Chapter 9. RESTful web services
This chapter covers
- The REST architectural style
- Implementing REST in Java using JAX-RS
- Using a Groovy client to access RESTful services
- Hypermedia
RESTful web services dominate API design these days, because they provide a convenient mechanism for connecting client and server applications in a highly decoupled manner. Mobile applications especially use RESTful services, but a good RESTful design mimics the characteristics that made the web so successful in the first place.
After discussing REST in general, I’ll talk about the server side, then about the client side, and finally the issue of hypermedia. Figures 9.1, 9.2, and 9.3 show the different technologies in this chapter.
Figure 9.1. Server-side JAX-RS technologies in this chapter. JAX-RS 2.0 is annotation-based but includes builders for the responses. URIs are mapped to methods in resources, which are assigned using annotations. Resources are returned as representations using content negotiation from client headers.
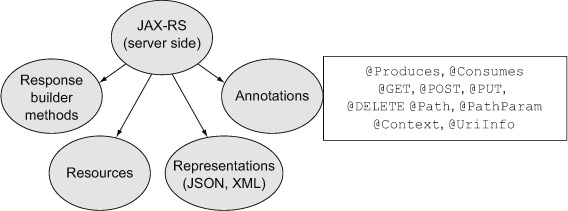
Figure 9.2. Client-side REST technologies in this chapter. Unlike JAX-RS 1.x, version 2.0 includes client classes. Apache also has a common client, which is wrapped by Groovy in the HttpBuilder project. Finally, you can use standard Groovy classes to parse requests and build responses manually.
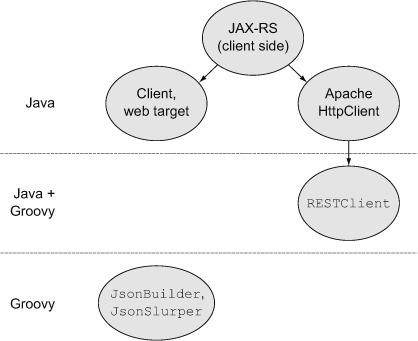