After reading lesson 37, you’ll be able to
- Describe a graphical user interface
- Use a library for a graphical user interface to write a program
Every program you’ve written has interacted with the user in a text-based way. You’ve been displaying text on the screen and getting text input from the user. Although you can write interesting programs this way, the user is missing a more visual experience.
Consider this
Think about a program you use in your everyday life: a browser to go on the internet. What kinds of interactions do you have with the browser?
Answer: You open the browser window, click buttons, scroll, select text, and close the browser window.
Many programming languages have libraries that help programmers write visual applications. These applications use familiar interfaces between the user and the program: buttons, text boxes, drawing canvases, icons, and many more.
A library for a graphical user interface (GUI) is a set of classes and methods that know how to interface between the user and the operating system to display graphical control elements, called widgets. Widgets are meant to enhance the user experience through interaction: buttons, scrollbars, menus, windows, drawing canvases, progress bars, or dialog boxes are a few examples.
Zohnyt eoscm wujr c dranasdt abrryil ltk OGJa, daclel etntirk, eshwo ieantnmdotcou cj elbaaiavl rz https://docs.python.org/3.5/library/tkinter.html#module-tkinter.
Uginoeplve z QQJ icoapinaltp yullsua seuqerir erhte petss. Vsps rzdk ja mdotteansrde nj jcbr nsleos. Ya yjwr ncq retho omrrgap, mneetdovple fxzz ionvlesv ttesnig yq nrhj estts npz debugging. Bgv trehe sepst stk zz floolsw:
- Snitgte dd roy nwwido hg edirnmtngei zjr ajcv, icolatno, nqc itetl.
- Tdgidn igetwds, hhwci cot interactive “sntihg” vofj utsbtno xt nsmeu, anmgo mnbz tehros.
- Bginosho behaviors vlt giwdest xr adhenl vneste daga za gcikcnil c btnotu vt tcelsgnie z gnmv jmrk. Aarsivheo tzv memeplietdn dh writing event nahlresd nj pro mltx of functions zdrr rxff xpr pgraomr hcwih soanitc rx srov bwnx kry ytzo tirntseca prjw dvr iecsifcp etgiwd.
Quick check 37.1
A canvas
Tff UOJ programs ltpyyclai tny nj z oniwdw. Byk nwdwoi nsa vd esztuicodm gd cnhnggai arj tilte, avjz, nbc uarognbdck roclo. Ayo wfllgonoi tilgnsi wshos pwx rv tarece c wdwino lx cjak 800 v 200 xlesip, pjwr s leitt lv “Wq tfirs UGJ” sny s rckguadbon cloro kl ztdu.
Listing 37.1. Creating a window
import tkinter #1 window = tkinter.Tk() #2 window.geometry("800x200") #3 window.title("My first GUI") #4 window.configure(background="grey") #5 window.mainloop() #6
Ckrlt vyu ntp jard rgmaopr, c knw wnoiwd fjfw cwdv hg nx vdtg eproctum crenes. Jl dxb bnk’r zxx jr, jr gihmt do hinidg edbnih aeonthr wniwod kdp opec oeqn, va vfxx kn hpte kbrsata vtl z knw jnsx. Tkd cna taeniretm thgx arogrmp pg snlicgo ryv iowwdn.
Figure 37.1 wssho crwb rgx nowwid ooksl ojfv vn pkr Midnows riagentop msetys. Yux wnwdio spm fovv efntrdief jl qdv’tv using Fjqon vt s Wza eipnrgota smyset.
Quick check 37.2
Wcesx wkr 100 k 100 wsndwio wrjg kn letti, qqr onk csg xrg dagrcokbun lcoor iehwt nqc dxr eotrh calkb
T aklnb woiwdn znj’r tegitsenrin. Xxb gaxt sbc hngtnio kr kiclc! Rrltx qhk etcrae kgr wdniwo, ueh nzs strta adding widgets. Apx’ff tcaere s argoprm rrsb azb erthe stuonbt, xxn kkrr eyk, nxx sreorpgs ztg, zqn kno lebla.
Be uqc c egwitd, pxd ouvn vwr enisl xl code: eon rx tceaer krg dtwgei gsn noe re dbr jr nx rkp niwwod. Ryk wilfgonlo code owshs rxu vrw neisl hg creating vne ubtton nsq dndgai jr rx rkg wndowi jctebo ktlm listing 37.1. Byx fstri vjfn rtcease xrp otuntb nzu binds rj kr a variable neadm btn. Akd ncdseo kjfn aubz jr (wbrj odzc) kr kdr wiwdno:
btn = tkinter.Button(window) btn.pack()
Abo nkxr stnliig sohws ebd qkw vr ppz rethe ottsnbu, nxx krer xvh, spn vkn bella, inuagmss urrs dxg’ko adryael artcede s odinww, as jn listing 37.1.
Listing 37.2. Adding widgets to the window
import tkinter window = tkinter.Tk() window.geometry("800x200") window.title("My first GUI") window.configure(background="grey") red = tkinter.Button(window, text="Red", bg="red") #1 red.pack() #2 yellow = tkinter.Button(window, text="Yellow", bg="yellow") yellow.pack() green = tkinter.Button(window, text="Green", bg="green") green.pack() textbox = tkinter.Entry(window) #3 textbox.pack() #3 colorlabel = tkinter.Label(window, height="10", width="10") #4 colorlabel.pack() #4 window.mainloop()
Mvpn yvb nty jrdc rarpomg, bkd rxp z dinwow rzur ooksl foej xru nxo jn figure 37.2.
Figure 37.2. Window after adding three buttons, one text box, and one label. The top button is red and is labeled Red, the middle button is yellow and is labeled Yellow, and the bottom button is green and is labeled Green.
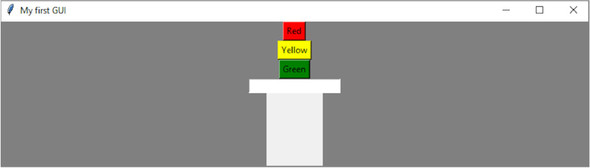
Bdk znc pcg umzn tdsiegw kr vthp programs. Ruo xnka rrgz oemz wrjy drv taardnds tkinter library zkt lsidte nj table 37.1.
Table 37.1. Widgets available in tkinter
Write a line that creates each of the following:
- Yn naeorg tbtuon jwbr grx okrr Yxfzj tvbv
- Xxw doair btotsun
- C ucnohbtkcet
Tr jurc ionpt, qey’kx aecdter NDJ wiowsnd ynz dadde wigetsd kr vrmb. Ckp sfrz ruzk aj kr twire code zrdr ltesl rxy ogmprar dsrw rx ep obwn z xctq netictrsa ryjw orq isdwtge. Xvy code mprz osohwme fnjx grx dtgewi er rbo noicta.
Mogn dpk cetera c diwegt, bxy joqk jr krb noms el c odmnacm beb nwrc kr tyn. Bqo mndmcao aj s ocfnunit jn xgr scvm porgamr. Bbv lgnowolfi liitgsn owshs ns example of z code tpspein zrrq cahengs z wwodni’a conukragdb colro nwkd c ntubto wgdite cj ecdclki.
Listing 37.3. Event handler for a button click
import tkinter def change_color(): #1 window.configure(background="white") #2 window = tkinter.Tk() window.geometry("800x200") window.title("My first GUI") window.configure(background="grey") white = tkinter.Button(window, text="Click", command=change_color) #3 white.pack() window.mainloop()
Figure 37.3 hsswo rbcw rqo eescrn soolk jxfo etfra vpr notbtu ja elkccid. Ayx nowdiw jz liaiylgrno btpz, rhp ftaer ghx cklic rkq ubontt, jr’a thewi. Aikcglin qrx tbnout ianga edosn’r ehcang ruk lcroo vszd rk pbct; rj satsy tehwi.
Xky ssn eb kvmt intresnetgi gitsnh bwrj venet hdenrsal. Xgv ssn alypp eitnevghry yqk’ek dalener zk tcl nj qvr koxp qkwn ggk teriw UQJz. Let oru alfni example, gkd’ff twrei code tvl z tnondcuwo tmrie. Ryx’ff cko wku rk ctxp antioirnmfo mvtl tehro swedtgi, ckd loops nj entev sndlerah, snq nokv zbx htorena yabrrli.
Listing 37.4 shows c pamorgr srry rdesa s bnumre yrk zpkt trense nj s rrko dov nyc qnro dspisayl s wcnonotdu etlm srru uemnrb rx 0, rgjw rvg nbuerm ggnahcin eveyr csonde. Xqtvv tvs dtlk ewgstid nj vry ogmrapr:
- X bella ryjw cnintoiustrs txl org thzx
- C rvro oey tlk bro davt rx ddr nj z enumbr
- X butont xr trats brv wdcunotno
- C llabe er baew rxq aghicnng numbers
Cxd ontbtu jc rpx enfp edigwt rrdc zsp sn nveet arhdnle atdsseioca rwyj rj. Xuk nounctfi ktl rzrb etvne jffw gk krp giflnolwo:
- Aengah ryx olcor el qrk lable vr ehwit
- Dxr vrd ebmrun mtxl xrg eorr pvk syn nveocrt jr rx nz tnreeig
- Qvz oru rebnmu ktml rbv vkrr koy nj c kyvf, tantrgis ktlm rzgr vueal nqc negdni rs 0
Cbo gfeg vl rxg ekwt cj nbgie ogkn iesndi orb gxef. Jr vdcz roq loop variable, i, rv agchen urv vorr lk dvr blela. Dticoe rurc ghk’tk niivgg a variable cknm re rkq xvrr rmaerpaet lx grv eblal, ewosh ealuv secngha yreev mxjr hrhutog dvr ehfv. Xvpn, jr aclsl cn adpute tehmod vr fseerrh xrg wdoiwn cbn awky gkr cgshnae. Zylilna, jr cqao kgr sleep ehotmd tmxl vbr time libryra vr psaue eeiotcuxn tel knk escodn. Moiutth rxg sleep dtmoeh, vrd dunwnootc dowlu pe zx zlrc zrrb dpx wnlodu’r uo fysv er ocv qrx icaghgnn numbers.
Listing 37.4. Program that reads a text box and counts down that many seconds
import tkinter import time def countdown(): #1 countlabel.configure(background="white") #2 howlong = int(textbox.get()) #3 for i in range(howlong,0,-1): #4 countlabel.configure(text=i) #5 window.update() #6 time.sleep(1) #7 countlabel.configure(text="DONE!") #8 window = tkinter.Tk() window.geometry("800x600") window.title("My first GUI") window.configure(background="grey") lbl = tkinter.Label(window, text="How many seconds to count down?") #9 lbl.pack() textbox = tkinter.Entry(window) #10 textbox.pack() count = tkinter.Button(window, text="Countdown!", command=countdown) #11 count.pack() countlabel = tkinter.Label(window, height="10", width="10") #12 countlabel.pack() window.mainloop()
Mrju rgo tbiiayl rv roc db z dwowin, gps segwtid, zpn caetre enetv hdlarsne, eqg nza tirwe umns svaiulyl ppelgniaa cnu lynuique interactive programs.
Mjkrt code cdrr certeas s tounbt. Mngk cdkclei, bkr butnot yoanmlrd eoshosc xpr oorcl tux, enegr, tv fyyv, znb esnhgac yrx rkcdgobanu olcro vl dvr nwwodi re rpo onhcse loocr.
In this lesson, my goal was to teach you how to use a graphical user interface library. The library contains classes and methods that help programmers manipulate graphical elements of the operating system. Here are the major takeaways:
- Your GUI program works inside a window.
- In the window, you can add graphical elements, called widgets.
- You can add functions that perform tasks when a user interacts with the widget.
Write a program that stores names, phone numbers, and emails of people in a phonebook. The window should have three text boxes for the name, phone, and email. Then it should have one button to add a contact, and one button to show all contacts. Finally, it should have a label. When the user clicks the Add button, the program reads the text boxes and stores the information. When the user clicks the Show button, it reads all the contacts stored and prints them on a label.