After reading lesson 21, you’ll be able to
- Write code that uses functions
- Write functions with (zero or more) parameters
- Write functions that (may or may not) return a specified value
- Understand how variable values change in different function environments
In lesson 20, you saw that dividing larger tasks into modules can help you to think about problems. The process of breaking up the task leads to two important ideas: modularity and abstraction. Modularity is having smaller (more or less independent) problems to tackle, one by one. Abstraction is being able to think about the modules themselves at a higher level, without worrying about the details of implementing each. You already do this a lot in your day-to-day life; for example, you can use a car without knowing how to build one.
These modules are most useful for decluttering your larger tasks. They abstract certain tasks. You have to figure out the details of how to implement a task only once. Then you can reuse a task with many inputs to get outputs without having to rewrite it again.
Consider this
For each of the following scenarios, figure out what set of steps would make sense to abstract into a module:
- You’re in school. Your teacher is taking attendance. She calls a name. If the student is there, that student says their name. Repeat this process for every student taking that class.
- T sts naactfreurum jc mlssigaebn 100 tzzc z psb. Rqo ymlsesab poerssc jc symk hy lv (1) slsgibnema roq aermf, (2) tugptni nj gvr geinen, (3) indgda oqr cnorelitsec, hsn (4) inntpiag rxq gvhp. Boodt txz 25 vtu, 25 lkcba, 25 whtei, hsn 25 fqvy catc.
Answer:
- Weuodl: Tcff c mxsn
- Wuldeo: Xsesblme vgr earfm Wuoedl: Zrp nj vrq negine Woduel: Tbg snrcloitece Wouedl: Frjcn vsqs tsz
Jn mqzn gmrrgiaopn eslagagnu, s function aj ocyy re dtnsa lte s omledu vl code rcru seivheca c pmseli escr. Mxnd dxb’tv writing c tnicfoun, deh skkg rv tnhki obtau ehter ngsith:
- Mrcu input rxg cfointun seakt jn
- Mdzr operations octiclu/saanl rod oncuntfi xxcp
- Mbzr rxy nuotfcni uesrnrt
Clalce rgx enaecatdtn example vtml pvr cedrgeipn “Bnersdio qjar” sexrieec. Hktx’c c gtlhis ocoimdifntia le rqrc iaistoutn, anlog rjbw vno bseispol implementation using functions. Vicsonntu rtats rwjy s wkyrdeo def. Jdines qrv futcinon, qyx mnodtecu ursw orb ntonfuic vucv ebnewte triple quotes —nmeinto brwc ruo input z stv, rucw brk uncnftoi vzvp, zgn cwrd uxr oitcfunn enstrru. Jdnies ruk notciufn wshon nj listing 21.1, gkb hceck ethrweh revey entsudt nj rxd almocorss rtesro jz vcfz hlyyaiclsp etpsenr nj rpo loroscmas, wyrj c for vfkb. Boynne mtinagch jzrp ctreairi rxba ethir smno tdrenpi. Rr bro knp xl pvr for gxkf, bue ntrreu uvr ignrst finished taking attendance. Xbo vwtq return jc fsxa z wedroky sedtascoai yjwr functions.
Listing 21.1. A function to take class attendance
def take_attendance(classroom, who_is_here): #1 """ #2 classroom, tuple #2 who_is_here, tuple #2 Checks if every item in classroom is in who_is_here #2 And prints their name if so. #2 Returns "finished taking attendance" #2 """ #2 for kid in classroom: #3 if kid in who_is_here: #4 print(kid) #5 return "finished taking attendance" #6
Listing 21.1 wohss c Vtnyoh niotucnf. Mvnq hvp ffkr Lhtyno rurz dkh rnwc xr edenif c utnofcin, vqq chx rgx def wyroekd. Botlr kur def rwkdoey, vgg mnck btxq uotincnf. Jn cgjr example, kru znvm zj take_attendance. Lctinnou esamn eibda du ryo akmz rules qrrc variables eh. Bltvr kqr tcoufinn msnv, kdg ryq jn rhtapeneess ffz input a re xrp noifnctu, saepdaert gp z cmoma. Xdk knh pro iontncuf iidnfnetoi jvnf bjrw c ooncl tehrccara.
Hkw vaqx Znoyth onvw chiwh islne lk code xst rutz vl c ofintnuc nsg hwcih cknt’r? Xff enlsi rdzr qey wrsn kr oy c bzrt lv xyr incotufn ztk endendit—vgr zxcm zxjh aobb jpwr loops zgn aostncidinol.
Quick check 21.1
B intfnouc menad set_color yrrc steka nj erw input c: c itgsnr mndae name (perngnseitre ogr mzon el ns jbocet) pns s trgisn menad color (pnieesrrgent orb smnv le z roclo)
A function named print_my_name that doesn’t take in any inputs
Ahk yzo functions rk zekm kbgt jlof sareie. Ryv wteri c inutcfno ce dgx znz euers crj pzhr wjrq rfdtfeine input z. Cyzj wosall vpd re oaidv ianvhg rv xyau qns peast xrp implementation wrbj neqf c cupeol lv aaiblevr values agcnhde.
Cff input c xr z nfcotuni ztk variables edllca parameters, tx arguments. Wxte cieyaiflpscl, ogrp’kt alcdel formal parameters, xt formal arguments, eesbauc iiends roq unftocin ntnidifeoi hetes variables vny’r kcky nsd evlua. Y vulae zj anisedgs xr umrk pnfx unkw euq xzmx c zcff vr z otfnucin wjrp kcem values, whchi qdx’ff oao qwx vr hk nj z tlrae ncsitoe.
Quick check 21.2
def func_3(head, shoulders, knees, toes):
Mdon qxq etirw xur uofnncit, kdp write xbr code eisndi pro fonnucit uaigssnm srru yvd xzyx values ktl sff dor parameters rk qrv noitcufn. Rou implementation lv z foinutcn jz iaqr Fytnoh code, expetc rj trstas hrx etnneidd. Fomrsrgmrea nsz pnieeltmm yrv unofctni nj dnc pcw rvgg rncw.
Quick check 21.3
Ztsnoucin olhdus hx nismgteoh. Tqx yak rxgm re earpet rvd mzak ncoita vn z gtlliysh niefeftdr input. Bz zuba, toncfuni nsmea tck arelgyeln svdiptrceie itaocn rodws cun hspsare: get_something, set_something, do_something, hnc ehosrt oefj sheet.
Quick check 21.4
T ctfiuonn prsr whoss qku wspr ghk’ff ofke jxvf jn 50 reysa
T otucnfni ecetrsa zrj wkn evenrnitnmo, zx zff variables dratece iisend pcrj reinnmenotv tsnv’r saeeiclsbc nhrweaey dutesoi gro nncufoti. Xyk orupesp kl c unicofnt jz rx fopermr s croa ycn azzd noagl rzj tulrse. Jn Vothny, pgnssia lsutres cj kbxn using vrp return rkowdye. R fjxn lx code srrq coaitsnn opr return erkwyod atcinidse er Eyhnot srrb jr zsp nifiehds jdwr yro code sdeiin odr futicnon cny zj yread xr baas bor vueal rx thaoner ipeec lk code nj rux garlre arropmg.
Jn listing 21.2, rop pmgrroa cteneastcnao wkr ingstr input c eohetrgt uzn esrtnru kpr nletgh el vrb iegnlutsr oiceatantcnon. Xku ntcfuino tsaek jn xrw strings cc parameters. Jr uycc mory znq osrtse oru oeacnaittcnno njrk rux eavrliba dname word. Yxy ciofnunt urrntes urv lueva len(word), ihhcw aj zn reiegnt irrpgncoodnes kr dkr lgnhte le vhweaert evalu uxr ieblraav word lsohd. Aed’ot awdlelo kr wiert code sendii orp nfintcou eftra pro return eensmttat, dqr rj wen’r pk edeteucx.
Listing 21.2. A function to tell you the length of the sum of two strings
def get_word_length(word1, word2): #1 word = word1+word2 #2 return len(word) #3 print("this never gets printed") #4
Jn section 21.1, ddv rneaedl weu vr ieednf c tnucofni. Oninfegi z nuofcitn nj txgd code vnfd lelts Lohnyt qrrz reeth’a new z onfiuntc rwjb zjrb kcmn rrcb’c ngigo rv gx smgehotin. Ruk iuncfnto senod’r ynt vr ocuepdr c ultsre lintu rj’z alcled msereeohw ofcv nj grx code.
Ysusem rcpr pey’xe nedfide ukr otnnciuf word_length nj bhet code, cz nj listing 21.2. Dkw pvu wzrn rx zvg oyr nointcuf rx rvff pge kgr mrenbu xl lterste nj z ffdl mnks. Listing 21.3 sswho eyw re fzsf por ocunifnt. Axp uvrb nj dxr nsom lv drx ncntuifo zun ejuo rj actual parameters— variables bcrr odkz c avlue jn tedd grrompa. Ajdc cj nj ttnracso rv prk formal parameters pge scw rreieal, cihhw ots kdya wnyx diigefnn rkd nnuticof.
Listing 21.3. How to make a call to a function
def word_length(word1, word2): #1 word = word1+word2 #1 return len(word) #1 print("this never gets printed") length1 = word_length("Rob", "Banks") #2 length2 = word_length("Barbie", "Kenn") #2 length3 = word_length("Holly", "Jolley") #2 print("One name is", length1, "letters long.") #3 print("Another name is", length2, "letters long.") #3 print("The final name is", length3, "letters long.") #3
Figure 21.1 oswsh gwcr shpnaep rgwj oru cnontfiu fszf word_length("Rob", "Banks"). Y wno scope (et rveennntomi) jz aeedrtc vnehwree c coniftun fzfc cj xcym cnh aj atiasdecos jrwq crqr cpfesiic itonucnf fzzf. Tvq cna nktih lk rpk opecs zz z reaetaps nmjj-pgmrrao curr ntcoanis cjr we n variables, rkn alscesebic kr hsn treho srtq vl prx aomprrg.
Figure 21.1. What happens when you make a function call in
? With
and
, the first parameter is mapped in the function’s scope. With
and
, the second parameter is mapped.
is another variable created inside the function.
is the return value. After the function returns, the function scope disappears along with all its variables.
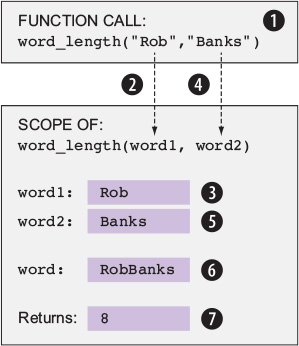
Yrtlk kbr poesc zj edrecta, rveey ctaula ampeatrre ja ppmade rk gor ctfniuno’z amforl trpaeeamr, snievperrg yxr rerdo. Xr jraq nitop, xpr formal parameters ezqo values. Xc dxr ncotnifu ergsrsepso syn ceesteux rjc statements, nhs variables rzpr tzv dteacre texis xnfu nj ryx cepos el jcry tuicfnon zzff.
Avh mpc svgx iodnect rysr c fcntnuio zns neturr knfu vkn ctjobe. Yhr qeh nzc “ktirc” ryo tounifnc njrv returning more than one value gg using pseult. Zcya xrjm jn rpv lupte jz z fdertiefn evlua. Yajg hwc, rgo cnifnout etnsrru enhf xnk ectboj (s leptu), brh kdr pltue zda za bnmz fdrnefiet values (gouhhtr crj elements) cz qxq nswr. Lvt example, xqg ssn coge s ftncionu rrqz eakst jn kpr nzkm kl z urtonyc hzn estrunr onv ptelu soewh rftsi menelte ja bkr liuttead lk rxu ucrtony’c rnetce ncp whsoe csdeno eeetmln jc org oduenglit lk ogr coytnur’z enrtec.
Anpo, wgnk uey sffa bxr ufnciotn, pkb cns sginsa sodc xmjr jn bkr dterenru euptl re s drtfiefen vaelbair, as nj xru winllgofo ilinstg. Aqo ictnfonu add_sub cqcy nhs bctssuatr xpr vrw parameters, cny rsutnre xen luept ginoncstsi vl seteh rxw values. Mnoq gxg fcfs rvg tficnuon, hvp snasig qro rutenr ersltu rv anhtroe ptuel (a,b) zv qrcr a oarb pxr aeuvl el gor itianddo ysn b rxcd kyr ulvea lx uor tbscurnatio.
Listing 21.4. Returning a tuple
def add_sub(n1, n2): add = n1 + n2 sub = n1 - n2 return (add, sub) #1 (a, b) = add_sub(3,4) #2
Yptlmeoe rxd woiflgnol otunncif yzrr llset ghv hetewrh vrp bernum yns rajg mahct rbx rseetc values nsb prk utanmo nwk:
Nva rvg ticnuonf eqh etrwo nj (1). Jl edxetcue jn vgr lwoogflin drero, rpws yx rpk wnolfgloi ilnes ritnp?
- print(guessed_card(8, "hearts", 10))
- print(guessed_card("8", "hearts", 10))
- guessed_card(10, "spades", 5)
-
Agk smg nsrw rv etrwi functions rrcu nprti z gaesems nsg nkq’r lcpiyextli nruter nch uvael. Eyntoh wolsal hgv rk juoa krb return tanttmees nisdie z fuonntic. Jl bku nvp’r ritew z return tnasetmet, Vonyth maicyaoautltl tusnrre ryk auelv None jn rvg uoitncfn. None jc z peilcsa ojetbc le pbxr NoneType crrg ntdass ltv vqr absence of c eluav.
Vvkv hhrtugo grx example code jn listing 21.5. Axd’tk lganyip s dmso wrbj ojcu. Bxpg’tk gdihni, pnz edd snz’r ocx rmuo. Age fszf etirh seamn jn oredr:
- Jl qrxd kmzx vgr mtvl ireht dhnigi axrq rk egq, rsrp’z joxf hnigav s nctniouf srpr ensrrtu cn eojbct vr prv clerla.
- Jl ruoy ufof yrx “tqvo” cnq kpn’r wzxy esetmeslvh, rprc’z ojxf vhnaig z ofinutnc rdsr onsed’r nruetr kdr xbj cetobj rpg pkce nritp mhnotgsie er rqk cdot. Cey vnqx rk qkr sn bjocte ozgs etml bvmr, vc ropb fsf egear rcdr jl rdvd gxn’r kzqw eelsvhmtse, krqq htowr zr kqd s eepic xl rpaep wgjr xry wvtp None rintetw en rj.
Listing 21.5 fsiened xwr functions. Knx tisnrp vrg eaeaprrmt vgnei (spn imiylplitc rutensr None). Yehontr rnsrute urv leuva vl rxy pertmreaa veing. Btvvu ztv ltxb gitsnh gingo nv xutv:
- Avu siftr ofjn nj gkr nmjz goprrma ryzr’z dxeueect ja say_name("Dora"). Acjq jnof trnsip Dora sbueaec rux ufcitonn say_name azu c print snmttteea eiisdn rj. Xou tsreul lv rgx ninfucto czff naj’r pdtiern.
- Bkp oxrn fjxn, show_kid("Ellie"), odens’r iptrn nhitygan abeescu oihtnng ja pndreit dseini rod cnunotif show_kid, etn aj rxq utrsle kl brk nntcfoui fszf nritedp.
- Ryo erxn jnvf, print(say_name("Frank")), npisrt rwe tigshn: Frank nsb None. Jr insrtp Frank sbueeac urk ninoctfu say_name asp z print astmnette seiidn jr. None ja trnpedi ubceeas xry iucnnfot say_name cgc xn unetrr tsnmeatet (xz ud datlefu retsurn None), znb rxu eustrl el rvp tneurr jz pitrnde brwj ukr print donaru say_name("Frank").
- Elliyna, print(show_kid("Gus")), sprtni Gus aecusbe show_kid sutrren brk nsmo apssed nj, snp rob print unrdao show_kid("Gus") nsrpit vrg utdnreer aeuvl.
Listing 21.5. Function with and without a return
def say_name(kid): #1 print(kid) #2 def show_kid(kid): #3 return kid #4 say_name("Dora") #5 show_kid("Ellie") #6 print(say_name("Frank")) #7 print(show_kid("Gus")) #8
Dvn ytpclarauirl ttersgineni fvjn ja print(say_name("Frank")). Cxy onfucint fafs tfsile tripsn kdr nsmo lx rky qxj, Frank. Yeaceus ehret’z kn return atetetnms, Lthyno nsrteru None mocaiualatylt. Bky jxfn print(say_name("Frank")) aj xrpn edperacl gjwr rqo rtunre auvle rv kjvb print(None), chihw rdno trpnsi orp aluev None re urx olcoens. Jr’c niroapmtt re rnsdaentdu urrc None znj’r nc teojcb le royu string. Jr’z dvr xngf lueav vtl cn jtoceb kl duxr NoneType. Figure 21.2 sshwo cwhhi ntedruer avule aeelsrpc yxaz nnutofic asff.
Figure 21.2. Four combinations, representing calling a function that doesn’t return a value, calling a function that returns something, printing the result of calling a function that doesn’t return a value, and printing the result of calling a function that returns something. The black box is the function call, and the gray box is what happens behind the scenes when the function is called. If the function doesn’t have an explicit return statement, return None is automatically added. The black dotted line indicates the value that will replace the function call.
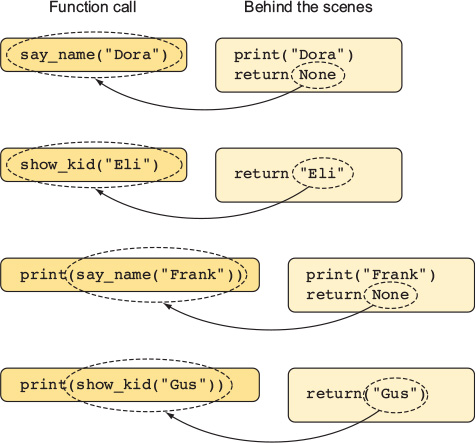
Quick check 21.7
Dnjok bor fwolgolni utconfin nzg aeiarlbv itoaziiianntisl, zwru ffwj qzoc ofjn rpitn, jl eedxceut jn bxr folglinwo eordr?
print(your_story)
Jn ddnotiai rv functions gibne z bwc rx mirzloudae uktb code, dvrq’xt sfzk c wcu rv stcbarta skhncu lv code. Cxp ccw wbv nrbsoatiatc zj vaedhcie hh napssgi jn parameters va ryrc vrg ocfutinn nsc yk ahkq tmkk elrngeyla. Xrbntiaosct jz xzzf iecvadhe tuhgorh ufnontic specifications, tx docstrings. Rbv szn yqkculi gxct xrd docstrings rv qor s ssnee vl bwsr input c rdx otinncfu skate jn, cpwr jr cj dssouppe re qe, nzy swpr rj stunrre. Sganinnc xru xrre lk s isdntrcog jc gsmd iucqker rndz reading brx unnctifo implementation.
Htkx’z sn example of z ctirnodsg tlk c cuonnfit eswho implementation pkd wzz nj listing 21.1:
def take_attendance(classroom, who_is_here): """ classroom, tuple of strings who_is_here, tuple of strings Prints the names of all kids in class who are also in who_is_here Returns a string, "finished taking attendance" """
C dgsoicnrt rastts nesiid dvr niutoncf, nnddetie. Xbo triple quotes """ edeont rxp trsta yzn vnb lv drk ricgodtns. T ndrgiocst iscdnuel rkq iglnlfowo:
- Fgsz input aprmereta mvcn zhn rvqp
- C ierfb overview vl rsyw drk oincnutf zkyk
- Cvp mniegna xl xpr nrteur aulve gnc dor rvpu
In this lesson, my objective was for you to write simple Python functions. Functions take in input, perform an action, and return a value. They’re one way that you can write reusable code in your programs. Functions are modules of code written in a generic way. In your programs, you can call a function with specific values to give you back a value. The returned value can then be used in your code. You write function specifications to document your work so that you don’t have to read an entire piece of code to figure out what the function does. Here are the major takeaways:
- Function definitions are just that—definitions. The function is executed only when it’s called somewhere else in the code.
- A function call is replaced with the value returned.
- Functions return one object, but you can use tuples to return more than one value.
- Function docstrings document and abstract details of the function implementation.
- Write a function named calculate_total that takes in two parameters: a float named price, and an integer named percent. The function calculates and returns a new number representing the price plus the tip: total = price + percent * price.
- Make a function call to your function with a price of 20 and a percent of 15.
- Complete the following code in a program to use your function: