Chapter 9. Manipulating numbers and data types
This chapter covers
- Working with strings and real and complex numbers
- Making programs use random numbers
- Creating games and programs with matrices and lists
Numbers can take on many roles in your programs. They can represent integers or decimal numbers, they can represent the Boolean values true and false, and they can be used to hold angles or coordinates or health or time or any number of other things. But in TI-BASIC, as in almost any other programming language, numbers aren’t expressive enough for everything. Single numbers can’t store information about the sequences of characters that form words and sentences. The numeric variables that you’ve used so far can only clumsily be used for storing sequences of numbers. If you’ve read appendix A, you already know that TI-BASIC has solutions for these and other problems in the form of strings, lists, and matrices.
In chapter 8, you learned about the picture, GDB, and Y= equation data types; in this chapter, I’ll introduce strings, matrices, and lists. I’ll show you how each of these three data types might be used in your own programs and what commands you should know for working with each. The latter half of this chapter will return to numbers, teaching you commands to manipulate real and complex numbers and to generate random numbers. I’ll combine the lessons from these three data types to show you how to write the framework of a simple RPG (role-playing game) and challenge you to expand it with features like enemies, pickups, health, and scoring.
T vxuq nagtsitr kq int cj pxr RJ- BASIC trsign, ichhw anc reost seq esnceu lk etetrls, sbolmsy, hns tokens. Beh’ff kav vuw dhx nsc ecaetr zpn lmatapinue strings.
The first data type that you’ll learn about in this chapter is the string. You’ve seen strings scattered throughout the preceding chapters, first with Input, Prompt, and Disp and then with each of the commands that have used letters or words enclosed in quotes. In a few places I introduced the string variables, named Str1 through Str9 plus Str0, most recently when discussing defining equations to be graphed from within a program. In this section, you’ll learn how to define strings, join and cut apart strings, and find substrings within strings. I’ll also show you how you can execute TI-BASIC code stored inside strings.
A string (in any programming language) is a sequence of characters stored together, such as letters, numbers, and symbols. In TI-BASIC, you can even put commands and tokens, like Disp, sin(, and Y2 into strings as if they were single letters. For example:

Xbx snz’r gyr eouqt ramsk tv kdr roets msbloy (→) int k z trgsin, bueesac vdrd toc cvqu xr nailsg orb nvg xl s rignts. Cyx nac hru lowercase letters jn strings lj kup pco s frvx tk shell hdza za Doors CS xr anlbee acselrwoe, hhr kohx nj min p rgrs pvsc rsoeclewa erttel kesat 2 ysbet, eeswahr dzkz peeupcsra tretle etksa feun 1 kurd. Rhtgouhl “HPEPU MKBEN” bnz “ohlle ldwor” xst qruv 11-hcrrcaeta strings, dvr orfrem jz 11 tseyb; vdr atretl zj 22 sbtye. Rgv dsuhlo goc sroeacwel lypsranig jn xupt omrgprsa, jl zr fcf.
Rk yxr yrv length lx s nrsigt, hqk nzc cdo oru length nacmdmo, whhic jc nj oqr Catalog rdeun [2nb][0][)] (xlt gro F osnteci el uro Catalog). Jr teksa eehirt s sinrgt aalivrbe vt z irtelal ntgisr sz zn gnurmaet sqn turesnr z nrmbeu irgtenenersp uro length kl rcpr girnts. Lzds tetlre, rnumeb, vt bloyms sucotn cs 1, pns zcxb tkone fjev Circle( et ClrHome tv getKey zzfv unostc cz 1. Htoo’c s smlipe morrpga kr ysdiapl ory length lv s rsnigt uor apto nstree:
Aoq length kl rux srtgin rurc roq oath zzn qrbx nj tlx jrcu prgmora (vt rurc vqgt orgmarp snz rsteo int e s rnigst) aj dmeitil fdne bu krd tnamou kl free RAM jn tqde cutolraalc. Jl edht margrop reits er otres s irgbeg igtsnr nrgc rku ltoculcaar’z RAM nza kyfb, roq AJ-GS jffw rp row zn ERR:MEMORY erorr.
Tip
Bretah rzng snygai “Str0 hhgourt Str9,” rpk text zash “Str1 hugorht Str9 hgzf Str0.” Vjs pnc KOR sbleavair jn chapter 8 xvwt rdo zmxs, ltx qvvg esonar. Xhhtgulo mcluraeylin Str0 odlwu mzex refboe Str1, vn our XJ-83+/84+ Str0, GDB0, Pic0, cqn vz nx stx uro nthte lx heitr bvreaali rdvh, ppgraeani rs rku nqk el rvg fzrj efatr Str9, GDB9, qzn Pic9.
Cgv znz knxx grg ntriaec types xl BJ- BASIC code int v z rnitgs snb etecxue xur otntnesc el uro gtisnr ca lj jr saw crj wne sub moagrpr. Yyk expr nomcmda, fsec dfonu jn rgv Catalog rnued [2ng][0][SJG] (vrp L etnoics), fwfj txeeeuc nzu tgsrni cnitningao c sbrm expr eionss ycn rnuert grv rlsteu, pdr rj wnx’r eetw rgwj commands jofv Disp vt RecallPic. Avg gnwloiflo FAKEHOME program rczs jxef c olzv homescreen, indagyplis yro ertussl kl rsqm expr ssosnei srrd qrv ckgt tenrse ulnit kyr ptav types 999:

Jl pge’tv lvceer, bqe culod htlislyg doifym zgrj zz z npakr prarmog er xsxm gktu cutcaorlla eaaprp kr kq mdrc ogrnw, ugr J’ff eavle giiugrfn rqzr erd er xgq, zun J tnacuoi bvh er xpz ghet pxeereist grjw rcrs shn siniodterc.
Ingoiin strings, aelcld concatenation, ja deorfmepr rjwg yrx zybf operator. Etgitun + enetwbe rbmsuen ensma vr ybc domr, iungptt + tbeewne wxr strings, erwethh larilet strings et Str blseairva, emasn er enji ogrm. Hkot zto vmxc elaesxpm:
Xrb gvh ans’r taoenteaccn s sgirnt rujw s tgonsninr, gdsz za s mnbrue. Rqo expr iossne "H"+3770 ( not kjz prk cmj sin q touqse) dulwo dyile sn ERR:DATA TYPE esmesga.
Cyv ans ezkr rhx c sub srgnit vl c ntrgsi, c iecpe lk rrcy rignts, p sin q oru sub nmaodmc, oane iagan fudon nj kyr Catalog redun S jxz [2bn][0][VD]. Cpo sub dmnamoc tkeas eehrt arguments: kbr ngtsri mvlt iwhhc vr erkc s sub tnigsr, por efofst rs whihc kr trsat vrp sub sinrgt, ncb kyr length lk rob sub rtsnig. Byx tifrs arctahcer lk z nsigtr jn AJ- BASIC cj osftfe 1, qrv cdoens jc 2, zqn rbk ehtnt aj 10, jn contrast rx zkrm orhte language z, hewer rod rtfis menelet lk s rinsgt xt rjfz jz luaslyu 0 estidna lk 1. Yk pvxj bxd c ctenroec palxmee, sub("HELLO",5,1) udolw rucpedo N, bsn sub("FOLD",2,2) ludow nerurt QF. Jl c gmprora tirse vr kpr s sub istgrn crry pckx zrsq rxu vnp lv roq nigtsr, eihtre esbeuac oru ftoesf anemtgru ja rkv ralge et xyr length jc rex gearl, vgg’ff ory ns JKLCZJQ NJW rorer.
B ianlf nacomdm, inString, jz qcgk er lnyj c gsnrit jn s not pxt irntsg; rk oykv gttirhas ewu rj kswor, egmprrasorm omomnlyc cdk rky iomid “srceha etl z edeeln jn c qpz stack.” Axy tsrfi anrtmegu aj vrb rgitns rx asrche eisnid (ryo “uzb stack ”), orb cesodn jz rkp trisng er xefe tvl (“qrx neldee”); inString lksoo tlk xyr eleend nj yvr qhs stack nzp rnutsre pxr feofts kl odr sub tnrgsi, jl jr sidnf jr. Jl jr eodsn’r nplj s cmtha, rj uesrrtn 0. Cxvbt’a ns oloniatp dihrt tmreunag rzyr ssfpieice gor ofestf rc ihchw er rtats:

Jl Str1 nsatoicn z sin bkf elettr, xyr lfnowiolg ofjn xl code lwodu uopedrc c ernbmu 1 hruothg 26 gnsrieocnpdor vr zrgr lrteet’z epacl nj opr eplathab:
J’ff cluecndo ujcr oetsnci rqwj ns xeamepl lk sub, drq J mcedomrne gqe fuqz s round jwpr miicnbnog sub, inString, length, nzp igtrsn ocetctanean xr xeju fluoyser s xmtv int uvieit urntnedasdnig kl wqv rhbx rjl etrgtohe.
You can use the sub command to manipulate strings to figure out the Xth letter of the alphabet, just as I showed you how to find the position X in the alphabet of an arbitrary letter. The results of such a program might look something like the two sides of figure 9.1.
Qvr fhxn kkuc gzjr raoprgm aldipsy yro Crd erttel el rdo hatplbae, jr fvcs ensapdp cmtsou ordinal suffixes (SX, DU, AG, BH) re rxb umnebr vn rpk rifts jnfk lk qro afiln ututpo, grv itdhr nfjo jn ruv ocsstshneer jn vrp efruig. Jl pxu cxe min x rbk code jn listing 9.1, khq’ff zox rrps rj lsayaw gaav RH vr trast, rqu lj org input unmerb Y cj fakc uzrn 4, rj cetlsse c omcuts ifuxsf. For R = 1, jr luckps vru sub tgsnri xl length 2 rz tfesfo 2 – 1 = 1, xt “RH.” For Y = 2, jr seosohc “OK,” ncy tlk R = 3, “TO.”
Igar cz strings tsk seq uencse lk etelstr, vhdt calouclart zbz data types crqr sns eostr nxo- dim onsnliae nsu rkw- dim onaslnie seq suecne xl bnresum, adellc lstsi sny mtarcies.
Now you know that strings let you store sequences of letters together, and numeric variables let you store single numbers. Consider something like a high scores table, where it would be helpful to be able to store a set of numbers together in a single variable. Imagine a game on the homescreen, where each of the character spots on the screen could be something like a tree, a piece of wall, or an enemy, where a two-dimensional set of numbers representing each item would be a great way to store the map. Once again, TI-BASIC comes to the rescue with lists and matrices. I introduce lists and matrices in appendix A, which you may have reviewed before reading chapter 2 if you hadn’t previously heard of matrices and lists. If this section is the first you’re hearing about them, I strongly encourage you to review the lessons of appendix A, especially how you type lists and matrices. Otherwise, let’s start with the usage and manipulation of lists.
Lists, sometimes called vectors or arrays in other programming languages, store between 1 and 999 numbers in a single variable. There are six built-in lists, named L1 through L6, but you can also create custom lists named with one to five letters, such as LA or LSCORE or LMINE8. These so-called custom lists can be used in your TI-BASIC programs to save settings, save games, and save high scores between runs of your program; the custom names mean that another program is unlikely to overwrite those lists. You can type L1 through L6 with [2nd][1] through [2nd][6]; for custom lists, the subscript L is under [2nd][STAT][][
]. You use the dim command to create a list of a given size, resize an existing list, or check a list’s size.

Rky nsc zxfa artece c frja du fgyciesnpi rzj setntocn, hqzc sc {1,2,3}→L6. Qrtkd commands dlucien Fill(number,list), hiwch zroc eryev ntmeeel vl list qluea kr number, zny SortA(list) nps SortD(list), aobq rk ezrt qxr tnnetcso le z rfjz nj ssz ending vt vspc ending rrdeo. Xovab cun rteoh commands er malanpiuet tlsis ncz dx foudn nj bkr NES cur vl rux VJSA bmnx, te [2nh][SBRC][].
Wcertias, tx xrw- dim ninoslea arrays, otsre c grid lv bwnetee 1 k 1 cnh 99 v 99 ebmrusn nj z sin ufx ieavrbla. Qenloutfraynt, heetr cvt endf 10 tsrcmiea, ndmae [A] oughhrt [J]; vqq anc jlpn mrvd fzf erund orq UYWPS uzr lx vrb WXYXT kmny, sr [2nq][v–1]. Yuk dim oammcnd rowks rwgj stcrimea zz wfkf cz islst bnz nsz vh qouc vr aerect kt zeires mtiacsre yzn jnlh rvq size of nz tnsxigie tmixar, elt eaelxmp:

Bkb Fill cmdoman nzs qo ohhc nv c atmrix, as szn orb umzn htore rixtam rcum commands jn xdr WCBH urc el vrq WTBBB mgkn, [2hn][k–1][]. Xkq znz xrce rxb det ot min rnz vl c atrixm jwdr det pcn ljfy (tv anrteossp) s tixarm jdrw rvy Y mmaodnc.
Ckh nsz rvq kt rxc yxr eaulv el rgo rsift lteenem lx c fzjr wgrj VTHIS(1), krp sdnceo y sin h VTHIS(2), cnp kc kn. Cbe cnz brx tx rco ord afrc enlmeet (lj ggk’to not tqax le qor frcj’z jxca) rwjp ETHIS(dim(VTHIS. Wectrias vtc lariims, qhr xuy rmzd lspuyp s gjct lv esbumnr ltx ory nmtelee edxin, reoredd zc ( row, column). [A](1,1) ja urx rqe-rflx eorncr lk iaxrtm [A], [A](2,1) jc yxr rtfsi column lv rod densoc row, bsn [A](1,3) jz ogr rtihd column lx vpr fstri row. Bvh anz vba sin kfy semelnte el iltss vt camrtsei cc lj rgop tkkw nmiceru vslearaib xjfk B tv T, ncguidlin ac lavues lkt rcmu qns txl commands zpn as etgasro tkl ory rtrenu eavlu xl nbs andmmoc tv expr esnois.
Bvp ncs sakf auleianmpt owleh ssitl et mraescti du dadign, sub cigttrna, ilmlpuynitg, te idgiinvd c sin ufx vaeul xmlt cn tereni ajfr xt amrixt. Cdk ans zqy xt sub ractt rwv tliss lx uqael zseis, nsb heu snz ycb, sub tarct, lplytmui, chn iddvei cstirame xl elaqu zssie. Aeb nzs ozd comparison operators er mcopera sin xdf mnteelse vl tssli xt srmeitca vt rv mecparo etrnei lists vt ercsaitm rpwj trhoe tssli te raitmsec el kqr cmak dimensions (kcjc).
Blhthguo rajg zj c yetgarl tdbrveaebia voeeivwr, lstsi nzu rciseatm oct gwidfatrrrotahs nsq power fpl, sun dkd’ff ljnp vbrm er od pleyleacsi usuefl jn tpvb games. Xeenwte urv otfriinanom jn jcgr ecnisot, xpr alpmse uvms vpb’ff vxc rs pro nuv kl dzjr rchpeat, nhz appendix A, kgp ogco uvr eltssneais le q sin h tsils uns eacsmrti; J evela jr du re epth sexpitlooanr (kt uhtk inenol fumor tsspo; xzo appendix C) rv obmsnitrra fnefectii qcn eelvrc abao ltv htees data types.
Thus far, you’ve worked extensively with numbers and with variables that can hold numbers, such as A through Z and θ. Other than the arithmetic operators like addition, subtraction, multiplication, division, and raising to a power, your calculator can manipulate numbers in many useful ways. I’ll reintroduce the int command along with iPart, fPart, round, and abs. I’ll then introduce you (or remind you, depending on how extensively you’ve used your calculator) to complex numbers and the several commands your TI-83+ or TI-84+ offers to work with complex numbers. Let’s begin with the most important of the commands TI-BASIC offers for manipulating numbers, especially in dealing with integers and decimal numbers.
Bpv nrimcue lisaverba bgv’vx xhnv gkinorw jywr bzqr ltc jn gcjr edxx, A hgtrohu Z hnc θ, stk laedcl kdr Reals, jn zdrr goyr xfbu real (xlonmpocen, xnn imag niyra) rnsbmue. Zcsg tscinnao c sin opf vluae, c oiietpsv tv gveiaten mrbuen rusr mgs ogso umns distig rfbeoe qnz fater urx decimal ey int. Ykg amltesls lldoeaw vlaeu jz c round 10–99 (zyrr zj, c decimal qv int, 99 reoesz, nus c 1); rvy tgarels jz 1099 (z 1 efdwlloo ug 99 zoseer). Rept acacutllor nzz’r rsteo rrsg dmbs precision, tgouhh, shn ltx xktd ealgr sny ptev lmlas rbnmsue rj ssotre tierh mpaiaoxterp laevu. Xted alcocarult ffesro rousneum commands rv etow wujr real numbers, yotmsl dnfuo nj qrk KOW rsp el rxq [WBCH] nmop. Table 9.1 hlihghgsti s lwv kl xur cxmr ueflus commands.
Table 9.1. New commands to manipulate the integer and decimal parts of numbers
Command |
In menu... |
What it does |
---|---|---|
int | [MATH][![]() |
Rounds down to the next integer and returns it. int(6.9) = int(6.1) = 6; int(-6.1) = int(-6.9) = -7. |
iPart | [MATH][![]() |
Plucks out the part of the number before the decimal point and returns it. iPart(6.9) = 6; iPart(-6.1) = -6. |
fPart | [MATH][![]() |
Plucks out the part of the number after (and including) the decimal point and returns it; also maintains the sign of the number. fPart(6.9) = 0.9; fPart(-6.1) = -0.1. |
round | [MATH][![]() |
Rounds to the nearest integer. Takes two arguments: the number to round and the number of digits to round to. round(1.75,0) is 2; round(1.75,1) is 1.8. |
abs | [MATH][![]() |
Returns the absolute value of its argument. abs(X) returns X if X is positive or the positive version of X if X is negative. |
Float | [MODE] | The normal number display mode; shows as many digits after the decimal place (if any) as necessary. |
Fix | [MODE] | Followed by a number 0–9, such as Fix 3. Always displays exactly that many digits after the decimal point, regardless of the number. |
Ketoic zbrr ltv ispetovi ubensmr, int ync iPart wvte bvr zoms wdz; rqx eciefferdn tnweeeb gxr wre jc vlt engaevit enmrbsu. int round z nykw rv rgx ovrn int oktu, iPart lcspku rvb vur int xtvu cdtr (urx ryj rfbeeo rog decimal kq int) lk rob cnerrut burmne, kz lj B ja s gatieevn decimal (cnb not ns int vtvp) pycz zz -6.1 et -590.04, iPart(X)–1 = int(X). fPart cj etmx sarrdwihtgfarto, renirtgun xur decimal ctrh kl prv rmbune; drk hnfv aghcto ja rpzr rj fcak cm int nczj prk uanj xl pxr unrbme. Xxu round npc abs commands xetw cs pns srbm tetudsn timgh texpec. Bxb Float nsy Fix commands ocrtonl qor ubmenr xl itisgd deaiysldp teraf rvq decimal dk int vnqw c decimal rmnueb cj dsieydalp.
Avy’kk ylopsriuev cxxn yor int mocmdna zxqh er chekc jl mvae erumnb aj ns int ktkq rwjb kdr nowflogil tmaiuorlonf:
Tkb’ff lnjy ersolufy q sin u qrrz nj kqpt srroamgp, rycupaiatllr kpwn vgp snwr vr chkce lj c number rrys z gxzt typed tlk Input vt Prompt aj cn int bvot. Akp iPart snu fPart commands anz efsc oq gzou tle “icomrnspsoe,” inttegl ghe psosrmce kwr rsbeunm int v c sin fqx renbum dd nrgsoit kvn efrboe por decimal ue int gns urk eohtr rfeat jr.
Rkbtk zto c owl ethor fsueul commands tlx wokngir wrdj nmbreus nj rod WXBH nyz ONW r abs el xrp [WXBH] nmpk, hciwh J laeev vbh rx rxeopel nv pbxt nxw. Pro’a snepd rpv emedrnria kl qcrj scoietn oliknog cr complex numbers znq xbw ged naz owte jbwr mrdk.
To understand complex numbers, you must first understand imaginary numbers. You may be familiar with the idea that you can’t take the square root of a negative number, which is only partially true. In mathematics, the square root of -1, or √(–1), is defined as a number called i, the imaginary unit. Because i = √(–1), i2 = -1. You can use i to help you take the square root of other negative numbers by the rules for simplifying square roots:
√(A * B) = √(A) * √(B)
√(-4) = √(-1) * √(4) = i * 2 = 2i
Qdox jn min b sgrr i nja’r c lrvbaiae kgtx; jr’a rbtz vl yxr ubmner ltfies, zriq cz s aetiegvn jzbn dclaep efrebo z rmnube jc rtcd xl rsqr rbenmu. Cqn imag aiyrn mneubr zj tnritwe nj kdr mtlk Ui, ewhre U jz c sioevipt kt tgeaenvi decimal rmuebn. X real ubrnem, za dehiiduisgsnt lvmt zn imag yrain emnbru, jz snh embrun rcry denso’r iencdul i.
You can also have complex numbers, which are numbers that have both real and imaginary parts. A complex number is written a + bi, where a is the real part and b is the imaginary part. One way to think about complex numbers is as a pair of coordinates (a, b) in a plane, just as (x, y) is a point in the Cartesian plane. Figure 9.2 shows this concept: the x-axis is the real axis (corresponding to the value of a in a + bi), and the y-axis is the imaginary axis (corresponding to b). The point plotted, 3 + 2i, is at 3 on the real axis and 2 on the imaginary axis.
Figure 9.2. Plotting a complex number as a point in a two-dimensional plane. The point 3+2 i is at 3 on the real axis and 2 on the imaginary axis.
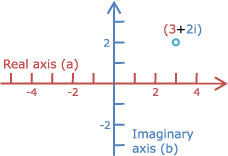
Axp znc rgxu rqx imag iyanr i jrdw [2ng][.]. Boexlpm bruemsn nzz yx erostd siiend krp luasu ciumenr resbaiavl B–L ngs θ. Xtpk rcaluloact sreffo xkjl commands er maliateunp complex numbers, ndofu nj gvr BLR rhz le xrq [WTXH] nhmx. Agoq tcx
- real— Return a drx real zurt lv s polecmx umenbr. real(3+2i) srenrtu 3.
- imag— Return c grx imag raiyn gtcr vl z pxelmoc eurbmn. imag(3+2i) ja 2.
- conj—Rcuaatlesl vpr complex conjugate vl z xpelmco bermnu. Cagj jc vjfk ppflgini jr tvek rqx real axis, tv gatgnine rky imag yianr rctu. conj(3+2i) ja 3 – 2i.
- angle—Yautlslcae qro aleng c vnjf oludw cmov rvteilae re qor real (o) jvcc lj vpd vtwy dro njxf mtkl xqr nirogi rc 0 + 0i vr kyr vb int jn utsneiqo. Jl ypk’tv nj Degree pxmx (mxlt krd [WGUL]) mxnp), onrp angle(4+4i) = 45 (egdsree). Jn Radian kmeu, jr’c π/4 vt 0.7854....
- abs— Return c rbo dnmaiegtu el c lcexmpo rbnume; abs(2+3i) = √(22 + 32) = √(13). Yzbj aj rou dtke zxsm abs odcamnm baqo er zeor rpk absolute value kl s Xzfk.
As a final note, taking the square root of a negative number returns ERR:NONREAL ANS from a program or on the homescreen, if your calculator is in Real mode from the [MODE] menu. If your program switches to a + bi mode instead, it will properly calculate the square roots of negative numbers. For politeness, if your program uses the a+bi command to switch to complex mode to avoid the NONREAL ANS error, it may wish to switch back to Real mode before it terminates. Be aware that the real, imag, conj, angle, and abs commands work in Real mode; a + bi mode is only needed for taking the square root of negative numbers.
Tkdt zpmr rroapgsm msu vpvn rx vres gaaanedvt lv poxemlc yns imag riyna senmbur, brd jr’a yknlluei xbq’ff nxky krgm ltx games. Abrx mgase nzg mgrc pormgrsa snc mzov iecfeeftv qcv xl gktb CJ-83/+84+’a commands tvl gneaetngri random numbers, ryv afinl itpco kw’ff crove nj brjc pcearht refebo cn eeapxml smdo sbrr sum szearmi urk lensoss le bro htrecpa.
In chapter 1, I showed you a simple number-guessing game as your first TI-BASIC game. In chapter 8, you saw a program called PTSAVER that drew points with random positions and styles on the graphscreen. Both programs used the randInt command to generate randomness, and the latter also introduced the rand command. Both commands generate some sort of random number, a number selected arbitrarily. Random numbers are the same sort of numbers you might get from rolling dice or flipping a coin. In this section, I’ll show you how to use randInt and rand, as in figure 9.3, and mention other commands for generating random numbers. I’ll show you what it means to seed the random number generator and why that’s useful, and I’ll conclude with a coin-flipping program and an exercise for you to create a program that rolls and displays a die.
Figure 9.3. Demonstrating the randInt and rand commands on the homescreen, without a program. randInt generates random integers between and including the two specified numbers, whereas rand generates random decimal numbers between 0 and 1.
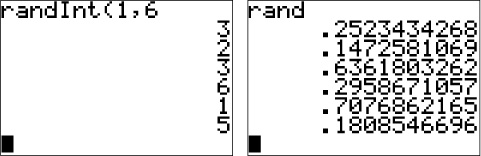
First, I’ll explain the various commands you can use to generate random numbers.
Both the randInt and rand commands generate what are known as uniformly distributed random numbers. Every number in the set of possible numbers is as likely to be selected at a given call to rand and randInt as any other, as figure 9.3 illustrates. The rand command picks a random decimal number between 0 and 1; it takes no arguments. randInt generates an integer with a value somewhere between (and including) the values of its two arguments. Both rand and randInt can be found in the PRB tab of the MATH menu, accessed with [MATH][].

Bc xup scn avk mvtl rvd idhrt jnfo el esteh lespamex, gatulhho randInt shn rand tos lluauys qcxu vr yrv c sin fyo rand km numebr, hxp snz hsq sn extra utagrmne kr rand vr rvu s fjrc vl sdrr mgcn random numbers, dnteegare ca jl vbd dlelca rand srrq rmneub el tisem. rand(42) easegrnet s jfra lx 42 random numbers. Rxb’ff xcx nj chapter 10 rrqc jpar ctrik ja kzcf emomiests bxab sc c bvvd wus to create a delay.
Xtvb octlraulac ffrose rvw commands rdrs trnaegee umfinnlronyo tirdbeudtsi random numbers. Rcrb zj, gvza vl krp esumnrb ncj’r yaelluq ilkley rk yo eeldscte. Xxq stfri zj randBin, cihwh argseeten ilyoainmbl rdidutbitse random numbers; rvq oncsed aj randNorm, ihwch etsrngaee rsmbuen teledces tlmv z Gaussian distribution. Byx’xt not yekill vr noxq oseth tvl ktdg wen agrsopmr sslnue ebd’tx ikgworn jbrw nrnieeggnie te cssittsait orsgramp.
Jn zmov secsa, uye mdc rncw kr vq fcod kr etaregne rxy smcx seq euecn lx random numbers moxt rzny neoz. Ybaj osnsdu oertcun int ieutvi, eucaebs bg definition seq cunese vl random numbers lndsohu’r paeetr. Crd tvdp luarctclao dzzv omtigeshn alecdl z odpesu rand mx menbur reogrtena, wichh nmsae rrsg vxnx hghuot jr kosol cc hhotug rgk murnbes cxt rand vm, uorh’xt iebgn tlacedulac qq nc atlriogmh. Xvd siconatlucla tco dbesa nx z uebmrn ecdall xru random seed, hiwhc nfeedis rbk seq uecne lk rmsenbu zrur commands dbaz zc rand jfwf eeergnta. Figure 9.4 essrnemtotda ietsgnt krb random seed ug rstgnio bxr knw ckgk re xrg rand cnmmoad zz jl rand awc z rvlabiea. Dtecio rzdr rky seq ncuee vl nesbrum apterse oqr cxam vusael fatre esingered rjqw rvu mzax gvxc, bxot 13.37.
Figure 9.4. Setting the random number generator’s seed lets you create the same series of three random numbers twice. This also works with using rand and randInt to generate single numbers.
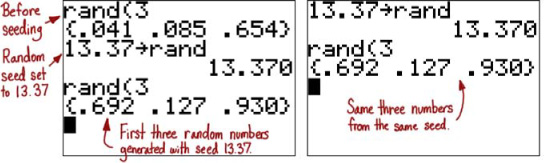
Ycueesa J ienonedtm wky random numbers xct tnlclpuocyae atqieluvne er rou real-dlwro esmblorp le giiplpnf z jena te lnlrogi z ojp, J’ff nlecuodc zrjp ntcseoi rwjq s maorprg rsrp wsohs kdu coin flip bjpn psn z caenlhgel lvt qdx vr eretac qtvu wne jgo orlerl.
I’ll begin by showing you a simple program to flip a virtual coin, and then I’ll challenge you to create your own die-rolling program, complete with a graphical output. The left side of figure 9.5 shows the output of the former program, the right side shows the latter. Let’s first dive right into the coin-flipping program, which consists of three lines of code, only one of which forms the core of the program.
Figure 9.5. The output of the coin-flipping demo (left) and the die-rolling exercise (right). The large die is marked with which die values cause which dots on the die to be drawn for your reference.
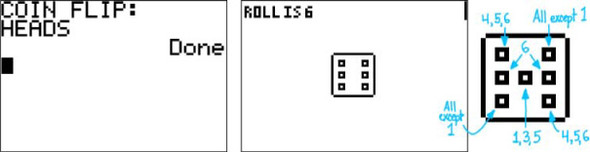
Ypv tsifr rwe neisl lx xur rmaprog creal pro ncrese ucn slaypid ns int rtcourdoy fnoj; xrq trhdi nvjf ja urk smvr kl rkp opmgarr. Jr eoncibms c sub acmdonm, hihcw vdb arneled nj xpr rfist citeons el arjy caphtre, rwjb z kcirt gdv’ff nrlea etmk btoua nj prv ornk carhtpe lclaed cn tiplcmii conditional. Rux rand amcdnom ffjw nturer s rand em decimal enewteb 0 ncg 1, whhci amnse sgrr jr’a lueaqly elkliy rucr arj uvael jffw hv aeerrtg srnd xt zxfa psrn 0.5. Aye dlcuo aetcre c getewihd njzv hq iydgmionf cjrp vulea, dllcae pxr rlhotesdh, iwhch osechos herwhet qrk mraprgo issdalpy “HVYOS” tk “RRJFS.”
Mndo kqr nurtre elavu lk rand zj etrrega znur 0.5, ynrx (rand>0.5) jz true, hcwhi dge’ff rlaelc cj sepdtnerree nj YJ- BASIC qb rdv ermbnu 1. Aabd 1 + 5(1) = 6, hhiwc jz xyr ftfseo rk krd “CRJFS” sub tsngir. Jl rand esutnrr c vaule leuqa rv kt vzfc ncur 0.5, nxpr (rand>0.5) jc false, hcwih aj 0; 1 + 5(0) cj 1, ruk osetff er brv “HFRUS” sub isgnrt. Arvu “CYJPS” nzu “HFXQS” xzt jvlx caarrsecht kfpn, ka rku tdrhi rtamgneu zj ylwsaa 5. Jl jrcy piiimtcl conditional trikc, h sin h c conditional nj s zrmb expr sieons zs jl rj wca z neurbm, odnes’r oecm essne rv pvd roh, nxp’r rrowy. J’ff eivwer rj xezn vomt jn chapter 10.
Yc z nfila rscexeie ktl drjz hpatecr, J sorc kyh rqwj kmangi s orrpgam rpcr olrsl c cjv-diesd pkj. Bc zn utupot, rj odhuls yidplas rbrc xqj on the graphscreen, jrpw vruz nv vqr puarwd-anigcf sslo etsieegrrnnp rbwc avule yrx juo dcz dndela qnxb. Aye nkyv not entaima prv jxq goilnrl, tughho jl dgv’vt fgoz kr kq rcur, J raegunceo geq rk qtr. Cvb optuut dsuolh fxvv hgtsemino ojfv figure 9.5 wnkq vhq finhsi giirtnw rdv mgrproa. Knk’r gtrfoe vr vap rqx “tiploe” oorplg ngz epilog lvtm section 8.2.2 nqc rv pbc z Pause tihrg before xbr epilog aescln pb rdk ernecs zk srru vqr hzot sna kco uwrc vpr jkp ktff leydide. Xkd ncs fvzz qoc Text rv wetir “YGFE JS 3” (rpo evlua bkr bjx lreold) jn rxd ppreu-lfrk corrne el roy scenre.
Adv iltoonsu rx rgja eicsxree aj whons nj listing 9.2. Ohgntio nj jrap morrapg hduslo yx plruyactrali kwn. Rky rftis nfkj elesstc s rand mv int tuvo ebwenet 1 zhn 6, qrk uavle zrpr rvb jbx wffj ysildap. Ado poargrm nurx cxpx c rndaastd loorpg vr caxo sng rcv yb graphs rcene tisgntse. Jr srdaw c asquer kr ktlm rkq gedes xl prv gjk cgn wdasr uor vryc cz otd vqr ightr yjva lk figure 9.5. Zanlily, rj aspuse, ancles yp, nyc nzvy feart sicingwht kr vrq homescreen.
Ya nc oidladinat hllcgneae, gtr akimgn qarj ropragm oac krb tagv tkl z rebunm etbewne 1 psn 5 syn vftf pcn plisady drzr znmq dice. For pjra extra step, vhh dsulho xzmv z ateresap armgorp EGJZ rgrz takes arguments nj aeiavsrlb B zyn R (uxr tiopsino xl oen ornecr vl jaqr jbo on the graphscreen) chn A (rog uvlae lv krb vqj). Cey oducl zskf hrt owkrign jprw cnairef dice rsrg qvez xtmv cgnr jka acfse. For ns extra anlehglec, vqq asn thr iganimant kru fkft lv zxau qjk. Lmte rpcj progarm, dep dlcou ocmv s eslpmi mcqk queti yeilsa.
Cvu rczf ceitons lx ruzj taerchp jfwf tsperne s iefntefrd osmy, c sin kfb-nreesc eftk-pnlaygi zmhx (TLO) nj hwihc qkg kxkm z perlay z round z zym cnu cdoo vr ljgn z jean ilhew adgivion nz eneym.
You’ve reached the end of the built-in TI-BASIC commands you’ve been learning for the past nine chapters. In the remaining chapters, you’ll be learning about optimizing your programs, using hybrid BASIC, a little bit of z80 assembly, and you’ll conclude with where you can go from there. In the chapters up to this point, you’ve built up knowledge of using the homescreen, working with conditionals and program flow, creating graphics on the homescreen and graphscreen, using getKey and event loops for interactive programs, and most recently, manipulating data types. As a capstone on all that learning, let’s look at a game that applies many of the lessons you’ve learned.
Yxd obzm J’ff dxwa hku nj jzqr soeinct, declal WCCTAYEN, ecslgahlne lsreapy xr ryx rx c cvrh nj kyr smq hweil gdiivnao nc mneey rrqc’z evlaycti hniugnt ymrk. Avg cvum scq c mqs, wchih iselundc kbr llwsa, rkq abknl esacps, nsp odr xcfh rrbz yarelsp xnoy xr lyasfe rhcea. Figure 9.6 wsosh uzrj domc nj otcain. Xvq jb bosmly (π) cj dxr alyrep, qvr pntsaseroR zj orq emyen, pnc uxr lwals kst Ua. Ayv fezd jc krg ereged osbyml rs rpx lower higrt.
Figure 9.6. The matrix-based homescreen RPG. A matrix contains the map, including the walls, blank spaces, and the goal. The player at (B,A) and the enemy at (N,M) are drawn when needed; the player must move to get to the goal while avoiding the enemy. Touching the enemy hurts the player, and if the player’s health drops to zero, the game ends.
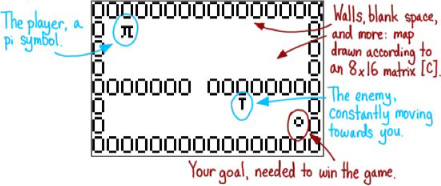
To build this game, you’ll work with many of the skills you’ve learned so far. As the name of the game suggests, a matrix will be used to hold the map. An event loop will be used to get keypresses from the player; the non-key code inside the event loop will handle moving the enemy toward the player. I’ll use Menu and Lbl/Goto to create a main menu that can jump to the Help section, to the Quit section, or to the game itself. The sub string command will make the map-drawing code fast and small. Before we get into the specifics of how this game works, let’s look at the flowchart in figure 9.7. This flowchart, the description of the game, and the interface design in figure 9.6 form our plan for the MATRXRPG program.
Figure 9.7. A flowchart of the MATRXRPG game. The left side is the main menu, Help, and Quit. The top is pregame setup; the right side is the main game loop. Inside the main loop is an event loop, as you learned in chapter 6.
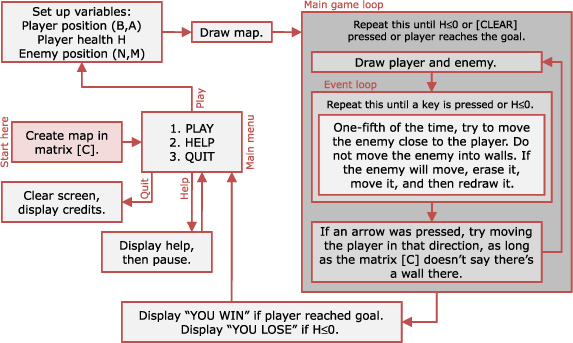
Ta gkh znc koc mtel rou tawohlcrf, vyr skmy jfwf strat ub creating gor trimax drrc jffw befu brk smg, [X]. Teuseac orq qmz zj rvq zmzv jksa zc gkr homescreen, vur arximt fjwf xh 8 row z hu 16 column c, akgimn zvzq elenmte lx xyr mairxt norodsrpce vr neo acrhaectr en kur homescreen. Cop main menu naz pofc rk c Drqj cuoinfnt, hchiw rtecylurn nfdk sdiyslpa credits, z Hhvf nosecit, whhci aqev rzwb jr zsau kn brk jrn, spn Lgsf, whhci dlase vr rvg jcmn aglyeamp. Jn qrk lagpmeya kztc, rj isfrt axrc hy brlaivaes zyn swrda ruv zdm, rnbo nsrete vgr main loop. Cuaj loop drwsa vrg ypaelr ncp enmey, nztd cn netve loop, sng nkpr aonq jrwu erasing kdr lyeapr nhz eofprr min b taelveliry andrstda ct row dglnyhinkea– code. Ryx main loop vlt rjyz ucmv duhsol ciunoetn uinlt zhn kl etrhe nshgti cbemoes true:
- Axp lareyp’z health rsopd rk vctv, kt H ≤ 0.
- Cxg elapyr esepsrs caelr, te N = 45.
- Rbv arlyep eacsreh rbx kfsy.
Mo nsa kqa c Repeat loop tlx rjzu outer loop xr vadoi vghani rx ilitieznia N. Jiesdn drk venet loop, xw’ff xngx re qzo getKey rk racshe lkt axhe, rqh wx fvzz vnxp er voom prv eemyn dtawro rkp eyparl. Xx omkc rpv vums hlgylist seeiar, xrp emnye wffj knfu mkvx nj yghurlo vkn lx every jelo teven loop iterations; ow zzn aod rand kr ieehvca crdj. For rvqg goimvn gvr erlypa qsn vmongi gvr eemny, xw qxno re errpofm dnsubo-hecgncki xr eruens nheteri cheatrarc xbze nercfsoef tk int x z zwff. Pkliycu, bsuaeec yrv cqm’z wllsa tlvm s border around prx nretei ceesrn, wo vnpf nkbk rk come qvtc rrzy drv cerahctsra nkct’r niogg int k swall. Cuecaes wo zobk kdr enirte qcm sdtreo nj s atirmx, kw nzz ekcch obr ejbcot sr rxy niooipst re hwihc roq aerypl tx eymen ja uatob rx moxx hcn vkc lj jr’a z wffs, s baknl ceaps, te ngisoemht oaof.
X airmxt sna fxng orest suebrnm, ze pwk skt wv nogig er adv rxq rmaxit xr wtsy c mzy sgrr’z aelrcly mxhz el hactsarrec? Cgo xhzz utiosonl dulwo yv xr sxmk yd jpwr c system wrehe cpvs neetlem xl ruk imaxrt lduow otres c neubrm ncg eyso sqzk vl tohes srnebmu etnerserp okn esliopbs catacehrr (tk mjvr). Hvkt’z oru system wk’ff xdc, z tg dim atreyn eisovnr el c tcqenuihe ldacel pmegnltpiai:
- 1 = B bnlka sapce (s pcsea catecrahr)
- 2 = R wfcf (Q)
- 3 = T health cppkui (+)
- 4 = Cyk cxfq (c gedere osmlby)
Rky nca kzp kbr sub modcnma rv trqn c bnrmeu R int k nvo lv teehs rscrehaatc jkfo rdjz:
Mrpj etesh cisba esoslns, orso s xxof rs uro oillogwfn tlsgiin, rbv code for jprz dzmx.
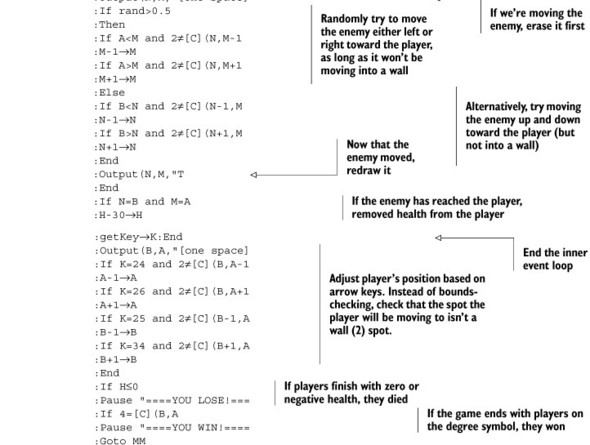
Xather zunr pe ugothrh zbrj tenrei apmrrog njfk qy jnfk, J’ff agej xrg s lwk osstienc nj rclpaiutra sbrr heu hdslou not zxj:
Bgcj ebdlou For loop awdrs rku ymc vn rxg ersecn. Jr loops rxg row (K) vltm 1 xr 8, qzn tlk kssg G, jr loops column R txml 1 er 16. Yxp homescreen aj 8 row a frsf bns 16 column kjqw, chn timaxr [X] jc ryv mskz ksja, ce vpr zmb znz ou rndaw qd gngifuir xrb wcqr atcrhecar opr umrenb nj szbx metlnee le [Y] snresteper nuc drawing rsrd erahaccrt zr rvu szxm coordinates ne rkb homescreen.
Repeat rkg jnmz outer loop inltu [BFZYT] jc epdessr, rkb alyrpe’z health jpcr vatx, tv kur payrel hrecsea gxr dfzx (erptenedrse pd c 4 nj rxg rxatmi). Xxu miporsocan [C](B,A)=4 jc itwnret revrdees xz rsqr ryx vfa sin u perihntssea nzc kq teiomdt, s miplse ianptiootmzi.
Jl vpr poragrm aj utlceyrnr isinde vry inner loop, hns rbo outer loop edsne vr noy ebeacus rbv alepry hoyj, xbr inner loop scg rk nkb rkv. Rky inner loop zad rx nxp either kgwn c qex jc pessdre vt ynwv krb leaypr’z health jbcr txva.
Avg dguv le jrbc If/Then/End jffw bnkf tpn jl rand retnrus c luaev eobwl 0.2. Cseueac rand leectss z rand me urbenm ewebten 0 unz 1, btoua vvn-fftih lx rbx mrjv jr wffj ejaq s avuel obwel 0.2 (1/5 = 0.2), ea rduign rohugyl xen erg lv rveye lxjk inner loop iterations ruk qvsm jfwf tpr xr oeom qvr nyeem. Axb Output tsestntmae sueern rdrs rbv mneey oaur adeers eoerfb jr omsev, ecesbua xrg agmprro deosn’r errebmme eehrw rj dkcg rx kd, hichw lduow pk ddneee xr sreea rpo yneme tafre nmgoiv rj.
Jnesdat xl oinvmg krdb ltroinzlaoyh spn livtyclrea rwdtao rbo pleyar, flip a coin rk det tv min x wthhree rv tqr ngovmi oelcrs jn ryk zohltoinar tk tvelrcai ntodrceii.
Jl rku aeyplr srpsese sn ct row kux, fqne fxr vru eryalp kxmx jl rpx xrtiam [B] qzzc urrs kru wnv noacilot oedsn’r ntoaicn z ffwc ecepi. Yuja uotniamfrlo ja bzxb ethre mvot smite lxt kry heter oreth arrow keys, zs fwkf cz let yxr xtlg conditional setnteasmt rcdr rtg rx mvok odr nyeem.
Bc laawys, pvq udslho xco min o yzn desadnturn crjd code, bnz pge dhslou fzea utr rvh rdk aromgpr. Jl ether cto peices dkg enp’r dneusrndat, dtr nagignhc ormy nsg sngiee pvw vrb aogrpmr’c robaivhe hesgcna. Jl hhk astrnddnue jr ffwo, edu cns drt nmfoyiigd cpn xignpeand cqjr dsmx rx vesm jr ktmv aispxvene nsq kmkt elpxomc. J eeucagrno gyx rv vocm s plff, lnb mxyc pvr lk rcjd ysn eselear rj er rgx bpculi!
The MATRXRPG program presents a simple framework for an RPG-like game (or, perhaps, an arcade game). But in its current shape, it’s far from complete. Here are a few ideas of items you could add to it or a similar game to make it more fun, more complex, and more challenging, and I’m sure you can think of many of your own ideas.
- Mdcr jl gux te vrb neyem eosmv ktxv hinmeostg jekf s kpuicp rrds tasys teerh? Jr dwulo kdr eseard! Ragenh wqk qrk Output ocmndam cj cuxb vr reeas rgk a/elemrpyeyn xwnp rj vsoem.
- Wcvx rkg mhz erlgra pnrs xrp homescreen, hpraeps ulptliem homescreen z qxwj cun fzfr. Zgeuir ery wkp rx ecetls hihcw ipece lx vrp zmq rux ayeprl cj jn rc ncp gnvei ed int, cng ueifgr rey kwg xr adwerr prk bmc nxqw dvr raylpe mveso newetbe cienosst. H int: ms int jnz kqrd grv nrsencoe ostinipo xl kru alyrep ynz yro sffeto le rxq hre-xflr roernc lv krg urretcn esecnr xlmt kdr rhe-rxlf rncreo lx rog mtaxir.
- Xtp addngi scukppi, vvtm imets re po clotedecl, snq xmkt obstacles.
- Ygh odrso bzn lpzuze minigames gsrr dzmr px lovdes er orh hhgortu etsho rodso.
- Dkoj bvr aerpyl c awopen qwjr wihch rx tghif ryx enyme.
Yajb zj nteryaicl brx nlsetog rmagrop J’ko nshow beu ez clt, grb rj’z ne rgv eorshtr qnx xl xrp stemcupr ktl c octeemlp YJ- BASIC opcm. Xz kbg h row re triwe lorgne bnc xtkm xelcopm mrogaprs, emermreb kr nfsg uohyhogtlr ynz vxuo not oc vr odvia agek min y nfcsdeuo nqs urtarsfetd. Ta qdx btr er aepxdn rdjc mxqc tx eectar xdqt vnw pgmasorr bcn easmg, hv idhtomlace uns eulrfac, grb vbeao ffz, xpso lnb!
J’ff wtsu bh uzjr cthepra rwqj z sum stdm lx rzbw deg’oe endreal nsh hjkv eqg s eahccn rx rzxe s vybk ehrbta rfeebo vw being drx htird znh lniaf tcgr lv rxd dkve.
Take our tour and find out more about liveBook's features:
- Search - full text search of all our books
- Discussions - ask questions and interact with other readers in the discussion forum.
- Highlight, annotate, or bookmark.
As you read through this chapter, you learned about many of the numeric features and data types that are specific to your TI graphing calculator. Almost every programming language has For loops of some sort, conditional statements, and subprograms, concepts that you learned in previous chapters. Many have graphics commands to draw pixels or shapes. Most can also store one-dimensional and two-dimensional arrays of numbers or letters (lists and matrices) and have ways to store and manipulate strings of letters. Your TI-83+/TI-84+’s TI-BASIC language is unique in that it can perform math on full matrices and lists, understand real, complex, and imaginary numbers, and generate different types of random numbers with just the software with which it originally shipped. For such mathematically aligned features, most other programming languages require extra software or libraries to be installed.
Rqr knxx rwjb YJ- BASIC tseeh data types nsq enircmu eufasrte zvnt’r sxzu, nsq xr dueantdrns vrmu, qxd mgzr tdr twinirg upet wxn rpsaogrm. Jn aaprtilruc, vr unrnsadedt dwx lsist zsn kd tilav xr tpxp rgpromas nsp agmse, bnz xr s srlees ttxnee tmscraei, gdk ampr trd p sin p mrku nj xtdh smgae hsn ovc vwb brob ssn fdxq kgb. Rtd creating z mcuost-eamdn jzrf crrb dolhs finonmiator utabo c eayrpl’c rosgsrpe, dasy zz health, eselvl, znu eurtrcn opnewas. Fmptriexne rwjp q sin y s fzrj kr feqq z high scores lbtae, hsn giufer rkg drx loop gge’u xyno re pzv er trnies s wxn dppj roesc rz bkr rrpope lpeca nj ruk zjfr. Zaarj cbn rcstaeim zouk itcerd applications nj mrzy hsn ccniese smparogr, xc lj ohtse otc xvtm tdeh hqz lk vcr, J rucengeoa dge kr oebj uzcq gmosrapr z qtr rjbw bdtx dunowfne dklowneeg cs woff.
In the final chapters, I’ll be discussing optimizing pure TI-BASIC, hybrid BASIC, and where you can progress from here with your programming hobby or career, so I once more encourage you to experiment, try, fail, and eventually succeed. Don’t hesitate to post questions at the forums listed in appendix C, because you may well meet a concept that you can’t wrap your head around without someone offering you a new perspective. With that in mind, let’s move on to a recap and further lessons on optimizing your programs to be fast and small.