Chapter 7. Mapping collections and entity associations
In this chapter
- Mapping persistent collections
- Collections of basic and embeddable type
- Simple many-to-one and one-to-many entity associations
From our experience with the Hibernate user community, the first thing many developers try to do when they begin using Hibernate is to map a parent/children relationship. This is usually the first time they encounter collections. It’s also the first time they have to think about the differences between entities and value types, or get lost in the complexity of ORM.
Managing the associations between classes and the relationships between tables is at the heart of ORM. Most of the difficult problems involved in implementing an ORM solution relate to collections and entity association management. Feel free to come back to this chapter to grok this topic fully. We start this chapter with basic collection-mapping concepts and simple examples. After that, you’ll be prepared for the first collection in an entity association—although we’ll come back to more complicated entity association mappings in the next chapter. To get the full picture, we recommend you read both this chapter and the next.
Major new features in JPA 2
Path: /model/src/main/java/org/jpwh/model/collections/setofstrings/Item.java
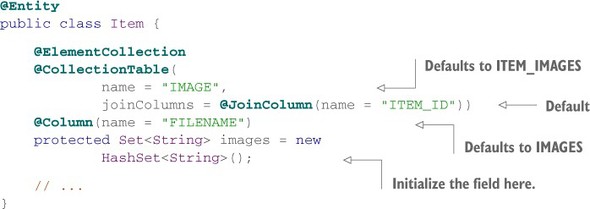
Path: /model/src/main/java/org/jpwh/model/collections/bagofstrings/Item.java
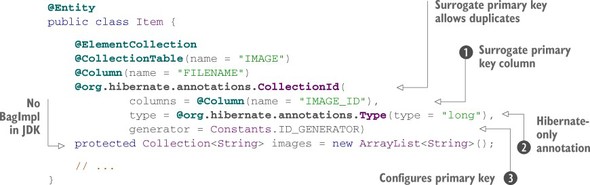
Path: /model/src/main/java/org/jpwh/model/collections/listofstrings/Item.java
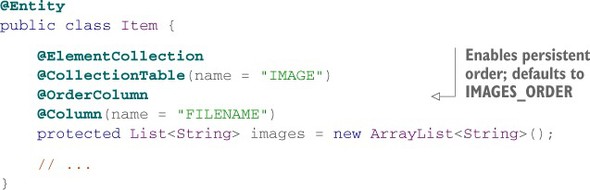
Path: /model/src/main/java/org/jpwh/model/collections/mapofstrings/Item.java
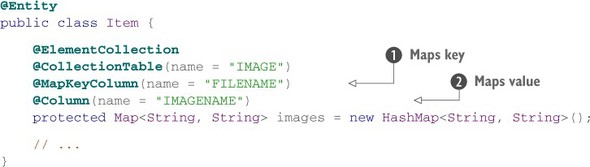
Path: /model/src/main/java/org/jpwh/model/collections/sortedmapofstrings/Item.java
Path: /model/src/main/java/org/jpwh/model/collections/sortedsetofstrings/Item.java
Path: /model/src/main/java/org/jpwh/model/collections/setofstringsorderby/Item.java
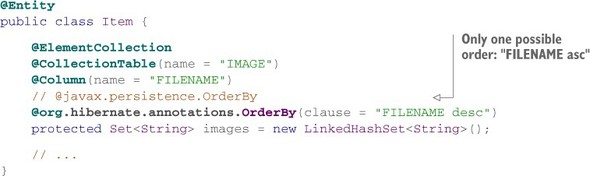
Path: /model/src/main/java/org/jpwh/model/collections/bagofstringsorderby/Item.java
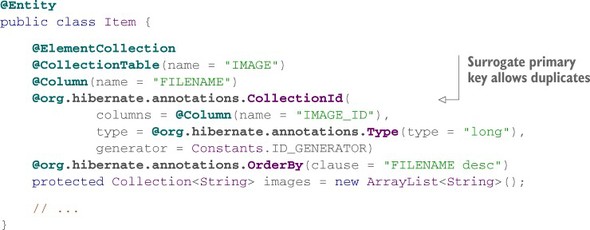
Path: /model/src/main/java/org/jpwh/model/collections/mapofstringsorderby/Item.java
Path: /model/src/main/java/org/jpwh/model/collections/setofembeddables/Image.java
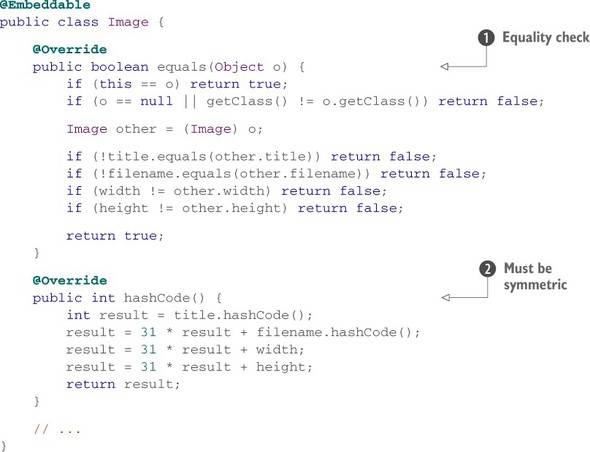
Path: /model/src/main/java/org/jpwh/model/collections/setofembeddables/Item.java
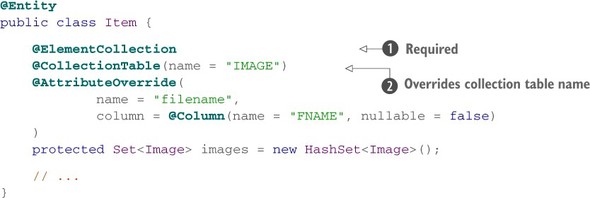