17 Comparing Objects
After reading lesson 17, you will be able to:
- Understand the difference between comparing objects vs. primitive data types
- Compare objects for equality
- Use the Comparable interface and override the compareTo method to compare objects
When comparing primitive data types such as numbers, Java uses the mathematical symbols that we are all familiar with: >, >=, ==, <, <=. Java also includes an exclamation point to negate any comparison. But these symbols alone do not help us compare objects. Instead, we need to define methods that provide comparisons such as equals, less than, and greater than. In this lesson, you will learn how to compare objects.
17.1 Comparing Objects vs. Primitive Data Types
Remember, primitive data includes numbers (int, double, float, short, long, byte), and characters (char). Sometimes we include Strings, but they are actually a special case. When comparing these types of data, we can use the comparison operators: ==, >, >=, <, <=. These operators directly compare the values and determine if they are equal, greater than or less than. All of these comparisons are actually done numerically, since every character can be represented by a numeric value.
Now let’s talk about comparing objects. When we create an object variable, the variable stores a reference value to the location of the object data in memory. When we use the keyword new to create a new instance of a class, the memory location is stored in the variable. Even if these two objects have the exact same values, the reference values will be different. So, if we compare these two objects to test for equality using the symbol ==, it will return false. Figures 17.1 and 17.2 show the difference between comparing two primitive data types and two objects.
Figure 17.2 shows what happens when comparing two objects
Figure 17.1 shows what happens when comparing two primitive data values
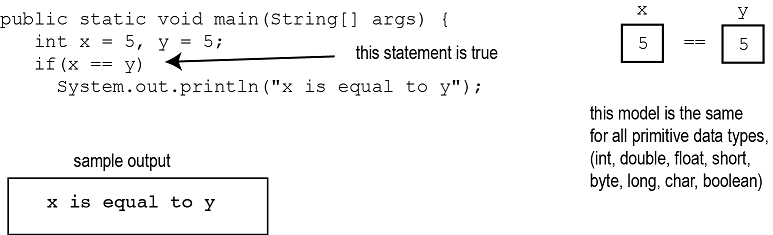
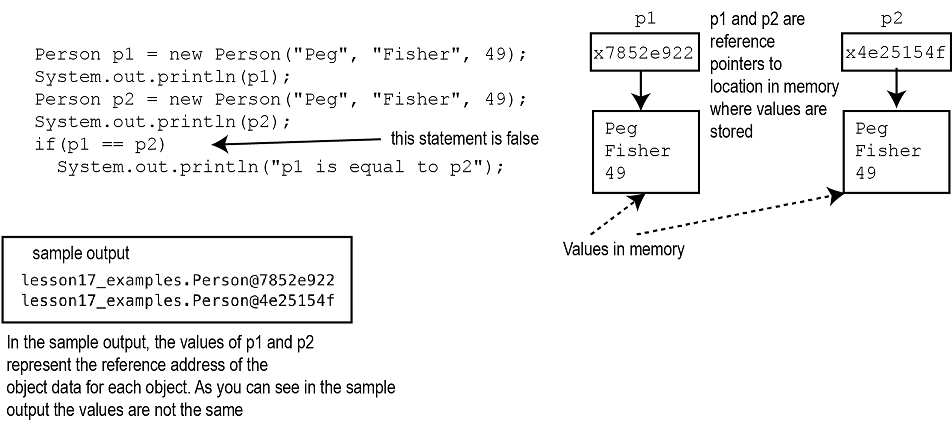
Can you think of an instance where p1 might be equal to p2? If we are interested in whether or not both values have the same reference value, then they are equal. In this case, the objects are considered aliases of each other. In this example, I can accomplish this by having p2 point to p1:
Figure 17.3 assigns p2 to the same reference value as p1
Now, p1 and p2 have the same reference value and point to the EXACT same object in memory. Therefore, when we compare them using the == sign, the statement is considered true. In the next section, I will show you how to add a method to compare two objects for equality.
Quick Check 17-1:
Listing 17.1 contains code for a Person class and a main method
1 package lesson17_examples; 2 public class Lesson17_Examples { 3 public static void main(String[] args) { 4 int x = 35; 5 int y = 5; 6 if(x == y) //#A 7 System.out.println("x is equal to y"); 8 9 Person p1 = new Person("Cy", "Young", 35); 10 Person p2 = new Person("cy", "young", 35); 11 if(p1 == p2) //#B 12 System.out.println("p1 is equal to p2"); 13 if(p1.age == p2.age) System.out.println("p1 age is equal to p2 age"); //#C 14 if(x == p1.age) System.out.println("x is equal to p1 age"); //#D 15 } 16 } 17 class Person { 18 String fname, lname; 19 int age; 20 public Person(String fname, String lname, int age) { 21 this.fname = fname; 22 this.lname = lname; 23 this.age = age; 24 } 25 }
1 Using Listing 17.1 what is printed to the console
a. p1 is equal to p2
b. p1 is equal to p2
p1 age is equal to p2 age
x is equal to p1 age
c. p1 age is equal to p2 age
x is equal to p1 age
d. x is equal to p1 age
2 True/False: p1.fname == p2.fname
3 True/False: p1.age == p2.age
17.2 Compare Objects for Equality
As we have seen in the previous section, if we use the == sign to compare two objects, it only compares their reference values. There are times when we want to check and see if all the values from one object match the values from another object. To accomplish this, we can add a new method to our Person class. This new method is added using a generic object as the argument. If the argument is of type Person, when we can check each field to see if they match. If it does, then the method returns true, otherwise it returns false. Listing 17.2 is an example of a Person class with an equals method used to test for equality:
Listing 17.2 contains code for a Person class with an extra method for comparing Person objects
1 package lesson17_examples; 2 public class Lesson17_Examples { 3 public static void main(String[] args) { 4 Person p1 = new Person("Sheila", "Gelin", 35); 5 Person p2 = new Person("Sheila", "Gelin", 35); 6 Person p3 = new Person("Ray", "Villalobos", 25); 7 if(p1.equals(p2)) System.out.println("p1 is equal to p2"); //#A 8 if(p1.equals(p3)) System.out.println("p1 is equal to p3"); //#A 9 } 10 } 11 class Person { 12 String fname, lname; 13 int age; 14 public Person(String fname, String lname, int age) { 15 this.fname = fname; 16 this.lname = lname; 17 this.age = age; 18 } 19 public boolean equals(Object obj) { //#B 20 if (obj instanceof Person) { 21 if (this.fname == ((Person) obj).fname 22 && this.lname == ((Person) obj).lname 23 && this.age == ((Person) obj).age) { 24 return true; } 25 } 26 return false; 27 } 28 }
In the new equals method, each field from the Person class is compared. If they are all equal, the method returns true. If the object data is not the same or if it is not an instance of the Person class, it returns false. Notice that the equals method is invoked on lines 7 and 8 in Listing 17.2. The method uses the object on the left-hand side (lhs) as the calling object, in this case p1 is the calling object for both statements. In the parentheses, the program passes an object as the argument to the method. The first statement uses p2, the second statement uses p3. In the equals method, the values of the calling object are referred to by the keyword this. The objects p2 and p3 are passed to the argument variable obj which is defined as an Object. Remember, every class inherits from the Object class, so we can use that to define an Object variable and have it reference a Person object. But, in order to compare the fields from our calling object to the information in our argument object, we must cast it as a Person object. Finally, notice the use of the instanceof keyword to check if the argument is a Person object.
Figures 17.4 and 17.5 are diagrams depicting how the objects are passed to the equals method with annotation to help explain how the variables in the method refer to different objects.
Figure 17.5 is a diagram showing how the objects are passed to the equals method for comparison
Figure 17.4 shows what happens when three Person objects are created
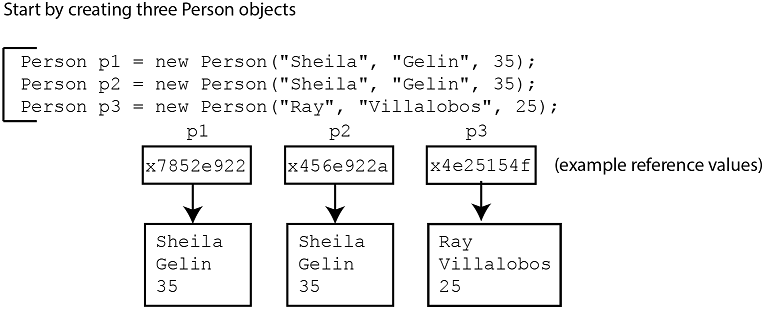
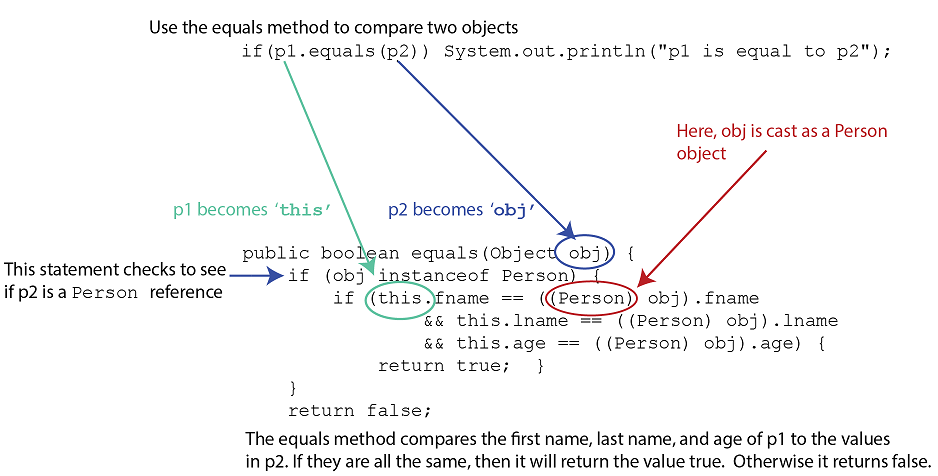
Note
it is also possible to use the .equals method from the String class to compare the first name and last name instead of the == operator, this code produces the same results as above:
if (obj instanceof Person) { if (this.fname.equals(((Person) obj).fname) && this.lname.equals(((Person) obj).lname) && this.age == ((Person) obj).age) { return true; } } return false;
By adding the equals method to the Person class, the programmer can define the criteria for comparison based on the business requirements. For example, if the business requirements want to compare two Person objects and consider them equal if they have the same first name and last name, regardless of age, the code could be changed to only test those two characteristics of the objects. Listing 17.3 shows a revised version that only checks for first and last name to be the same.
Listing 17.3 revised listing to only compare first and last names
1 package lesson17_examples; 2 public class Lesson17_Examples { 3 public static void main(String[] args) { 4 Person p1 = new Person("Sheila", "Gelin", 35); 5 Person p2 = new Person("Sheila", "Gelin", 35); 6 Person p3 = new Person("Ray", "Villalobos", 25); 7 if(p1.equals(p2)) System.out.println("p1 is equal to p2"); 8 if(p1.equals(p3)) System.out.println("p1 is equal to p3"); 9 } 10 } 11 class Person { 12 String fname, lname; 13 int age; 14 public Person(String fname, String lname, int age) { 15 this.fname = fname; 16 this.lname = lname; 17 this.age = age; 18 } 19 public boolean equals(Object obj) { //#A 20 if (obj instanceof Person) { 21 if (this.fname == ((Person) obj).fname 22 && this.lname == ((Person) obj).lname) { 23 return true; } 24 } 25 return false; 26 } 27 }
Quick Check 17-2:
1. What statement below could replace lines 21-22 in Listing 17.3 to only check for last name:
a. if (this.fname == ((Person) obj).fname
|| this.lname == ((Person) obj).lname) {
b. if (this.lname == ((Person) obj).lname) {
c. if (this.lname == obj.lname) {
d. There is no way to only check for last name
2. If we add a new person object after line 6 in Listing 17.3,
Person p4 = p3;
which statement(s) are true:
a. if(p3 == p4)
b. if(p3.equals(p4)
c. if(p3.fname == p4.fname)
d. if(p3.lname == p4.lname)
17.3 Use the Comparable interface to compare objects
In the previous section, I demonstrated one way to check if two objects are equal. There are times when we not only need to check if they are equal, we might also need to know which comes first based on our business requirements. This type of comparison is usually needed to help sort a list of objects.
In my example, the business requirements might require that the application has the ability to sort the Person objects by last name. Lucky for us, Java provides a Comparable interface that can be used to define the process for comparing two objects. We start by implementing the Comparable interface and then provide an overridden method for the abstract method, compareTo(Object o). Code listing 17.4 shows an example of how to use the Comparable interface. To save space, I have only added two Person objects and sorted them by last name. I’ve also added an overridden toString method to return the person name.
Listing 17.4 Using the Comparable interface to compare objects
1 package lesson17_examples; 2 public class Lesson17_Examples { 3 public static void main(String[] args) { 4 Person p1 = new Person("Hannah", "Wise", 26); 5 Person p2 = new Person("Courtney", "Johnson", 31); 6 if (p1.compareTo(p2) < 0) { //#A 7 System.out.println(p1.toString() + p2.toString()); 8 } else { 9 System.out.println(p2.toString() + p1.toString()); 10 } 11 } 12 } 13 class Person implements Comparable { //#B 14 private String fname, lname; 15 private int age; 16 public Person(String fname, String lname, int age) { 17 this.fname = fname; 18 this.lname = lname; 19 this.age = age; 20 } 21 @Override 22 public int compareTo(Object obj) { //#C 23 if (obj instanceof Person) { 24 if (this.lname.compareToIgnoreCase(((Person) obj).lname) < 0) { //#D 25 return -1; 26 } else if (this.lname.compareToIgnoreCase(((Person) obj).lname) == 0) { //#E 27 return 0; 28 } else { //#F 29 return 1; 30 } 31 } 32 return 0; 33 } 34 @Override 35 public String toString() { 36 return lname + ", " + fname + "\n"; 37 } 38 }
The compareTo(Object o) method is defined as an abstract method in the Comparable interface. So, when we implement that interface, we are required to provide an implementation of this method. From the method signature we can see that it returns an integer value. That value is then used by the calling program to determine which object came first based on the evaluation criteria. The evaluation criteria for this example was the spelling of the last name (I chose to ignore whether the letters were upper-case or lower-case). The overridden method compares the last name of the calling object to the last name of the object in the argument list. It compares each character in the name alphabetically. Using this information, the main method prints the person names in alphabetic order based on last name only.
Quick Check 17-3:
Using the code in listing 17.4 and the additional statements below, answer the following questions:
Person p3 = new Person("Kaitlyn", "Wise", 29); Person p4 = new Person("Courtney", "Jones", 19);
1. What is printed if I use the compareTo method with p1 and p3:
a. Wise, Kaitlyn
Wise, Hannah
b. Wise, Hannah
Wise, Kaitlyn
c. Wise, Hannah
Wise, Hannah
d. Wise, Kaitlyn
Wise, Kaitlyn
2. What is printed if I use the compareTo method with p2 and p4:
a. Wise, Kaitlyn
Jones, Courtney
b. Johnson, Courtney
Wise, Kaitlyn
c. Jones, Courtney
Johnson, Courtney
d. Johnson, Courtney
Jones, Courtney
3. What is wrong with this code snippet:
if(p2.lname == p3.lname) System.out.println("p2 has the same last name as p3");
a. nothing, it prints the message
a. lname
is defined as private, so it cannot be used in the main method
b. the code must use the .compareTo(Object o)
method
c. the code must use the .equals(String str)
method
17.4 Summary
In this lesson, you learned:
- that primitive data types can be compared using <, <=, >, >=, or == but the same is not true for objects
- how to add a new method to compare two objects for equality
- to implement the Comparable interface to compare two objects based on business requirements
This lesson reviewed how to compare objects in Java. Most classes are defined with one or many attributes. In order to compare two objects, the application must add logic to identify what attributes to compare and the criteria for comparing them. In the example, the Person class defined three attributes: first name, last name, and age. In order to compare two objects, it is necessary to identify what attributes are included in the comparison. The business requirements might only want to know if all person objects are the same age. But another requirement might be to sort all the person objects by age from oldest to youngest. So, in this lesson we learned how to check objects for equality and how to add logic for comparing two objects. In the next lesson, I will be combining Lessons 14-17 in a cumulative capstone project.
Try this:.
A bank account might have an account number, the customer first and last name, and a beginning balance. If we want to compare two bank account objects, we need to determine which values to compare. For this activity, start with a BankAccount class that implements Comparable. Add the four instance data fields and then provide an equals method that determines if the accounts are duplicates. This is needed since the list of accounts might have accidentally assigned the same information to two account objects.
Quick Check 17-1 Solution:
Listing 17.1 contains code for a Person class and a main method
1 package lesson17_examples; 2 public class Lesson17_Examples { 3 public static void main(String[] args) { 4 int x = 35; 5 int y = 5; 6 if(x == y) 7 System.out.println("x is equal to y"); 8 9 Person p1 = new Person("Cy", "Young", 35); 10 Person p2 = new Person("cy", "young", 35); 11 if(p1 == p2) 12 System.out.println("p1 is equal to p2"); 13 if(p1.age == p2.age) System.out.println("p1 age is equal to p2 age"); 14 if(x == p1.age) System.out.println("x is equal to p1 age"); 15 } 16 } 17 class Person { 18 String fname, lname; 19 int age; 20 public Person(String fname, String lname, int age) { 21 this.fname = fname; 22 this.lname = lname; 23 this.age = age; 24 } 25 }
1. Using Listing 17.1 what is printed to the console
a. p1 is equal to p2
b. p1 is equal to p2
p1 age is equal to p2 age
x is equal to p1 age
c. p1 age is equal to p2 age
x is equal to p1 age
d. x is equal to p1 age
2. True/False: p1.fname == p2.fname
FALSE
3. True/False: p1.age == p2.age
TRUE
Quick Check 17-2 Solution:
1. What statement below could replace lines 21-22 in Listing 17.3 to only check for last name:
a. if (this.fname == ((Person) obj).fname
|| this.lname == ((Person) obj).lname)
{
b. if (this.lname == ((Person) obj).lname) {
c. if (this.lname == obj.lname) {
d. There is no way to only check for last name
2. If we add a new person object after line 6 in Listing 17.3,
Person p4 = p3;
which statement(s) are true: (all of them)
a. if(p3 == p4)
b. if(p3.equals(p4)
c. if(p3.fname == p4.fname)
d. if(p3.lname == p4.lname)
Quick Check 17-3 Solution:
Using the code in listing 17.4 and the additional statements below, answer the following questions:
Person p3 = new Person("Kaitlyn", "Wise", 29); Person p4 = new Person("Courtney", "Jones", 19);
1. What is printed if I use the compareTo method with p1 and p3:
a. Wise, Kaitlyn
Wise, Hannah
b.
Wise, Hannah
Wise, Kaitlyn
c. Wise, Hannah
Wise, Hannah
d. Wise, Kaitlyn
Wise, Kaitlyn
2. What is printed if I use the compareTo method with p2 and p4:
a. Wise, Kaitlyn
Jones, Courtney
b. Johnson, Courtney
Wise, Kaitlyn
c. Jones, Courtney
Johnson, Courtney
d. Johnson, Courtney
Jones, Courtney
3. What is wrong with this code snippet:
if(p2.lname == p3.lname) System.out.println("p2 has the same last name as p3");
a. nothing, it prints the message
b. lname
is defined as private, so it cannot be used in the main method
c. the code must use the .compareTo(Object o)
method
d. the code must use the .equals(String str)
method
Solution to the Try This activity:
Listing 17.3 Solution to Try This Activity
1 package lesson17; 2 public class Lesson17 { 3 public static void main(String[] args) { 4 BankAccount ba1 = new BankAccount("Ande", "Withers", 1234, 1000.40); #A 5 BankAccount ba2 = new BankAccount("Ande", "Withers", 1234, 1000.40); #A 6 BankAccount ba3 = new BankAccount("Ande", "Withers", 1234, 100.40); #A 7 if(ba1.equals(ba2)) System.out.println("ba1 is equal to ba2"); #B 8 if(ba1.equals(ba3)) System.out.println("ba1 is equal to ba3"); #C 9 } 10 } 11 class BankAccount { 12 private String fname, lname; 13 private double balance; 14 private int accountNumber; 15 public BankAccount(String fname, String lname, int accountNumber, 16 double balance) { 17 this.fname = fname; 18 this.lname = lname; 19 this.accountNumber = accountNumber; 20 this.balance = balance; 21 } 22 public boolean equals(Object obj) { //#D 23 BankAccount ba = null; //#E 24 if(obj instanceof BankAccount) { //#F 25 ba = (BankAccount)obj; //#G 26 } 27 if(ba != null) { //#H 28 if(this.fname == ba.fname && this.lname == ba.lname && //#I 29 this.accountNumber == ba.accountNumber && 30 this.balance == ba.balance) 31 return true; //#J 32 } 33 return false; //#K 34 } 35 }