Chapter 8. Advanced entity association mappings
In this chapter
- Mapping one-to-one entity associations
- One-to-many mapping options
- Many-to-many and ternary entity relationships
In the previous chapter, we demonstrated a unidirectional many-to-one association, made it bidirectional, and finally enabled transitive state changes with cascading options. One reason we discuss more advanced entity mappings in a separate chapter is that we consider quite a few of them rare, or at least optional. It’s possible to only use component mappings and many-to-one (occasionally one-to-one) entity associations. You can write a sophisticated application without ever mapping a collection! We’ve shown the particular benefits you gain from collection mappings in the previous chapter; the rules for when a collection mapping is appropriate also apply to all examples in this chapter. Always make sure you actually need a collection before you attempt a complex collection mapping.
Let’s start with mappings that don’t involve collections: one-to-one entity -associations.
Major new features in JPA 2
Path: /model/src/main/java/org/jpwh/model/associations/onetoone/sharedprimarykey/Address.java
Path: /model/src/main/java/org/jpwh/model/associations/onetoone/sharedprimarykey/User.java
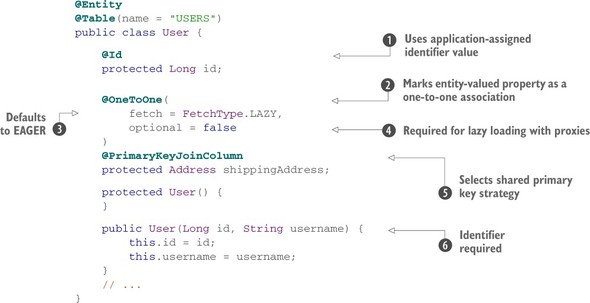
Path: /examples/src/test/java/org/jpwh/test/associations/OneToOneSharedPrimaryKey.java
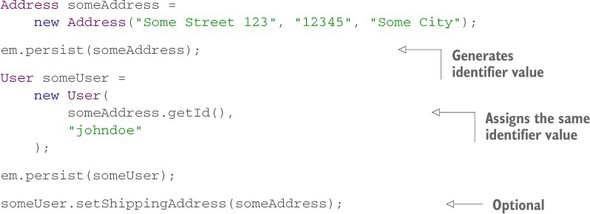
Path: /model/src/main/java/org/jpwh/model/associations/onetoone/foreigngenerator/User.java
Path: /model/src/main/java/org/jpwh/model/associations/onetoone/foreigngenerator/Address.java
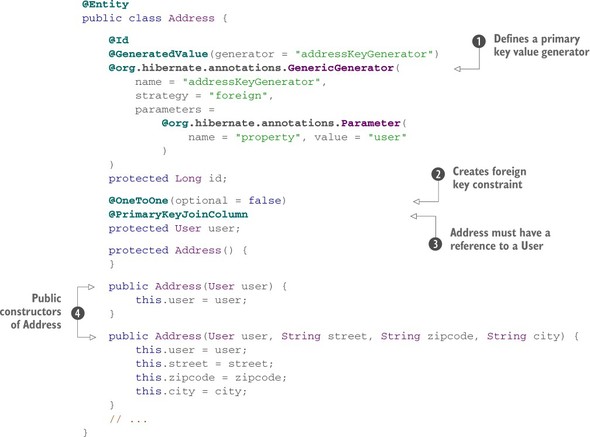
Path: /examples/src/test/java/org/jpwh/test/associations/OneToOneForeignGenerator.java
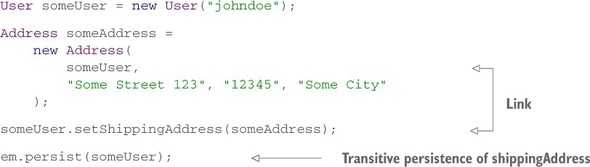
Path: /model/src/main/java/org/jpwh/model/associations/onetoone/foreignkey/User.java
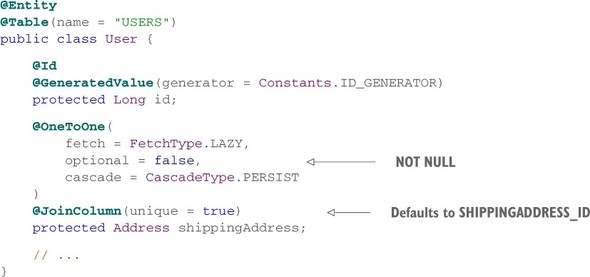
Path: /examples/src/test/java/org/jpwh/test/associations/OneToOneForeignKey.java
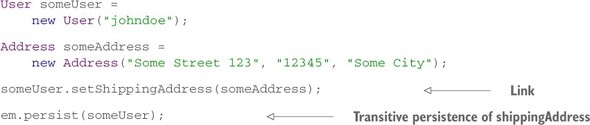
Path: /model/src/main/java/org/jpwh/model/associations/onetoone/jointable/Shipment.java
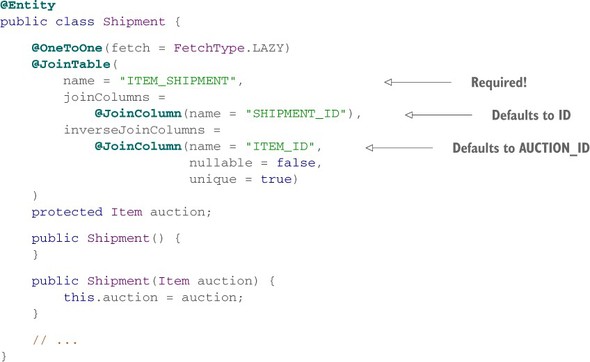
Path: /examples/src/test/java/org/jpwh/test/associations/OneToOneJoinTable.java
Path: /model/src/main/java/org/jpwh/model/associations/onetomany/bag/Item.java
Path: /examples/src/test/java/org/jpwh/test/associations/OneToManyBag.java
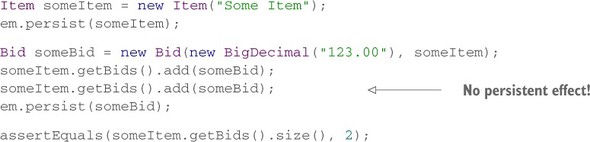