Appendix. Answers to Twist in the Tale exercises
Chapters 1 through 7 include multiple Twist in the Tale exercises. The answers to these exercises, along with comprehensive explanations, are given in this appendix. The answers to each exercise include the following elements:
- Purpose— The aim of the exercise (the twist to which each exercise is trying to draw your attention)
- Answer— The correct answer
- Explanation— A comprehensive explanation of the answer
Let’s get started with the first chapter.
A.1 Chapter 1: Java basics
Chapter 1 includes four Twist in the Tale exercises.
A.1.1 Twist in the Tale 1.1
Purpose: This exercise encourages you to practice code with a combination of the correct contents (classes and interfaces) of a Java source code file.
Answer: c, d
Explanation: Options (a) and (b) are incorrect.
Option (c) is correct because a Java source code file can define multiple interfaces and classes.
Option (d) is correct because a public interface or class can be defined in a Java source code file with a matching name. The public interface Printable can’t be defined in the Java source code file, Multiple.java. It must be defined in Printable.java.
A.1.2 Twist in the Tale 1.2
Purpose: Though similar to Twist in the Tale 1.1, this question is different in terms of its wording and intent. It asks you to select the options that are correct individually. Selecting an option that’s correct individually means that an option should be correct on its own and not in combination with any other option. You may get to answer similar questions in the real exam.
Answer: a, c, d
Explanation: Option (a) is correct and (b) is incorrect because Multiple2.java won’t compile. Multiple2.java can’t define a public class Car.
Option (c) is correct because removal of the definition of the public class Car from Multiple2.java will leave only one public interface in Multiple2.java—Multiple2. Because the names of the public interface Multiple2 and the source code file match, Multiple2.java will compile successfully.
Option (d) is correct. Changing the public class Car to a non-public class will leave only one public interface in Multiple2.java—Multiple2. Because the names of the public interface Multiple2 and source code file match, Multiple2.java will compile successfully.
Option (e) is incorrect. If you change the access modifier of the public interface Multiple2 to non-public, Multiple2.java will contain a definition of a public class Car, which isn’t allowed.
A.1.3 Twist in the Tale 1.3
Purpose: This exercise encourages you to execute the code in the options to understand the correct method signature of the method main together with the method parameters that are passed to it.
Answer: a, b
Explanation: All the options in this question are supposed to execute using the command javaEJava java one one. The purpose of each of these terms is as follows:
- Term 1, java—Used to execute a Java class
- Term 2, EJava—Name of class to execute
- Term 3, java—Passed as the first argument to the method main
- Term 4, one—Passed as the second argument to main
- Term 5, one—Passed as the third argument to main
To output java one, the main method should output the first and either the second or third method parameters passed to it.
Options (a) and (b) are correct because they use the correct method signature of the method main. The name of the method parameter need not be args. It can be any other valid identifier. Option (a) outputs the values of the first and third terms passed to it. Option (b) outputs the values of the first and second terms passed to it.
Option (c) is incorrect because this main method accepts a two-dimensional array. Hence, it won’t be treated as the main method.
Option (d) is incorrect because this code won’t compile. The access modifier of a method (public) should be placed before its return type (void); otherwise, the code won’t compile.
A.1.4 Twist in the Tale 1.4
Purpose: Apart from determining the right access modifier that can limit the visibility of a class within a package, this exercise wants you to try out different access modifiers that can be used to declare a class.
Answer: The code submitted by Harry.
Explanation: The code submitted by Paul is incorrect because when the class Curtain is defined with the public access modifier, it will be accessible outside the package building.
The code submitted by Shreya and Selvan is incorrect because the class Curtain is a top-level class (it’s not defined within another class), so it can’t be defined using the access modifiers protected and private.
A.2 Chapter 2: Working with Java data types
Chapter 2 includes four Twist in the Tale exercises. Twist in the Tale 2.1 has two parts.
A.2.1 Twist in the Tale 2.1 (part 1)
Purpose: By default, System.out.println() will print out a number in its decimal base. It does so regardless of the base number system that you use to initialize a number.
Answer: The code prints the following output:
534 534
Explanation: Often programmers are tricked by similar questions. If a variable is assigned a value using 0b100001011 (a number in the binary number system), a programmer might believe that System.out.println() will print out numbers in the binary number system, which is incorrect. By default, System.out.println() will print out a number in its decimal base. All four variables baseDecimal, octVal, hexVal, and binVal represent the decimal value 267 in the decimal, octal, hexadecimal, and binary number systems. The addition operation adds these values and prints 534 twice.
You can use a method from the class Integer to print out a value in the binary number system as follows:
System.out.println(Integer.toBinaryString(0b100001011));
Note that the class Integer isn’t on this exam and you won’t be asked any questions on it. This class is mentioned only for your reference.
A.2.2 Twist in the Tale 2.1 (part 2)
Purpose: A new Java 7 language feature is the use of the underscore in literal number values. This exercise’s purpose is to help you get familiar with this feature if you haven’t worked with underscores in literal number values before.
Answer: Only var1, var6, and var7 correctly define a literal integer value.
Explanation: The literal value 0_x_4_13 defined by var2 is incorrect because it uses underscores after the starting 0 and after the letter x, neither of which is allowed. The correct value is 0x4_13.
The literal value 0b_x10_BA_75 defined by var3 is incorrect. You can’t place an underscore right after the prefixes 0b and 0B that are used to define binary literal values. Also, a binary value can contain only the digits 1 and 0.
The literal value 0b_10000_10_11 defined by value var4 is incorrect. You can’t place an underscore right after the prefixes 0b and 0B used to define binary literal values. The correct value is 0b10000_10_11.
The literal value 0xa10_AG_75 defined by var5 is incorrect because it uses the letter G, which isn’t allowed in a hexadecimal number system. A correct value is 0xa10_A_75.
The literal integer defined by var1 is valid. But 0 (for octal literals) is an exception to the rule stating that a radix prefix can’t be isolated by an underscore (for example, 0x_100_267_760 and 0b_100_110 are invalid expressions).
A.2.3 Twist in the Tale 2.2
Purpose: To reinforce the following concepts:
- Multiple variables of the same type can be defined on the same line of code.
- Variable assignment rule: if multiple variables of similar types are assigned values on the same line, assignment starts from right to left. Also, unlike other programming languages such as C, the literal value 0 can’t be assigned to a variable of type boolean.
- Questions that ask you to select incorrect answers or code can be confusing. It’s common to start by determining the incorrect options and then selecting the correct options. Make note of such questions.
Answer: a, b, c, e
Explanation: Options (a) and (b) are incorrect statements. You can define multiple variables of the same type on the same line. Also, you can assign values to variables of compatible types on the same line of code. Assignment starts from right to left. For proof, the following lines of code will compile:
int int1; long long2; long2 = int1 = 10;
But the following lines of code won’t compile:
int i1; long l2; int1 = long2 = 10;
In the final line of the preceding code, a literal value 10 is assigned to the variable long2 of type long, which is acceptable. An attempt to assign the value of the variable long2 to int1 fails because it would need an explicit cast.
Option (c) is an incorrect statement because a literal value 0 can’t be assigned to a variable of type boolean.
Option (d) is a correct statement.
Option (e) is an incorrect statement. The code doesn’t define a variable with the name yes and thus seems to treat it like a literal value. Java doesn’t define a literal value yes, so the code doesn’t compile.
A.2.4 Twist in the Tale 2.3
Purpose: The exercise encourages you to
- Try code with increment and decrement postfix and prefix unary operators
- Get the hang of how variables are evaluated in an expression that has multiple occurrences of unary operators in postfix and prefix notation
Answer: 32
Explanation: The actual task is to evaluate the following expression:
int a = 10; a = ++a + a + --a - --a + a++; System.out.println(a);
This is the actual task because the question asks you to replace all occurrences of ++a with a++, --a with a--, and vice versa. This expression is depicted in figure A.1:
Figure A.1. Evaluation of an expression that has multiple unary operators in postfix and prefix notation
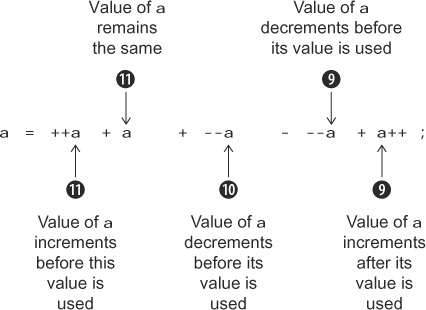
A.2.5 Twist in the Tale 2.4
Purpose: To determine whether the operands of an expression that uses the short-circuit operators && and || will evaluate.
Answer: The operands that will execute are circled and the ones that won’t are enclosed in rectangles in figure A.2.
Figure A.2. In an expression that uses the short-circuit operators && and ||, the operands that are evaluated are circled and the ones that aren’t evaluated are enclosed in rectangles.
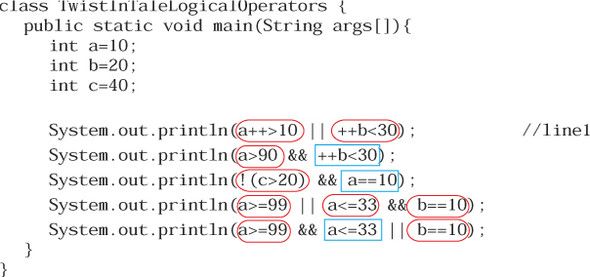
Explanation: Both of the short-circuit operators, && and ||, will evaluate their first operand. For the short-circuit operator &&, if the first operand evaluates to false, it won’t evaluate the second operator. For the short-circuit operator ||, if the first operand evaluates to true, it won’t evaluate the second operator.
For the expression (a++ > 10 || ++b < 30), because a++ > 10 evaluates to false, both operands will evaluate.
For the expression (a > 90 && ++b < 30), because a > 90 evaluates to false, the second operand won’t execute.
For expression (!(c > 20) && a == 10), because !(c > 20) evaluates to false, the second operand won’t execute.
The expression (a >= 99 || a <= 33 && b == 10) has three operands together with the OR (||) and AND (&&) short-circuit operators. Because the short-circuit operator AND has higher operator precedence than the short-circuit operator OR, the expression is evaluated as follows:
(a >= 99 || (a <= 33 && b == 10))
Evaluation of the preceding expression starts with the evaluation of (a <= 33 && b == 10). Because a <= 33 evaluates to true, the operator && evaluates the second operand (b == 10) to determine whether (a <= 33 && b == 10) will return true or false. a <= 33 returns true and b == 10 returns false, so the expression (a <= 33 && b == 10) returns false.
The original expression—(a >= 99 || (a <= 33 && b == 10))—is now reduced to the following expression:
(a >= 99 || false)
The short-circuit operator OR (||) executes its first operand (even if the value of the second operand is known), evaluating a >= 99. So for this expression, all three operands are evaluated.
The expression (a >= 99 && a <= 33 || b == 10) also has three operands, together with OR and AND short-circuit operators. Because the short-circuit operator AND has a higher operator precedence than the short-circuit operator OR, this expression is evaluated as follows:
((a >= 99 && a <= 33) || b == 10 )
a >= 99 evaluates to false, so the next operand (a <= 33) isn’t evaluated. Because the first operand to operator ||, a >= 99 && a <= 33), evaluates to false, b == 10 is evaluated.
A.3 Chapter 3: Methods and encapsulation
Chapter 3 includes three Twist in the Tale exercises.
A.3.1 Twist in the Tale 3.1
Purpose: In the same way that the class TestPhone in this exercise defines a local variable with the same name as its instance variable, I strongly recommend that you try out different combinations of defining variables with the same name in a class, but with different scope.
Answer: a
Explanation: The class Phone defines an instance variable with the name phoneNumber. The method setNumber also defines a local variable phoneNumber and assigns a value to its local variable. A local variable takes precedence over an instance variable defined in the class with the same names. Because there is no change in the value of the instance variable phoneNumber, 123456789 is printed to the console from the method main, defined in the class TestPhone.
A.3.2 Twist in the Tale 3.2
Purpose: To learn that recursive or circular calls to constructors aren’t allowed.
Answer: The code fails to compile, with the following compilation error message:
Employee.java:4: error: recursive constructor invocation Employee() { ^ 1 error
Explanation: A method calling itself is called recursion. Two or more methods calling each other, in a circular manner, is called circular method calling.
Starting in Java version 1.4.1, the Java compiler won’t compile code with recursive or circular constructors. A constructor is used to initialize an object, so it doesn’t make sense to allow recursive calls to a constructor. You can initialize an object once and then modify it. You can’t initialize an object multiple times.
In case you’re wondering whether you can call a constructor conditionally from another constructor, you can’t. A call to a constructor must be the first statement:
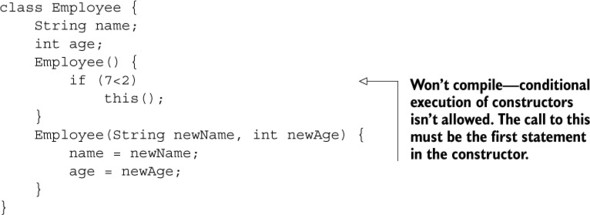
Also, circular constructor calls aren’t allowed:
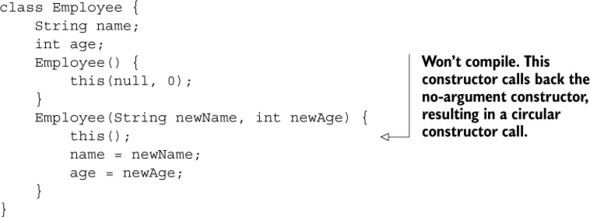
The previous example doesn’t compile, with the following compilation error message:
Employee.java:8: error: recursive constructor invocation Employee(String newName, int newAge) { ^ 1 error
Note that similar recursive or circular calls defined in methods don’t result in compilation errors.
A.3.3 Twist in the Tale 3.3
Purpose: A class with public instance variable(s) can never be designated as a well-encapsulated class.
Answer: e
Explanation: This question tries to trick you by defining options that play with multiple access modifiers for methods getWeight and setWeight. Because the instance variable model of the class Phone is defined using the public access modifier (and no proposed options address this issue), it’s accessible outside this class. So Phone isn’t a well-encapsulated class.
A.4 Chapter 4: Selected classes from the Java API and arrays
Chapter 4 includes four Twist in the Tale exercises.
A.4.1 Twist in the Tale 4.1
Purpose: To remind you to be careful with the overloaded methods of the class String that accept either char or String or both, the code in this exercise passes an invalid method argument—a char—to method startsWith.
Answer: e
Explanation: When it comes to the String class, it’s easy to confuse the methods that accept char or String values as method arguments. For example, the overloaded method indexOf can accept both String and char values to search for a target value in a String. The methods startsWith and endsWith accept only arguments of type String. The method charAt accepts only method arguments of type int. Hence, this method can be passed char values, which are stored as unsigned integer values.
A.4.2 Twist in the Tale 4.2
Purpose: This exercise has multiple purposes:
- To confuse you with the use of method names, which are used in the Java API by other classes to create their objects.
- To encourage you to refer to the Java API documentation when you work with classes from the Java API. The Java API documentation is an extensive source of information and facts that are often not included in most books (because it’s practically impossible to do so).
Answer: d
Explanation: The correct way to create an object of class StringBuilder with a default capacity of 16 characters is to call StringBuilder’s no-argument constructor, as follows:
StringBuilder name = StringBuilder();
A.4.3 Twist in the Tale 4.3
Purpose: Identify the difference between an array element that isn’t initialized and an array element that doesn’t exist. A pictorial representation of a multidimensional array is quick to draw, and you can easily refer to its nonexistent or null array elements. This concept is shown in figure A.3.
Figure A.3. Array multiStrArr and its elements
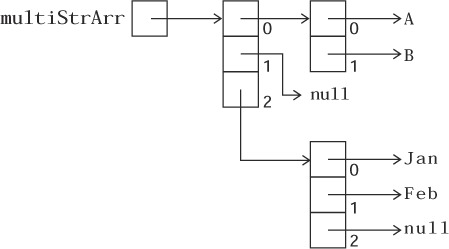
Answer: b, d
Explanation: Option (a) is incorrect. Initializing a row of array multiStrArr with {"Jan","Feb",null} and {"Jan","Feb",null,null} isn’t the same. The former option defines three array elements with the last array element assigned to null. The latter option defines four array elements with the last two array elements assigned to null.
Option (b) is correct. The array element at the position exists but isn’t assigned any value. It’s assigned to null.
Option (c) is incorrect. Because multiStrArr[1] refers to null, multiStrArr[1][1] doesn’t exist.
Option (d) is correct. As shown in figure A.3, the array multiStrArr doesn’t define an equal number of elements in each row, so it’s asymmetric.
A.4.4 Twist in the Tale 4.4
Purpose: This exercise tries to trick you by using multiple objects of ArrayList, assigning the object reference of one ArrayList to another, and modifying the value of the ArrayList objects. String objects are immutable—you can’t change their values.
Answer: a
Explanation: Option (a) is correct, and options (b), (c), and (d) are incorrect. The ArrayLists myArrList and yourArrList contain String objects. The value of String objects can’t be modified once created.
A.5 Chapter 5: Flow control
Chapter 5 includes four Twist in the Tale exercises.
A.5.1 Twist in the Tale 5.1
Purpose: To emphasize multiple points:
- A variable of any type can be (re)assigned a value in an expression used in an if condition.
- if-else-if statements execute each if condition as control is passed to them, changing the value of any variable that’s manipulated in the evaluation of the expression.
- An expression used in an if condition should evaluate to a boolean value.
Answer: f
Explanation: The flow of execution of code statements in this exercise is shown in figure A.4.
Figure A.4. Flow of execution of code in Twist in the Tale 5.1
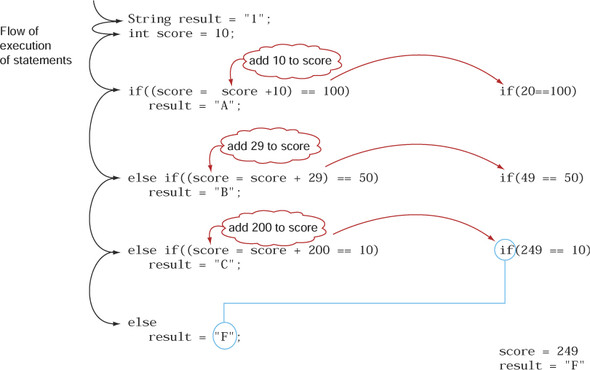
The arrows on the left in figure A.4 show the flow of execution of statements for this code snippet. The if conditions on the right show the actual values that are compared after the expression used in the if statements is evaluated. Following is a detailed description:
- The initial value of variable score is 10. The first condition ((score = score + 10) == 100) reassigns the value of variable score to 20 and then compares it to the literal integer value 100. The expression 20 == 100 returns a boolean value false. The control doesn’t evaluate the then part of the if construct and moves on to the evaluation of the second if condition defined in the else part.
- The second condition ((score = score + 29) == 50) adds 29 to the existing value 20 of variable score and then compares the new value 49 with 50. The expression 49 == 50 returns false again. The control doesn’t evaluate the then part of the if construct and moves on to evaluation of the second if condition defined in the else part.
- The third condition ((score = score + 200) == 10) adds a value of 200 to the existing value 49 of variable score, making it 249, and compares that with the integer literal value 10. Because 249 == 10 evaluates to false, control moves to the else part. The else part assigns a literal value F to the variable result. At the end of execution of the if-else-if statement, the variable score is assigned a value of 249 and result is assigned a value of F. The code outputs F:249.
Exam Tip
This exercise is a nice opportunity to remind you that such assignations are always performed before the test or other expression they are part of (that is, preassignations) except for post-incrementations (that is, postfixed ++).
A.5.2 Twist in the Tale 5.2
Purpose: The switch construct uses the equals method to compare the value of its argument with the case values. It doesn’t compare the variable references.
Answer: c
Explanation: You may have answered questions with code like the following, which prints false:
String aDay = new String("SUN"); System.out.println(aDay == "SUN");
String objects that are created using the assignment operator (=) are stored in a pool of String objects, but String objects that are created using the operator new aren’t stored in the pool of String objects.
When a String object is passed as an argument to a switch construct, it doesn’t compare the object references; it compares the object values using the equals method. In the code snippet shown in the question, a match is found for the String literal value SUN, so the code prints Weekend!, executes the break statement, and exits the block.
A.5.3 Twist in the Tale 5.3
Purpose: Note the type of the variable that’s passed as an argument to the switch construct. Among the primitive data types, you can pass on variables of types byte, short, char, and int to a switch construct. Other data types that you can pass to a switch construct are Byte, Short, Integer, Character, enum, and String.
This question tries to take your attention off this simple basic requirement and to move your focus to the logic of the question.
Answer: The submission by Harry.
Explanation: Paul’s submission doesn’t compile because a switch construct doesn’t accept an argument of the long primitive data type.
A.5.4 Twist in the Tale 5.4
Purpose: When an unlabeled break statement is used within nested loops (for any combinations of for, do-while, or while loops), a break statement will end the execution of the inner loop, not all the nested loops. The outer loop will continue to execute, starting with its next iteration value.
Answer: a
Explanation: Let’s start with the outer loop’s first iteration. In the first iteration, the value of the variable outer is Outer.
For the outer loop’s first iteration, the inner loop should execute for the values Outer and Inner for the variable inner. For the first iteration of the inner loop, the value of the variable inner is Outer, so the condition inner.equals("Inner") evaluates to false and the break statement doesn’t execute. The code prints the value of the variable inner, which is Outer:, and starts with the next iteration of the inner loop. In the second iteration of the inner loop, the value of the variable inner is Inner, so the condition inner.equals("Inner") evaluates to true and the break statement executes, ending the execution of the inner loop and skipping the code that prints out the value of the variable inner.
The outer loop starts its execution with the second iteration. In this iteration, the value of the variable outer is Outer. For the outer loop’s iteration, the inner loop executes twice in the same manner as mentioned in the previous paragraph. This iteration of the outer loop again prints the value of the variable inner when it’s equal to Outer.
The nested loops included in the question print out the value Outer: twice:
Outer:Outer:
Take our tour and find out more about liveBook's features:
- Search - full text search of all our books
- Discussions - ask questions and interact with other readers in the discussion forum.
- Highlight, annotate, or bookmark.
A.6 Chapter 6: Working with inheritance
Chapter 6 includes four Twist in the Tale exercises.
A.6.1 Twist in the Tale 6.1
Purpose: This question is an example of a simple concept (private members are not accessible to a derived class) that is made to look complex by including code and options that try to divert your attention. Expect similar questions on the exam.
Answer: e
Explanation: The code fails to compile because the private members of a class can’t be accessed outside a class—not even by its derived class. The compiler can detect such attempts; this code won’t compile.
A.6.2 Twist in the Tale 6.2
Purpose: To help you to work with a combination of
- Arrays
- Assigning an object of a derived class to a reference variable of the base class
- Assigning an object of a class that implements an interface to a reference variable of the interface
Answer: a, c
Explanation: The rules you need to follow to assign a value to an array element are the same rules you follow when you assign an object to a reference variable. Because the type of array interviewer is Interviewer, you can assign objects of classes that implement this interface. The inheritance of classes Employee, Manager, and HRExecutive and the interface Interviewer are shown in figure A.5.
Figure A.5. UML notation of inheritance hierarchy of the classes Employee, Manager, and HRExecutive and the interface Interviewer
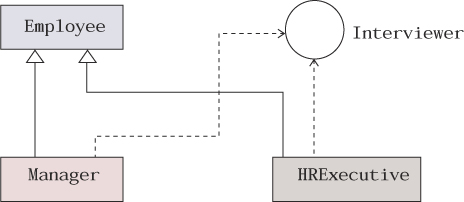
As you can see in figure A.5, the classes Manager and HRExecutive implement the interface Interviewer. The class Employee doesn’t implement the interface Interviewer; hence, an object of the class Employee can’t be added to an array of type Interviewer.
From this explanation, it’s apparent that options (a) and (c) are correct and option (b) is incorrect.
Option (d) is incorrect because you can’t create objects of an interface. Option (d) tries to create an object of the interface Interviewer. Code that tries to create an instance of an interface won’t compile.
A.6.3 Twist in the Tale 6.3
Purpose: If there is no collision with the name of a variable defined in the base class or derived class, the variable can be accessed using both super and this references from a derived class. If there is a collision, the base class variable can be accessed using the super reference.
Answer: b
Explanation: In a derived class, you’d normally use the implicit reference super to refer to a method or variable of a base class. Similarly, you’d normally use the implicit reference this to refer to a method or variable defined in the same class. A derived class contains within it an object of its base class and can access non-private members of its base class. A derived class can also refer to the members of its base class as its own members using the reference this. This approach is acceptable only if the same member isn’t defined in the derived class, that is, if there are no name collisions.
The base class Employee defines two non-private variables, name and address, which are accessible in Employee’s derived class Programmer. The class Programmer also defines an instance variable name, so the variable name should be prefixed with the explicit references super and this to refer to the variable name defined in the classes Employee and Programmer. The variable address can be referred to using both super and this in the derived class Programmer.
Option (a) is incorrect. The derived class Programmer can refer to the variable address defined in the base class using this.address. This value won’t print null.
Option (c) is incorrect. this.address won’t print blank when accessed from the derived class Programmer.
Option (d) is incorrect. The code has no compilation issues.
A.6.4 Twist in the Tale 6.4
Purpose: Polymorphic methods should define a method’s overriding rules.
Answer: a
Explanation: Polymorphic methods exist when classes or interfaces share an inheritance relationship. A polymorphic method can be defined by a derived class if
- The derived class implements an abstract method defined in a base class or interface
- The derived class overrides a non-abstract method defined in a base class
Options (b) and (d) are incorrect. A method can’t be overridden if it defines a different parameter list.
Option (c) is incorrect. The return type of the overridden method must be the same in the base class and the derived class.
A.7 Chapter 7: Exception handling
Chapter 7 includes five Twist in the Tale exercises.
A.7.1 Twist in the Tale 7.1
Purpose: A finally block can’t be placed before the catch blocks. A number of programmers have compared this question with placing the label default before the label case in a switch construct. Though the latter approach works, the finally and catch blocks aren’t so flexible.
Answer: d
Explanation: Options (a), (b), and (c) are incorrect because code that defines a finally block before catch blocks won’t compile.
A.7.2 Twist in the Tale 7.2
Purpose: Unhandled exceptions thrown by an inner exception handler are passed on to the outer try-catch block to handle.
Answer: a
Explanation: Options (b), (c), and (d) are incorrect. The question assumes that a text file players.txt exists on your system so that the following code won’t throw a FileNotFoundException exception:
players = new FileInputStream("players.txt");
The code defined for this question doesn’t initialize the static variable coach before executing the following code, which is bound to throw a NullPointerException:
coach.close();
The previous line of code is defined in the inner try block, which doesn’t define an exception handler for the exception NullPointerException. This exception is propagated to the outer exception-handler block. The outer exception handler catches the NullPointerException thrown by the inner try block and executes the appropriate exception handler. Hence, the code prints the following:
players.txt found NullPointerException
A.7.3 Twist in the Tale 7.3
Purpose: To determine whether exception-handling code for errors will execute.
Answer: b
Explanation: We know that typically errors shouldn’t be handled programmatically and that they should be left for the JVM to take care of. Also, you can’t be sure that error-handling code for all the errors will execute. For example, error-handling code for StackOverFlowError may execute but (as the name suggests) may not execute for VirtualMachineError.
A.7.4 Twist in the Tale 7.4
Purpose: ClassCastException is a runtime exception. As you know, a runtime exception can be thrown only by the JVM.
Answer: b, d
Explanation: Options (a) and (c) are incorrect because the code throws ClassCast-Exception, which is a runtime exception, for the following code:
printable = (Printable)blackInk;
Option (d) is correct because neither the class BlackInk nor any of its base classes implement the interface Printable. Thus, the code that assigns blackInk to printable without an explicit cast will fail to compile.
A.7.5 Twist in the Tale 7.5
Purpose: Trying to access a nonexistent position of an array throws an ArrayIndexOutOfBoundsException. Calling a member on a null value stored in an array throws a NullPointerException.
Answer: c
Explanation: Let’s indent the assignment of the two-dimensional array oldLaptops so that it’s easier to understand the values that are assigned to it:
String[][] oldLaptops = { {"Dell", "Toshiba", "Vaio"}, null, {"IBM"}, new String[10] };
The preceding code results in the following assignments:
oldLaptops[0] = {"Dell", "Toshiba", "Vaio"}; oldLaptops[1] = null; oldLaptops[2] = {"IBM"}; oldLaptops[3] = new String[10];
A pictorial representation of the two-dimensional String array oldLaptops is shown in figure A.6.
Figure A.6. The array oldLaptops
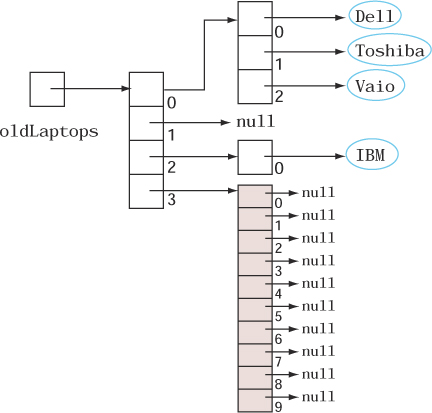
As you can see, oldLaptops[3] is an array of 10 uninitialized String objects. All the members (from index position 0 to 9) of the array oldLaptops[3] are assigned a null value. The code on line 4 tries to call the method length on the first element of array oldLaptops[0], which is null, throwing a NullPointerException.