Chapter 11. Transactions and concurrency
In this chapter
- Defining database and system transaction essentials
- Controlling concurrent access with Hibernate and JPA
- Using nontransactional data access
In this chapter, we finally talk about transactions: how you create and control concurrent units of work in an application. A unit of work is an atomic group of operations. Transactions allow you to set unit of work boundaries and help you isolate one unit of work from another. In a multiuser application, you may also be processing these units of work concurrently.
To handle concurrency, we first focus on units of work at the lowest level: database and system transactions. You’ll learn the APIs for transaction demarcation and how to define units of work in Java code. We’ll talk about how to preserve isolation and control concurrent access with pessimistic and optimistic strategies.
Finally, we look at some special cases and JPA features, based on accessing the database without explicit transactions. Let’s start with some background information.
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
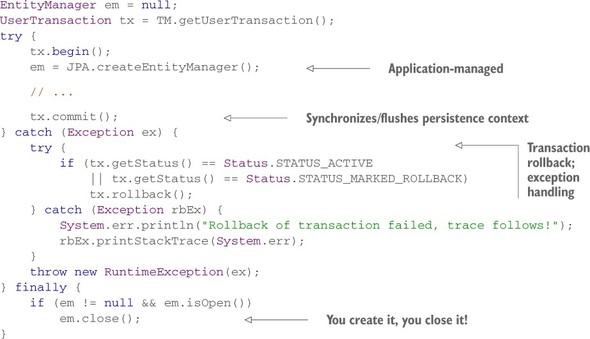
Path: /model/src/main/java/org/jpwh/model/concurrency/version/Item.java
Path: /examples/src/test/java/org\jpwh/test/concurrency/Versioning.java
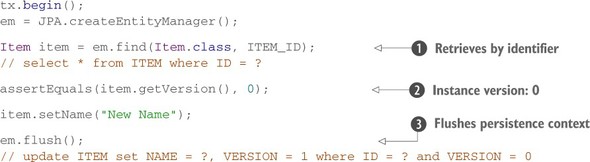
Path: /model/src/main/java/org/jpwh/model/concurrency/versiontimestamp/Item.java
Path: \model\src\main\java\org\jpwh\model\concurrency\versionall\Item.java
Path: /examples/src/test/java/org/jpwh/test/concurrency/Versioning.java
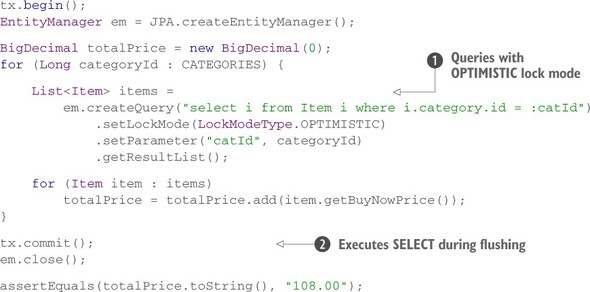
Path: /examples/src/test/java/org/jpwh/test/concurrency/Versioning.java
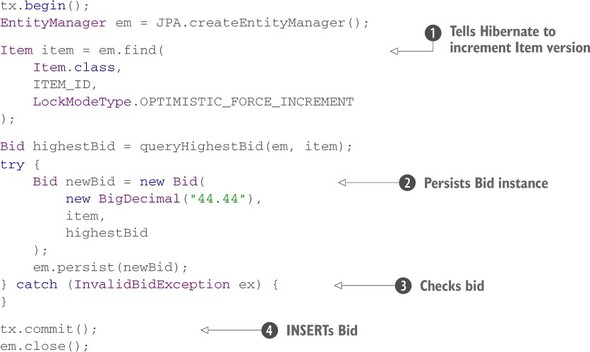
Path: /examples/src/test/java/org/jpwh/test/concurrency/Locking.java
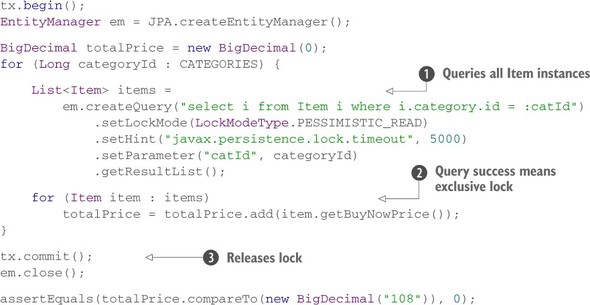
Path: /examples/src/test/java/org/jpwh/test/concurrency/Locking.java
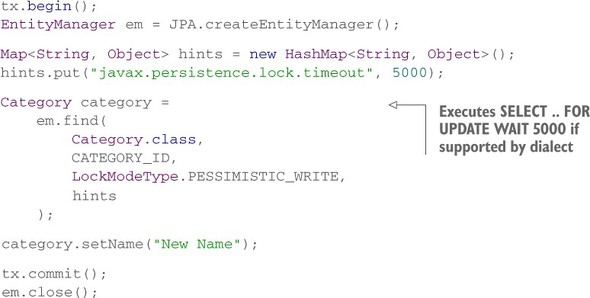
Path: /examples/src/test/java/org/jpwh/test/concurrency/NonTransactional.java
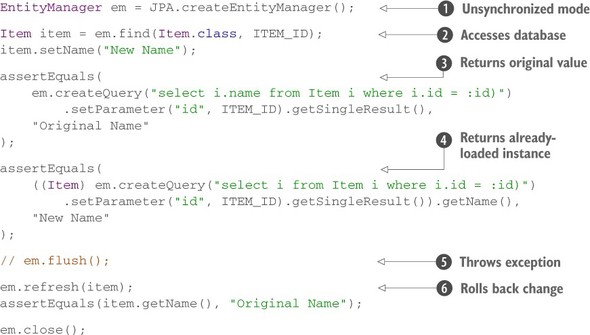
Path: /examples/src/test/java/org/jpwh/test/concurrency/NonTransactional.java
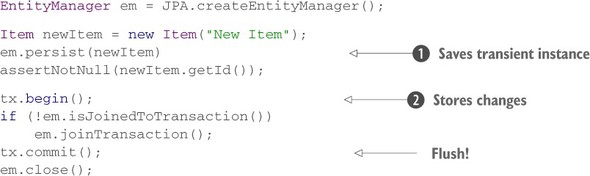
Path: /examples/src/test/java/org/jpwh/test/concurrency/NonTransactional.java
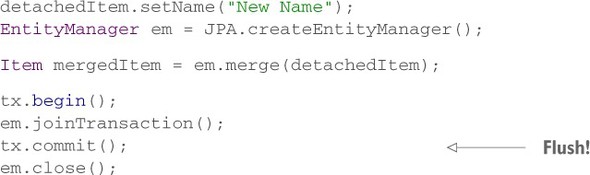
Path: /examples/src/test/java/org/jpwh/test/concurrency/NonTransactional.java
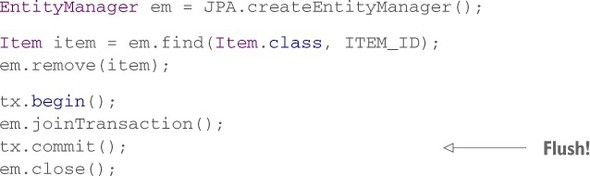