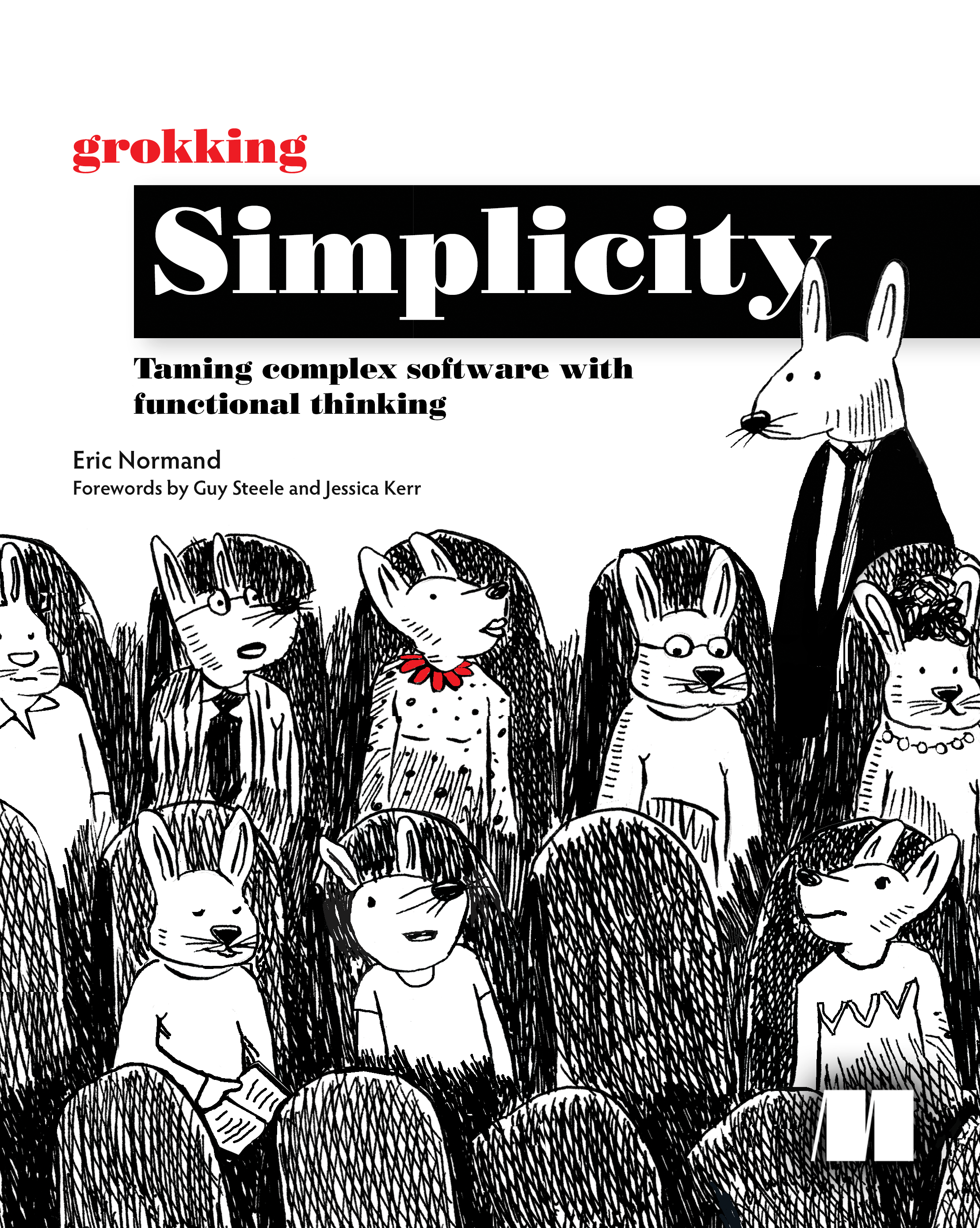
foreword
Guy Steele
I’ve been writing programs for over 52 years now. I still find it exciting, because there are always new problems to tackle and new things to learn. My programming style has changed quite a bit over the decades, as I learn new algorithms, new programming languages, and new techniques for organizing my code.
When I first learned to program, in the 1960s, a well-accepted methodology was to draw a flowchart for the program before writing actual code. Every computation was represented by a rectangular box, every decision by a diamond, and every input/output operation by some other shape. The boxes were connected by arrows representing the flow of control from one box to another. Then writing the program was just a matter of writing code for the contents of each box in some order, and whenever an arrow pointed anywhere but the next box you were about to code, you would write a goto statement to indicate the necessary transfer of control. The problem was that flowcharts were two-dimensional but code was one-dimensional, so even if the structure of a flowchart looked nice and neat on paper, it could be hard to understand when written out as code. If you drew arrows on your code from each goto statement to its destination, the result often resembled a mound of spaghetti, and in those days we indeed talked about the difficulties of understanding and maintaining “spaghetti code.”