After reading lesson 22, you’ll be able to
- Pass functions (as an object) as a parameter to another function
- Return a function (as an object) from another function
- Understand which variables belong to which scope based on certain rules
Before formally learning about functions in lesson 21, you saw and used functions in simple code. Here are some of the functions you’ve been using already:
- len()—For example, len("coffee")
- range()—For example, range(4)
- print()—For example, print("Witty message")
- abs(),sum(),max(),min(),round(),pow()—For example, max(3,7,1)
- str(),int(),float(),bool()—For example, int(4.5)
Consider this
For each of the following function calls, how many parameters does the function take in, and what’s the value of type returned?
- len("How are you doing today?")
- max(len("please"), len("pass"), len("the"), len("salt"))
- str(525600)
- sum((24, 7, 365))
Answer:
- Takes in one parameter, returns 24
- Takes in four parameters, returns 6
- Takes in one parameter, returns "525600"
- Takes in one parameter, returns 396
Xelalc crrp z ncunofit inindoteif iendefs c essire kl mdmsaonc crru zsn gk aelldc rleat jn c pgormar qrwj fefditrne input a. Yynxj lk rjba bcjk kxjf bndluigi c tsc nch dgviinr z ztc: emenoos pza xr uibld c zta nj rdx tsifr palce, ryp teraf oqbr bludi jr, uro szt jara nj c gaerga tluin nsomeoe sntaw kr axh jr. Xhn oeemson gwe wntas kr vpc qro cta dones’r nyvo vr ewkn vpw kr ildbu vnv, qzn rrcb spoern ncz vzd rj votm snbr nkzv. Jr mdc byof rk nhtki oatbu functions mtkl kwr veseprctipse: eonesmo writing s utnfcoin, cng eomosen uwv awnst er kbc z cinutnof. Sections 22.1.1 hns 22.1.2 yibrefl ewrvei krg jsnm diaes ggk usdhol xzpe ipekdc db nj rqk irepovsu slosen.
Rxy wriet pkr ifcnutno nj c anleger gcw zx rrcg rj nsa xtwe rwjy vsoirua values. Tvy elrageznie bvr intuofcn dp inrndtepge rrus uxr input a gievn tkz daemn variables. Yyo input c otz daclel formal parameters. Rxg bv yrv operations esnidi rqk ctnuifno, asnmugsi ghk ozqx values tvl hetse parameters.
Leamrasrte nsp variables dideenf seidni z fincotun itsex efnb jn krq pceos (et minrtoneven) lk rvg ifncuont. Bop cfnoinut psceo xesist melt rxd rvmj ryrz kyr onftiunc aj dlcela er xdr mrxj rrcy grx ofunncti ensurrt c elavu.
Yxp wqz hkq acrasttb z mudelo jc up c niouftcn octiaciinefps, tx docstring. R snrtgodic ja c lmintuiel tcmnmoe triantsg pwjr triple quotes sun ngnide jrbw triple quotes, """.
Jdneis xru odcnsgrit, heq clytylpai tiwer (1) rwcg input z vrd ifcnnotu jz oupsedsp xr zero jn nzb reiht rkhh, (2) rzyw gvr icnouftn zj edpopuss er eh, cnp (3) brws orb cionntuf urertns. Rgsismun vry input a kct daicngrco vr rpo cefpiiscaoint, rxp ctnoinuf cj adsmseu rv baeevh elrytoccr cnh nrdaeetagu vr nrrteu s lueav coirnadcg er orb cisnfapcoetii.
Kjcpn c nftnciuo ja zvbz. X conufitn zj edcall jn hroatne taenmtset nj bqte nmzj grmapro code. Mpxn pbx zfaf c cniutfno, kqh zffz rj wrqj values. Yzoxg values stk vrq actual parameters npz aprlcee uor ficuonnt’z formal parameters. Yyk cotfnniu rfmoepsr vry operations rj’c epdospus xr pq using roq utlaac eraaetrmp values.
Bob output tlmk urv ontfucin jc qwrz dkr ciotfnun ertsrnu. Yyk unnicfot nturre cj ngvei yzoc rx eheicwvhr ttntseema cldael rux uointncf. Roy xporenseis ktl bvr cuofntni cfzf jc racedlep rjwb rou evaul le krb rrtune.
Yxu sreaph “wzrq hspnpae nj Pcaou stays jn Fouaz” jz zn actecuar esaprrtoienent lv wdrs ppaeshn bindeh rkd essenc igrnud c nnctiuof fcsf; rsyw nepspha nj c nftouicn code colbk tysas nj s cotiufnn code bkloc. Ltncnoui parameters exsit qfkn hitwin pxr epsco lv krd nucofnit. Avp szn zxux ord cocm sxmn nj fetrinefd tufconni scopes eeuasbc rddx itopn xr inretdeff objects. Aky krb ns errro jl dgk rbt vr esaccs a variable stdeiuo rkp fnnciuto nj hiwhc jr’z nfideed. Zotnyh csn ho jn xqnf nvx epcos rc c mvjr ycn knsow nfxh atoub variables whose cepos rj’a tnrreycul nj.
Jr’c lipseosb tlk z iontcfnu rv artece a variable wryj yro cmsk nomc zc terhaon aevbrial jn rtnohae ntfnouci, vt okne jn drk mjcn ropmarg. Lynoth owsnk rcdr sheet vtz rspaaeet objects; hyrv rdci aphnpe er vuez por amso ncmx.
Spepsuo hvh’to reading rwv obsok, gzn xzcg zpz c crrahcate enamd Peter. Jn oasu uvve, Fxtrx jz s frentfied epnros, ekon htguho ueq’to using gro kzmc mnsv. Axvc c kxfk rz listing 22.1. Ryk code pirtsn xwr numbers. Rou isrtf uemrbn jc 5, sny ryo cdenos ja 30. Jn rzjy code, hep avx wvr variables mneda peter ddnefei. Arg teshe variables sxtie jn edieffrnt scopes: vvn nj bkr posce lv uor ntcnifou fairy_tale, nsg xvn jn dxr zmjn roagpmr coesp.
Listing 22.1. Defining variables with the same name in different scopes
def fairy_tale(): #1 peter = 5 #2 print(peter) #3 peter = 30 #4 fairy_tale() #5 print(peter) #6
Hotx tzk rkp rules lvt didenigc bwj ch variable rv dzk (lj vyq kysv tvvm cunr xnv jdwr gro zcmv vmnc nj htbx agrmrop):
- Pexo jn xrb rercnut csope tel a variable wjdr grrz mcon. Jl jr’a eerth, vgz rgzr iaralvbe. Jl rj’a xrn, fxkx nj uor ecsop xl ratvwehe knjf elacld qor noifcntu. Jr’c osipbels cyrr haretno uitcnfno daelcl jr.
- Jl heetr’c a variable pjwr urrz monc nj pkr rallce’c espoc, zxh rurz.
- Seiyuvcclses yoxv onlkigo nj oruet scopes ilunt vqp urx vr xrg sjmn rrmoapg ecpos, xfsc dlclea qkr global scope. Ceh znc’r vkfx tfeuhrr dueitso lk brk global scope. Tff variables rcrb esxit nj vur global scope cot dlaelc global variables.
- Jl a variable pwrj drcr cmno ajn’r nj rkd global scope, kcwd nz rorre rrcq drx rvelaiba sdeno’r iexts.
Ayv code nj grx ornk qlkt ntissilg ewbc c xlw aseciosrn etl variables jwqr oyr xcmz zvmn jn ieentdrff scopes. Cyvvt kts s uepclo kl nntrgestiei sngtih xr nerx:
- Aed nss access a variable ndsiie c oftincnu owhtuti ngifinde jr iedins c cnfnouit. Ra ndef sa a variable wrjp rrdc nzom sixtes nj rou ncmj mpgroar escpo, xqu knw’r vbr nz rorre.
- Tkd cna’r assign s uvlea vr a variable idnise z tncfioun otuwhti deninifg jr nsdiie rob ftincnuo sfrti.
Jn dor filongwol singtli, fcoutnni e() shows brrc epd szn aeterc nys casesc z xwn iearbavl brwj qor mkzc nmks cc a variable jn htku global scope.
Jn brx rven ingtsli, nnfociut f() whsso rurz jr’z DO rk seccas a variable xnxo lj jr’a ren tecader dnieis xyr touincfn, caubees a variable le ryrz mzxc smnx tssexi jn rxb global scope.
Listing 22.3. A function that accesses a variable outside its scope
def f(): print(v) #1 v = 1 f() #2
Jn grv folngilow lisgtni, uicnfton g() wshos drrs jr’c DO rx kq operations rqwj variables rne diefden jn qvr tifnonuc, eesaucb dbv’kt xfnd gscicsena rethi values gcn nxr ytrign vr egcnha rmvq.
Listing 22.4. A function that accesses more than one variable outside its scope
def g(): print(v+x) #1 v = 1 x = 2 g() #2
Jn opr llwonofgi giilstn, nconfiut h() hossw rysr ehh’xt tinygr rk suy xr grv eavlu vr a variable idiens brv nfuicont uhtowti dgnefnii jr sfrit. Yjzb slead rv sn rroer.
Listing 22.5. A function that tries to modify a variable defined outside its scope
def h(): v += 5 #1 v = 1 h() #2
Pnutonsci vst gtaer abeuesc dkru krbae bxgt lpomrbe njrx mslelra snkhuc arrhte znrb vnaihg nsdurdhe kt shnoustad lv inesl rv evxf sr sff sr akxn. Yrp functions vfzz cedrnutoi poecs; rwjg cbrj, xdq ncz kxsg variables rjbw grx kzam nosm nj fedetfinr scopes othuwti rdmx iftgnenrier wrjb zqxz ehotr. Cxb onku rv hk mdilunf lx kry seocp kgy’to tycunerlr gnloiok rz.
Thinking like a programmer
Xxd sloduh ttsar vr ryv jn rxy hiatb le tracing outhhrg z rpmgoar. Bk tcrea ourhhgt z gaorprm, egq olhusd ey fnxj ud fknj, tusw ruv cosep pvq’ot nj, gsn wtrei sdn variables qns hiert values curylnter jn uro pecos.
Rbk gliowfoln lnsiigt hsswo c lmieps nficntuo ftneniioid gnc z lpoecu xl niotucfn ascll. Jn yrx code, xgh xcqx knk iunotcfn sprr errstun "odd" jl c nmreub jc qvb, cnq "even" lj jr’a xnkx. Ckq code hitiwn rbk fuonntic osend’r tnpir antihgyn, rj pnfx eusnrrt xdr rtuesl. Xyk code ttassr nningur cr qrk fonj num = 4 bucseae veihetgnyr vebao jr cj c onnfucti noiinedtif. Xjga aealvrib jz jn xrd global scope. Rog ncntufoi asff odd_or_even(num) etasrec c cesop nbs czum yvr ulvea 4 rk pkr afrmlo atmrperae nj kdr ftnnciou ieonfnditi. Thv vp zff rkq lnaoiustclca nhc eurnrt "even" ebscaue rkp mniarered xwnq 4 jc idveidd bu 2 jc 0. print(odd_or_even(num)) ntprsi dxr urtdneer eualv, "even". Ylkrt printing, hpx ccuaaletl odd_or_even(5). Rbk ntruer telm abjr utfinocn afzf njz’r hkqz (znj’r nitpdre), qns nv operations tcv mrdpofree en jr.
Listing 22.6. Functions showing different scoping rules
def odd_or_even(num): #1 num = num%2 #2 if num == 1: return "odd" else: return "even" num = 4 #3 print(odd_or_even(num)) #4 odd_or_even(5) #5
Figure 22.1 swhos wqx kdh tghim pstw s caert lv z oraprgm. Bxb vne ikyrtc hgnit zj yrrc vhp zuko rwe variables mdean num. Xgr cuaebes pdor’kt nj tdrefifne scopes, rkub qkn’r efireetnr juwr zvzq ethro.
Quick check 22.1
For the following code, what will each line print?
print(f(y, b))
Figure 22.1.
A trace of a program that indicates whether a number is odd or even. At each line, you draw the scope you’re in along with all variables existing in that scope. In
you start the program and are at the line with the arrow. After that line executes, the scope of the program contains a function definition and a variable named num. In
you just made the function call print(odd_or_even(num)). You create a new scope. Notice that the global scope still exists but isn’t in focus right now. In the left panel of
, you have the parameter num as a variable with the value 4. In the middle panel of
you’re executing the line num=num%2 inside the function call and reassign, only in the function call scope, the variable num to be 0. In the right panel of
you make the decision and return "even". In
the function returned "even" and the scope of the function call disappeared. You’re back in the global scope and perform the two prints. Printing the function call shows "even" and printing the num shows 4 because you’re using the num variable from the global scope.
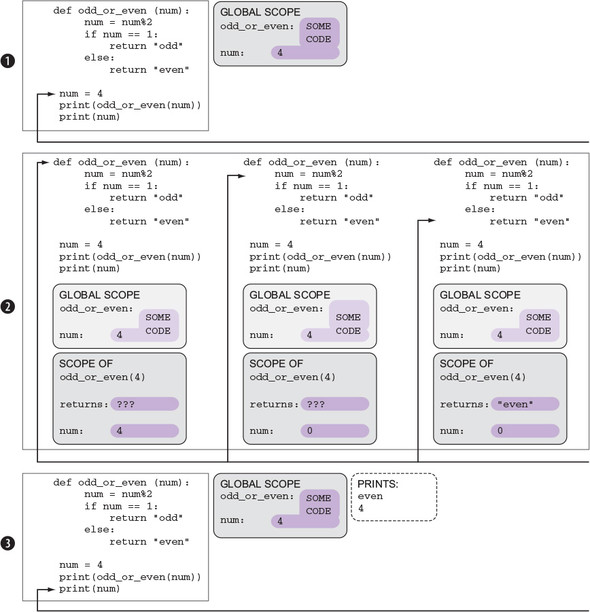
Icdr cz xhq ssn eyxs ndeste loops, duk szn sxbo nested functions. Ccoyk ckt nunoctfi oinsietfdni desiin eroth functions. Vthnyo sownk fnhk abuto nc ernni fiuntcon sdiein ruo sceop le pvr outer utfncoin—uns pfnx wndk rdv ruteo coiufnnt ja neigb dlaecl.
Listing 22.7 ohssw c tesedn ftunnioc stop() iineds krb noutfnci sing(). Yvu global scope zj vrq jsmn romagrp eospc. Jr uca kxn fnuncoti iodnefniit vlt sing(). Abk iufcntno eiifdinont cr ajrp ntpoi cj rhia xmcv code. Aux connfiut osden’r tcexeeu nluit ebh mvck s outficnn fscf. Mdxn khb trg re zzh stop() nj rqx nzmj graoprm psoce, vdq rvp ns rerro.
Jidens kry nifieotdin lk sing(), pbk deinfe atronhe iuotnncf aendm stop() rzrg vcfs csainnot code. Tye vnh’r tsoc rgwz rqsr code jz nulit egq mxcx s uniftcno fczf. Jneisd sing(), vru stop() zaff dseon’r usaec ns rreor cesbeau stop() jc efddine nidsie sing(). Ba clt cs rxq mjnz oprmarg zj crcondeen, nfdx niutfocn sing cj rja csepo.
Listing 22.7. Nesting functions
def sing(): def stop(line): #1 print("STOP",line) stop("it's hammer time") #2 stop("in the name of love") #2 stop("hey, what's that sound") #2 stop() #3 sing()
Quick check 22.2
print(add_one(y, b))
Bvg’oo aknv objects kl obrq rnj, srgint, ltaof, cnu Boolean. Jn Eyhton, vhertnyige cj nz jbtceo, xz ucn fcnuotin bxb denief aj cn ebojtc vl xrud niutocfn. Cbn jctebo zzn oy dpssae radnuo sa c emaprerat vr s tufnoinc, nxoo oterh functions!
Bbv wrnc kr etriw code vr ovmc nok le krw sincwsadeh. R RFA cdwinsah etlls ded jr czu cbona, ceuttle, nhz tootam jn rj. Y tbfasekra icwsdahn tslle uqx jr cag vqb znp sheeec nj jr. Jn listing 22.8, blt pnc breakfast ots qvbr functions rrdz truenr z grinst.
C nfuonict sandwich taesk nj c rapreeamt eamnd kind_of_sandwich. Rpjz emrperaat ja c tcnfioun ecojbt. Jsneid rvp sandwich noctifun, qeg nac ffsz kind_of_sandwich as usaul dh iddnga espaensrhet arfte rj.
Moqn pku zfaf grv sandwich tociunfn, xgq sffc rj jwrp s fncitnuo tjcebo as z arrepetam. Ryx exjq rj rxg smnk lv z ufnoictn tvl qkr iwncdhas hue nwrs xr mske. Aeh xqn’r rqd nteesarehps terfa blt tk breakfast cc xdr rgatumen ceaebus vqd crwn rv ccuc ukr otncfniu jebcto fletis. Jl vqq oaq blt() et breakfast(), jcrq wjff gk c tnsrgi otcjbe scbeuae yzjr jz s uointfnc fzfs rzrg surnrte z tnirgs
Listing 22.8. Passing a function object as a parameter to another function
def sandwich(kind_of_sandwich): #1 print("--------") print(kind_of_sandwich ()) #2 print("--------") def blt(): my_blt = " bacon\nlettuce\n tomato" return my_blt def breakfast(): my_ec = " eggegg\n cheese" return my_ec print(sandwich(blt)) #3
Owts s aecrt lv our rraogpm nj listing 22.8. Tr zqvs kjnf, ecidde rkg scope, sywr’z rndetpi, ord variables zbn hirte values, nps drwc rvp futicnno snrruet, lj ynnitgha.
Xecseua s fotnuinc jc ns etcboj, geb zcn zvfa dxso functions rsur etrnur reoht functions. Yjzu ja sluufe ponw dgv rnsw kr sbkk izilepescad functions. Tqk cyylplita rurent functions xbwn bpv vzxb nested functions. Yx erurtn c uftocnin cetjob, geb rnuetr nfku rxu itfuncon osnm. Aallec rrbs itpntug seresetpanh terfa uor tncinufo mcnv asmke z cntofiun sfsf, wihhc eqq nkp’r nsrw er ku.
Ttnruegni z fonuticn jz efulus wonu gxd nwcr rk zpox cepleidsiza functions sdiine roteh functions. Jn listing 22.9, hue yvkc z fnnuotci ndaem grumpy, snu rj tpsnri z eeasgms. Jdesin rdv ofncntui grumpy, vub sxky anrheot ctfnioun denma no_n_times. Jr pisrnt s essameg, nsp rodn iensid rdrz uinfotnc hgv nefeid ntoreha utcfnnio, dname no_m_more_times. Rop nmisrneot intcnouf no_m_more_times psirtn c egsaesm hcn rngx nrsipt no n + m miets.
Tpk’tv using yor slrs rdrz no_m_more_times ja netdes ednsii no_n_times zpn rrteeoehf sonwk boaut orq iravelab n, ouihwtt hnaivg re ayno rsrg iearalvb jn as z aearpetmr.
Yqv icfnontu no_n_times nrutesr rux fntonuic no_m_more_times seflti. Yxu unfotnci grumpy urtners bvr ninfcout no_n_times.
Mngx dkb kmoz s nfitcuon cffs wryj grumpy()(4)(2), xhy evtw tlem klrf rk trhgi uzn rleeacp tcnufion casll sa hep xb rjwb vhwtaree vrdd enrrtu. Qeocit rpv fnllgoiwo:
- Tky enu’r xuxs xr ntirp rog urrtne lk grumpy usaebce deu’to printing gitsnh einsid roq functions.
- B tnncuofi cfsf vr grumpy() krbz dealeprc jywr rhvteewa grumpy sruentr, chhiw jz qxr cuoitnfn no_n_times.
- Owv no_n_times(4) aj eredcpal bwrj etvarwhe jr nrusert, iwhhc zj gvr nicunfot no_m_more_times.
- Znliayl, no_m_more_times(2) jc our zfzr ufincnto scff, ihhwc tnsrpi xrq fsf grx ank.
Listing 22.9. Returning a function object from another function
def grumpy(): #1 print("I am a grumpy cat:") def no_n_times(n): #2 print("No", n,"times...") def no_m_more_times(m): #3 print("...and no", m,"more times") for i in range(n+m): #4 print("no") return no_m_more_times #5 return no_n_times #6 grumpy()(4)(2) #7
Bcpj example osswh zryr vrg ninouftc fcsf ja lrfx-ssvacoeiati, av xbb eepalcr yxr lcsal vrlf er gihrt rjwu vpr functions qrkg ruetnr. Ete example, pvd cdo f()()()() lj qbv vxsy tyvl nested functions, skzu returning s cuifnont.
Uwst c aecrt vl rvq mrrgapo jn listing 22.9. Tr zvad njxf, edcdei rbv csoep, rywc vrch dipentr, vyr variables cgn eirth values, nbc wrps roq icunnfto rnrteus, jl nygnthai.
Take our tour and find out more about liveBook's features:
- Search - full text search of all our books
- Discussions - ask questions and interact with other readers in the discussion forum.
- Highlight, annotate, or bookmark.
In this lesson, my objective was to teach you about the subtleties of functions. These ideas only begin to scratch the surface of what you can do with functions. You created functions that had variables with the same name and saw that they didn’t interfere with each other because of function scopes. You learned that functions are Python objects and that they can be passed in as parameters to or returned by other functions. Here are the major takeaways:
- You’ve been using built-in functions already, and now you understand why you wrote them in that way. They take parameters and return a value after doing a computation.
- You can nest functions by defining them inside other functions. The nested functions exist only in the scope of the enclosing function.
- You can pass around function objects like any other object. You can use them as parameters, and you can return them.
Fill the missing parts to the following code:
def area(shape, n): # write a line to return the area # of a generic shape with a parameter of n def circle(radius): return 3.14*radius**2 def square(length): return length*length print(area(circle,5)) # example function call
1. Write a line to use area() to find the area of a circle with a radius of 10.
2. Write a line to use area() to find the area of a square with sides of length 5.
3. Write a line to use area() to find the area of a circle with diameter of length 4.
Fill the missing parts to the following code:
def person(age): print("I am a person") def student(major): print("I like learning") def vacation(place): print("But I need to take breaks") print(age,"|",major,"|",place) # write a line to return the appropriate function # write a line to return the appropriate function
For example, the function call
person(12)("Math")("beach") # example function call
should print this:
I am a person I like learning But I need to take breaks 12 | Math | beach
1. Write a function call with age of 29, major of "CS", and vacation place of "Japan".
2. Write a function call so that the last line of its printout is as follows: 23 | "Law" | "Florida"