15 Designing solutions with recursion and backtracking
This chapter covers
- Recursion for designing solutions to programming problems
- Dynamic backtracking to try different solution paths during run time
Recursion is a software design technique that we can use to create solutions to certain programming problems. It involves a function that calls itself, and nearly all modern programming languages support this important technique. If we use recursion properly with certain classes of programming problems, we can design solutions that are simple and elegant — and some might claim magical. With recursion, can design solutions that may be difficult to solve otherwise.
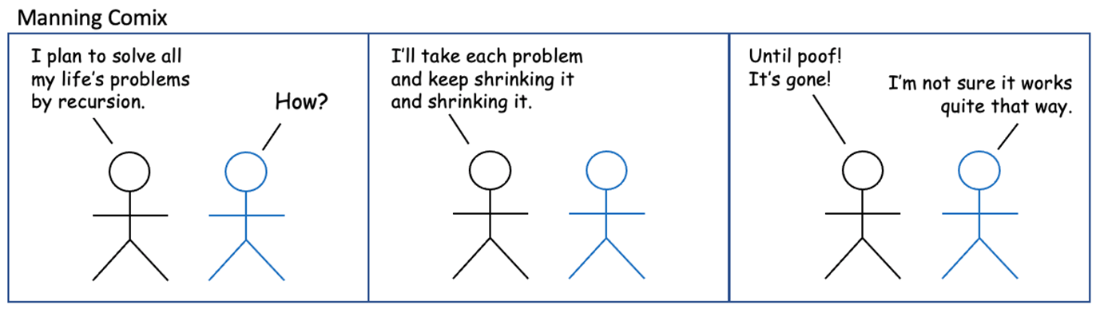
Recursion requires a different way of thinking about how to design a programming solution to a problem. Unfortunately, for some programmers, it is too mysterious and forbidding to use. This chapter clears away the mystery and demonstrates how combining recursion with dynamic backtracking gives us even more powerful design tools. But we’ll also see how misusing recursion can cause surprising performance disasters. With experience, we can learn when it is appropriate to design with recursion.
15.1 Recursion compared to the for loop
We can remove some of the mystery behind recursion by comparing it to the familiar for loop.