Chapter 10. Building and testing web applications
This chapter covers
- Groovy servlets and ServletCategory
- Groovlets
- Unit and integration testing of web apps
- The Groovy killer app, Grails
While Java on the desktop has its adherents, Java found a true home on the server side. Java’s growth and adoption in the early days neatly follow that of the web itself. It’s a rare Java developer who hasn’t at least worked on a web application.
In this chapter I’m going to look at modern web application development and where Groovy can make the process simpler and easier. Sometimes Groovy just simplifies the code. Other times it provides helpful testing tools, like Gradle and HTTPBuilder. Finally, there’s the most famous framework in the Groovy ecosystem, Grails. I’ll review them all and try to place them in the overall context of web applications.
Figure 10.1 is a guide to the technologies discussed in this chapter.
Figure 10.1. Guide to the technologies in this chapter. Spring provides mock objects for testing that are also used in Grails. Using plugins and some configuration, Gradle builds can do integration testing of web applications. The ServletCategory class makes session, request, and other objects easier to use. Groovlets are a quick way to build simple applications. Finally, the HTTPBuilder project provides a programmatic web client, and Grails applications use Groovy DSLs and elegant metaprogramming to combine Spring and Hibernate in a standard convention-over-configuration framework.
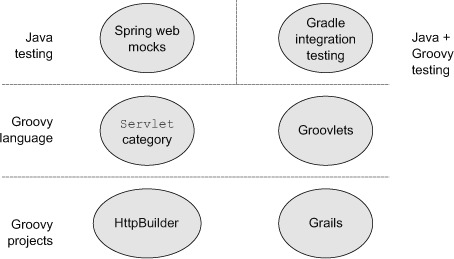