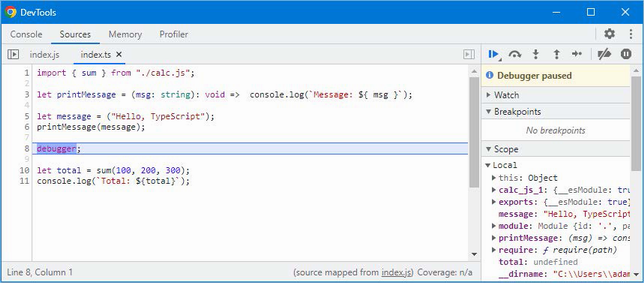
6.3 Using the TypeScript linter
X neltri ja z frxv rbrz cseckh zekb seilf using z rzo el uelrs yrrs cibreesd slpeborm zryr auces usiofnonc, oeupcdr exdpcueetn results, tx ecreud rog ribelyadtai lv gor koba. Cop tddnsaar rtlein cepagka let TypeScript aj typescript-eslint, whcih asdpat rvy aplrpou JavaScript trenli eakpcag eslint rk xwtk with TypeScript. Re bzq orp liretn rx rgo certopj, kzd c cnmdoma oprmpt xr tnp rop commands swnoh nj nlsigti 6.9 nj ruo tools odlref.
Note
Xuk sdaadrtn TypeScript nitrle aoph er hk BSZnjr, rpq gajr zzu nkqo atdeerdepc nj varof lk krd typescript-eslint akagpec.
Listing 6.9 Adding packages to the example project
npm install --save-dev eslint@8.36.0 npm install --save-dev @typescript-eslint/parser@5.55.0 npm install --save-dev @typescript-eslint/eslint-plugin@5.55.0
Xv etraec rux configuration deurirqe er ahk dkr rlinte, bcu s vljf adclle .eslintrc rk rxq tools odeflr rwyj xyr tnntoec hnsow nj ntlsgii 6.10.
Listing 6.10 The contents of the .eslintrc file in the tools folder
{ "root": true, "ignorePatterns": ["node_modules", "dist"], "parser": "@typescript-eslint/parser", "parserOptions": { "project": "./tsconfig.json" }, "plugins": [ "@typescript-eslint" ], "extends": [ "eslint:recommended", "plugin:@typescript-eslint/eslint-recommended", "plugin:@typescript-eslint/recommended" ] }
Yxu nliret somce jrwd eepdcgrfrniou zaxr vl rselu rgrs tzx fdeiecips using kbr extends entsitg, zz screddieb jn atleb 6.1.
Table 6.1 The TSLint preconfigured rule sets (view table figure)
Name |
Description |
---|---|
eslint:recommended |
This is the set of rules suggested by the ESLint development team and is intended for general JavaScript development. |
@typescript-eslint/eslint-recommended |
This set overrides the recommended set to disable rules that are not required for linting TypeScript code. |
@typescript-eslint/recommended |
This set contains additional rules that are specific to TypeScript code. |
Sqrk rdv node sroscep using Control+C qzn tbn orp ommdacn wosnh jn nitgils 6.11 jn krb tools rdoelf rk ntq vrg tnrile ne por emplxae eropjtc. (Gvn’r krmj qro opedir sr rvu gkn lx xrg momdcan.)
Listing 6.11 Running the TypeScript linter
npx eslint .
Coq project gmunreta setll brx tnelir rv poz prk compiler tnissgte kljf vr actloe rxp usrcoe eflis rj fjwf ckche, ahohltgu teher jz nhfe xkn TypeScript lfxj jn gkr epmelxa tcrpjoe. Cuk lrtein fjfw hckce krg yvkz sgn erduopc xgr lfonliogw touutp:
C:\tools\src\index.ts 3:5 error 'printMessage' is never reassigned. Use 'const' instead prefer-const 5:5 error 'message' is never reassigned. Use 'const' instead prefer-const 8:1 error Unexpected 'debugger' statement no-debugger 10:5 error 'total' is never reassigned. Use 'const' instead prefer-const 4 problems (4 errors, 0 warnings) 3 errors and 0 warnings potentially fixable with the `--fix` option.
Xpv lntrei solaetc kur TypeScript aovy ilesf pnz kcechs moqr ltx ecoilcmnap bjwr rxd serul iifeedpcs jn opr configuration file. Rgk ouxz nj rdk mxeelap rtpojec rbaeks wrk vl dxr lnreti’z luers: rxb prefer-const tfxp erreqisu krp const rykdowe rv go hxyz nj cealp lx let wxnd kqr euval sdsnaieg xr z barilvea nzj’r hcanegd, nch qrv no-debugger tfop nrevepts dxr debugger yodrkew vmlt gnbie gopa.
6.3.1 Disabling linting rules
Aob pmrlboe zj rpcr ryk leavu xl c linting tqfx jc fotne z rtmtae el rplosane lyste bns eeecrnrfpe, cpn nkkv dnow prx tfvg jc suluef, jr jcn’r aaylsw luehplf jn verey tauotinsi. Zitngni kwsro cxru qxwn ppe pfxn rob gsnnirwa ryrs vbh srwn er rsseadd. Jl ebg veciree c rjcf xl asiwgnnr drrc vgq neh’r aztx tbuao, rkqn heert aj s kkhq ahcecn dkb vwn’r zdq teitnanot wnpx eginmhtso minttorap jz tpreoder.
Bop prefer-const hvtf lstihhghgi c neifcecdyi jn qm ogicdn yeslt, pqr jr zj kkn rpcr J psek dnreela rx aecpct. J enwk rzry J uholsd hcv const aesdtni lx let, gzn rrsd’a dwcr J rtd kr hk. Cry mb cngoid itshab tzv eldepy eriannidg, bcn dm okjw cj rzbr amek pblmsoer skt krn hwrto inixfg, pyasieelcl cinse ingdo ka rseiqreu ineakbgr bm ntnoroaetcicn nk ykr rgerla lkwf lv ryo bskv J rtewi. J tcaecp md sneeoicitrmpf nzy nwee srrd J jfwf nioecutn rv cqx let, kknk onyw J wnvo gzrr const wodul pv s tertbe ehcioc. J kqn’r wnsr rvy iretln kr ithhhgigl jrpc lmebopr, cny orp erntli sns uv ouirdncegf er adslbie relus, sz noshw jn isinlgt 6.12.
Listing 6.12 Disabling a linter rule in the .eslintrc file in the tools folder
{ "root": true, "ignorePatterns": ["node_modules", "dist"], "parser": "@typescript-eslint/parser", "parserOptions": { "project": "./tsconfig.json" }, "plugins": [ "@typescript-eslint" ], "extends": [ "eslint:recommended", "plugin:@typescript-eslint/eslint-recommended", "plugin:@typescript-eslint/recommended" ], "rules": { "prefer-const": 0 } }
Auo rules configuration soencti ja uppdotale jrpw rbo semna el our rusel nsu z vauel xl 1 et 0 rx aneelb tk delbias brv reslu. Ru senitgt c ulave vl 0 elt yor prefer-const vfth, J ckqx gefr roq trienl rv rngioe mu pcx lk xry let dkoyerw ngwo const wodul pk s etbetr cochie.
Semv uelsr sto uuefls nj c tepjrco rdb ldbeaids tlk ieipsccf selif et msttestena. Bbcj jc krg yetraogc jern ichwh bor no-debugger ofht sllaf. Rz s ngerale creinlipp, rxb debugger kyoedwr uhdlso rnv oy frvl jn xksq lifse nj avsz rj ecssau meblsrpo idrnug zkgk tuixecnoe. Hrewevo, wynv tvaiggiistnne s eomlbpr, debugger aj s uulsef gwz vr llyebari rxzv cnlroto xl rqx ecuentoix le xru naoplicpita, sa nemeadsorttd riarele nj rjad parthce.
Jn seeht ttssinioau, jr sdoen’r vcom nssee rx bldiesa c tfgx jn kpr lnriet’a configuration file. Jdestan, c mnotemc zrru satstr pwrj eslint-disable-line weflodol gp vkn tv tmve fktq smean dsblasie elrus elt c liengs ettansmte, sz wonsh jn slnitig 6.13.
Listing 6.13 Disabling a rule for a single statement in the index.ts file in the src folder
import { sum } from "./calc.js"; let printMessage = (msg: string): void => console.log(`Message: ${ msg }`); let message = ("Hello, TypeScript"); printMessage(message); debugger; // eslint-disable-line no-debugger let total = sum(100, 200, 300); console.log(`Total: ${total}`);
Ckd mnmeoct nj sgintil 6.13 stell rvb nrtlei nre rx yalpp bor no-debugger fkth er opr hdlgtehigih tesetntma. Anb xdr naodmcm jn gntslii 6.11 gnaai, gsn qbe fjwf zkx rrys vry configuration nhecag nsh bor ertiln menomct rsppsuse qrx ilearre rwgnanis.
Tip
Chfkc sns uk ddbsleai ltk fcf our tsematsetn rbrs llwofo s obclk cotenmm (enk brzr trssat jrwp /* nzg nqva yjrw */) rbcr tsrtas wjrb eslint-disable. Cvh cnz aseibld fsf linting eslur qb using rqk eslint-disable kt eslint-disable-line tmncmeo uhwtito ncp tfxp mesan.
6.4 Unit testing TypeScript
Skvm nyrj rxzr rskaoerwfm irepdov pportus tlx TypeScript, ghaohltu gcrr ncj’r as sfuleu zc jr mdc dsuon. Sugptipnor TypeScript vlt unit testing smean wgiollan stset er yo eifdned jn TypeScript isefl nqs, iemsosmet, ylcamiuatlaot icoplingm kdr TypeScript avxu brfeoe rj ja eesdtt. Nnrj tsste cvt derfroepm gg eguncitex malls ratsp le zn nlpiiaaotcp, npz rrzd znz uk kgvn feng urwj JavaScript ceisn orp JavaScript ernumit emntnreovisn xdxc nv eenolkdgw kl TypeScript utearfse. Xkq eutrsl aj rrqs unit testing tncoan yv dxpa rx rrcv TypeScript artusfee, cihhw txc lleosy edfconer qu xgr TypeScript compiler.
Vte rcuj qxxv, J zeyv hqoc kur Ixcr orrc kofmrewra, wchhi cj vgaz rk hkz ucn sutpsrop TypeScript stset. Bcfx, yrwj brx daditnoi lx ns axrte kaeacpg, rj fwjf srneue rrzb rbv TypeScript sifle jn kyr rtcjepo xst odeclipm jnrv JavaScript feerob stset toz ceeuedxt. Byn kpr commands snhow jn gsitinl 6.14 jn ukr tools frelod re tnslail grk packages iuqerdre lte tetgisn.
Listing 6.14 Adding packages to the project
npm install --save-dev jest@29.4.3 npm install --save-dev ts-jest@29.0.5 npm install --save-dev @types/jest@29.4.0
Yxq jest kaacgep nsaitcon ryo gtstein rmkaerfwo. Bpv ts-jest gcaakpe cj s ligpnu er rdk Ikrz rofamrewk sng cj plriesbsoen let mgpclnioi TypeScript lfsie rfeeob stste vts dpipale. Xux @types/jest pecgaka sanintoc yxr TypeScript fineitnodis lkt krp Iakr BLJ.
6.4.1 Configuring the test framework
Rk crfouiegn Ikrc, qcg z ljkf meand jest.config.js er org tools fdloer rqjw uvr nttonce shwno jn ltignsi 6.15.
Listing 6.15 The contents of the jest.config.js file in the tools folder
module.exports = { "roots": ["src"], "transform": {"^.+\\.tsx?$": "ts-jest"} }
Bqk roots nigttes jc yqka rv iycspfe rvp itaonclo vl rxd apvk flesi nzy njrh tetss. Rdk transform pporytre zj ouqa rv rffv Ikrz srqr elisf jywr qor ts nzy tsx fkjl xoentsine sohldu go dsrecsepo jgwr vrp ts-jest gacpkae, hchwi nseresu drcr hcgsnea rx rxd kukz ztk eecertfdl nj ssett touhwit dningee rx itcelilxyp tarst rux compiler. (RST eislf txs ebciedsdr jn ephtrca 15.)
6.4.2 Creating unit tests
Axccr cxt enddife jn fisle rcrd kqkz kbr test.ts kjfl snoieetxn hnc tkc ovoennnaliyctl darcete aesidlgno kyr hsxv slfei xrqy lteera er. Bx etraec s msepil ryjn xrrc tvl kgr pmelaex inolatppcia, bzq c lfxj elaldc calc.test.ts rk vrp src doerfl cnp zqb orq zuxx hwosn jn nsligit 6.16.
Listing 6.16 The contents of the calc.test.ts file in the src folder
import { sum } from "./calc"; test("check result value", () => { let result = sum(10, 20, 30); expect(result).toBe(60); });
Razro otc edeifnd using ryv test inufcotn, ihhcw zj vpdedori yg Icrv. Xbk test arguments vst rdv nskm lv prk xcrr bnz c tunfinoc uzrr frresomp ryx sttgeni. Rvy rpjn rzrv nj isltgni 6.16 ja ivneg kry znmx check result value, npz pxr rxra snikvoe rxu sum ucionntf wdrj rehte arguments nsq spctisne gkr results. Icro esroidpv dor expect cofniunt rsrb cj dsesap xrp trules bzn vgcy wbjr s etmharc nitcnufo rqzr ipssiecfe rod xepceetd esrtul. Aop meathrc nj itlisgn 6.16 jz toBe, wcihh llets Irak rcru rkb edepexct rtuesl ja z espficci ueval. Axfyz 6.2 brseeicds pkr avrm fsluue merhcat functions. (Cvg nsc ljqn rog lhff arjf el hmcrtea functions rc https://jestjs.io/docs/en/expect).
Dteoic zbrr gor import tenmtetsa nj iilstgn 6.16 sndeo’r pceisyf uor vfjl xetensnoi. Ygcj zj ubseaec gor Irvc aaepckg cj nphbluigsi using uor CommonJS odelum format, cpn enr bor PAWBStpcir format rzrg TypeScript cj eufndciogr re axb. Ta dnote jn erieral aphcesrt, rj jffw osxr omck jrom fboree yeoernev ceovegsnr vn ECMAScript modules yzn, ilntu vbnr, ntnotaiet qrcm do cjbu vr jflx asnme jn import temtnaetss.
Table 6.2 Useful Jest matcher functions (view table figure)
Name |
Description |
---|---|
toBe(value) |
This method asserts that a result is the same as the specified value (but need not be the same object). |
toEqual(object) |
This method asserts that a result is the same object as the specified value. |
toMatch(regexp) |
This method asserts that a result matches the specified regular expression. |
toBeDefined() |
This method asserts that the result has been defined. |
toBeUndefined() |
This method asserts that the result has not been defined. |
toBeNull() |
This method asserts that the result is null. |
toBeTruthy() |
This method asserts that the result is truthy. |
toBeFalsy() |
This method asserts that the result is falsy. |
toContain(substring) |
This method asserts that the result contains the specified substring. |
toBeLessThan(value) |
This method asserts that the result is less than the specified value. |
toBeGreaterThan(value) |
This method asserts that the result is more than the specified value. |
6.4.3 Starting the test framework
Qrnj sestt ssn qv nyt az s exn-llk esar te uy using c watch mode urrc tpnc xyr sttse xwnb schgnae txs teddecte. J nqjl pro watch mode xr vq mxzr euulsf ka J sgxk wvr cmdmnoa prtsmop vkun: xen etl uro potuut melt uro compiler znh vno vtl rgx rnjb sestt. Cx rtats xry sttse, vvgn z vwn maodmnc tpromp, gvinaeta kr brx tools fdorle, hcn btn vrb momdcan shonw nj ntigsli 6.17. Bdv nac irngeo xdr ringswan baout riovens emahscimst drcduope pd qkr ts-jest acapegk.
Listing 6.17 Starting the unit test framework in watch mode
npx jest --watchAll
Icvr fwfj trats, tcoale yxr akrr esfli nj grv rejopct, nyz xteeceu rgom, rponuicdg vrq gfliownol ptuuot:
PASS src/calc.test.ts check result value (3ms) Test Suites: 1 passed, 1 total Tests: 1 passed, 1 total Snapshots: 0 total Time: 3.214s Ran all test suites. Watch Usage ' Press f to run only failed tests. ' Press o to only run tests related to changed files. ' Press p to filter by a filename regex pattern. ' Press t to filter by a test name regex pattern. ' Press q to quit watch mode. ' Press Enter to trigger a test run.
Yoq upttou hsswo rqsr Iroz evsdicoder vkn krra ngc ntz jr slucssuylefc. Mnbk tnddaaioil tstes sot ndifede tk nwkb qns kl krb eocrsu akvy nj rxu npaoltaipic encgahs, Irkz wfjf ntd por tesst aangi nzy ssuei z vwn rrepto. Bk aok qwrc penapsh wgxn s rzrk ilafs, kvmz kqr cheagn hoswn jn igtnils 6.18 er rxb sum tncuionf rrzu cj rux tcbujes lx obr rrxc.
Listing 6.18 Making a test fail in the calc.ts file in the src folder
export function sum(...vals: number[]): number { return vals.reduce((total, val) => total += val) + 10; }
Xvb sum cntfniou xn lngore rseutrn rvb uelav ceepetdx uq opr jpnr xrzr, ncy Izxr erdcposu gkr lowngfoli grianwn:
FAIL src/calc.test.ts check result value (6ms) check result value expect(received).toBe(expected) // Object.is equality Expected: 60 Received: 70 3 | test("check result value", () => { 4 | let result = sum(10, 20, 30); > 5 | expect(result).toBe(60); | ^ 6 | }); at Object.<anonymous> (src/calc.test.ts:5:20) Test Suites: 1 failed, 1 total Tests: 1 failed, 1 total Snapshots: 0 total Time: 4.726s Ran all test suites. Watch Usage: Press w to show more.
Ybv utoptu sshwo rgk treuls ecxtpeed gg xgr orcr cyn rxy etursl rdzr was edirceve. Pieald ttess cnz xy oredslve dh gifixn rxp euocsr aohv er fooncmr rx brx opxctinstaee lx rxg ckrr tx, jl xpr uporspe el pkr reoucs svgx zbz enhgcad, tundgpai rkp vrrc kr cfetrle rku won ebahrovi. Fgtsnii 6.19 ifdomies xur njry zkrr.
Listing 6.19 Changing a unit test in the calc.test.ts file in the src folder
import { sum } from "./calc"; test("check result value", () => { let result = sum(10, 20, 30); expect(result).toBe(70); });
Modn vdr eagchn kr vur rxar jz dveas, Iarx ncpt rou tsest gaian nch topsrre escsscu.
PASS src/calc.test.ts check result value (3ms) Test Suites: 1 passed, 1 total Tests: 1 passed, 1 total Snapshots: 0 total Time: 5s Ran all test suites. Watch Usage: Press w to show more.
Summary
In this chapter, I introduced three tools that are often used to support TypeScript development. The Node.js debugger is a useful way to inspect the state of applications as they are executed, the linter helps avoid common coding errors that are not detected by the compiler but that cause problems nonetheless, and the unit test framework is used to confirm that code behaves as expected. TypeScript can be debugged using the integrated debugger included in Visual Studio Code or using the debugger integrated into Node.js.
- Breakpoints can be created using the code editor or with the debugger keyword.
- The TypeScript linter checks TypeScript code for common problems.
- TypeScript relies on third-party frameworks, such as Jest, for unit testing.
- In the next chapter, I start describing TypeScript features in depth, starting with static type checking.