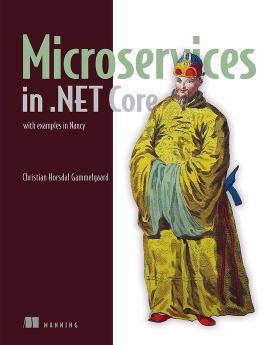
About this Book
Microservices in .NET Core is a practical introduction to writing microservices in .NET using lightweight and easy-to-use technologies, like the awesome Nancy web framework and the powerful OWIN (Open Web Interface for .NET) middleware. I’ve tried to present the material in a way that will enable you to use what you learn right away. To that end, I’ve tried to tell you why I build things the way I do, as well as show you exactly how to build them.
The Nancy web framework, used throughout this book, was started by Andreas Håkansson, who still leads the project. Andreas was soon joined by Steven Robbins, and the two of them made Nancy great. Today Nancy is carried forward by Andreas, Steven, the Nancy Minions (Kristian Hellang, Jonathan Channon, Damian Hickey, Phillip Haydon, and myself), and the broader community. The full list of Nancy contributors can be found at http://nancyfx.org/contribs.html.
OWIN is an open standard for the interface between web servers and web applications. The work on OWIN was started in late 2010 by Ryan Riley, Benjamin van der Veen, Mauricio Scheffer, and Scott Koon. Since then, a broad community has contributed to the OWIN standard specification—through a Google group in the early days, and now through the OWIN GitHub repository (https://github.com/owin/owin)—and to implementing OWIN.