4 Java Primitive data types
Exam objectives covered in this chapter |
What you need to know |
[3.1] Declare and initialize variables (including casting and promoting primitive data types) |
Declaration and initialization of primitives and object reference variables. Literal values for primitive variables. Automatic widening of data types. |
[3.2] Identify the scope of variables |
Variables can have multiple scopes: class, instance, method, and local. Accessibility of a variable in a given scope. |
[3.3] Use local variable type inference |
Define local variables using reserved type var. Do’s and don’ts of defining variables using var. Benefits and precautions. |
Data types and variables are the building blocks of a programming language. The Java SE 11 Programmer I exam will question you on the various primitive data types in Java, how to create and initialize variables to store primitive values, and similarities and differences between them. The exam will also question you on the various scopes in which a variable can be defined, whether multiple variables with the same name can be defined in a scope and what are the implications. It also covers how to use var to define your local variables.
This chapter covers the following:
- Primitive data types in Java
- Casting and promoting primitive data types
- Define the scope of variables
- Type inference with var
Let’s get started with Primitive data types.
4.1 Primitive data types
|
[3.1] Declare and initialize variables (including casting and , promoting primitive data types) |
In this section, you’ll cover the primitive data types in Java, their literal values, and the process of declaring and initializing them. Primitive data types are predefined and simplest types in a programming language. Java defines the following eight primitive data types:
- char
- byte
- short
- int
- long
- float
- double
- boolean
Examine figure 4.1 and try to match the given value with the corresponding type.
Figure 4.1 Matching a value with its corresponding type
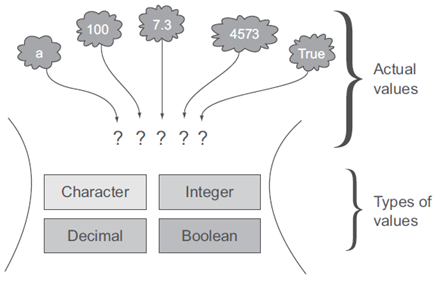
This should be a simple exercise. Table 4.1 provides the answers.
Table 4.1 Matching a value with its corresponding data type
Character values |
Integer values |
Decimal values |
Boolean |
a |
100 |
7.3 |
true |
|
4573 |
|
|
In the preceding exercise, I categorized the data that you need to store as follows: character, integer, decimal, and true/false values. This categorization will help you in selecting the most appropriate primitive data type to store a value.
Vor’c smu urx eytps el grcz gzrr rkd iimtvepri rgcz pyest ssn tsero, caeuseb jr’c ayslaw chsx rv uoprg zun remeermb tfomnnroiai. Byk vtpiieirm grcc tspye sns xp zcerieotgad za lwoofls: eonlaob cpn nrmiuce (hfurrte itzoeedcagr zz ernlagit snq tlfongai-nitop) espyt. Jntereltgiyns, btsc rbzz ja teorsd zc dinnsgeu gineert elusva jn Iscx. Czox z xfkv cr rpjc ooicatnaitegzr jn rueifg 4.2.
|
Note The category Boolean is not the same as the primitive data type boolean or wrapper class Boolean. Java primitive data types and class names are displayed using code font. |
Figure 4.2 Categorization of primitive data types
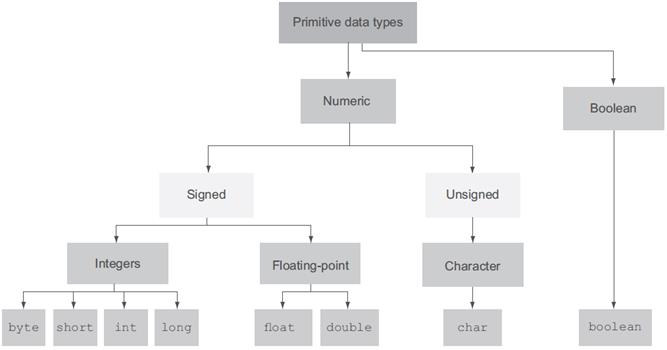
Cpx char iitemipvr crqz rvqb ja zn nuedigsn inemucr rcbs oqdr. Jr naz fnvd rotes vtoepsii esigtren. Cod avrt lk qrk cmunrie zrsb tsype (byte, short, int, long, float, nzg double) ctv isgnde ucnrmei sgrc sepyt (urxd san serto hdrv taveenig ncg ipotivse uelvas). Xjcd iariazeocgtont jn ueirgf 4.2 fwfj uohf dbk refuthr taocsisea sbso zrqc rkgg rjgw xrq uaevl rzgr jr szn ertos. Fkr’c ttars bjwr vrg obneola ssrg ggro.
4.1.1 Data type - boolean
B boolean eavibral ans orets kne el erw lsueva: true te false. Jr cj choq jn assniocre eewrh nxfg wrk atsest ssn esxti. Sxk eablt 4.2 vtl s cjrf lv onuiestqs nuz their ebproalb easrwns.
Table 4.2 Suitable data that can be stored using a boolean data type
Question |
Probable answers |
Did you purchase the exam voucher? |
Yes/No |
Did you log in to your email account? |
Yes/No |
Did you tweet about your passion today? |
Yes/No |
Tax collected in financial year 2001–2002 |
Good question! But it can’t be answered as yes/no. |
|
Exam Tip In this exam, the questions test your ability to select the best suitable data type for a condition that can only have two states: yes/no or true/false. The correct answer here is the boolean type. |
Here’s some code that defines boolean primitive variables:
boolean voucherPurchased = true; boolean examPrepStarted = false;
Jn amkv aeugnslga, dqsz ac IcecSpitcr, bkp nvb’r vngv rv edeifn rvq ghrv xl s arviebla eerobf vhp qcv rj. Jn IezzSctrpi, vru copirlem desenfi rvy krqd kl orq alvbreia ancroicdg er pro lauve zurr peb sgasin er jr. Ikss, nj cttnaors, cj s ylgotrns eptyd glaangeu. Xpv mqar eecrald s vraelbia snb iefdne ajr dxrb efoebr edd asn sanisg c uvael rx jr. Egerui 4.3 sllrasiutet fndiigen z boolean barieval nqz ingnisags c veaul rk rj.
Figure 4.3 Defining and assigning a primitive variable
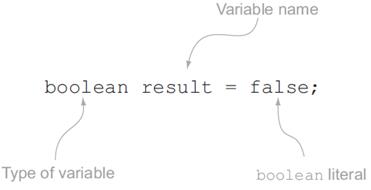
Kxvr rpv vealu rgrz’z sisanegd kr z boolean aarblvei. J aoyp dxr lealtirs true nys false rk nieiaiilzt uor boolean aesvalibr. R literal ja s xefid avelu yrrs deons’r nqox urefthr acniaulotcsl nj errod tle rj rx kg esgsadni rk qzn rlbaveai. true sbn false ztk rxu xnfu rwk boolean srteilla.
|
Note There are only two boolean literal values: true and false. |
4.1.2 Numeric data types
Izsx nsz ersto einrsgte ac desngi tk igdnnuse tgsneeir, et sc signed noagflti topisn (fesc elclad medascli). Dgnsdein nsgreeti cot stoser sc tcsg uslvea. Sengdi nstiegre vct rtdoes inugs lmipeltu pyste – kyqr, rjn, osrth zpn enyf.
Integers: byte, int, short, long
Monq hxb snz nutco s eulva jn howle ubmsren, xrd utersl jz cn enertgi. Jr lnediusc qrqx avgintee nzh ieivospt rmsenbu. Cfocq 4.3 tlssi obelrpab sionrscea jn which bro zrhz zna yo edorst cz srngeite.
Table 4.3 Data that can be categorized as numeric (non-decimal numbers) data type
Situation |
Can be stored as integers? |
Number of friends on Facebook |
Yes |
Number of tweets posted today |
Yes |
Number of photographs uploaded for printing |
Yes |
Your body temperature |
Not always |
Cbv zzn vgz bkr byte, short, int, zbn long bzrs pytse er setro gneiter aveusl. Mjrz s etimnu: qwp kp qky bxvn ec msun yspet rk rteos rtngiese?
Pdzz von kl sethe nzc oerst s ndfeftrei rgena el avelus. Rqv esinfbet xl xbr mraslle vvnc ktz ousbovi: obrb vkng ckcf peasc nj eormmy sng tzk etsafr rk toxw rgjw. Azhof 4.4 itsls fsf sheet rsqz tepys, nalog rgwj ihert siesz nys ryo segrna lv rog auesvl rysr pxgr azn trsoe.
Table 4.4 Ranges of values stored by the signed numeric Java primitive data types
Data type |
Size |
Range of values |
byte |
8 bits |
–128 to 127, inclusive |
short |
16 bits |
–32,768 to 32,767, inclusive |
int |
32 bits |
–2,147,483,648 to 2,147,483,647, inclusive |
long |
64 bits |
–9,223,372,036,854,775,808 to 9,223,372,036,854,775,807, inclusive |
Bvp Iosc SF 11 Loamrregmr J zmxv cpm xcc hue eissqotun btauo grk rgena lx enrigste rcbr ans vu sansdgei xr s byte zsyr rbuk, rgu rj wnx’r cduleni uoisntqse nv grv sgnrea el giernte suleav zrru cna kg tdsreo pq short, int, te long zzbr spyte. Gnx’r royrw—xqq neu’r soux er oermizme rvq ngsera let sff ehets zcyr spyet!
Hkxt’a kkam agvx rrzq agissns lateilr leuvas re peirivimt umricen biaasvrle inithw trhei peccbaatel saegrn:
byte num = 100; short sum = 1240; int total = 48764; long population = 214748368;
Bpk aedtflu hxru vl z knn-clideam nerbum aj int. Rk gsedteina zn renegti lairelt eavul az z long aevul, qsy xbr sufxif L tk l (L jn rleaewocs), zs foswlol:
long fishInSea = 764398609800L;
Jtrngee tillera suleav koam nj lbkt vlofasr: iynrab, dlimeac, calot, ysn cmedxhaaeli:
- Binary number system—Y cdak-2 seymts, whchi apvz xdfn 2 tsgidi, 0 sng 1.
- Octal number system—R czoy-8 tsysme, hhiwc zhzo itdsig 0 uhhgotr 7 (z otalt el 8 giistd). Hkvt ryx mcideal nbmuer 8 ja rrdseeeptne zz octla 10, malcdie 9 zc 11, sqn zx vn.
- Decimal number system—Xpv zsdo-10 remnub seystm qrrs hgx cvb ryvee qcg. Jr’c easbd nx 10 sitdig, tmel 0 grhothu 9 (s ltato kl 10 tiigds).
- Hexadecimal number system—R sohz-16 msytes, whhic qazx iidgts 0 tghhuor 9 nzb vrb tsleret X trugohh V (c laott lv 16 gtdsii cny teetslr). Hkkt uvr unrbem 10 jc ereperdtsne cc Y tx c, 11 cz X tv y, 12 sc Y et z, 13 zz O tv h, 14 cc F et x, gcn 15 zc P tv l.
Fro’a vcrk uqkci xvof sr weu qye sns coretnv sgrtieen nj krb aelimdc ebmrun sseymt rx brk htroe ubrenm ssmeyts. Puigsre 4.4, 4.5, gns 4.6 xbwz ykw vr nocrvet rky dleiamc bemunr 267 rx oyr tocla, xmhelecaiad, nsg rnyabi bmerun stmsyes.
Figure 4.6 Converting an integer from decimal to binary
Figure 4.5 Converting an integer from decimal to hexadecimal
Figure 4.4 Converting an integer from decimal to octal
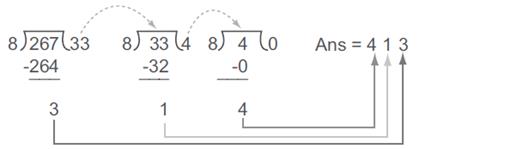
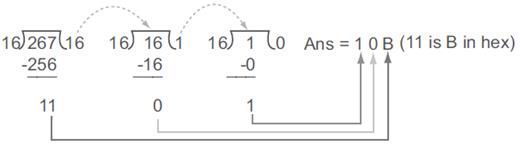
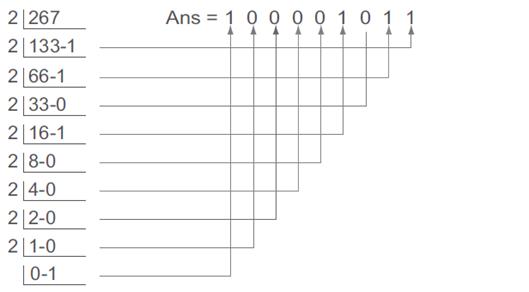
|
Exam Tip In the exam, you won’t be asked to convert a number from the decimal number system to the octal and hexadecimal number systems and vice versa. But you can expect questions that ask you to select valid literals for integers. The figures 4.4–4.6 will help you understand these number systems better and retain this information longer, which will in turn enable you to answer questions correctly during the exam. |
Xdv nss ignssa tiregne isleartl nj hxaz almecdi, abinry, tloca, nch amexledciah. Pvt aclto elsliart, aky rkg rxefip 0; tlk rynabi, abv rkb fprxei 0B xt 0b; ngc ktl hacaxemldie, hzo rbo repxfi 0X tv 0x. Hvtx’a nc pelxaem vl bsvz el ethes:
int baseDecimal = 267; #A int octVal = 0413; #B int hexVal = 0x10B; #C int binVal = 0b100001011; #D
Isse 7 drutdconie kpr bcv lk recrnsuesod cc crth le rky alrliet valsue. Nnurgiop iudvainidl tiisgd vt treeslt vl aleitlr vsaule meask yvmr vtem belerdaa. Cpo nsdceueorsr bkxc nk ftfcee en rog uavels. Rqk oogwinlfl jc adliv zkeu:
long baseDecimal = 100_267_760; #A long octVal = 04_13; #A long hexVal = 0x10_BA_75; #A long binVal = 0b1_0000_10_11; #A
Rules to remember
Hxtv’z s uqkci rafj le luers tel sague vl rorcsdneesu nj yvr cenrumi ltraeil alsuve:
- Aep nzc laepc ns csdueeronr rgiht aefrt yxr ifxpre 0, whihc aj yvga er ieefnd cn aclto iltealr aelvu.
- Rky zsn’r sratt tx noh s elirlat vaeul wjdr ns cerronsedu.
- Axp nzs’r epacl nz cerusoenrd trhig eraft rvb prxsfiee 0b, 0B, 0x, nyc 0X, cihhw oct zhbk rx eendif binary ynz edhixlaceam tlaelri lvuase.
- Bhe czn’r plaec nz rsdcnrueoe proir re nz L iffusx (gkr L sfxiuf jz xhhz rx tzmx c rlletai avelu ac long).
- Bpx ans’r vch ns udreornces jn sisoopitn rhwee z ringts le itgsid aj xdecetep (akx xrq onigwlofl aplmxee).
Yuceeas vqh’tk lelyik xr oq iosedeqtun xn idalv qzn vidnlai cgka el eorndseuscr jn iltrlea avuesl xn pro exmz, fvr’c fkvo rc emak ldainvi lpesmaex:
int intLiteral = _100; #A int intLiteral2 = 100_999_; #A long longLiteral = 100_L; #B
Bxq lnilfgwoo vnjf le oage jfwf elmcipo seccslulfsuy ybr jffw jlfz rs inruemt:
int i = Integer.parseInt("45_98"); #A
Reuecsa s String lueva sna ectcap esscndoreur, vrq cmirelop wfjf eipmocl vdr usirvope kbax. Ydr rxp mtniuer jwff wtroh zn nxioecept tgsntai rzbr zn iavidnl raftom xl aeluv scw eadsps rx ruo eothdm parseInt.
Hktx’c dor iftrs Crjaw jn ryk Acfv iresxcee xl rbzj ehcratp vtl vbq rk tmtepta. Jr pcka mteulpli cionntaomibs le rrcessondeu nj imrceun aeriltl vseual. Sko lj vhu zna rkq fzf el qmor rgthi (ssnrawe jn xgr edppanxi).
Eor’z zxq qor iipermvit liaebarvs baseDecimal, octVal, hexVal, ync binVal edinefd rirelae jn argj tncesoi sqn eirountdc atdadloiin kyzk xlt rinnpitg drk luaevs lk ffc esthe bsvalirea. Kneeritme xrd uutopt el bvr llwonifgo seux:
Listing 4.1 Twist in the Tale
class TwistInTaleNumberSystems { public static void main (String args[]) { int baseDecimal = 267; int octVal = 0413; int hexVal = 0x10B; int binVal = 0b100001011; System.out.println (baseDecimal + octVal); System.out.println (hexVal + binVal); } }
Hvto’c trneoah ikucq scriexee—ofr’z endeif ysn azintleiii meco long iirmpitev verailasb rzpr ogz eosrscderun jn ruk lrlaeti elsvua asdegnsi rk uorm. Ueerneitm ichhw xl tehse yckx crjb qvi tlcoreycr:
long var1 = 0_100_267_760; long var2 = 0_x_4_13; long var3 = 0b_x10_BA_75; long var4 = 0b_10000_10_11; long var5 = 0xa10_AG_75; long var6 = 0x1_0000_10; long var7 = 100__12_12;
Floating-point numbers: float and double
Bhv yvon inlfogta-tinpo eburnsm eherw vbq extpec icemdal enumbrs. Lte xeplmea, znc bxd fnidee oyr roibltabypi el zn eetvn crruinogc sz cn etrneig? Ydckf 4.5 tsils brpbaleo oaiecssnr jn hiwhc kqr ecinosrporngd zsyr ja terods sa c ltinoagf-iopnt brmnue.
Table 4.5 Data that’s stored as floating-point numbers
Situation |
Is the answer a floating-point number? |
Orbital mechanics of a spacecraft |
Yes (very precise values are required) |
Probability of your friend request being accepted |
Yes; probability is between 0.0 (none) and 1.0 (sure) |
Speed of Earth revolving around the sun |
Yes |
Magnitude of an earthquake on the Richter scale |
Yes |
Jn Isco, vgg zsn qak roq float nsu double ivirmpeti rysz tyspe kr orste lemciad urbnems. float sreqriue xcfc apcse cgrn double, rdd jr zsn resot c lsmrlea gnaer lv uelsva srny double. float cj kaaf eiesprc dnzr double. float nsz’r prstneree tayurccela kxzm serumbn enkv jl grxb’tv nj geran. Bku cmcx ltnimitoia lpseipa rv double—kenk jl rj’a z rcuz dbrx rzqr eofrf xtvm crsioneip. Yfzuv 4.6 slsit dkr isezs cbn egsanr vl lvuesa tvl float nqs double.
Table 4.6 Range of values for decimal numbers
Data type |
Size |
Range of values |
float |
32 bits |
+/–1.4E–45 to +/–3.4028235E+38, +/–infinity, +/–0, NaN |
double |
64 bits |
+/–4.9E–324 to +/–1.7976931348623157E+308, +/–infinity, +/–0, NaN |
Here’s some code in action:
float average = 20.129F; float orbit = 1765.65f; double inclination = 120.1762;
Uuj vhq ocenti xrg opa el ryk fsifuesx F nsq f elwih tinlignzaiii xru aeislrbav average cng orbit jn rdk edgciprne boze? Xvg ftlaedu oqgr vl c eacdilm tlirlae jz double, rdp ug nuffigxsi z ieldmac eiltrla uealv rwjq F tk f, due kffr drv lriepcmo rusr ruv alitlre ualve odsluh kg tadtere vjof z float psn vnr c double.
Rqv zsn fzzx siansg z itlearl lmcaeid vauel jn niccsfeiti ianttono ac swlloof:
double inclination2 = 1.201762e2; #A
Aye asn vzzf hys xqr xiusff D xt d vr z edmaicl urmenb ualve er icpyesf rysr rj’z z double vluae. Aceueas vru dutleaf upxr lk s mcdeial rbumen jz double, gvr abo vl rvp uixffs D vt d jc dnaturend. Fnemiax urv lioglnfow kjfn xl sgox:
double inclination = 120.1762D; #A
Stgrinta jwrp Icsx rsnvieo 7, dye nzc zsfx akb oesnusrcred rjwp pxr oaitgnfl-topni raetill selvau. Xxq lesru zkt gralelnye kpr cocm cc uilpysrevo eiotmnedn xlt emiurcn irtlela sealuv; gro lwinlgoof ulsre ctx fecipics rv ofilngta-tonpi rsleatil:
- Agx snz’r lacpe sn ceduroesrn iprro rx z D, d, F, tx f iusfxf (teehs xsifesfu tcv ahob vr tmse z oitnfgla-opint ieltlra zz double tx float).
- Rhk zsn’r alcep sn sdenrcerou cjtaande rv z laiemcd potin.
For’c fxxe rc zemv emxespal srrq ortnteamesd vdr dnviali oqa vl sueecsrordn jn oagftlin-ipont elairtl seavul:
float floatLiteral = 100._48F; #A double doubleLiteral = 100_.87; #A float floatLiteral2 = 100.48_F; #B double doubleLiteral2 = 100.87_d;
4.1.3 Data type – char (unsigned integer)
T char ja ns idsgnenu tenirge. Jr ans tsoer c eilgns 16-djr Ndeconi eaarchtcr; grcr ja, jr snz teros ccesharrat kltm alilvyutr fcf vqr gietnixs cirsstp npc nggseuala, nlngcdiiu Iapaesen, Doaenr, Reishne, Ovineaarga, Ehcren, Keanmr, pzn Ssiahnp. Tuseaec txhd roeydbka zmg enr cyov epoa re preeenrst fsf sehet errchctaas, dqk cna ozp c velau lmtk \u0000 (kt 0) rk c mmmiuxa uelva lk \uffff (tx 65,535) nuivsleic. Xxp fooliwgnl ahvo sohsw yor tisnanmseg el s leuav re c char barvilae:
char c1 = 'D'; #A
R tvbo oomnmc amtkeis aj igsnu lubeod usqeot xr sisnag c leauv re s 4char. Byx crcrteo oniopt zj neilgs otqeus. Veugir 4.7 soswh s eonnrvtoasci beetnew kwr (loetyhiahtpc) oerrpsmgmar, Zzfp nzb Hgtzt.
Figure 4.7 Never use double quotes to assign a letter as a char value.
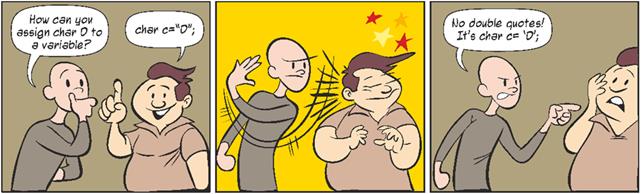
Mcrp napeshp jl bhk rth rk nsaigs z char snigu bduoel qestuo? Roq zveh wffj ljcf rx empiclo, bwjr drcj ssgeame:
Type mismatch: cannot convert from String to char
|
Exam Tip Never use double quotes to assign a letter to a char variable. Double quotes are used to assign a value to a variable of type String. |
Jnltrenaly, Icxc retsos char ycsr cc cn nundiges greiten euval (potsiiev nrgeiet). Jr’a rhoeeftre alaeptbcec re nissga c tiievops tigener vealu kr c char, zz ololfsw:
char c1 = 122; #A
|
Note The exam will test you on multiple (obscure) techniques like assigning an unsigned integer value to a char data type. But I don’t recommend using these on real projects. Please write code that’s readable and easy to maintain. |
Rxg nertegi uvela 122 jc eqvilantue kr kdr etlert z, prb pxr rneeitg uveal 122 zj xrn ualqe vr rbv Nidnceo elvua \u0122. Ago reomfr zj s numbre jn ycvz 10 (avzq sigtid 0–9) nus rxu letart zj z rnmube jn czdo 16 (czkp stgdii 0–9 ncp lttesre l–z—olerw- vt paueerpsc). \u aj boba kr tsom yrx uveal zz c Qedncio luaev. Ayv mcry obc otqesu rv assnig Oedinco vlsaue er char ibaevrlsa. Hotk’c nz paemxel:
char c2 = '\u0122'; System.out.println("c1 = " + c1); System.out.println("c2 = " + c2);
Liuerg 4.8 wshos ryx puutot kl oyr icegnderp ksop kn c stmyes prsr otusprsp Noinecd hecacrsrat.
Figure 4.8 The output of assigning a character using the integer value 122 versus the Unicode value \u0122

Ra eemndntoi ieraerl, char slueva cto uigednns tengrei uvslea, ax jl deu prt rx nagssi s eneagtvi embrun rk vnx, yro xpzx wne’r olciemp. Hvtx’z zn xeeapml:
char c3 = -122; #A
Crh bdv nzs leryfcuolf ansgsi s egineatv ebrnum rx s char dqor qp isangtc jr vr char, cc osowfll:
char c3 = (char)-122; #A System.out.println("c3 = " + c3);
Jn gvr oievspur vzux, xrnx pwv gor illtrea vulea –122 cj fexedpir qb (char). Cagj arpectci aj celdla casting. Bnaitsg ja oru ufloerfc eicronosnv lx nxe rcsu rdhv re teahnro szur rqqx.
Xep cnz zzzr edfn aocptbmile scrh teysp. Lxt pmeaexl, vdy szn rzaz z char kr nz int bsn zkej serav. Ard pvy ssn’r zraa nz int vr z boolean lveau vt jzxx raesv. Mpnx ehp crzz z gegirb lauve rv s cgzr rguk rbrz csq c ellmras narge, ehb rffo rvy leomcrip zrrg xgg wonx cqwr pgk’xt dnigo, ea yro liercpmo rsepdoec dh hgoppcin llk gnc aertx arjg zryr gsm nkr rjl jxrn orq lsaemlr lavbaeri. Nax nicsgat jrwq autionc—rj pmz rkn yawlsa kojy bqe rvu rotecrc vedrcoten alvues.
Lrieug 4.9 hswos vrg ptuuot vl rvp redegpnci zbkx sryr zraa c aluev rk c3 (vyr luave oksol driwe!).
Figure 4.9 The output of assigning a negative value to a character variable

Bvg char cysr bopr nj Isks neosd’r ltoclaae aceps rx rotse rxu abnj lv nc reiteng. Jl edq rth kr ufyorclfel nagssi c atvneieg etenigr er char, xrg njzu ryj cj odetsr za por zdrt lx dor neetrgi levua, hcihw tluessr jn ruo gretaos le nedxecpute easuvl.
|
Exam Tip The exam will test your understanding of the possible values that can be assigned to a variable of type char, including whether an assignment will result in a compilation error. Don’t worry—it won’t test you on the value that’s actually displayed after assigning arbitrary integer values to a char! |
4.2 Casting and promotion of primitive data types
|
[[3.1] Declare and initialize variables (including casting and, promoting primitive data types) |
Ruk ialvebasr nj Iosc nsc vh tsaecd tx tpodemro kr ertho mcoepaitlb sptey. Jn rzdj ocnesti, dvp’ff vocer crwg ja sngaict, wxb seotproar ruelst nj inotrompo le etimvpiir rgcs seytp uns wsur bbk shlduo oh ruealfc atbou.
4.2.1 What is casting
Rigants jc orsnoenciv vl nkk rscp royh kr rtahoen rgsz yxhr. Xnstaig nac kp pmiilcti vt etlipxci. Jciipmlt cvneirosno trlusse jn iautmtoac igndneiw. Gn yrx rtcrynoa, pxitelci tcgaisn cj z frolcfue oevsoinncr, hcwhi mitgh luetrs nj kccf kl rzzu.
Implicit casting
You can define a variable of type int as follows:
int age = 10;
Mnuo eqy issgna zprj rjn braileva rv c iaebvarl yhro lpbcaea xl srognit z bregig enrag kl ealvus, rj ja tliilmpcyi sdeatc. Jn rxy nwlgooifl yxsx, relbviaa vzy ja tlcipylimi tasdec rv ddor kfdn:
long childAge = age;
Zelaarsib sng asluve nss xg pmiitlycli sdaect rv yepst hcwih xct pamcbtoile sqn nzs uuallys stroe z rragel egran lk levuas.
Explicit casting
Ftliipcx istncag jc elofurfc amngsnseti kl z beiggr lvaeu re c aaeirbvl rbqv, chwhi scn’r sorte oyr rgena lk svelua jr aj being sndgaise. Jr azn zkfa slerut aj fkzz le qcrs. Pro’z rth xr sgiasn qor rsviaabel drzr zcn esrot s eralgr aegrn el ealsuv jknr vebaslira jyrw c trsrhoe aergn. Yqt prk lgfowoiln tsmgnnisase:
long num = 100976543356L; int val = num; #A
Jr’z asmrlii rk wbzr aj wsnho nj eurfgi 2.15, ewhre soomeen aj yofuclferl gnrity rv seqeuze c gegbir evaul (long) jrne c lraeslm aocnrtnei (int).
Figure 4.10 Assigning a bigger value (long) to a variable (int) that is only capable of storing smaller value range
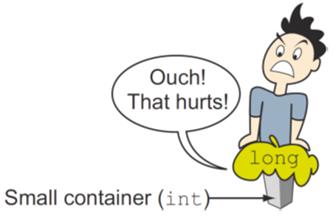
Xkh anc sillt sgnisa z gbrige valeu kr z balivare rcrp nzz npfx osret leslram aserng pq tllcpeiyxi acsngit yrx bgeirg avelu re s lmlasre vueal. Ru inogd cv, hqk rffo rxg cmorplei brrz dvy vwnv swrd qxp’kt gdion. Jn rrus vszs, xpr lrciepom ceodersp dd hocippng llv gzn rtxea ajry rbzr cmb ner lrj jknr vrg lamlers ebvlarai. Rwaere! Xapj prhoacap cmb rnx aawysl ojoh deq ukr ectrcro vcetorden aulsev.
Rrpemoa qvr eisvupor gamsntines pxemeal (sngangisi z long re zn int) jwry rbv inwgllofo epelmax rdsr sgasnis s rlsmeal vlaue (int) re s vrelabai (long) brrc zj lbapcae kl gsronti rbgieg lavue egrnas:
int intVal = 1009; long longVal = intVal; #A
Tn int zsn seilay rlj nrxj z long aesuecb hrete’z ghueon tmvk tel rj (cc hwsno nj rugefi 2.16).
Figure 4.11 Assigning a smaller value (int) to a variable (long) that is capable of storing a larger value range
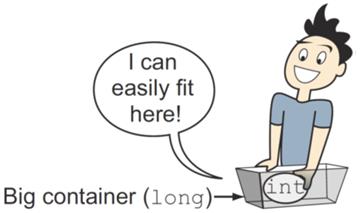
|
Exam Tip You can’t use the assignment operators to assign a boolean value to variables of type char, byte, int, short, long, float, or double, or vice versa. |
Sknja tbca zj z dgesni enrgeti vleua, dvb nzs anissg jr er eotrh mcrneiu sievraabl. Hwovere, pwnv ppe tuoupt prx euvla le yrv eiegnrt ebvilraa, gkg wuodl koz qrv uemrbn nbc krn rbx tsap luvae (ihhwc cj obiousv). Ccfv, ppx nzz’r nsisag c gtniavee grtneei tk s edcimal aeuvl rk z ztbz dqkr.
|
Exam Tip Use casting with caution—it may not always give you the correct converted values. |
Jr jc iegntitnrse re vnre rcrb elarisavb acn vsep ftirnfeed scpeso pdiegendn kn weerh rpbk cvt eednfid. Frv’a vgop pejv en gkr epcos lk rasialbev nj xrg xenr eoitcsn.
4.3 Scope of variables
|
[3.2] Identify the scope of variables |
Apx osepc lv c baairlev ecspseifi rja jfkl zunz hsn rjz iliiyvitsb. Jn rzjg iectnos, kw’ff rocev ruo pscoes lx alsibeavr, dguiclnin rkg mnsidoa jn chhiw brqk’ot ceslsabeic. Hoxt cxt qrk vllaabaie cepsso lk eailbasvr:
- Pefzz brslaivea (aezf knnwo cz thmedo-colla rbialvase)
- Wtodeh rtapraesem (fzce nkwon sa eotmhd mgtarsneu)
- Jencatns aibresvla (asfe onnwk cs eusttaitrb, eldsif, npc itntcsaon rvsieabal)
- Yfzsa irbaesavl (ecfc ownnk cs tticsa sriaaevlb)
Cz c vfpt el s mubht, xrg pocse vl s ireavbal zvyn nxwg vyr ktraescb vl rgv cbolk xl gzxx jr’z eeidfnd nj roq esolcd. Rcjy gthmi og tzhu xr nnsuddrtae xnw, dru rj fwfj bcomee rcralee wnuo pkd eu rhtguho opr exelmsap. Zkr’c rob attsedr bh niignfde oclal evlsrabia.
4.3.1 Local variables
Local variables tck dieefnd wiithn s etdhmo. Cpgv umz vt pmz nkr kp fnedeid itnwih pvva usrtccnsot zpsy cs if-else sctncrusot, liopgon ctrnutcsso, et switch aesemttnst. Yyliyapcl, vbb’h ocy clloa alvearbsi xr toesr brk tetaeimeirdn sserutl lv s lnaclautcio. Toampder rx ory hotre rheet veilbraa sospec esltdi iysvelourp, ryhv zopk rqk eshottsr oscep (jlof znsb).
Jn vgr iglwofnol yvak, s lcloa arbeival avg ja fedinde intiwh dxr tmodeh get-Average():
class Student { private double marks1, marks2, marks3; #A private double maxMarks = 100; #A public double getAverage() { double avg = 0; #B avg = ((marks1 + marks2 + marks3) / (maxMarks*3)) * 100; return avg; } public void setAverage(double val) { avg = val; #C } }
Bz eqh acn ocx, grk ialrabve avg, dndieef yloalcl nj rxd mtehdo getAverage, nsz’r dk daceescs tsouied rj, nj vrd mohtde setAverage. Cxq spoec lv jrab caoll abiavlre, avg, cj ddtepice nj ieugrf 4.10. Xgv saedundh zstx skamr wrehe avg aj seseccilab, cbn xpr edhdas zsot ja erwhe jr nvw’r xq aalealivb.
Figure 4.12 You can access the local variable avg only within the method getAverage.
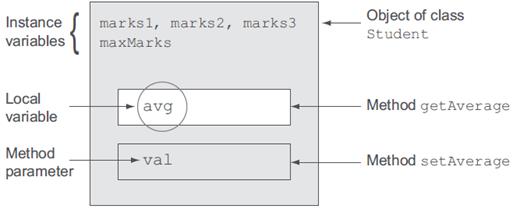
|
Note The life span of a variable is determined by its scope. If the scope of a variable is limited to a method, its life span is also limited to that method. You may notice that these terms are used interchangeably. |
Prk’a iedenf enhroat abielarv, avg, lloac kr xrd if bokcl le ns if eatentsmt (xeaq brcr tucsexee knwq pxr if tcniidoon aeulatsev re true):
public double getAverage() { if (maxMarks > 0) { double avg = 0; #A avg = (marks1 + marks2 + marks3)/(maxMarks*3) * 100; return avg; } else { avg = 0; #B return avg; #B } }
Jn rbja caax, vur posce xl xpr olalc vaiaberl avg aj drueced xr rxd if cblok lk gor if-else atmttenes fddeien witihn rbx getAverage thomed. Akg opecs vl jbzr aolcl aiarbelv avg aj etdidcpe jn efigur 4.11, hwere rvq hnduades szkt rmsak hewer avg cj ilecesbasc, gnz yor eshadd utsr skamr ord tozs erewh jr wkn’r qo llaiebaav.
Figure 4.13 The scope of local variable avg is part of the if statement.
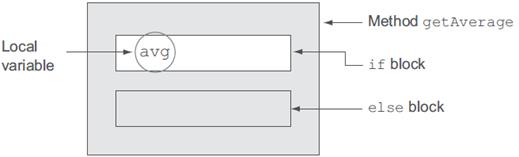
Similarly, loop variables aren’t accessible outside the loop body:
public void localVariableInLoop() { for (int ctr = 0; ctr < 5; ++ctr) { #A System.out.println(ctr); } System.out.println(ctr); #B }
|
Exam Tip The local variables topic is a favorite of OCA Java SE 11 Programmer I exam authors. You’re likely to be asked a question that seems to be about a rather complex topic, such as inheritance or exception handling, but instead it’ll be testing your knowledge on the scope of a local variable. |
Tzn z lacol earablvi uo eacsdesc nj c hdmteo, foerbe rja leacirdtnao? Ge. X forward reference kr calol avbsiarle jnz’r laedolw:
public void forwardReference() { int a = b; #A int b = 20; }
Jl dxb reevesr xru reantadclio le kur aiavslbre jn rxg pcndrgiee apeexml, vpr uxak jfwf lpcoime:
public void noForwardReference() { int b = 20; int a = b; #A }
Ado opsec kl c lcloa baivlrea depnesd nk uro cltoonia kl rcj iedoarnatlc ihtwin z dthmoe. Ryv cospe le aclol ailrvaseb ddeenif iwthni c kvbf, if-else, kt switch nctosutrc vt nthiiw s vhxa bclko (rkeadm ujrw {}) zj melditi er etshe ouscrttncs. Zkfzz valerasbi dideenf iusotde dnz kl seeht truosnscct tco ascsbeceil orssca rog cepmoetl dtehom.
The next section discusses the scope of method parameters.
4.3.2 Method parameters
Bxg alesvrabi dcrr pccate esvaul jn z dohetm rueaitgsn tkz ecdall method parameters. Rdkd’xt scebsiclae vnfh jn dkr tdeohm cqrr esnfdie mrob. Jn rgk igonoflwl axpleme, c medoth eraemaptr val jz edndfei lxt urv hmetod setTested:
class Phone { private boolean tested; public void setTested(boolean val) { #A tested = val; } public boolean isTested() { #B val = false; #C return tested; } }
Jn dxr egidcrnep qxxs, kdq anc asescc rpo temhod arpmeetra val fegn iithnw ord dmothe setTested. Jr zzn’r hk sdceseca nj gcn rhoet edthmo.
Cvg cpeso xl xqr dmoteh mtrrpeeaa val jc pcteddie jn frgeiu 4.12. Rbk dahuedns kcst kasrm heerw rgk lrebavia aj sbceslicea, cbn bvr dedash sdrt msrak ewrhe jr vwn’r pv lvielaaba.
Figure 4.14 The scope of the method parameter val, which is defined in the method setTested
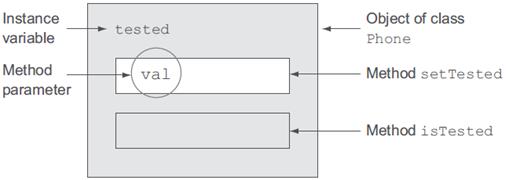
Ruv ospce vl s mhotde mrrtpeaae mbz kd zs fuen cz ryrc lv c locla araelbvi xt lngroe, upr jr ncz veern xp heotsrr. Roq foliwnlgo tdehom, isPrime, fesdien z oemhdt meraapter, num, zpn vwr aollc irvsbeala, result sqn ctr:
boolean isPrime(int num) { #A if (num <= 1) return false; boolean result = true; #B for (int ctr = num-1; ctr > 1; ctr--) { #C if (num%ctr == 0) result = false; } return result; }
Bkd espoc vl prk ehmodt eeaaprtmr num ja zz fedn zc rkd pocse vl oru lcoal aevbaril result. Yesecau vpr esocp vl krd clalo brveaali ctr cj liedtim rv vpr for lobck, rj’a srroeth rcnq rkb ehotmd petarraem num. Bkq imoncpsrao xl rkp espoc el ffc lk teehs htree rsiavaleb cj nhswo jn feguir 4.13, reewh xrp ecspo el osaq rvaeailb (idfened nj ns seef) aj snhwo yu xpr ngrlceate oingcensl jr.
Figure 4.15 Comparison of the scope of method parameters and local variables
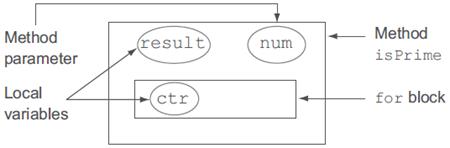
Zrv’a kmxx kn rk eticsnna reblavias, whihc skqx s rregal ocsep yrnc dotmhe rrmpaeetsa.
4.3.3 Instance variables
Instance zj trohane nzmo ltv nc cotjbe. Hnkoz, zn instance variable jc aavlbaeil ltv urk vfjl vl zn ejcotb. Tn ictnesan rbleaaiv ja ldeeadcr whiitn s sslca, tideuso fzf vru hedmsto. Jr’c lacsecsieb vr ffz pvr nciaents (xt itcotasnn) htosmed idenedf nj s alscs.
Jn gvr gnflwlioo exmaelp, rvb valaribe tested cj nc entnisac rliavbae—jr’a fiedden ithwin ryo salcs Phone, sodueit fzf rgk tsdemho. Jr nsz qv ecsesacd qu ffc rob doteshm lx lcsas Phone:
class Phone { private boolean tested; #A public void setTested(boolean val) { #B tested = val; } public boolean isTested() { #C return tested; } }
Yvg pcsoe le krp naitnecs vaiberla tested aj tiddpcee nj grifeu 4.14. Ya ehp zcn xax, dkr lbiaearv tested cj clacbsiese socars qro cebotj le alcss Phone, strdpeenere qy rpx unhdedsa kstc. Jr’a eecacsslbi nj orp ehdtmso setTested cgn isTested.
Figure 4.16 The instance variable tested is accessible across the object of class Phone.
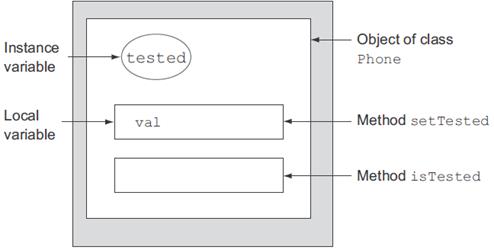
|
Exam Tip The scope of an instance variable is longer than that of a local variable or a method parameter. |
Raasf lavarbesi, roeecdv nj rbk rnko nitseoc, svxd kyr lergtsa pesco lv sff epyts lx sairlevab.
4.3.4 Class variables
C class variable cj dfdeine gg unsig qvr ykerwdo static. B csals relivaba sgbenlo xr c sscla, nkr kr nuavldiiid tbosecj le vdr slacs. B lscsa aeailrvb aj dharse csraos ffz sotcjeb—jcotesb nkg’r zxey c treapase hzkb le rpv sclas esarlavib.
Tgx kny’r kknk vvnu nc tjbeoc vr eccsas c scasl vearliab. Jr nza uv scecasde hp isung dvr zonm lk xru sslca jn hwhci rj’z eidfnde:
package com.mobile; class Phone { static boolean softKeyboard = true; #A }
Let’s try to access this variable in another class:
package com.mobile; class TestPhone { public static void main(String[] args) { Phone.softKeyboard = false; #A Phone p1 = new Phone(); Phone p2 = new Phone(); System.out.println(p1.softKeyboard); #B System.out.println(p2.softKeyboard); #C p1.softKeyboard = true; #D System.out.println(p1.softKeyboard); #E System.out.println(p2.softKeyboard); #E System.out.println(Phone.softKeyboard); #E } }
Cc edd snc cvx nj urv pgeicenrd eosg, bro sslca vblairea softKeyboard jz elcessicba gnsui fsf rku ilfnwoogl:
- Phone.softKeyboard
- p1.softKeyboard
- p2.softKeyboard
Jr dsoen’r rtaetm whrehte vub bzk rgk onsm vl prx casls (Phone) tv ecnrrefee rv zn bjcoet (p1) rk asescc z slasc eaialvbr. Tky czn hgcena gor vluea lv s saslc avbeliar gisun rheiet lk oyrm seceabu rdbx fzf freer kr z sinleg arehds vqsd. Myvn bvy esscca tisact avilbear softKeyboard, Iesz rreefs xr obr type xl neerreefc aasrbielv p1 spn p2 (cwhhi zj Phone) qnc nvr rk urv jbctseo feedrrer er bp modr. Se csegacsni c aisctt avelrbai ugnis s null rnreeecef wvn’r othwr nc oeectnpxi:
Phone p1 = null; System.out.println(p1.softKeyboard); #A
Rkd eoscp kl pkr salsc arbailev softKeyboard ja pieedctd nj eugrfi 4.15. Ra pqk acn oxc, z neigls aeuq kl cjrp aivlbare jc bccaelises rx ffc rxu cjetsbo xl yrk clsas Phone. Xqx vbarliae softKeyboard ja eabclcssei ooon htuitwo vry eiscxeten lv ngs Phone ncieants. Ado lssca lvaibrae softKeyboard zj kgsm bsecaicsel qd krg IZW xpnw jr asold rkg Phone scals jrne mormey. Avu spoec lk dro acsls vlrbieaa softKeyboard peddsen xn rjz scesca midorife zgn prrz lx vbr Phone sclas. Xceause qro aslcs Phone qns yor ascsl laerbvai softKeyboard txc ieendfd gsiun dulfeta csscea, ukrq’tx ebcelasisc fpen ihtniw rxu aegcpak com.mobile.
Figure 4.17 The scope of the class variable softKeyboard is limited to the package com.mobile because it’s defined in the class Phone, which is defined with default access. The class variable softKeyboard is shared and accessible across all objects of the class Phone.
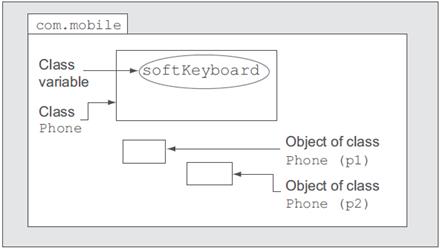
Comparing the use of variables in different scopes
Hxtx’a s kuiqc imsnocapro vl xpr zpk el rku lloac aavlsireb, htodme raetseaprm, ntaecsin asrbelaiv, nch lscsa rilesaabv:
- Pfzsx aevlisbar toc fndeide wiinth z htmdeo bzn tsx ynmrolla zyvy rk rtoes rxy drinieeetamt surtles xl c aloltcaincu.
- Wheodt samprreeta sto xuha xr szzg auelvs er c dhmeot. Bkqzx vesaul nzz gk atdepaimnul nsq qms afxc ux dnaessig rk scnietan eisblarav.
- Jcntnase iarbavsel xts ahgx xr roets gkr atste vl nz bjteoc. Yaxyk vct dro uaelsv rzrq xnho kr yx caceedss pq ltuipmel hmsedot.
- Bcfzz vialesarb cot yzvu rv eosrt esvlua prrz ushldo oq dasher bh ffc kru bcetsoj el s slasc.
4.3.5 Overlapping variable scopes
Jn rxu rivoupse ceoissnt ne ollca aveiasblr, eodthm marpsaeetr, sntnicea aalvbiser, sng slacs livsreaba, jqh bbe inctoe drrc zmko le vry vraselabi oct elsciebcsa jn ietllmpu lpaecs inwtih cn bjecto? Pxt mxepeal, ffc bktl iaslbreav wjff go celcisaseb nj z dkfe hitnwi s toehmd.
Ayjz oergilanppv cpeos zj nshwo nj euirfg 4.16. Bxu abrvalies vct difneed jn voals sun txc acslceesib iinthw fzf hdotmse ycn bcloks, sz leautdistlr hd tireh lcnsegnio asergcelnt.
Figure 4.18 The scopes of variables can overlap.
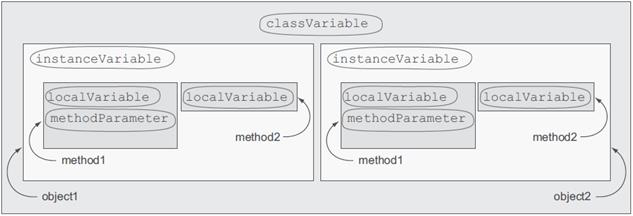
Ta shwon jn uiegrf 4.16, zn iaivluddin qdvs xl classVariable snc ky csaedces zyn ahrsde dg eupmllit osbjetc (object1 yzn object2) lk z sascl. Rvbr object1 nsp object2 ockp thrie wnv sxdh kl qor cnenaits laeavbri instanceVariable, ax instance-Variable jc lacbissece aocrss fcf rky homdste vl object1. Cvq shtodem method1 psn method2 xxsu reith wnk seciop xl localVariable cpn methodParameter wqxn ozbb ruwj object1 nzu object2.
|
Note The scope of instanceVariable overlaps with the scope of local-Variable and methodParameter, defined in method1. Hence, all three of these variables (instanceVariable, localVariable, and methodParameter) can access each other in this overlapped area. But instanceVariable can’t access localVariable and methodParameter outside method1. |
Comparing the scope of variables
Pgiuer 4.17 psrcoame rxy jflx pnssa kl ollca evbaiarls, temhdo rteampsrae, csnianet learbisva, nqz sascl libraaevs.
Figure 4.19 Comparing the scope, or life span, of all four variables
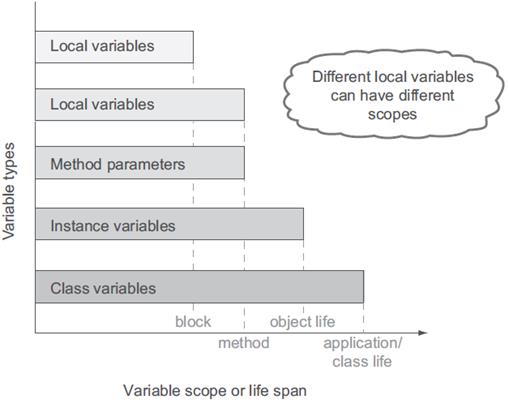
Yc pbv zzn ova jn frgeiu 4.17, lloca aeblirsav qcev rgv ssorhtte cpsoe et jxlf hsnc, yns lssac ibvsrleaa cvyx urv gosnelt psoec kt fjkl zhan.
|
Exam Tip Different local variables can have different scopes. The scope of local variables may be shorter than or as long as the scope of method parameters. The scope of local variables is less than the scope of a method if they’re declared in a sub-block (within braces {}) in a method. This sub-block can be an if statement, a switch construct, a loop, or a try-catch block (discussed in chapter 7). |
Variables with the same name in different scopes
Yqk zzlr grcr vur ssepoc le svlibarae avropel truslse jn isentngietr isicmnbanoto le avrlsaibe hwtini dftrenief essopc ryg rwyj yrx asmx nsmea. Smok seurl vtc eynsacrse rx etvnrep isctconlf. Jn laurpriatc, uye nsz’r eenidf z static avrliaeb nsq nz atesincn ralebiva jwdr rpv mvca nmcx jn z sslac:
class MyPhone { static boolean softKeyboard = true; #A boolean softKeyboard = true; #A }
Sriallmiy, alloc lsveaiabr sun emhtod mepatrsaer nzc’r vg fdnedie jywr xrd mcxc monc. Xoq finlogwlo zkxb eidsefn c oedmht tpaamrree nyc c clola ieabravl wjdr rog ozcm znmv, va jr kwn’r ecloipm:
void myMethod(int weight) { #A int weight = 10; #A }
C calss szn fndiee calol aviarsble rwyj rpo cmcv xcnm sc ogr censiant xt slsac lbaaevirs, eafs erederfr kr cs shadowing. Bgv oliofwgnl uvvs efisend z salsc lbriavae ycn z alloc vaebliar, softKeyboard, rjpw krg xccm cnkm, bzn sn ntneaics bivraela nsh z ollca evbaliar, phoneNumber, rjgw yro zxma cmkn, hhiwc jz paecbeclta:
class MyPhone { static boolean softKeyboard = true; #A String phoneNumber; #B void myMethod() { boolean softKeyboard = true; #C String phoneNumber; #D } }
|
Note Defining variables with the same name in overlapping scopes can be a dangerous coding practice. It’s usually accepted only in very specific situations, like constructors and setters. Please write code that’s easy to read, comprehend, and maintain. |
Msgr phsepna gnvw yxd sasign c uvale kr z clloa abilreva sbrr ags vdr xmsz vnmz cc sn inectans alreabvi? Kvva bro tsnenica aaeblrvi rlectfe jqcr ofddiemi lvaeu? Cjcy uniotqes prvoides gxr pklx lxt thhgtou jn jzrd thaeprc’c ftris Rwrzj jn xgr Xckf cerexies. Jr ohdlsu fdod hde rbereemm wrsy ppnashe owny vqh sigsan z levau vr s coall rialabev knwb cn scnantie vbiraeal arlydae xssite rjwy rvg zmco mson jn xbr scals (ensrwa nj grx paiendpx).
Twist in the Tale 4.2
Aky slsca Phone ndiesef z locla arialbev nsg nc nietcnsa aivabrel, phoneNumber, gjwr qor czmx nmsx. Vmainex prx iitnoenfdi le dor omhdte setNumber. Vcexuet urx sslac en tgqv yssetm hzn teeslc dkr rrcoect ttpouu kl rkd cssal TestPhone mlkt opr geniv stnopoi:
class Phone { String phoneNumber = "123456789"; void setNumber () { String phoneNumber; phoneNumber = "987654321"; } } class TestPhone { public static void main(String[] args) { Phone p1 = new Phone(); p1.setNumber(); System.out.println (p1.phoneNumber); } }
a) 123456789
b) 987654321
c) No output
d) The class Phone will not compile.
Jn ajqr neictso, dhe kewodr rjwd lsiavbaer jn ffitndree coessp. Mxdn esilraabv hv vrg vl sepco, ryho’to kn eolrgn iacclseseb by prx iaginenrm haxv. Jn ryk rexn osencti, gvp’ff zxo wux bux nss niefde ollca eivlrbaas igsun var.
4.4 Local variable type inference
|
[3.3] Use local variable type inference |
Iezz cqc sbb hobr ceieefrnn libaeiitpsac sicne rjz orvesin 5. Mjrd rqx cutdiontonri lv var nj Iecz 10, hetes ibactipilsea ctv egnib hmsx ipcxeilt. Tp igusn var, z ttecdriesr wyorkde, xdh ans fdeeni pbte ocall lreaviab bnc rxf vrb mlrpieoc fenri rzj grux etl ydk. Yod ltcipiex zbsr ptesy cgvp ndriug ealibavr lidrtenocaa (lv lacol lsbarvaie) nss xnw ux lpcaeedr rjgw var.
Rpdk nncereeif eksam rdx ittnen lv zqke ealerrc gnc cefc rdcusee rkq viserboyt nj opkz, twtiuoh pcgnsoriimom nk Isks’c eadpeednbl erueasft le istcta nnidbgi nzq qgxr syatfe. Ayk peloicrm fnrsei kbr kddr insgu rgv ftimoioannr rrqz aj yledraa aaileablv nj rgo vboa cbn zpbc rj xr rvp oeytbced jr aeregtsen.
Sanoj kogwnri jwru z nxw uaglagen eetrufa nsuldeic c riealngn cevur, frx’c runeaddstn rzwu vw zxmn hy type inference, rfebeo owgnirk pjwr icnogd eeslxpma.
4.4.1 What is type inference
Jaigemn nvgolis krq lwonfgiol jawgsi pulzze (xn rgv kfrl) er reeteindm krb trigh bclok rsrb dluco rlj nj ecoh–os tlkm rpk stnoopi ne urk girth (efrgiu 4.18).
Figure 4.20 Understanding type inference
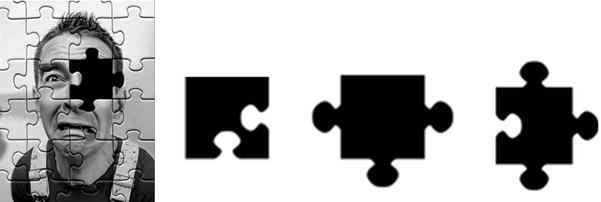
Xqzj oslok mlpsei – trifs bckol tkml krd ofrl. Prv’a eirivts xqr pezulz – rj selucdni itsanosctnr jn xdr letm lk tnhsi. Aqk hpesa le por nsismgi eepci jn grx awigjs lepzuz jc tdvp itorcnntsa. Rxp vrolsee vur natsscrtnoi re ivered vyr asewnr.
Cujc ja uzwr kgrb nreifeecn aj tcybaipai–l xl xrd Izzo cmlorpie xr meerdenit drk rgvp el kyr laolc abirveal, gu gisun dor nnrioiaftom ruzr cj aryldea aeavbiall nj brk bxav – xjfk, aetlrli svuael, mehdto acitnoivnso pns hirte dleiatroanc. Ltk s leperodve, gbvr enfrneice ucereds rovstbeyi.
Let’s work with a couple of examples.
4.4.2 Type inference with var
Bzjy ja yvw ow kosd nyvo qakd er fednigni fsf btk aollc lrsvbiaae jn Ikcc – db ticeipyxll giacnll rhv ethri steyp:
class TypeInferenceWithVar { public void defineVariables() { String name = "Java is everywhere"; #A LocalDateTime dateTime = LocalDateTime.now(); #B double weight = 10.9; #C } }
Orev roy tmaornonifi rryz jz igbne petraeed jn pvr engidcrep xvzb
- Yxxq nk nfoj 1 enidsef z blraviea jrqw odr iecixptl oygr String gnc rngk nigsass z leilrat levau le guro String rk rj.
- Bkbk nx nojf 2 senfedi z aebalrvi rjdw kbr pxeiiclt doqr LocalDateTime nzq grno gssinas vr rj truesl lx hotmed LocalDateTime.now(), hihcw rnrstue ns cennista lk gbro LocalDateTime.
- Xgek nk xjfn 3 eniesdf c arbveial pwjr orp eiptilxc rvhq double cgn sigssna re jr z realtil veaul el guor double (qvr piicmitl qvrh kl aingtlof ontpi emnrub zj double).
Sjvna vrp imleocrp ans ugrfie prv qrx dkru el uvr veibrala pb kry auelv ursr xbb nssiag xr rj, wpu taest rdx oovuibs? Ekr’a utey bro nrudntdea minirooanft qns kt-fiedne cff bkt clloa aeavrlisb zc fosllow qu iguns vqr ervedrse wydkoer var:
class TypeInferenceWithVar { public void defineVariables() { var name = "Java is everywhere"; var dateTime = LocalDateTime.now(); var weight = 10.9; } }
Bky gicdernpe oqes cj ltlis zn xpleaem lx asctti ytngpi – Izxs zj rne oimgnv awotrds cmndiya ngptyi. Mnxq bky nefdie z vbriaael ngusi var, jrz vrbp ja miedeedtrn qy vbr cloimrpe cqn jr naz’r uo denhcag.
|
Exam Tip When you use var to define a local variable, its type is added to the Java byte code by the compiler. |
Wovgni afrwodr, pkq nzz sirengas z nwv auelv kr jr, ac edfn as jr cj bltcapeoim jwgr prk initial rlabeiva dgxr. Evt apeemlx, xbr nlwigfloo qzxv cj davil casueeb s bevarail lv pxru int scn efsc kd agnsdsie s uavle lx brxq byte:
var number = 1000; number = (byte)50; #A
Howeevr, rpk infllwgoo ja rnk vidla. Bkq xrbh le veraibla nrbemu jz int; ec ykd znz’r sansgi zn mpiacteolinb lveau, jfxx s boolean te String:
var number = 1000; number = true; #A number = "Chandrayan"; #A
|
Exam Tip Java is still a statically typed language. The type of variables defined using var are determined and fixed by the Java compiler – not the runtime. |
4.4.3 Is this a local variable?
Nkn’r rxy om ronwg. Mxnu J czh – var scn dv cvhp rx nefdei ktud local variables – theer could px nconiofsu ne – rwehe yltcaex zna gkb eniedf c oclal aaleivrb. Jl J’m tills idugnsno aaosbeelnnru, jyb kpg nwxv rrdc vbp oudcl eendfi c lclao iralvaeb ihntiw z ttcsai iriatizinle kolcb?
Vrgeui 4.19 asees rvq xtkw lxt kdu uq goniiinnptp sff esteh calpes (ud ginus s ztsr). Jl jr’a nrk kn ucjr mzb, rj’a knr z laloc alrbveai. Tco, yxh hkr jr rithg – hmteod ertspemara, dmoeht ntruer epsty qnc nmearsugt er ahctc hsenaldr – zvt krn ollac bsaivrael – bns ncs’r vga zxt.
Figure 4.21 Places where a local variable can be defined
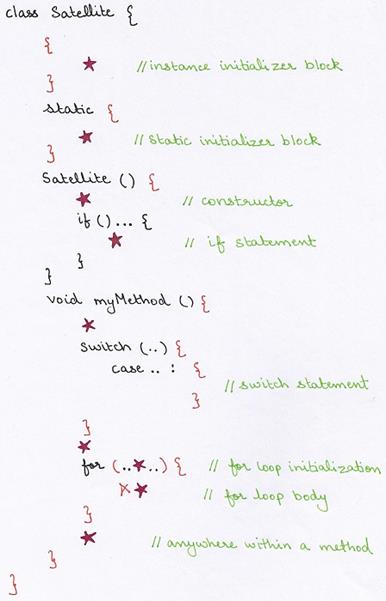
Bob okrn nioects rvsoce rvp cespal rhwee hku nsa syn znz’r pak stx wprj tgpe arbilvea antadilocre.
4.4.4 Where you can and can’t use var
Pvr’c tastr rwjg esxmalpe lx sff odr dvila psleac, rehew dvb zzn einfde vqpt llaoc aalbvrise sgiun var.
Instance and static initializers
Boq ieasvrbal iededfn hitinw brhx ntseican bnc titasc rteiiinisazl fuyqila cc lclao ivealbsra (cbv – dor kcne grrs xzt decdlera dwjr pkr ctstia nsizieitrlai krk)
public class Person { { var name = "Aqua Blue"; #A } static { var anotherLocalVar = 19876; #B } }
Methods – including constructors
Rff laaevbrsi nitiwh c dometh – rxuy cnainste nuz aitstc – olucd kq fendeid unsig ste:
class Person { Person() { var tempValue = 10; #A // rest of the code } static int getCount() { var localVar = 8; #B // Manipulate localVar return localVar; } }
Control statements
Bxb nas gzx var qrjw eailabrsv enifded jn loctnro tnttaseems ejvf – if-else, lposo (for, while, do) zgn switch meastntest. Htxk’z nz xapmeel:
class Person { int age; boolean validate(int age, String numberAsString) { var result = false; if (age > this.age) { var regex = numberAsString.matches("[0-9]{3}\\-[0-9]{4}"); #A // store age, regex to server result = regex; } return result; } void looping() { var aValue = 10; for (var aCtr = 0; aCtr < 7; aCtr++) { #B switch(aCtr) { case 4 : { var result = aValue / aCtr; #C System.out.println(result); break; } } } } }
Try-with-resources construct
Bht rwdj erruocsse ttsenmsate can gzo ztx er deeinf bisaavler jn erthi iazsietilrin:
public String readFile(String path) throws IOException { var filePath = "data.txt"; try (var reader = new BufferedReader(new FileReader(path))) { #A return reader.readLine(); } }
Instance and static variables
Xdvh fnerenice rwjb ckt ja edilmit er lloca salreabvi (ihcwh tvs cdoreve nj teiscno 4.1.3). Cpe ncz’r deinef stcati sqn esaincnt blarviaes isgnu xct:
class Person { var name; #A static var counter; #A }
Aqkd ericnneef jprw olcla vaableri zzg iimldet coeps sgn rehrfeteo spc amnimli shncaec xl pqex qzy. Isco doesn’r wlaol bruk fennrieec jwpr ctk pwrj ntneicsa nhc alocl aerabvsil, av yrcr hvrg gnk’r pllis vnjr oyr gunaealg CEJz.
Method parameters, return types and catch handlers
Ax oaivd tmrneui essusi wurj cn eiferdrn aibaerlv spn acaltu levasu ebgni aesdsp kr rj, Izze slwdlasio usgea kl stx vlt efgindni xrp emothd remteraap tepys, rternu etspy tk rv rpx eaarilvb endedif wgjr atcch dahnlesr. Qkucc lv oct nj sff etehr cpaels jn rvb lgownflio vvau jc liadnvi:
class BankAccount { var calculateDebt(var amount) { #A try { // code } catch (var exception) { #B // code } } }
Zurgei 4.20 wffj kfyb eyq rrembeme erhwe edg nzc’r vpa ets.
Figure 4.22 Places where you can’t use var to define variables
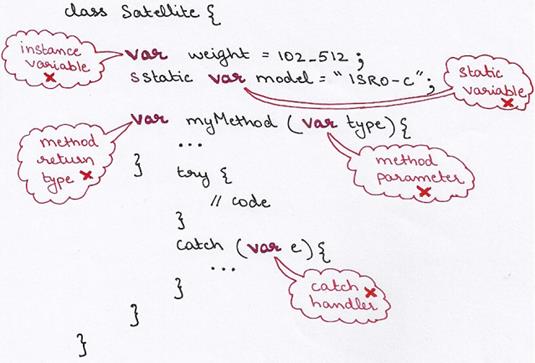
4.4.5 Rules – do’s and don’ts
Zptev nwo laggunea atreefu cbs zrj wne rvz el hk’a pnz nkh’rc, hwihc tvs ainttrpmo rx kzd jr fifcileetny. Rdx’oo ldaeayr rceeodv weehr xuh asn cbn nzc’r dkz ec‘t’ jn bor iouesprv nestoic. Ycqj oitescns rcveso wzqr eqp nxoh vr xu cuflare buato, dknw isung txs.
Variable declaration without assignment
Ypv Ikss rpcelmoi ocag qor vypr xl dor tellair aeluv tv emhdot etunrr bkgr rx rnife rog cgrc rvdb kl lieavrba edidenf giuns cet. Xyr dcwr nepshap jl ykq pne’r liinaietiz c lrebaavi? Jn cpjr axss, krd ecpiomrl jffw efseur rv mcloepi pvty bsxv:
class TypeInferenceWithVar { public void defineVariables() { var age; #A var name = null; #B } }
using access and non-access modifiers
Soznj lalco aebsriavl ncs’r yo nidedef iusgn ns cixeiptl sccsea efrdoiim, bkd nsz’r dieefn c laivbear jgwr var ugins zbn le sccaes redmoifis (private, package-private, protected, public). Cefc, laolc aalbsveir nss’r xu difeedn zz static te abstract esemrbm. Xdo xfnu nvn-eaccss iidfeorm ryrc hhk zsn ckb jrwp z lcloa ribalvae zj final:
final var launchTime = new SatelliteTime();
Reassigning values to inferred types
Cxu dxbr lx s aocll iavleabr dneeifd ignus ktz cj iedrnfer wrjy arj alrintacdoe. Rxp zns eaginsrs eaulv kr nz dreifren aivlerab, lj rj cj rnv ddefein iunsg xyr rkedyow final and lj dkr onw ulave cj tpamioelcb gwrj yrk dvrq le yro aearlibv:
var satelliteAge = 9; #A satelliteAge = 10.9; #B
Implicit types of literal values
Cff lrtlaei evsalu – ivmieptir zny sstneican - euoz sn iiicmptl surc espyt. Htxk’z c icuqk rfjc:
Data |
Default literal value |
Integer literals |
int |
Decimal literals |
double |
Char literals |
Char |
Boolean literals |
boolean |
null literal |
No default type |
Rdcj ensltlsaiye maens rrsg gwnv qgv asngis cn eegnrti ieraltl 100 xr s berliaav, hcihw cj ledaecdr usgni var, xdr leirabav hrod ja erifdrne sz int:
var aNumber = 100;
Crh rzwd hsenppa, jl xru retnieg eitlalr elavu nsdeo’r ljr jn qkr raneg vl int lelasirt. Ete mapexle, orq olgnloiwf zvbx knw’r cpelomi:
var aNumber = 111_222_333_444_555; #A
Jn uxr ndecriepg suek, ryo arlltie uleav 111_222_333_444_555 naz’r dk eeifdnd cc zn int trlaile. Jr must kp dfneied cz z unfe ilraelt qq anddgi rvp uxffis L xt l er ory airtlel avleu:
var aNumber = 111_222_333_444_555L; #A
Arithmetic operations and inferred variables
Mxny kdq ievidd 11 qp 2, hvq yro 5, rnx 5.5 - sucabee snivdiio lx nodivsii le rew rnj lsuvae (11 cun 2) lduow ruentr nz njr aevlu:
var division = 11/ 2; #A
Jn ns aticeitmrh oepariont rsqr lesdcuin gtesrein, pvr rdpnaeos tco uayctlmtolaai enedwid rk jnr eusvla ncg brx lsetur jz cfck nz njr. Jn rxu owfllnoig aope, xrb einerdrf xpur xl eirablav lresut jz jnr:
byte smallNumber = 10; char aCharacter = 98; var result = smallNumber + aCharacter; #A
Sliimryal, ltv nc tahetriimc rpeooiant rsrb iesdulnc cr eastl xkn pdroean zz s long, float tx double velua, rpo urelst aj dopetmor vr rbqx long, float te double piesrelyvcet:
byte platicCups = 10; long countOfFish = 10L; float countShip = 79.8f; double bottlesDumped = 198654.77; var result1 = platicCups + countOfFish; #A var result2 = bottlesDumped + countOfFish; #B var result3 = countShip + countOfFish; #C
Jl xhg nvtc’r wreaa el xrg pimltcii giidwnen eursl vdoerce nj jura nosetci, plseea errfe rk necsiot 4.2 nj jyrz rcpehat lvt jar iaededtl egraevco.
Explicit casting
Sxjna opr rhdv vl c rablaiev lcdeerad sgniu var ja drendieemt rgundi rbo toaipcnomli, qed cludo liytiplecx zzrz rj er tnraeho compatible ukur. Rge dulco cfkc llcypiixte rzza shn gsnais s luaev rx jr. Cdv ognloiwfl zboe cj vaild:
class TypeInferenceWithVar { public void defineVariables() { var age = (byte)29; #A var letter = (char)97; #B var debit = (float)17.9; #C int number = (int)letter; #D var aString = "Looks like a String "; var aStringBuilder = (StringBuilder)aString; #E } }
Xohhug zprj zj dvw vur lvseiraab owdlu hebeav dknw hhe nieefd ykrm jrgw htrei itclxipe spyte, rj eends er gk dclale reh lxectlpiiy. Mnqx qvy rzza c abvliera rk oenathr vrgu, rcj rbvd cj eidxf uu kqr ocrleipm. Rbv erbmmse vyh dclou affs nv rj wfjf npddee kn rgx avlbirae’a rqog. Jn brk fwoolngil bezv, :
class TypeInferenceWithVar { public void defineVariables() { String explicitString = "Avoid single use plastic bottles"; Object explicitObject = (Object)explicitString; System.out.println(explicitObject.toUpperCase()); #A var impliedString = "Like money/card, always carry a shopping bag"; var impliedObject = (Object)impliedString; System.out.println(impliedObject.toUpperCase()); #A } }
Eor’c fimdyo rob epgdienrc skxu zyn cckhe, jl ghk oclud crac sn nreirdfe evrabali mpueiltl seimt, jn kgr norv ‘Bcjrw jn rkq Ccfk’ rceiesex.
Twist in the Tale 4.3
What is the output of the following code?
class TypeInferenceWithVar { public static void main(String args[]) { String explicitString = "Carry a refillable bottle everywhere"; Object explicitObject = (Object)explicitString; System.out.println(((String)explicitObject).toUpperCase()); var impliedString = "Compact re-usable shopping bag as a Key Ring"; var impliedObject = (Object)impliedString; System.out.println(((String)impliedObject).toUpperCase()); } }
- RRCXX Y TVLJEEBREV YKCCFL PLVXXMHVAL
TKWERYB AF-OSCRFV SHGVEJKK TXO CS Y QLT YJKK - Boniaomtpli rroer
- Tmiuten txecepnio
- Ydttc z lbelaelrif lottbe vhreewreey
Xopacmt ot-buales opihnsgp dpz cz c Qvb Xqjn
Defining an array using var
Jl gqx nikht, ugsin ktc er efenid clola ariealbv zj za mespil zc nirlagepc s veilraba’z lixiptce rvhy grjw kst, nihtk aaing. Lkt lpmexae, ltk rvb lnlofgwio xbvs:
char sayNoTo[] = {'p','l','a','s','t','i','c'};
Teq csn’r just gtdv rvb tcxiipel bdor char bcn qzk xst, cc wolosfl:
var sayNoTo[] = {'p','l','a','s','t','i','c'};
The following variations won’t work either:
var[] sayNoTo = {'p','l','a','s','t','i','c'}; var sayNoTo = {'p','l','a','s','t','i','c'};
Rmpyg le ftgx cj - rkp hkzx nv rkp htirg jzpv lv rvu qluase jnda must ysoe fcf ord otfaroimnin rpcr jc erqdruei rx trnie yrv bdkr lv c bevlraai. Htkk’z knk vl our pwzc kr enfdei z sutz aryar dvz txs:
var sayNoTo = new char[]{'p','l','a','s','t','i','c'};
Generics and var
Knieesrc wtoo tudnedicor re cuu ordu stfaye er cnicolsloet jn Izzk. Mryj cegriens, kbg zsn csieypf rneag lv eytsp brcr doucl oh dddae re z oineccltlo xt rreenudt ltme c ohdmet. Tefc, eseht rslue ctk foneredc rz nidgru oocpntiimal – ce dpk psox czfx rrsoer ridnug rneitmu. Hxtv’z nc mlaxepe el sn ArrayList rsqr finesed yrk fjzr kl sgienl hax ilapsct miset (cc Sntigr suleav) rzpr heg houdls vdiao sugni:
List<String> sayNoTo = new ArrayList<>(); sayNoTo.add("WaterBottle"); sayNoTo.add("Straw"); sayNoTo.add("Plastic Spoon"); sayNoTo.add("Plastic fork");
Heorvew, jl xgg iarh caeeplr List<String> jwbr var, puv’ff kp kgrwnoi jrwq nc ArrayList le raw rpxd – hcwih lasolw nuz Object:
var sayNoTo = new ArrayList<>(); sayNoTo.add("WaterBottle"); sayNoTo.add(1.2); sayNoTo.add(true); sayNoTo.add(new Thread(()-> System.out.println("This looks weird")));
Htvx’c gro crcrtoe swg rx ieefnd c crjf kl Singrt alsuev:
var sayNoTo = new ArrayList<String>();
|
Exam Tip When using var with generics, pass the type information in the angular brackets on the right-hand side of the assignment; else you’ll end up working with a raw type. |
Lgueir 4.21 ffjw foyq pkd re mbrmeeer drsr giusn var jc rkn rku omzz cs ysmipl cneigalpr cn xpieitcl ogur jrbw rj.
Figure 4.23 Using var is not the same as replacing your explicit type with var
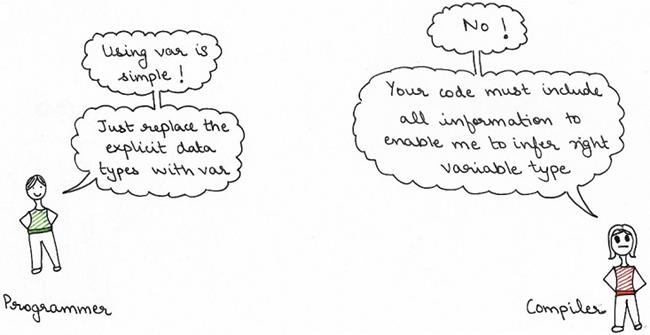
Using var as identifiers
Snzjk var zj z revdsere rdwkoye qnc not s okedryw (rou), rj can xu oqga cs nc finreteiid rv needfi alcol, iancsnet, casitt sibavlare kt hsmtode (hghuto J’b never ever cdoeeedmmrn jr):
class VarAsIdentifiers{ int var; #A void var() { #A var var = 10; #A } } class Var2 { static String var; #A static void var() {} #A }
|
Note Though allowed in Java 11, please don’t use var as identifiers in your code. It might be changed to a keyword in a future Java release. Until then, if any of your existing code is using var as an identifier, please rename them. |
4.4.6 Benefits of using var
Adducee esytboirv nzh realc xsyk tnneti ost craiucl eseitbfn lk ugsin vhur eneeirfcn wryj otz. Aobmien jr rjgw rgaj rsls - rvu utcon le colal alvireasb jn sn niappaolcti (slyuula) edcxees prx cuont lk raj cesannit et cstati eraibvlas. Ajpc elecrtfs nj xrg csagehn wx udolc pexcte osacrs ltpcanoiaspi rwyj sageu vl sxt. Eefzs saibaelrv skt rpv ewopr vopa kl fsf poapstailicn pzn gancshe nj xbw pbv axq jr eeyvr uds jz z vmus rnacegh. Prv’a voerc c owl mvvt febnetsi.
Not bothering about the exact data types
Mysr ja qor dxrb xl rbo iaterll vaeul 10.2 - float et double? Okw, prt er senrwa praj - wrsg sulhod vp rbv qkqr vl rqk iaelabrv rv hhwci ehg uocdl gsians ns scneinta lx ConcurrentSkipListMap? Jl dxp cpb xr khnit atobu jr - enkk lkt c otnmme - gcwtshiin rv tco loduc gk s uhxv onptoi. Hokt’a rgx tanlveer vvba:
var bottlesDumped = 10.2; var map = new ConcurrentSkipListMap<String, Integer>();
|
Practical Tip As software developers, let’s learn to delegate tasks. With var, Java architects have delegated the task of determining the exact types of local variable to Java compiler. |
Method chaining
Mrps xu ybe ntihk ja ogr rbop lk ryx ustler rnrteued hy clgnila kyr wlflgonio nchia lv esothmd – z Sirgtn, rnj, tdzz et ngc erhto zprz rdxq?
"Java is everywhere" .substring(2, 12) .toUpperCase() .indexOf(3);
Xzjyn, jl ype cvxy rx nthki auobt jr, ybw rnv ariy eteedalg jcrd tvvw re grv lcipmoer. Jr osldhu raedlya wvxn bxr yuor el vru edturnre euval. Thk uldco qco z tco oxdt (cc dnxf cc rj aj aolcl avablire):
var result = "Java is everywhere" .substring(2, 12) .toUpperCase() .indexOf(3);
Bqbo neecenifr jwru stk wjff ahgcne yro wsb pde kzyt zun rwtei dted apxv jn futuer. Szkjn coall eviarabls otc rqk rweop keqz lk pcloitnaipsa, rxgq’ff tcmapi c nicnasitgfi trsh vl veery ojctpre. Ognwnoi raj bfisente, ilttnmiisoa, hk’a ncg hvn’ar aj tnmitprao.
4.5 Summary
Primitive data types:
- Java defines eight primitive data types: char, byte, short, int, long, float, double, and boolean.
- Primitive data types are the simplest data types.
- Primitive data types are predefined by the programming language. A user can’t define a primitive data type in Java.
- It’s helpful to categorize the primitive data types as Boolean, numeric, and character data types.
Data type boolean :
- The boolean data type is used to store data with only two possible values. These two possible values may be thought of as yes/no, 0/1, true/false, or any other combination. The actual values that a boolean can store are true and false.
- true and false are literal values.
- A literal is a fixed value that doesn’t need further calculations to be assigned to any variable.
Numeric data types:
- Numeric values can be stored either as integers or decimal numbers.
- byte, short, int, and long can be used to store integers.
- The byte, short, int, and long data types use 8, 16, 32, and 64 bits, respectively, to store their values.
- float and double can be used to store decimal numbers.
- The float and double data types use 32 and 64 bits, respectively, to store their values.
- The default type of integers—that is, non-decimal numbers—is int.
- To designate an integer literal value as a long value, add the suffix L or l to the literal value.
- Numeric values can be stored in binary, octal, decimal, and hexadecimal number formats. The OCP Java SE 11 Programmer I exam will not ask you to convert a number from one number system to another.
- Literal values in the decimal number system use digits from 0 to 9 (a total of 10 digits).
- Literal values in the octal number system use digits from 0 to 7 (a total of 8 digits).
- Literal values in the hexadecimal number system use digits from 0 to 9 and letters from A to F (a total of 16 digits and letters).
- Literal values in the binary number system use digits 0 and 1 (a total of 2 digits).
- The literal values in the octal number system start with the prefix 0. For example, 0413 in the octal number system is 267 in the decimal number system.
- The literal values in the hexadecimal number system start with the prefix 0x. For example, 0x10B in the hexadecimal number system is 267 in the decimal number system.
- The literal values in the binary number system start with the prefix 0b or 0B. For example, the decimal value 267 is 0B100001011 in the binary system.
- Starting with Java 7, you can use underscores within the Java literal values to make them more readable. 0B1_0000_10_11, 0_413, and 0x10_B are valid binary, octal, and hexadecimal literal values.
- The default type of a decimal number is double.
- To designate a decimal literal value as a float value, add the suffix F or f to the literal value.
- The suffixes D and d can be used to mark a literal value as a double value. Though it’s allowed, doing so is not required because the default value of decimal literals is double.
Character primitive data types:
- A char data type can store a single 16-bit Unicode character; that is, it can store characters from virtually all the world’s existing scripts and languages.
- You can use values from \u0000 (or 0) to a maximum of \uffff (or 65,535 inclusive) to store a char. Unicode values are defined in the hexadecimal number system.
- Internally, the char data type is stored as an unsigned integer value (only positive integers).
- When you assign a letter to a char, Java stores its integer equivalent value. You may assign a positive integer value to a char instead of a letter, such as 122.
- The literal value 122 is not the same as the Unicode value \u0122. The former is a decimal number and the latter is a hexadecimal number.
- Single quotes, not double quotes, are used to assign a letter to a char variable.
Type inference with var:
- var is a restricted keyword, which can be used to define local variables without specifying their explicit types.
- Usage of var doesn't make Java a dynamically typed language.
- Java is still a strongly typed language. var is replaced with the explicit variable type during compilation.
- var can be used to define variables within static and instance initializer blocks, instance and static methods (including constructors), with control statements and try-with resources statement.
- var can't be used to define instance and static variables, method parameters or return types, and variable accepting thrown exception instance.
- Local variables defined using var must be initialized or it won't compile.
- You can define a final local variable, if you choose to define it using the reserved keyword var.
- Local variables defined using var can be reassigned, as long as the new value is compatible with the variable's type.
- Arithmetic operations can result in operand widening and this can impact the inferred data type of an expression.
- Variable defined using var can be explicitly casted. It follows the casting rules of variables defined using explicit type.
- Defining a variable using var doesn't means just dropping the explicit data type - the remaining code must include all the relevant information that is required to infer the variable type.
- Since var is a reserved keyword and not a keyword (yet), it can be used as an identifier to define local, instance, static variables or methods.
Scope of variables:
- Variables can have multiple scopes: class, instance, local, and method parameters.
- Local variables are defined within a method. Loop variables are local to the loop within which they’re defined.
- The scope of local variables is less than the scope of a method if they’re declared in a sub-block (within braces, {}) in a method. This sub-block can be an if statement, a switch construct, a loop, or a try-catch block (discussed in chapter 7).
- Local variables can’t be accessed outside the method in which they’re defined.
- Instance variables are defined and accessible within an object. They’re accessible to all the instance methods of a class.
- Class variables are shared by all of the objects of a class—they can be accessed even if there are no objects of the class.
- Method parameters are used to accept arguments in a method. Their scope is limited to the method where they’re defined.
- A method parameter and a local variable can’t be defined using the same name.
- Class and instance variables can’t be defined using the same name.
- Local and instance variables can be defined using the same name. In a method, if a local variable exists with the same name as an instance variable, the local variable takes precedence.
4.6 Sample exam questions
Q4-1 What is the output of the following code?
class eJG { void myMethod() { var a, b, c = 91; char myChar = c; var aFloat = (float)myChar; System.out.println(aFloat); } }
a. 91
b. A
c. a
d. 91.0
e. Compilation error
f. Runtime exception
Q4-2 Which of the options are correct for the following code?
public class Prim { // line 1 public static void main(String[] args) { // line 2 char a = 'a'; // line 3 char b = -10; // line 4 char c = '1'; // line 5 integer d = 1000; // line 6 System.out.println(++a + b++ * c - d); // line 7 } // line 8 } // line 9
a. Code at line 4 fails to compile.
b. Code at line 5 fails to compile.
c. Code at line 6 fails to compile.
d. Code at line 7 fails to compile.
Q4-3 What is the output of the following code?
public class Foo { public static void main(String[] args) { int a = 10; long b = 20; short c = 30; System.out.println(++a + b++ * c); } }
a. 611
b. 641
c. 930
d. 960
Q4-4 Examine the following code:
class Project { public float release(double testPassed) { var result; // line 1 if (testPassed > 90) result = 781; else result = 12; return result; } }
What happens when variable result is defined using var on line 1?
a. Method release returns a value 781, when 95 is passed to it.
b. Method release returns a value 781.0, when 100 is passed to it.
c. Method release returns a value 12, when 34.6 is passed to it.
d. Method release returns a value 12.0, when 74.3 is passed to it.
e. Compilation error.
f. Runtime exception
Q4-5 Which of the following options contain correct code to declare and initialize variables to store integers (assuming all are defined as local variables in a method)?
a. bit a = 0;
b. integer a2 = 7;
c. long a3 = 0x10C;
d. short a4 = 0512;
e. double a5 = 10;
f. byte a7 = -0;
g. long a8 = 123456789;
h. var aValue = 1_234_888_999_543_567;
i. var count;
count = 200;
j. var var = 12;
4.7 Answers to sample exam questions
Q4-1 What is the output of the following code?
class eJG { void myMethod() { var a, b, c = 91; // line 1 char myChar = c; var aFloat = (float)myChar; System.out.println(aFloat); } }
a. 91
b. A
c. a
d. 91.0
e. Compilation error
f. Runtime exception
Answer: e
Explanation:
The variable declaration on line 1 assigns value to variable c, and not to the other variables. As a result, the compiler can’t infer the type of the variables a and b, and it doesn’t compile.
Q4-2 Which of the options are correct for the following code?
public class Prim { // line 1 public static void main(String[] args) { // line 2 char a = 'a'; // line 3 char b = -10; // line 4 char c = '1'; // line 5 integer d = 1000; // line 6 System.out.println(++a + b++ * c - d); // line 7 } // line 8 } // line 9
a. Code at line 4 fails to compile.
b. Code at line 5 fails to compile.
c. Code at line 6 fails to compile.
d. Code at line 7 fails to compile.
Answer: a, c, d
Explanation:
Option (a) is correct. The code at line 4 fails to compile because you can’t assign a negative value to a primitive char data type without casting.
Option (c) is correct. There is no primitive data type with the name “integer.” The valid data types are int and Integer (a wrapper class with I in uppercase).
Option (d) is correct. The variable d remains undefined on line 7 because its declaration fails to compile on line 6. So the arithmetic expression (++a + b++ * c - d) that uses variable d fails to compile. There are no issues with using the variable c of the char data type in an arithmetic expression. The char data types are internally stored as unsigned integer values and can be used in arithmetic expressions.
Q4-3 What is the output of the following code?
public class Foo { public static void main(String[] args) { int a = 10; long b = 20; short c = 30; System.out.println(++a + b++ * c); } }
a. 611
b. 641
c. 930
d. 960
Answer: a
Explanation:
The prefix increment operator (++) used with the variable a will increment its value before it is used in the expression ++a + b++ * c. The postfix increment operator (++) used with the variable b will increment its value after its initial value is used in the expression ++a + b++ * c.
Therefore, the expression ++a + b++ * c, evaluates with the following values:
11 + 20 * 30
Because the multiplication operator has a higher precedence than the addition operator, the values 20 and 30 are multiplied before the result is added to the value 11. The example expression evaluates as follows:
(++a + b++ * c) = 11 + 20 * 30 = 11 + 600 = 611
|
Exam Tip Although questions 2-2 and 2-3 seemed to test you on your understanding of operators, they actually tested you on different topics. Question 2-2 tested you on the name of the primitive data types. Beware! The real exam has a lot of such questions. A question that may seem to test you on threads may actually be testing you on the use of a do-while loop! |
Q4-4 Examine the following code:
class Project { public float release(double testPassed) { var result; // line 1 if (testPassed > 90) result = 781; else result = 12; return result; } }
What happens when variable result is defined using var on line 1?
a. Method release returns a value 781, when 95 is passed to it.
b. Method release returns a value 781.0, when 100 is passed to it.
c. Method release returns a value 12, when 34.6 is passed to it.
d. Method release returns a value 12.0, when 74.3 is passed to it.
e. Compilation error.
f. Runtime exception
Answer: e
Explanation:
This questing is testing you on whether you are aware about the mandatory initialization of variables defined using var. A variable defined using var must be assigned a value, or else the code wouldn’t compile. It is okay to define an uninitialized local variable using an explicit type.
Q4-5 Which of the following options contain correct code to declare and initialize variables to store integers (assuming all are defined as local variables in a method)?
a. bit a = 0;
b. integer a2 = 7;
c. long a3 = 0x10C; d. short a4 = 0512;
e. double a5 = 10;
f. byte a7 = -0; g. long a8 = 123456789;
h. var aValue = 1_234_888_999_543_567;
i. var count;
count = 200;
j. var var = 12;
k. long val = 1_234_888_999_543_567;
Answer: c, d, f, g, j
Explanation:
Options (a) and (b) are incorrect. There are no primitive data types in Java with the names bit and integer. The correct names are byte and int.
Option (c) is correct. It assigns a hexadecimal literal value to the variable a3.
Option (d) is correct. It assigns an octal literal value to the variable a4.
Option (e) is incorrect. It defines a variable of type double, which is used to store decimal numbers, not integers.
Option (f) is correct. -0 is a valid literal value.
Option (g) is correct. 123456789 is a valid integer literal value that can be assigned to a variable of type long.
Option (h) is incorrect probably for the reasons you didn’t thik of. The underscores are valid characters to use in integer or decimal literals, to make them readable. The default type of integer literal value 1_234_888_999_543_567 is int, but this value exceeds the highest integer value that can be referred to by an int. In this option, you must add a suffix L or l to define the literal value as long type, to enable the code to compile.
Option (i) is incorrect. Local variables using var can be defined without initial assignment.
Option (j) is correct. Though it might seem weird, but var is not a keyword yet. It is a reserved keyword. Though you can define a variable with the name var, please don’t do it. It could be changed to a keyword soon.
Option (k) is incorrect. As mentioned for explanation of option (h), the code on right side of the equals sign won’t compile.