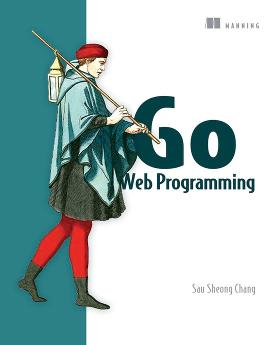
About this Book
This book introduces the basic concepts of writing a web application using the Go programming language, from the ground up, using nothing other than the standard libraries. While there are sections that discuss other libraries and other topics, including testing and deploying web applications, the main goal of the book is to teach web programming using Go standard libraries only.
The reader is assumed to have basic Go programming skills and to know Go syntax. If you don’t know Go programming at all, I would advise you to check out Go in Action by William Kennedy with Brian Ketelsen and Erik St. Martin, also published by Manning (www.manning.com/books/go-in-action). Another good book to read is The Go Programming Language (Addison-Wesley 2015), by Alan Donovan and Brian Kernighan. Alternatively, there are plenty of free tutorials on Go, including the A Tour of Go from the Go website (tour.golang.org).
The book includes ten chapters and an appendix.
Chapter 1 introduces using Go for web applications, and discusses why it is a good choice for writing web applications. You’ll also learn about key concepts of what web applications are, including a brief introduction to HTTP.
Chapter 2 shows you how to build a typical web application with Go, taking you step by step through the creation of a simple internet forum web.