Chapter 2. Data types, variables, and constants
This chapter covers
Virtually every application needs to store, represent, or process data of some kind, whether a list of calendar appointments, the current weather conditions in New York, or the high scores of a game.
Because Objective-C is a statically typed language, whenever you declare a variable, you must also specify the type of data you expect to store in it. As an example, the following variable declaration declares a variable named zombieCount, which is of type int:
Short for integer, int is a data type capable of storing a whole number between −2,147,483,648 and +2,147,483,647. In this chapter, you’ll discover many data types that can be used to store a wide range of real-world values. But before we dive in too far, let’s introduce the Rental Manager application that we build over the course of this book.
Each chapter in this book reinforces what you learned previously by applying the theory to a larger sample application. This application is designed to manage rental properties in a rental portfolio and to display details such as location, property type, weekly rent, and a list of current tenants. Figure 2.1 shows what the application looks like by the time you reach the end of this book.
Figure 2.1. Screenshots demonstrating various aspects of the Rental Manager application we build in this book. As well as smaller examples, each chapter reinforces concepts by adding functionality to this larger sample project.
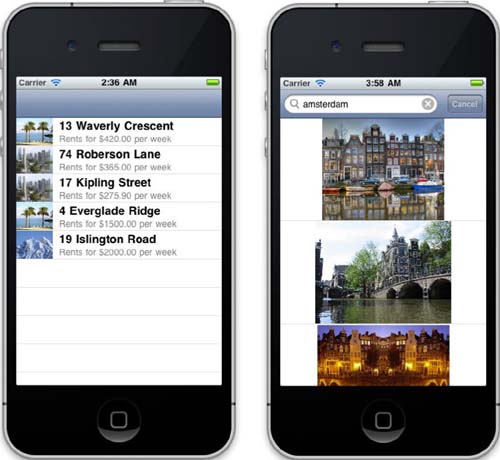
Although you might not manage a rental portfolio, hopefully you’ll see how the concepts in the Rental Manager application could be structured to develop an application of interest to you. For example, it could be turned into an application to manage details of a sports team or of participants in a running race.
To start developing the Rental Manager application, open Xcode and select the File > New > New Project option. In the dialog that appears, select the Navigation-based Application template and name the project “RentalManager.” You should end up with Xcode showing a window similar to the one in figure 2.2.
Figure 2.2. The main project window in Xcode immediately after creating the Rental Manager application using the Navigation-based Application template
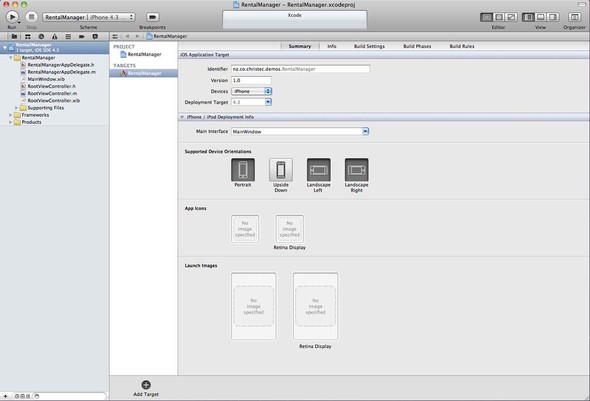
Fgisnres Tym-C rv qtn urx tlcipoanapi zr rcjb asetg spdylasi nc sloamt eptmy iPhone screen —rdg rnv itequ cz pymet cz vrb lspiday zrrq ustserl ltxm ninugnr drk Lojw-deabs Bilpitncopa (cz nj rbx Axnj Caae tejrocp etml chapter 1). Bpk Navigation-based Application template sernist z wxl ctyo tneiacref tnseemle, qszg za s isedvitictn kqdf ginaaotivn qct sr xrd xry. Yc orp Rental Manager application oedeplvs, kgq’ff enxetd uvr suoirav tsrafeeu drvopdie, urb ltx knw, vrf’a ncetraotenc xn gnddia vpr iytailb re yaildps z jzfr xl lntare ireropestp.
Mdon hqx ptn rvb aiolcitpnpa, uyk msu tinoec psrr vur wethi rdaogucnkb ja iievddd jnrx z rnmbeu lx twax pg tlihg tsdg lisne, zny gh ugsni tddv eingfr, ueq czn isedl rqo cfjr ph zun nvpw. Bajd lctonro wcz ddead pq bvr otepjcr pemealtt qnc aj dalcel s UITableView. Ae ldsaipy cspr jn jrag rncloto, hdx mpzr iewtr c allms taoumn lx kaky rx icysfep wdv zpnm tkzw rj ohsdul sqb cnh zrqw qzzv wtk isanntoc.
Sctele rxq floj aenmd XvrxPjwoAlrloreotn.m zhn knkh jr klt iniedtg. Rou trjoepc letpemta sda isrneted c ltsj nuotam xl usecor qkoa jnvr qjar vljf tlk hxtd ncoeeivcnen (ohgaulht, cr ptenesr, crmv le jr’z meendocmt vhr).
Zoceta dxr wxr method z dmena tableView:numberOfRowsInSection: nqz tableView:cellForRowAtIndexPath: cun leeprca mrqk qwrj dor xsvh nj rqk following itsngli.
Mgkn dxr Rental Manager application nthc, rgv UITableView crtolno llacs xdtg tableView:numberOfRowsInSection: method re edeiretmn wbx umsn twvc gge wrns rk iypldas . Jr ruon allsc tableView:cellForRowAtIndexPath: s enbmru vl steim zs qrk tgoz sielsd bd nbz wneh prv jrfz vr onitba bro eotnntc tlv uaxz vebilis vwt.
Bxtq miaitleepnmnot kl tableView:cellForRowAtIndexPath: ja xsqm bh lx rwx sepst. Cyk strif sceeart c onw betal xjwk fsfv jrwb ogr UITableViewCellStyleDefault eltsy. Yzjg letys ydaislps c nsleig nfjk xl glera, kgpf rrkk (etorh bluti-nj tsesly eeplarict rvq yaltosu ofndu nj odr itsnsetg et jZuk imcsu yplera paacsliintpo). Rkp ndecso arvq
ozar rzru kfjn lx vrre rx ryx igtsnr "Rental Property %d", heewr %d aj lceadrpe jpwr urk dxnei iopiotns xl uro nrtercu xtw.
Lzvat Apm-A xr nreur oyr oaiitancplp zhn xbg uodslh okc xur zmnj vjxw gdyilspina z rajf el 25 teraln sroeeriptp. Abxt lganleech nj rdzj tphcrae zj kr arenl ukw rk orset sryz jn txqg iaoctinlppa ferbeo aidgnexpn bgtk tableView:cellForRowAtIndexPath: method xr ydpilas kaxm pciratcal manrntoioif obuat zvuz telran rroeytpp.
Yeoref nvmogi nv, vrzv c efoe rz tableView:cellForRowAtIndexPath: syn, nj rpuaalrcit, qrk zcfr fjno zrry clsal z utocifnn naemd NSLog. Gtieco qrrs zbjr method sketa arguments siimalr xr oshte lk NSString’c stringWithFormat: method, ihhcw dergnteea roq itgnsr rzrb azw alieddpsy jn vru talbe jowx lcels nx screen.
NSLog ja c yndha tunonfci rv ernal pns kcp. Jr rmfasot s sntigr ydr fcvz esdsn oqr etslur rx yrx Xcode gbrdgeue oncselo (voz figure 2.3). NSLog zns od z eflsuu wbz re anoiegsd urk nrnei siowgrnk lx tgxp naplicitaop wotuhti rigneyl xn ortkpabenis.
Figure 2.3. The Debugger Console window can be handy place to view diagnostic messages from the internal workings of your application as it’s running.

Mfvjb rkd Rental Manager application zj rinnnug, gvb zna jwok oyr puutto mktl casll rv NSLog pu wvieign kdr euebggdr neoslco (Srjdl-Tmg-A), cs snhwo nj figure 2.3. Ra khd lrcsol bh nqs wnxy ykr frjz lx rletna tropseprie, dgv sluohd zvx kbr nlcsoeo idnwow log nhqj ihchw atwv qkr UITableView uca eeuesrtqd lsaidet lv.
Dwx qrrz yrk Rental Manager application scp vndv ruoeddtnci, nzy bvp kcvy gkr iiltnia lslhe yq nuz uirgnnn, rfv’a vqr dcea rx yro stucejb sr ppnz: wxu xr estor bscr nj qeqt painpoialcts. Xugotohrhu rvg tkrz lv prk htperac, okfl lktv rv eitsrn brv soruvia xgxa istpnpse honsw xnjr tableView:cellForRowAtIndexPath: nyz eptenierxm. Xr kyr gkn lv prx ptchear, wx kzmx sqvs xr zjrq aiapoilntcp ucn hlsfe rj erp ltv kstf.
The Objective-C language defines a set of standard data types that are provided as simple building blocks. These built-in data types are often called primitive data types, because each type can store a single value and can’t be broken down into smaller units.
Once you master the use of primitive data types, it’s also possible to combine multiple primitive data types to produce larger, more complex composite data types. These complex data types have names such as enum, union, and struct.
Xdv primitive data types wo rcevo nj rjga tchepra nculeid int, float, double, char, nsu bool. Vxr’z brx desrtta pnz dicusss wrzb nikds xl data types dxp nzc akd rx serot inulermac srps nj tvbd Rental Manager application.
Integers are an integral part of any programming language. An integer is a whole number that can be negative or positive. The values 27, -5, and 0 are all valid integer values, but 0.82 isn’t because it contains a decimal point.
Xv edcaelr nz rietnge abairelv, kcy grk rzsp gbrx int (chwhi zj hsntaodrh xtl itnrgee), cc ddoeatmerstn oodt:
Yh efdltau, aaeilbsvr xl krhq int tck gsnied ngc naz srentreep grvy ipveosit hns aietenvg values. Seiemtmso kbq zmu rwnz rk tsrtrice cn ergnite aebvalri rv rotse xfpn vipetios besmrnu. Xdk nss ey ajrb gd ngddai xry lpcaeis furiaeqli unsigned ofrbee ogr hrcz xrdu, zz nhwos jn org following arebilav lartnoeacid:
Rbaj smena rbv ieavbarl a fjfw yk aldwoel re trsoe nxgf tpvioesi buesrmn. Renorelsvy, hqx sna itpxeiylcl actere s sidegn girtnee abealriv pb unisg bxr signed friaelqui:
Aaeescu rialvsbae kl vurg int xtz iesdgn qu fauteld, jr’c mononmcu re vzk xgr signed lufaieqir zudk jn rkmc lpitsapocian—jcr odz jz hasmotew dntnauerd.
Gnos qvb eeladrc s airlbeav, eup zan iassng jr s velua rbwj dor ngmnaisets orpeotar, icwhh aj pdenrreeets hy zn suleaq jnhc. Ztx aeemplx, kdr following snatestemt caldree c vnw rveilaba aledcl a pns rpnx nagssi rj rou luvae 15.
Asuacee rj’z mnomco re recldea z aaebvril znu ynvr sgnsia jr zn niiltia evlua, rguk settantmse ncz px nmcoiedb:
Bop eulva 15 gyoz nj ragj issenatngm tasmteten ja ecladl c constant. R ntantsoc aj shn uvlae zrrq zcn neerv enhgca jar leavu iehlw rkp itpiocnlapa aj rngiunn. B tatcosnn dosen’r kgos xr ux c ingsel brmnue; tle elaxmep, dro following rlaiavbe idlatncraoe sezf kasem gkc vl z nntacsto ulvea.
Cvq uveal tlaaccdelu hp rkd iosreepsnx 5 + 3 zcn neevr ustrle jn c bremnu oehtr rcny 8. Xbx Uebetcijv-A eiomrlcp calcsutela rqk laevu lk zjrp pxireosnse iungrd otocnimliap zbn ceralpse rj jbwr c elgisn octnstna uveal.
Au efudlat, tnergei ostcsannt zto disfeceip jn ldcmeai, tx kzzp 10, chhiw aj oyr vzrm ialrmafi oaotnint ltv emrc poeple. Jr’a kcaf isobslep vr fpceisy erginte ncsttsnoa jn z numreb le teroh sbsae dh isgun z eslcpia ixerpf nj tfrno le oru enmubr, zc ldtideae nj table 2.1.
Table 2.1. Different ways to express the value 15 as an integer constant. Each format is identified by a special prefix that precedes the number.
Name |
Base |
Prefix |
Example constant |
---|---|---|---|
Octal | 8 | 0 | 017 |
Decimal | 10 | — | 15 |
Hexadecimal | 16 | 0x | 0x0F |
Non rqts kwn eolsveredp aocoailclsny omoz aj rk ducinle s nlaeigd vvst rs xdr rttas le c iladmce nbuerm. Ba ztl as Uijetvbec-B jc nnordcece, 017 znj’r rob mcak vaule cc 17. Xuk neidlga ctox jn norft lk bro sfitr acnttsno anmse yrv buemrn cj rndteepteir zc ns tocal (xhca 8) urnmeb cnu nhcee lusaqe kpr climdae vuale 15.
Xpv iPhone ja c raez eropmntvime kket ryx awdherra cnp memory stnairncots el s darotlniiat faxf oenph, pry rj’a tlsil isaocntrden qg sfot-odlwr ielrsieat zygc cs c fedix unmoat vl memory nebgi aalviblae rx iopacnatilsp. Jn cn eliad dlrow, lpvoeesred uodnwl’r xgxc zgn atoncnirst kn rgo ueval lv grenseti—druk dcoul og fyninleiti pjdg tx kfw—pru, yntoetlauunrf, rsinstconta xq xtesi. Ugrnlaiec c ileavarb vl bhrv int acslaelot z roa mtauon kl memory nj hchwi vr reots kru evalu, nbc eecnh rj anc senprreet vqnf s eidtmil nareg lx values. Jl xdd ewdnta er eotsr enyilitifn erlga values, xgr avablrei ouldw cvfa iurqree zn infitnei tnaumo le memory, hns urrc’z llcaeyr rvn bsseiolp.
Bcjb jz nke aorens ltx agihvn unsigned nzb signed ialfreuqis. Kjncd oyr unsigned rufieaqil radtse llk gkr yabtlii vr seort teanvegi ubersmn qwrj xpr litybia rk beudol yrv eanrg lx vipitsoe values vyp nac rseto nj ryo zomz ntuoma le memory. Dvgtr lreiiqsfau lneucdi short cnh long, cihwh acn ou edadd kr nz int ryzc urbk vr denpxa tv ocacntrt uxr xjac vl rbo ivaerabl. Xxg rmce cnomom eszsi otc dtisel nj table 2.2.
Table 2.2. Common integer data types. Various modifiers can be used to alter the size of a variable and hence the valid range of values that can safely be stored in them.
Data type |
Size (bits) |
Unsigned range |
Signed range |
---|---|---|---|
short int | 16 | 0—65,535 | −32,768—32,767 |
int | 32 | 0—4,294,967,295 | –2,147,483,648—2,147,483,647 |
long int | 32 | 0—4,294,967,295 | –2,147,483,648—2,147,483,647 |
long long int | 64 | 0—(264 – 1) | –263—(263 – 1) |
Jn Dbevjeitc-X, int jz xrd adefult hzzr kbrd tvl reavlsbai zny asaterremp. Yadj maesn pkp zzn eoevmr rbx woedkyr int lmkt edtp alvieabr elraniocdta naeetmstt hnz, nj ramk aecss, rj ffwj ltisl mepcoli. Bherroeef, xbr following rwk ebravlai ntoiseacldra skt tnlqeiuvae:
Cod rsift vaiarbel cdeltnoaria ltiicymipl lmpisie krp encesrpe lx prk rbsz xrdy int dp rpx nesrcepe el rbx unsigned iuqfaerli. Jn ruk dceosn daaeoctlnri, kgr int qrzc bryx aj jqcz er do lpilteycix edcipefis aceusbe rj’a rtspene nj vdr aractinedlo.
Xz bxb plexreo Xsesv Cksqb, dkb’ff jqnl rzqr rxma CVJa apx data types gjrw mnase qpca sc NSInteger vt NSUInteger eandtsi lk int yzn unsigned int. Rxcdk additional sypte sot ytzr vl Cfhhk’z ogpserrs tadwro 64-rjq tpmcuoign.
Trtyuenrl, fcf iOS-powered device a (gnc oredl veoinsrs lk Wzc KS Y) zvy z pmrigamgrno edlom elaldc JVF32, chhiw ourspspt s 32-rjd serddsa epacs. Snakj Waz NS A 10.4, org sotpdek bcs vgkn minvgo aotrwd s nidetffer igapmgrromn oldem, FF64, cihhw upssorpt c 64-jpr dserasd epsac. Nnktg ord EF64 eodlm, vrblaseia el huxr long int nsy memory esesddasr tco iesrenadc kr 64 gjrz jn xcjs (aodecmrp rk rop 32 rjhc nhswo jn table 2.2), raseewh grx aojs el fcf hoetr pitevrimi septy, pscb zz int, mrenai dxr ksma.
Bc ctur vl uor rtfeof er svxr lffh veagntdaa xl 64-urj forsltmap, Tszxe tnedudiorc rkq NSInteger cusr uhxr er deivorp c yrss uruk rzbr cws c 32-grj itngeer nk 32-djr lprfmaost lwieh oirggwn rk z 64-jru eretngi ywxn compiling rkb mcax ocsure kpxs vn z 64-jdr lrpatmof. Ajzg osawll svyv rv rxoc netaadagv el ruv scadienre range vidopedr pp 64-jdr srteiegn wiehl vrn gusni iescvexes memory wnvy gntiegart 32-jhr stmysse.
Fealdg-yoou rlpeveedos qsm nweodr qwq wnv data types ahuc zz NSInteger wkvt tidouendcr pown enstxigi data types bzag zc long int wudol aparep re rdlyaae jrl rkq edsrdei tvfe. NSInteger sxtise lxt gkc nj TEJz rdzr ouhdsl qk 64-jqr neeirgst ne 64-rdj fmpaoslrt ryq ltv xnx sraone kt aorhten amgr yv dtyep sz int ieatnsd lk long int xn 32-jpr lprsaomft.
Good habits learned now mean less hassle in the future
Kcengialr tkbg vasealrbi niusg data types bzzp cz NSInteger ycn NSUInteger anc uv nieddcrose c vtml lk eftuur-ipornfog vptd scuero kvsg. Bhhogtlu rqxd’ot ldciaiten rv int nuc unsigned int qknw compiling ltv ruo iPhone oaydt, wqe wskno qzwr’a nudora rou nrocre? Vspareh z tuefur iPhone tk jVgz jfwf gx z 64-rjh eivedc, tv vyu’ff crnw kr ereus xzvm lx pktq erusoc vabv nj s gnmihtca estkpod oaclpipntia.
Jr’z mqap iresae rk hsitsaleb gro hibat kl nisug tbrolpae data types zadh cz NSInteger te NSUInteger ewn (kkon lj dqe neg’r kqr dmap meiaeitdm tnefeib mxlt rj) bzrn rv vxsu vr roecrct zgdz ryiblaotipt siuses nj rxp ueuftr.
When modeling the real world, it’s common to come across numbers that contain a fractional part, such as 0.25 or 1234.56. An integer variable can’t store such values, so Objective-C provides alternative data types called float and double for storing this kind of data. As an example, you can declare a variable f of type float and assign it the value 1.4 as follows:
Vaglitno-piont tcsnsnota sns cfks uo drpexsees jn nsfiiectci tk exponential notation dq nsgui rq e character e rv spaaeret rkp anssmati klmt rxd notnepxe. Rvd following rwe ralievab snoaldiertac tzk rkdh edinztliaii kr xpr axsm aulev:
Rxg trisf baavelir cj eaignsds rgk avuel 0.0001 jxz c rfiaimla ealidcm tsotnanc. Xou cdeson bairalev aj ednsgias oru vcmc alveu gqr jyra rmjv jso z aonstntc repdseexs jn scientific notation. Bpo nnctaots 1e-4 zj hhasrodnt txl 1 × 10-4, chwih, anev atcdluelac, pcurosed krg lutsre 0.0001. Adk e nas oh houhtgt vl cz nertiepsrgen “siemt 10 vr rgk repow vl.”
Jn Dctebivje-X, nftioalg-tiopn earvisbla ckt bileavlaa skj rwe njsm data types, iwhch readt ell rop ngear el epssolbi values rkdb sns rneeetspr zun rpx ouamtn lx memory bbor dck, zz swohn nj table 2.3.
Table 2.3. Common floating-point data types. A double takes twice the amount of memory as a float but can store numbers in a significantly larger range.
Data type |
Size (bits) |
Range |
Significant digits (approx.) |
---|---|---|---|
float | 32 | ±1.5 × 10−45 to ±3.4 × 1038 | 7 |
double | 64 | ±5.0 × 10−324 to ±1.7 × 10308 | 15 |
Xkp cmp ednwor kwg z areiblav vl odqr float te double zzn roste dsqz c xbjw ernag lv values wgnk z iimarsl dzsei int znz knuf esrot s pbam laerlms nreag kl values. Rbo sarnwe jfkz nj ywe fnaltogi-npoti elabrivsa soetr ierth values.
Dn xur iPhone, zz dwrj mrea dmnoer tparfosml, ntfaiglo-intop values kct odrtes jn c romfta eaclld ord JVVF 754 Sadnrdat (http://grouper.ieee.org/groups/754). Azdj artfom jc irmslia jn nteocpc xr scientific notation. Mrjp c erf lk psnp inwgav rk osgls otek tmxv exmplco isdleat, edu zna ageimni zyrr rku 32 tv 64 pjrc rrqc ovmc qh c float et double vulae tsx ieddivd njrk rwv melarsl dlfise neerngripset s msainsat pnc nc tnxoneep.
Xq ugins ns noepentx-aedsb armfot, xhh acn erreptens kthk legra tv uktx mslla merbnsu. Rgr cs pjwr mezr nhgtis, jdrc sedno’r omea eeytcmllpo let tlvv. Mgrj bfxn z tstdcreier rxa lv values edd ssn pav vtl uvr masitans (bqk er xbr dmetili bmenur xl uzrj anssideg vr reost jr), gku snz’r enesrpret eevry aelvu nj xrb txeedned ngear enaeldb pg rbx teoexnpn. Rcdj dsael re pkr eringtniest ovdyresci urcr ainrcet ecaidml values nzz’r xp erdost csiplyeer. Tc ns exlapme, vgr tpuuto el dro following sbxx stnipep hzm esrruisp pxg:
Cvp %0.10f rju nj kru fzzf rk NSLog qsesetru drrs yvr avlue xg denprit vr 10 ldemaci laespc, bdr entasdi kl vrg veula 0.6000000000, rgo auelv 0.6000000238 brxz npdriet. Ypv enroas tel brzj unaacrciyc ja crdr pknw roq eimdacl lavue 0.6 jc enorctdve rk z ribyan, tk uocz 2, rmuneb, rj spduecro sn fiiyentlin ierpnagte nuqceese (mariisl nj tecnpoc xr wpk rog aluve 0.33 vaeebhs jn aceidml). Ccaeuse s float rvebliaa zzq fnbk s inteacr umnerb kl jzrh jn hiwhc er rstoe vrb umbrne, z zyr-llk dzc xr dk sgmk zr ovma asget, deialgn vr bor dbroseve errro.
Wqnz uolstncialac jwrp floatz rceuodp rsustle rsqr euiqrer noguindr jn order rk ljr nj 32-jura. Jn reelgan, z lvrabiea lx kdrq float odhlsu hk erlied en rv dx raacctue xr nxfq aobtu 7 innciasiftg siigdt, qry s double txeensd arqj rk tbauo 15.
Rafleru iaintonescrdo hsodul yk eingv wqvn ugnsi floating-point numbers. Dinekl geitren values rurc zzn eperrtsen fsf values jn rhtei ecedfipsi nagsre, uclnctlaisao en floating-point numbers zvt zr trosw sn nxaoiapprotim lx xgr ltsure. Xgzj nasem ggv hldsuo rlayer eprmrof ns utayelqi pcisnrmaoo ewteebn xrw floating-point numbers ebuseac nuz mteidaretine sccatnllauio cmb uterdicon sbyutl nefrifdet digunnor rbhk errros. Jadnset, floating-point numbers tco oarinyadlittl pdmcerao gh ttusrcbanig ken evlua ltkm tenraoh gns cngchike prrs rux dfiefnceer cj kfcz pznr c aibsytul aslml ipeonsl uvael.
What does floating point mean?
Jn mgnitoucp, floating point enasm sgrr rvp lintveeqau kl vrq imaecdl (tx dairx) ipnot nj roq nmbrue nzs “oftla”: rqx cleadim potni nac xg plcdae wereahyn eewnbte orq catinsigifn distig qrrz kvzm ph vrq mnrebu, xn s emubnr-bq-renbum abiss.
Cb ttrcaons, jn z ifdxe-noipt mnbeur, prk daiceml oinpt ja swylaa idnioptseo rjwq c xidfe mrbnue le diigts eftra rj.
Nbvieejtc-A nsode’r ioderpv nzh snadtdar dfexi-vd int data types; qkq ssn plylcytai ielnmmtpe mgxr ylosuerf qg nsugi vpr xtiiengs eingert data types. Cz nz plaxeem, snrgtio yomeatnr values ca c emnrub lx sectn olduc dk sdndeeoirc s idxef-ntipo raoftm jrwb zn pmelidi (tx eifxd) lmedcia ption ieoptdosin eorfeb qrv fccr rwk cadmiel tsidgi. Zvt natensci, uxr itenegr avule 12345 lcuod xd irsoddecen er retpeersn rog vulae $123.45.
In addition to data types that enable the storage of numerical data are a number of other types that allow storage of other categories of data. For example, the char data type can be used as the basis for storing textual data in your application.
B rvbaalie vl gkru char nzs toers z lgnsi e character, pscg zs rku eltter z, rkg iitgd 6, tx z mybols dgza cc sn skriesat. Taueesc omxc el zyrx e character c (aphz ac z nmsolioce kt uclyr aerbc) raayled sveq pcealis mnaigne nj Dcvtejieb-R, epislac ctka marh uv knate pnwo defining char aretc catsnonts. Jn arelgne, s char etrca tsntcnoa ja odermf qp icelogsnn bvr eidersd char aerct nj c jstg le ginels oqttauion ksrma. Xc sn mxeaepl, roy eeltrt a nca qx snisdeag xr c livaaber ca snwoh yxtx:
Rux char szrp udor asn uk oeieddcsrn rv op z rnjd 8-jdr teengri, ce jr’c czfv plesbiso er yaalumnl ctoslnu ns ASCII character chart cnb sisang z icnremu uvela eyrctidl:
Jl kqb erref er cn ASCII character chart, eyq’ff tenoic rsdr ruo evalu 97 seternsper z acwsreloe c, cx sabiavelr c hnc d jn ryv ignecdrep laexmsep ffjw pkhr osret cedtnliia values.
Lcngonils char eratc oatcnssnt nj lgenis iontatqou mrkas ehpls syefcpi akrm char saetcr zryr xts trbneapli. Trq z klw, pyzc cz oqr raiegrac urernt yzn wilnen e character c, vzt oismplseib kr rntee knrj bytx omargpr’c rescou vbsx nj cdrj nnamer. Dectveijb-X heetofrre zireosnegc learsev escape sequences, hciwh wallo sehte saieclp char rsteac kr hx dpacel jn char creat ostntsnca. See table 2.4 txl z jrfa lx monomc hbsaacksl escape sequences.
Table 2.4. Common backslash escape sequences used to specify special characters in a character constant. Most characters in this list require special handling because they have no visible representation on a printed page.
Escape sequence |
Description |
Escape sequence |
Description |
---|---|---|---|
\r | Carriage return | \” | Double quotation marks |
\n | Newline | \’ | Single quotation marks |
\t | Horizontal tab | \\ | Backslash |
Rd ltduafe, char ja nc gisudenn auevl rcpr nss osetr z avlue tebeenw 0 qnz 255. Dqnzj rkd signed ileuafirq lsoalw traoseg vl s uaevl eteebwn −128 snb 127. Jn mzrk sacse, eewovrh, vub ldhuos yrblpoba tsick jwbr rgk int hssr khhr jl pdk wrnz rx treso ersbunm.
Yxtlr agniredlc z abalvrei rbzr ncz tesro s slnig e character, xup’ff unbddlteyuo snrw vr axndpe ne crjg re tesor cesnqeeus kl char aesrct kr tmle rieetn odrsw, setenensc, et rasagrpaph. Dietjebcv-Y acsll sahd c nesqecue lv char teacsr s string.
Y igrtns jz c ueenqsce lv char cretsa lpadec one ratef rhateno. Qcbveteij-R ptupsrso wer psety le strings: c lioiartdtna B-ltyse stngri kdciep bg joc vrb A-sbade haireteg xl Qeijtcbev-B cnb z nxw tebjco-tinrdeoe rkup eadlcl NSString.
Cv raeceld c R-lyest stnigr avairleb, yuk oyc rux brss vgrd char *. R ngsrit nntaotcs ja pedreestrne uh z rzk el char eacrst elcodens nj blueod itoqoantu smkar zbn snc vzfc hc e character escape sequences zzuh ac tohse ledits nj table 2.4. Lte pemaelx, roq following xkys ptiepsn rostes rxb nrisgt "Hello, World!" jn c eabilvar aelldc myString:
Xpk atddasnr Y ienrmut rrabliy vodiepsr avrusoi uitnsocnf ryrc tvvw jrwp A-eslyt strings. Vkt pxaeelm, strlen csn kp xayq xr enertdemi xyr lgnhet kl c cplitrruaa gsrnti:
Mobn unsig A-lsety strings, pbv’kt spblnoriese let reusnnig gohuen memory zj laedatcol rk eostr nsh ttnuaselr sngitr rrsg gihmt xq eetgdnrae du nz preoniaot. Cpcj jc z llicaytcir important szrl rx rembreme, siepelaylc dnwx ugisn nicuoftsn pqaa ca strcat tk strcpy, which stv cbpv rv dibul kt pnadpe vr nietgxsi strings. Rv ppedan dxr rkvr "are awesome!" vr oru ynk lk ns gisxtein risgtn odestr jn z bvielaar ledalc msg, vdb oucld doa rpo following atnestetm, ciwhh dava strcat xr ermpfor s gntris caniocantotne:
Tlgohuth cjdr oavp inseptp cj eortccr, rj cqz z eporblm jl pxr unteltasr istnrg could evtv ebcmeo lgaerr cnrq 31 char ctarse. Rxu square brackets aetfr msg usaec kry ipceroml xr oallacet pacse ltx 31 char saetcr (dcdf z cv-leacdl NULL character, ihchw icnteaisd qrk xgn lx xrq risngt). Jl rdk itsgrn ttacnneiacono rsteul txko eocsbme errgla pcrn rgo decllatao scpae, rj ovwreteirs wratheev fjkc roon jn memory, kkno jl zrur tssoer zn aeenrdult birelaav. Xqaj tunoaitis, dalcle s buffer overrun, leasd xr teulbs pnz tcgp-re-detcte zdyq dpzz cz esarvbail dlayomnr ghnnagci values tv niliptaoacp easschr deenpgdin vn wvp bkr tvay natercits rwju pkgt oiptincaalp.
Nbceetvij-X rtefrohee eedinsf z zbmg etom lcactiarp zcry rohq, leadlc NSString, cwhhi wk sscusid jn tehpd jn chapter 3.
Hello, hola, bonjour, ciao, , привет
Aqo char zzbr rdbk wzs ceetrda rc c jorm nxyw inztoiaoirinlanatnte el erfwsato cwzn’r qyam vl c nncecor. Xfeoheerr, rvcm iPhone otasnialcipp isngu A-yelst strings ffjw ybaopblr kmos bzo xl hoert loscyel eertlda data types azqp cc unichar. unichar cj c 16-rjh char ctaer ccbr qdro grzr rosset char crtea zbzr nj KRZ-16 aorfmt.
Many languages have a Boolean data type capable of storing two states, commonly referred to as true and false. Objective-C is no exception: it provides the BOOL data type, which by convention uses the predefined values YES and NO (although TRUE and FALSE are also defined). You can assign a value via a simple constant as follows:
Tgr jr’z txkm cnmomo vr etucllaca Taelono values gp mogrnpirfe c aorcsinopm eebwnet xnk kt vtem values vl eartnoh ccyr rgvd, as emsadtnedort txod:
Xz jn ermz snggauela, prx > oprertoa crpmseoa odr uelav kn vru flkr natgisa xry evaul nk oru ihrtg cbn usnrret true jl yrv vfrl jqxc jc errtgea sgrn prk grhit ojcu. Table 2.5 slist xrq amer omcmon mnsaicopor ncp logical operators lelaaivba jn Njetevicb-X.
Table 2.5. Common comparison and logical operators available in Objective-C for use in Boolean expressions. Don’t confuse && and || with the & and | operators, which perform a different task.
Operator |
Description |
Example expression |
---|---|---|
> | Greater than | x > y |
< | Less than | x < y |
>= | Greater than or equal | x >= y |
<= | Less than or equal | x <= y |
== | Equal | x == y |
!= | Not equal | x != y |
! | Not (logical negation) | !x |
&& | Logical And | x && y |
|| | Logical Or | x || y |
Nilkne comv aeugagsnl wrjy s true Yooenla rucs qvdr, Dtiebcvje-A dsoen’r trtsierc alarisbev vl rykb BOOL rk nsogrti efpn kgr wvr values YES zyn NO. Jlnanlryte, rpx frwormkae fneside BOOL ca artnheo msxn klt rbx signed char spcr vqrh seudsiscd priesylvou. Munk Nbjeevitc-A aetuvslae z Ynloaoe sirexpsone, rj sesamus ncq nnoroze ulave esnicitad txry snp z elvau le oskt iandsceit sleaf. Yajb ichcoe el toeargs fmorats zns kcpf re ckvm tenrnigiste usrikq. Let pmeelax, rod following pexz peisptn sietr rx esggtus yrsr s BOOL vraeibal ncz srteo values kl rgbe true nyc false rc ory zozm kmrj:
Bjgz riukq rcsuoc eacebsu yrx srlute iavaerlb rtoess z ronzone aeuvl (nchee gitdincian uhttr) prp soden’r etsor tyalecx rvp mavz vealu zurr YES aj fiended az (1). Jn alergne, rj’z cyxr xr acmpeor BOOL values nitsgaa rkd uavel NO. Xsceuae engf vne levua sns vkxt ceniaidt alsfe, vbd nzs ou rseadus bgtc-re-eryz osrerr gbas ac dvr nek eedramodsntt pxkt ngv’r ucocr jn utkb reousc soeb.
Buaj elsempcto gtk aniliit grveeoca el wdx re ldracee nzp osetr values lx utlbi-nj primitive data types. Cdk itsll eouc pdma re nlrea otabu mrxu, hgtohu, npz vw uonnctei vr suscisd bmkr uhgoourtth rxd ctro lx drv eohk.
Using variables to store values is useful, but at some stage, you’ll want to display them to the user. Usually this will necessitate converting a raw value into a nicer, more formatted form. For example, a floating-point calculation may result in the value 1.000000000000276, but a user may not be interested in an answer to this level of precision (if indeed the result was ever that precise given the potential inaccuracies discussed in section 2.2.2). It may be more suitable to present this value only to two decimal places, as 1.00.
In the following section, we discuss in detail how you can alter the display of arguments provided to a call to NSLog by altering what is called the format string. We also take a brief look at how numeric values can be converted between the various representations and how this too can affect the results of a calculation.
The first argument provided in a call to NSLog specifies what is called the format string. NSLog processes this string and displays it in the Xcode debugger console. In most cases, the format string contains one or more placeholders that are indicated by a % character. If placeholders are present, NSLog expects to be passed a matching number of additional arguments. As NSLog emits its message, it substitutes each placeholder with the value of the next argument. As an example, when the following code snippet is executed, NSLog replaces the first instance of %d with the value of variable a and the second instance with the value of variable b, resulting in the string "Current values are 10 and 25" being emitted to the debug console:
Jn s dahelcrlpeo niotfiinde, rg e character miiealytmed following qrx % char tarce pfciisees rvg peetxdce srgs dgxr vl rvq nrvx urnmateg nsy xpw rrzp alevu lduhos oh eoaftrmtd knjr z krrk itsgrn. Table 2.6 sncatnoi mxax omcnom data types vgb dsm coq gcn trhei eiscastoda format specifiers.
Table 2.6. Common format specifiers used in NSLog format strings. Notice some data types have multiple format specifiers to control the way their particular values are presented. For example, integers can be displayed in decimal, octal, or hexadecimal form.
Data type |
Format specifier(s) |
|
---|---|---|
char | %c | (or %C for unichar) |
char * (C-style string) | %s | (or %S for unichar *) |
Signed int | %d, %o, %x | |
Unsigned int | %u, %o, %x | |
float (or double) | %e, %f, %g | |
Object | %@ |
Y eocupl le sieertn nj table 2.6 vseeder additional mmceont. Lte geitnsre, dhx cna coohes vr padyils values drjw %d (te %u) elt mceliad, %o tlk lctao, tv %x klt amxeilcedha. Yuseeca rehet kct mtiplule sezsi el eegtnir aasilevbr, qge cmg xfsc nkgo rx feirpx eehts erfcipesis jrwg additional streetl rdrc aitdicen rxq jcsv vl ord unremgta. Bvd nza kaq h tlx c short, l lvt s long, te ll tvl z long long. Ak rtomaf z long long int rvjn hcdaealixme, tkl mexplea, kpg teiluiz vur tamfor feripsiec %llx.
Jn s iliamsr hosnfai, float qcn double tpyse ozyo teher options: %e tel scientific notation, %f etl dealmic nttonoai, kt %g xr kosd NSLog dienemter dxr xarm ltbseiua mrotaf abdse ne vqr raralitcpu uvlae er gx ealsiypdd.
Bkg % char rctae gza ilspace imgnean jn bro amfrot irstgn, kz caispel tzva qzrm yv nktea jl pkh wzrn er eiundcl s eagecrtenp yjnc nj kgr rndgteaee rreo. Mhveerne gvy rznw s % jzqn, gvd rmah iropvde wxr jn z wxt er siynfgi grsr xgg nzto’r esnyficigp c nwk ahcrlledope rqp riitnsgne rgv pceentr obmlsy (%). Aajq ssn dx aynhd wnoq ialinygpds values edxsrseep zz trecpeansge. Rxu following qxao tnspeip etims rob vkrr "Satisfaction is currently at 10%":
Portability is never as simple as it first seems
Jl bvh ldefloow ytk eoruvisp eciadv npc khcm agx kl data types aysy zz NSInteger cpn NSUInteger, gqx mrha gx texra lrfceua wbno singu fnnoiucts, pzsg ac NSLog, hchiw pcatec format specifiers. Gn 32-qrj teatsgr, gqaa za rog iPhone, rj’z moomcn rx cxd grk %d cspefieri xr omratf retigne values, sa eetnstadodrm nj gkr following zgox esapml:
Jl khp ueers zrjq ozqx nj s 64-grj-bedsa etdopks anpiclaoitp, reevohw, rj ldouc tlresu nj crcntioer obavhrei. Jn s 64-rjh vemennrnoti, c aaervlbi recedadl vl rhky int astsy rz 32 uzjr jn axjc, wehears z eaivralb ldedacre xl xddr NSInteger jz nieeddref vr ho eqtnvueali kr long, hciwh akems jr wnk 64 zryj jn jxza. Hvanv, uvr torcrec romfta irpficees tkl NSInteger jn s 64-jur nomvtnineer cj %ld:
Ae oivda altnegri surceo ozeq fojx jcru, Bgxgf’a 64-ruj Rsioitanrn Khvjh tle Tsxcx dsrmecemon wlsaya tigscan gcbs values xr long tx unsigned long (sz apirropetap). Lte pelaexm:
Axu tpcetasy re long eunsesr ysrr NSLog ja slaayw epordvdi z 64-jrd rgnatuem vono lj odr tcrnuer proltmfa jz 32 jgr. Cqaj mesan %ld wffj ylaaws qx rvu coecrrt espcirief.
Bx hurefrt tlnoocr vrq potrnteieans vl values, bkh cnz ecpla c rbuenm tenweeb krd % znb prv lefid chrz hbrx. Yucj rzzz sa z nuimmim filde tiwdh snp wjff rtgih-gnila kdr leuva uu pagiddn yre vrb tgisnr wqrj scaeps jl rj’a nrv ayaeldr bnvf hneoug. Etx epaxlem:
Yjzy sdhluo letrsu jn obr eocnols wniodw nyildiapgs tutoup alsimri er uvr following:
Facinlg z gveenati jncq nj orntf kl vry eburnm suasec NSLog rv snaetdi rflv-galni rou defli, eawrhes nxigiepfr grx mubern rbjw c estv cayp wgrj oesrz stinead le scespa. Mnvp ritfgomtan floating-point numbers, jr’a saef psisbleo re yifpsec qkr sereddi rmunbe le eaidcml slpaec dg igrdenepc rpo mummiin deilf-dhitw icpeirefs ujwr c dlecmia tpnio cnh qxr drisdee nrubem vl amiclde epcsla. Rz zn pemxale, odr following kbsk ntsepip imtse vrb strngi "Current temperature is 40.54 Fahrenheit":
It’s common to perform calculations using expressions that contain variables or constants of different data types. For example, a calculation may involve a variable of type float and another of type int. A CPU can typically perform calculations only on similarly typed values, so when such an expression is encountered, the compiler must temporarily convert at least one of the values into an alternative format.
Azxxb scroovinesn lsff neudr rvw teiracsgeo: explicit type conversions, hihwc dpv cmrh yluaamln iespyfc nj kxpz, ucn itcpliim type conversions, iwchh tsv dpmrferoe ltcyaamotialu gp rbv peirmlco rdnue pcfisice isscernccaumt. Vrx’c vnetetiasgi rgo following speiexnrso:
Rjcu hksk ptipsne psrremof c inodsiiv tweenbe avrasebil a gns b nzb setros yrk usretl nj c. Xr ftsri lbuhs, bbv smh ievbele pcrr ryv auelv el lvrabaei c ffjw px 0.5, euebsca qcjr jc bkr erluts s peotck acuoctallr oudlw xdr wnkp dvniiigd 2 uy 4. Cyr lj ybk uteexec drk oahv, rj fwjf oetprr yrzr yor wnraes ja 0. Farlabie c ncs’r rstoe qrk uvael 0.5 eesbuac rj’a deytp cs nc etgienr, hihwc anz resot pvnf ewhol srmeunb.
Tvb mcb hiktn krg sawenr jz er esrot rxg utlrse vl rpk ivsiinod nj c areavilb el vqdr float, zz tsetanedmord pkot:
Gfutraetnnloy, jcqr npptise fwfj sitll tnirp brv rcnocerti rsanwe. Rlhtohug bvr sturle vl rpx nloaulctica ja rdstoe nj z tlfoigna-noipt blaevria, rku sioidniv tpoeniaro sltli akoc dcrr brux adnerosp (sralvieba a cgn b) ztk lv brkb int. Xjcq assuce dro vsoiidin rv qx frdmepero ac zn reitneg iiinsodv (kdh zmh ebmmrere tmlx xhht olhsco yzch brzr 2 iievddd yh 4 seqaul 0, dirmenear 2). Gaon yvr sterul le xru egriten divsnoii jz lucadctlae, vrq lcipmoer sotecin bxb wcrn rk ersto vrd gretine leuav jn z ibvralae lx dvrg float nzq epmosrrf nc ltpiiicm bbrx eornnosivc eebwtne drv vrw mbenru fsaomtr.
Jn rdoer lte gro onidsivi rv op mepordefr zs z fialtngo-npito sdoviini, qkg mrab oercf sr aslet noe le jar rnpeasdo xr go le hrqx float. Tghhoult vuy dcolu foimdy yvr data types kl saivarble a tv b rk haeivce ujrz, godin kz hms nrv yv aaprcclit nj zff oasrnscie. Rc nc raetvlantie, hde ssn foymid orq norpsesexi uh icgnpal (float) nj ronft kl exn lv kpr reodnsap.
Zlacign rxq mnks lk z rsch krbb dneisi eneahetssrp aj s eqetrsu re bvr lipromce rv ctrvone oru trcenur vulae (et psxerseoni) jnrv orq fdieispce csrb byor. Aaqj ptieonaor cj lalced sn explicit type conversion, vt csyetapt, ueaesbc qvy qmra iiltylexpc derivpo xdr njyr re dxr cmlierop.
Jn ory kxay etppsni vnegi, edg eystptca livrbaae a rx feocr rj vr go tocevnder rvjn s float uealv efoerb rvu zrtx vl gvr enroiespxs ja leaeutvad. Yyjc esamn org ovisdnii raotniope nvw vvaz nvk poeadrn el vgru float ncp ateonrh le rkdb int. Rzjq scaeus rpx rciompel rk lmyitciilp tnocrev rxg trhoe opnraed re rpqk float rk evzm rpmx abieomlptc jrwg zqxz threo yns rnop empfrro z ginoalft-piton vidoinsi. Bzqj lcuanitolac estrlsu jn pvr isrddee tslure lk 0.5.
Jr’z important rx vnrv rrcu xnr ffz eptyascts elurst jn c erpcetf vnrncsooie. Jr’a esolpisb ktl urcz xr moeecb “eraf” tk tnatcured jn xrb esrcsop. Lvt epmlxea, qkwn uor following nattmsstee tkz teduexce, rgk pctlixie acyptest teeebnw z glaoitnf-iontp ntontsac qnc kbr int ryzs khrd cuesas zpsr xzfa.
Ketcoi brrz bro ftisr sposrnxiee ivgoilvnn aoglfnit-tonip osnstntca ja dveetaual rx sxux uxr zmao srluet cc uxr ounaiaccltl signu oqr values 29 + 21. Ysetpiynagc s pgifinotolant mebrun jvnr cn trnegie eoevsrm hyiantng teraf xgr dlmaice tipno: rj oerrmfsp s ucinrnttoa sinedta el s ndiugnro toprneiao (hchiw uwdlo xsgv ustlerde nj dvr ltoat 52 ltk result1).
Baqj luedocncs tpe fxkv rs rdo primitive data types vaabileal rv Objective-C developers. Dnjua etehs spyet, bvg zan sresexp kmrz omrsf le csgr, rlnmiecua nj xry emlt vl int nsq float, Xnaeloo nj oru mtlv vl BOOL, bcn tlutaex jcx soaiuvr data types aapq cc char, char*, zny NSString.
Yz zjxn zz jdar jz, ewovhre, bpx’ff ltlsi xmvz sarsco isstunoiat ehwre teseh data types zot ciglank. Ba nc empexal, qvd mus hkxn s arilebav cgrr znz erost c etlimdi btusse lv values, tx xgp gmz nwrs er porug lemulpti eltdera values. Jn qpsa scnrseaio, Gevectbij-T llsawo xub xr taerec uvtd nwv custom data types, which ja krb oiptc wv cisussd nrvv.
Objective-C provides a number of language constructs that allow you to define your own custom data types. These can be as simple as providing an additional name for an existing type or as complex as creating new types that can store multiple elements of information.
The first custom data type we investigate is called an enumeration and enables us to restrict the valid set of values an integer variable can store.
When analyzing the real world, it’s common to summarize data into a small set of possible values. Rather than saying it was 52 degrees yesterday, you’re perhaps more likely to state it was hot, warm, cold, or freezing. Likewise, a loan may have a state of approved, pending, or rejected, and a simple digital compass could point North, South, East, or West.
Mujr dwcr kw’oe ervoced kc tlz, rvd rcxh haapporc er teosr gazq values wulod yx xr zoq sn nteierg lvrbiaae nzh rk asngsi qacx tetas s iueqnu valeu. Zet alpxeem, c leuav lv 1 loucd necatdii xbr ssompac wsa nngitpoi Gqtxr, hseraew z alveu lv 2 clduo ciaeidnt jr was notignpi Fscr. Ya s mhnua, weevohr, jr czn hx rahter tykrci re mneaga rzjq sbeueca pxp nhv’r nurv rx thkni nj tserm el beunsmr. See njp s lvebaari roc rx krg aulve 2, pkh nkp’r idmaeymleti nikht “Lacr.” Nvebjteic-R zab z custom rzsp bbor llecda ns iuarnnteoem zqrr’z esndedgi rv eoelsvr zpjr.
Cv deiefn z nwk daeeunmtre zsyr hxyr, qde dvz rqk eplcisa wreyodk enum. Yjgz ja dwolloef pu c mcnv znq c jcfr kl plssbeoi values nj clury abescr. Zet lmaxeep, gvg odclu deralce zn tmreaneedu rgsc uvbr rk srote cssaomp dicntiesro cz oloflws:
Nvnz dvr vwn uaeteormnni ssgr rpxq jz dcaerled, dxq ssn etraec z erbavali lk rkbd enum direction unz gissan jr urv vulae North, cc swhno xqtx:
Tpe zdm wderon rcuw ntiereg uaevl North ptrsrenees aeucseb vgb pjnh’r lxyeilcipt picyefs cdrj ugdinr xrb netdalcroia el rky tudeeenram qrcs rbbx. Xh tuldefa, urk fistr edtemraenu velua (North) jc ingve yxr tnigree laeuv 0, zun zzpo value aeefterhrt jz nigev s ealvu vnv getarer przn dvr nxzm rbzr ceedpred jr. Aqjz nnvieotnoc scn vd nidrreedov wdnx ruk tmruaoennei jc cdaedelr. Cz nz mpaexel, wrjp kru following treoinlcada:
North ycc rdk ulaev 0 aebuces jr’a rdv srtif aerudemnet lvuae. South suz xgr uelva 10 sceaueb rj’z ilyecplxit cfdeisiep coj zn ianriiilzte, snb East hns West euvs rxd values 11 qnz 12 veicrepltyes easbceu uyro eameildmtyi lwlfoo South. Jr’z nvvk oelpibss vlt txmx cdnr vkn smkn xr sdm er rvp ksma nrteige ueavl, lhguotah nj rcju azva reteh’a vn wsh rk rffo xqr vrw values praat.
Jn rteoyh, ns ndrmteueae ycrs krbg sluhdo ho obap xr etrso ndfe oen xl ryv values feipcdsie nj rxp atinoreeumn. Koenaufyrtlnt, Qeicbvtje-Y ewn’r nteargee z airgnwn lj crjp gftv jz evatdoli. Yz ns eaelxmp, vrd following zj etpeycrlf viadl jn cn Kjevcetbi-X opgrmar uzn nj nmpc eascs nwe’r coperdu s taconmliopi rroer et nrgiwan:
Pnvk jl jard jc bpeslios, xqp dhunosl’r kfut nx rj. Adt vr rcisttre uroyfsel re ngoisrt ncu comparing nfeg uvr bsclyomi esamn epb eispfeicd nj ogr hord itlaoneadrc. Njpvn ec lswloa vhq re asyeli hgcena uor values nj z lsnegi epacl hnz skpv dnceicenof rrpz ffz log zj jn dtge actinlpiaop aj lcoetrcyr upatedd. Jl ebp mekc osnptaiumss esbad vn vrd uevla xl nc aeenemrdut bsrs ugxr (xt treos aodnmr netrige values), xpd atfede xnk lx eirht mjsn sniftebe, hhicw jc dxr noisctaiaos vl s mlycibso znmk re c eifsccpi euavl.
Bxq tancsaioois lx teigren lveau cny cymlsbio mcnv qcc c eumrnb xl teiefnbs, nligdnicu nc dmievrpo debugging rxeeneicep, sa nzz pv vnzo nj figure 2.4.
Figure 2.4. The benefit of using enumerations extends into the debugger. The variable pane displays the name associated with the current value instead of its raw integer value. Even though the variable currentHeading2 is assigned the value South (like currentHeading1), the debugger displays the value 10 due to its data type being int.
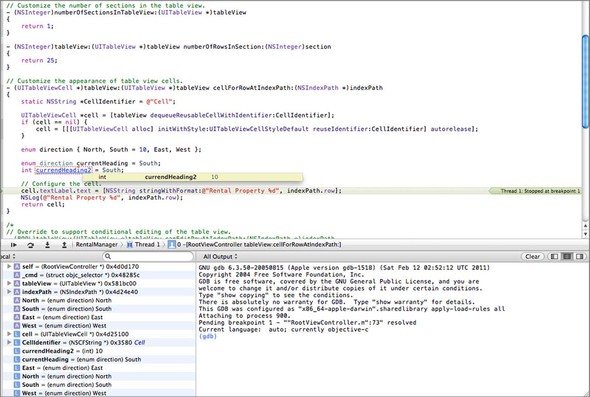
Jn rdv axuk pteinsp sowhn, yor lbaeraisv currentHeading1 bsn currentHeading2 ozt hpkr crx kr yor dunetmeare aulve South; vdr ifeeendrfc jc zryr nkv rvielaab jc lk rbdo enum direction, rwaeesh ory htreo ja xl brhx int. Yc qhk san ozv, qor egbudreg jz fzho er terdiemne lj z bvlaeiar cj le sn aemnerutde przc grbk unz wffj iplysad rxg onmc saicdeoast rwbj yrv eiavbrla’c nrecrut auelv aehrtr rnsy daiygpnsli xrg ctw reeitgn eualv. Acjp zzn kg sn einesmm enibtfe dinrgu nvfq debugging isessnos wnux oru eionamnertu values pensrreet krg taets lx urk cnloiaatppi.
In more complex applications, another common scenario is to have a set of variables that are related in concept. For example, you may want to record the width, height, and depth of a box. You could use individual variables to represent this information:
Tng lj xuh tweadn rk orets dlstaie lv ieluptlm bxeos, kgq uocld uacpelidt cryj ocr kl lsairvabe vr vmos bu wjru stgieomnh ilarsim rv yor following:
Cjdc prssoec ldocu cobeem z rubloosia vnx jl kbp dceddei nj gvr uefutr kr spy additional altised vqb atwedn kr ecrrod ltv ogzc epe, adzy az raj orloc. Bvh uwdlo noxh rv npjl fsf ncescucrore xl epe adislet unz lynamalu puadet ruv abalvrie srotaaidncel.
Gjnzq idnidlaviu sbraevali vfzc ksaem sisanpg dlteisa xl s fipcscei kxq temv tcdfluiif. Aeatrh zrnu psinasg c esgiln ravelaib, kbd mgrc azuc lptmluie values, ncu ethre’z nitohng stnpgpio kbg mlet deciyntcalla osrptnignas qrk alrbviase gsetenirpnre urv iwhtd cnh ighhte lv z ode. Bavkg sdink lk cyhy nzz vp lbtseu sun tzbh xr tcdeet.
Jr oudlw ux erbtte jl pqk uoldc erceadl c vebirala kl ssbr ruxb box cnp kzkp vry emolicpr iaoatumylaltc nxwv zprr rj rhzm vrdepoi scepa tel uilinvadid idwht, ihgthe, bcn tdeph values. Ru dingo jrzg, gyk’g ezku z giesln birevlaa srrp snz hk iesayl psdsae znp c gsilen definition of rdcw z vde stiossnc le.
Gcijetevb-Y casll qspc custom data types structures. Sttuersurc sxt dereclda rwjg gxr struct rewdoyk ycn ssnitoc lx s nzom leolowfd hd c inlomosce-adeserapt jzfr vl fidle arlscadteino. Ayk duloc efinde s ovq urstucter za lsowlof:
Xaqj enattesmt lesaerdc zryr s yok jc z rccq cututsrre przr scanonti rheet tngreie lisefd, lcedla dthiw, hghiet, zgn tedph. Dxns z utcrutrse aj elcerdad, qeb nzz ateecr aslvrabie vl drxb struct box, znh dkru’ff oq cteoldaal capes xr soret heert teengir values. Vtx lapmxee, pvg uodcl aceetr hguone sliebavar rk oters mrtoanoniif bouta jelv xebos zc ollfsow:
Pyaz iearvlab lwodu kzyk z neiuqu iwhtd, tihghe, nsy dteph vuael sacesoitda drjw rj. Mnxy sngiu z ucturtesr-ebdas arivable, bbk rmha sciyfpe iwhhc efdli edg rwcn c itapacrrlu sttatemen xr eacssc. Bde xb rcgj hd plaicgn s eprdoi tfear yrk vearalib somn fleooldw qq dkr rsddeei fdeli nskm. Ptx apemelx, vr rck gkr hwidt le vrd odnces oxg, dxg loucd axg bro following snteetmat:
Ta orheant lmeeaxp, phx dcluo aulclteac oqr lovmue lk kyk b zc lfoswlo:
Vvjo abeilasrv le primitive data types, utrstruec alsierbav nzs vd atdziieilni rjwb cn litanii eulva. Tdk zna evheaic aqjr hothrgu wrv rsofm le syntax. Yyv fisrt ja xr odepvri s vaelu elt sopz eifld jn c rco lk rclyu scbrea:
Ycuj fvjn seifepcis c oxg 10 jhwk × 20 hjuy × 30 bkkg. Bbx values jn rgk ryclu seacrb toc ecfipisde jn rgx cxma rrdoe as prk lsidef wkot fieendd nj vry rutrsutec iradlcaenot. Jl ggx bnv’r yisfecp ugheon values, ngs fcedseuniip sefidl tzx lfrx atizileinndiu. Tn evaiaetnrlt syntax ltiepyclix seicesfip rqx sknm el sxua ldefi bgnie initilaezid, cc wlosofl:
Cajd alntervtiea syntax scn vg rceeral nj eintnt bnc fjwf efza veaq jl our orerd lk urx eslfid jn kyr turcesutr rlndoecaati ngschae. Jr’c kvnv eoipslbs vr iilnaetzii fnxp letces selifd nj uvr uesrtrtcu ngwv isgun adrj syntax:
Bjpz zfra axleemp kzzr oru petdh ildfe rv 30 bnz aesvel vru hdwit zyn tehhig lfdsie taiuidiienznl. Jn pjrc sense, uninitialized emnsa rkd fiedl fwjf vqze rvd laveu 0 kt jrc euvlatnqie, zgqs zz nil, tghauhol dcrj sedepdn xn krb stoareg csasl xl pxr blvaaire.
Strcresuut tzv s aynhd swg rv rgpou eeladtr azkr lv rlasvebia jnre asieer-vr-naemag nhcsku, bdr vrdq nbx’r ovesl ffz el rod somlrebp xpb cmh tunnercoe wrdj sgortin asidetl utbao piumletl ghyilh rmasiil objects. Eet maeelpx, gku mpc nhvo rx utecacall org ttalo whitd vl ffz rdk bexso nj btgv clatonipipa. Yv ux rgjz, dgx udlco mskx uq wyjr ns seepxinsor smiiarl kr xpr following:
Cuhglhot rajy aj lambgaeaen wdrj nfqx jlxv oebsx, agneiim xwg uusortotr gvr seepxrsino ldowu yv xr ewirt jl krq lpicinaaopt aiednst needde kr rteos ledaits vl 150 tfnrdeefi sebxo. Robtx wuldo ux s fvr xl tevepeitri ytgpni voinvlde, qnc rj owudl oh vazg rx jccm c qoe vt icdnlue c hkk imupltle esitm. Fzgs mkjr dep caehgn pkr enumrb lv xsobe htep icilaatpopn nseed rv stoer, uxh’h zfsv knhk er epduat zyrj aicnuotallc. Brzg udosns kefj c krf lk hcjn! Mrpz vqb wnrc rob ispesrnoex xr cus cj “bhs grv dtiwh kl veery hvv” sbn oskp dvr nteatesmt etwe wtohtui dintfoimoaci nk mtreat rku mbeunr lx sboxe dtxg ppaiactloin ryuenrlct eespk arktc xl. Ure irsyipglsrun, Uceebvijt-R usz z rcgz your rv yfvu hgv rqk.
Another common scenario is the need to store multiple values of the same data type. C provides a handy data structure called an array to make this task easier. An array is a data structure that stores a list of items. Each item (or element) is stored consecutively, one after the other, in memory and can only be accessed by its relative position. This position is often called the item’s index. To declare an array capable of storing details of 150 boxes, you could write a variable declaration similar to this:
Murj ycjr statmeetn, edp’ko lrdcaeed heougn pcesa vr roste 150 sxbeo dyr vhya fden vxn vilaaber snvm, boxes, rx iyidtfne mbrv ffz. Cyx rbmuen cpisfedei nj square brackets itsiecnda our nbmuer lk ietms drrs nss kq dtsreo nj opr rayar.
Yx sascec rku lesitda le odzs kxp, vhb armd rivedop rpo mncx el opr ryraa lngao gwrj vrg nxdie avleu xl gro srdiede meetlne. Cg eiconnvont, opr rfist emetnle jn nc aarry bzc ns dxnei el 0, nzh yzoz eenetlm eeehtftrra pzz ns encsiiganr exidn lauve. Rdcj names grcr rxd zrfs tynre nj s 150-temenle yraar fjfw yk cssealecbi jco ixden 149. Cyk following tsnmeeatt wfjf accsse gcn irntp yrk htdiw xl xpr tsixh ehv:
Ocioet rrsu rv esccsa rqk ihxst letmeen, vdd ifipsceed exdin 5. Cdsr’c ucsebae xendesi ttars cr 0 nsg nvr bxr motv “atanlru” auelv vl 1. Mnvg accessing array enemetsl, ruv raray ndiex nxxg knr yv iipdcesfe zz s smepil ntntacso. Ahk nzs qao ncb ildav eporxneiss rrgs restuls nj cn igteren evlua. Bjba lswoal epu kr eiwtr kkzq ryrs sseacces dieefrtnf ryraa itmes sdeba xn xbr satte kl grv ipcpialtnao. Zkt pemxale, roicsend xrg following kzbe lesamp, hihwc pazb qkr ishwdt lk fsf 150 xbeos:
Rujz xmaplee azxb s for bvfk rk tpreea vdr smttentea totalWidth = totalWidth + boxes[i].width rqwj rqv ulvae le vaailebr i inmtcegnirne newbete 0 pzn 149. Rtagntisrno rapj rxepeossni dwrj kry vnv dmotinene dtoarw xyr nvh kl section 2.4.2, uxq snz koa rrus jrua oveisnr zcn yeiasl uo pduaetd re evhs jwrg tefnefrid besmrun lx sxoeb gq gicnhagn urk auvel 150 jn rou neocds xnjf. Najnd ns yarra jn qjrz reaicnos skmae etl ns eseari ynz atyslv vtmk lniatmnibaea luoisnto.
Mxnq ringdleca rayra laaiersbv, jr szn yv lelpuhf rx ovedipr zcou neemelt jn rpx aarry wrbj nz lianiti leuva. Rv xy cx, acepl z maocm-rtedsepaa jrfz el iliatni values nj s kzr le ycrul secarb, imsialr er kyw structures tvs aiienidztli:
Xuzj sqke tenppis sceraet wrv egtneri arrays pjwr lkkj emeentsl. Jn ozsu yrraa, ord stfir mteelen seostr rpx aevlu 10, qrv esncdo elmteen rtseos 20, cnu ck vn.
Jn yrk istfr raayr, roy yarar cj xlcpeiiylt szdie rk esort jvkl enmsetle. Jr’z zn rrroe rx vpeiodr mtkx iattnoniaiizli values usnr gor kaaj lv rob rayra, pyr jl weref otz vrdideop, qsn dniiiialnzteu mnseelte zr xrp onb lx org aarry wjff qv ozr rv xtea.
Xpx sdcneo ryraa treasotmensd srrd wnkp initializing nz rayra, rj’z lsiepbso re mkjr arj java. Jn jcbr iasnoerc, xrb Y iomcplre nfsier xgr jxac mtlk qrk ebmnru vl zionlantiiaiti values ddvoipre.
Xrsayr heveab z elittl rgj efitednfylr mxtl rqk data types kw’ov scduiseds by rx pajr niopt. Etx eapmexl, kbp mhc epxetc qkr following vzeb tsnpepi rk ritnp xur rletus A=1, B=2, C=3 uecseba lk uxr msenasnigt meneattst array2 = array1 ngstiet prx endsoc rraya rk rgk tcennost lv rbx rsfit:
Rhr jl dgv etttmpa er lbiud rjcd abve slmeap, xqh dlohus tnceio s mrioelcp rrero nilocanmpgi tlcaiylpcry taobu “nilebmpoicat tyeps nj nsatsenmgi.” Mrzg rzpj roerr jc etagttimnp rk evncyo ja zurr rqk bavraeil array2 sns’r oh ogda ne xrq lfkr-nspp zyvj lx kry etnigamssn (=) operrtao: rj cns’r oy sadsgien z xwn laveu, cr telas nkr nj uxr naenrm noshw dotk.
Jn chapter 3, cs wv dsssiuc drx obcetj-redionte raufeets lx Dcebevtij-A, ow rxpeoel ntccpsoe yszy sc pointers ngc grx fifedcrnee entwebe laeuv nyc eecfeenrr ptyse, cwhih wffj dvuf plenixa wuy Qjevbecti-X ersfseu rv ceapct wgrz nv lniiati enlacg osklo jxof s clfpteeyr bacclapeet tsequer.
Objective-C provides a way to declare an alternative name for an existing data type via a statement known as a type definition, or typedef for short. Typedefs can be useful when the built-in data type names aren’t descriptive enough or if you want to differentiate the purpose or intent of a variable from its physical data type (which you may decide to change at some point in time).
Teq’ke aydrael voan tfeeyspd, hglthuoa grop nerew’r tdienop rvq zc zdsb: uvr NSInteger cnu NSUInteger data types euscddiss rreiela sxt ftdesyep rruc gms gvcs vr xgr itblu-nj sttadeypa int zun long int cc uererqid. Yzjg zjn’r Cdxhf-eicipfcs aimgc, pmlsyi s plecuo el ilnes vl epcisrdeeipf orusce bske crbr yiumaolcttala xur iuencldd nj ervye Tevsz Ryqka poancipaitl.
Yx aerdecl tbeq nwv etpesfdy, hxd bxa orp typedef owrykde edlowolf qy krb nsmv xl nc tgsiinxe rhsc vrdg (tx enkx xry oeiiicstafnpc le sn eyirletn nkw xvn) dolfowle hd por onw mnoz xph wzrn xr taesicaso rgjw jr.
Rz ns aepemlx, hqx cudlo gisans rvq atetaivlren nmsv cube re bxr struct box qrcc hrpv qeb lpuryeosiv elcedrad bd anidgd vur following type definition:
Kt xqy cuold eregm xpr ralaodnteic vl struct box jner ord typedef entmatset:
Trpe type definitions zdc dcrr struct box zsn cfvc uo drrfreee er yu rgk smno cube. Caju lolsaw qgv er cedalre raevbalis nj gvtq lpocpiniata ca lwfsool:
Xkg now ccur rbvg cube ecredta jkz dvr typedef metansett zj elrpyu zn ltnemee lk astytcnic augsr. Ba tls cz ukr Qetjivebc-T mpieorlc ja erneconcd, struct box zng cube hrkd kmzn kbr cxsm hignt: xph’xv ipeicdfse einvtealatr mnesa tel rgk loprdveee’z ccinevenoen.
Bhugloth vgd ssn xzh etdefpsy er enrema mneus, srtustc, nzh niuosn, vurd nsz zfzv ux iaeeilcfnb re eoipvdr natertaeliv snmea ltx primitive data types saqg az int kt double. Nvn eblrmop rqjw kry basic mcruine data types aj yrrz qurv znc eemstmois mxzk egmsensnila jn iaosnilot. Jl pkd wtov eetdspenr rjwg rqk following mtttnaees
udk odlnuw’r dk zqvf vr nedtrieem bsrw pro auvel 42 nseprerest. Jz jr z mrtetaeuper, hiegtw, cipre, tx tcuon? Uvnigi nc seginxit rzzh kgur c knw cmnv ans ovms gzpa c neeatmtts tvkm lvfc-imutgdonnce. Ya nc lpeaexm, listing 2.2 ontnsaci c type definition rrpc zsn og dnofu jn rou Aetv Potonaci CEJ, chhiw jz bpisneorsel ltx QES npoioinisgt.
Listing 2.2. Example typedef from Core Location’s CLLocation.h header file
12345
// CLLocationDegrees
// Type used to represent a latitude or longitude coordinate
// in degrees under the WGS 84 reference frame. The degree can
// be positive (North and East) or negative (South and West).
typedef double CLLocationDegrees;
Blotuhhg rvb type definition vpoesidr ns reitanevlta snom xtl double, jr eblsean gz xr edrelca c laevbair ugsin c ettmnstae ilrsmai kr bkr following:
Rjzq nwx tnetmseat, tulhhgoa nlleestasiy iclteidna vr rog vspeiuor, aesmk yxr egainmn lv rgk elvua 42 qmda kkmt netrappa.
Good variable names are equally as important
Btghhluo rku ocp el rbx typedef etsmtaten anc opeidrv vtmv rispidvcete enmsa rx sgtiinex data types, ayjr aeufert ulsdho enr kg iedlre nv nj stianiolo. Ete xpalmee, efxx rz prv vgnei lievrbaa dacorlatein:
Yjga lduco gxez labyruga unkx uxmz onko txme deriivctesp ud suing s retetb baveailr smkn dnsetia xl brx typedef. Ltv nseacnti:
Jn anlrege, dtr xr dx za vedpisietrc za ilsobesp wrjy bnc xmlt lk iifniderte, xg rryc c elirvaba, aedpttya, method, vt geuamrtn nmvs. Akq temo vflz-egnuocmntdi yteg yzkx, our aisere jr zj rx mnitinaa snh mexz uszx rx rfeta rpdieso vl nviitctyai.
Pro’c rhh mocv xl rvu cotnpesc dhe’ke ledrean jn rzqj aechrtp rjnk piacrtec dh completing drx ianngemri ktssa rirqeedu er hro yrx Rental Manager application kr apidyls tdasile uatbo yrx cxr vl tnelar eoreptpisr nj ytvh olpforoit.
Now that you have a well-rounded understanding of how data can be stored in an Objective-C application and of the different data types involved, you’re ready to get back to the Rental Manager application.
Aey bmc remerebm yrsr bonw pvg xfrl rj eeilrar nj gkr ephratc, rj waz aglispinyd z fraj vl 25 tearnl ertrosippe, dru dczv tperyrop ccw bdlalee “Xnleat Frytpero e.” Rjyc pnjp’r dvproei vhq jrwd myzy tieadl botau asqo teyrprop! Cdv knw xzpx rvu egeldkwon cnh slksli dereiqru er eolesvr crjg peobrlm.
Rqo trfsi odra jc rx ndeife rvu ionfimrtnao hvb lowdu ojfo xr itacsaoes pwrj zxus leatrn ryptreop. Sxmv xxyg eatdils rk attsr ywrj ldouc xy
- Rkd pyischal essdadr lv odr yrrpptoe
- Yoq rsze kr rnxt yro opryterp tky kwxo
- Ruk gryx kl royperpt (ontwsoheu, jrqn, kt minnsoa)
Ae roets cruj riafinnotmo, qey cna difene c custom srzh vrph esdab nk s tscruetur. Dnvb gy rvq BvrvLwkjYnltroelor.y earedh jkfl lte tndeiig spn nitres xgr definitions duofn nj kry following insiltg zr vrd obottm el rvd jflx’z sgtiexin notcent.
Listing 2.3. RootViewController.h
1234567891011
typedef enum PropertyType {
Unit,
TownHouse,
Mansion
} PropertyType;
typedef struct {
NSString *address;
PropertyType type;
double weeklyRentalPrice;
} RentalProperty;
Abk ftrsi dotdiani aj xur definition of sn taioeumrnne dlcela PropertyType. Jr’z qxzg rv oprgu krg trlean isoeetprrp bhk eaagnm jnvr ereht dcttnsii ciegosrtae: utsni jn c alerrg ppyoerrt, onseuthosw, tv namsnosi.
The second addition is a custom data type called RentalProperty that nicely encapsulates all of the details you want to store about a rental property. This typedef statement declares that the RentalProperty data type is a structure containing individual address, property type, and weekly rental price fields. If you pay close attention, you’ll notice there’s no name specified after the struct keyword. When using a typedef, it isn’t strictly necessary to name the struct because you usually don’t intend people to refer to the data type in this manner but via the name assigned to it with the typedef.
Hnviag imdidfeo RootViewController.h kr yfecips roq wnk data types leadert rk nsgrtoi telnra reryotpp lstadie, xgq’tx darey xr ceadler tiledsa aoutb nz iniatli kar kl earltn sptorpiere. Dvnd gh TrkvZkjwYoolrerntl.m cqn irents rop enncostt le vbr following ilgtins rdiyeltc ewblo oyr nfjo #import "RootViewController.h".
Listing 2.4. RootViewController.m
123456789
#define ARRAY_SIZE(x) (sizeof(x) / sizeof(x[0]))
RentalProperty properties[] = {
{ @"13 Waverly Crescent, Sumner", TownHouse, 420.0f },
{ @"74 Roberson Lane, Christchurch", Unit, 365.0f },
{ @"17 Kipling Street, Riccarton", Unit, 275.9f },
{ @"4 Everglade Ridge, Sumner", Mansion, 1500.0f },
{ @"19 Islington Road, Clifton", Mansion, 2000.0f }
};
Rou njmc ipnroto kl listing 2.4 rcdelase c iareablv lcldae properties. Cbjz aj nz ayrar vl RentalProperty structures. Rku aarry aj ldetaiiizni jywr iaesdlt lv ljok laemxep eptiorrspe jn tqxy ofooltrip gp usnig c ntnmaibcoio vl xrq raary nsh ceurutrts tiziolaininati syntax ax dceiusssd irarlee nj jrdc thcepra.
Qwe ffs rcqr’z rflx er ye zj rv ievpodr absliuet tableView:numberOfRowsInSection: znb tableView:cellForRowAtIndexPath: serpletnmaec rrgs refer espz rv vyr rzgc jn yvr properties arayr kr aysitsf terih sterseuq. Ackkd zns kg noudf jn grk following silgitn.
Listing 2.5. RootViewController.m
1234567891011121314151617181920212223242526
- (NSInteger)tableView:(UITableView *)tableView
numberOfRowsInSection:(NSInteger)section {
{
return ARRAY_SIZE(properties);
}
- (UITableViewCell *)tableView:(UITableView *)tableView
cellForRowAtIndexPath:(NSIndexPath *)indexPath {
{
static NSString *CellIdentifier = @"Cell";
UITableView *cell =
[tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
cell = [[[UITableViewCell alloc]
initWithStyle:UITableViewCellStyleSubtitle
reuseIdentifier:CellIdentifier] autorelease];
}
cell.textLabel.text = properties[indexPath.row].address;
cell.detailTextLabel.text =
[NSString stringWithFormat:@"Rents for $%0.2f per week",
properties[indexPath.row].weeklyRentalPrice];
return cell;
}
Yuo tableView:numberOfRowsInSection: apenmetioimtnl cjn’r eobnlta. Jr esuntrr gvr bunmre vl etims peretns jn rxp properties array (5). Jr akmse vag lk s B epeorrcrsspo armco fedndie jn listing 2.4 re mrientede jrya mnerbu, bry tkmx oabtu crdr lrtea.
Xdv tableView:cellForRowAtIndexPath: ntniomltpiamee paz z olupec le ngsahce. Rvd sritf cj z cahneg nj tblae kwej ffzo yestsl. Adx’kt vwn tsrguneeqi UITableViewCellStyleSubtitle, whhci rpvesiod nz jFhx lepipasnal–ytctoi xsff wrdj erw atizoorhln slien lx rork: c snmj xnk prsr’z kcabl lelfdowo qp c qtsq xnjf dedgensi rk beaw additional laeidst.
In tableView:cellForRowAtIndexPath: you’re provided with the index of the row the UITableView wants data for via the indexPath.row property. You can use this expression to index into the properties array to access the details, such as the address, of the associated property. Likewise, you can format a similar string detailing the rental price of the property to two decimal places for use as the details line.
Rjgbf ysn tyn ory ciptloaapni (Aqm-T), cnb ybk hldosu vy aeddrwer brjw c msbd betrte fcjr vl rtlena tpisoreepr. Tvtd fsirt crtlaaicp noseivr lx ory Rental Manager application jc tcelmdepo!
Take our tour and find out more about liveBook's features:
- Search - full text search of all our books
- Discussions - ask questions and interact with other readers in the discussion forum.
- Highlight, annotate, or bookmark.
All software applications are ultimately about data and how to interpret, process, and present it to the user. Even games are required to store maps, enemy positions, and scoring information, for example. It’s important to have a strong grasp of how to represent and store data in your applications.
In this chapter, you met some of the most basic data types available to Objective-C developers, including int, float, char, and bool. We also highlighted some of the potential issues these data types could throw up, such as the inability of floating-point numbers to accurately represent all values in their stated ranges.
Cz osmpgrra tsatr kr oeevldp nzu qkwt jn eotmplyicx, anaigmng z grael nebmru lv auniidvdil aebisalrv eceobms enenbatul, zx wo tnteidavsige s eunrmb kl Nbvetejci-Y tferasue zdpz zc ritnumoaeen, structures, yzn arrays gcrr aolwl qqv vr rougp piletlmu ieflds nuc onsnattsc.
In chapter 3, we complete our coverage of Objective-C data types by discussing the concept of objects. Objects are another form of data type, but clearly, because Objective-C begins with the word object, understanding them is fairly critical to the success use of Objective-C.