2 Getting Started with Testing
This chapter covers
- Basics of unit testing
- Basics of Go testing framework
- Writing idiomatic tests and code in Go
- Adding a URL parser package to the Go Standard Library
Let’s go back in time. The Go team at Google is busy writing the Go Standard Library. You, the programmer, recently joined the team. At Google, other developers in a team called the Wizards are working on a redirection service.
When their program receives a web request, they check the URL to see if it's valid, and if the URL is valid, they want to change some parts of it and redirect the web request to a new location. But they realized that there isn't a URL parser in the Go Standard Library.
So they asked you to add such a URL parser package to the Go Standard Library. You know the basics but don't yet know how to write and test idiomatic code in Go. I'll work throughout the chapter to help you write idiomatic code by implementing and unit testing a new library package called url from scratch.
Figure 2.1 Parsing a URL
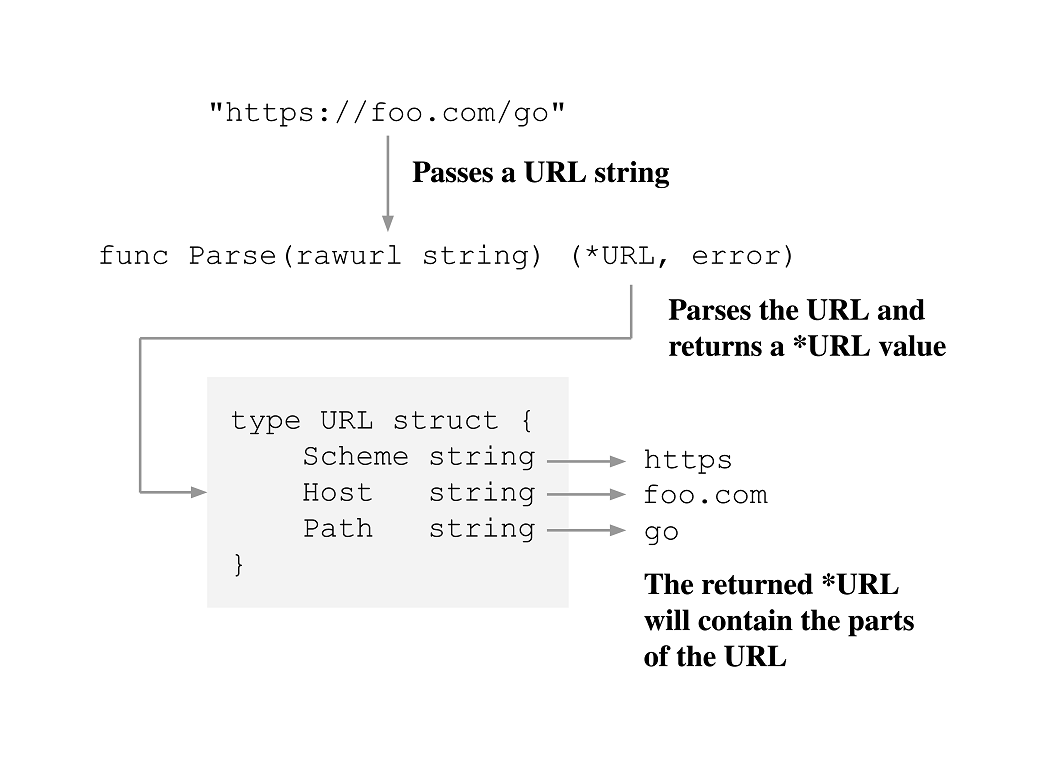
- Parse a URL to check whether it's a valid URL
- Separate the URL into its parts: Scheme, host, and path
- Provide an ability to change the parts of a parsed URL
As you can see in Figure 2.1, the code passes a URL string to the Parse
function. Then, Parse
parses it, creates a new URL value, and returns a pointer to the URL value. It returns a pointer so that you can change the fields of the same URL value.