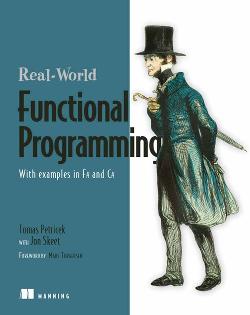
About this Book
If you are an existing .NET developer who knows object-oriented technologies and are wondering what this new “functional programming” movement is about and how you can benefit from it, this book is definitely for you.
It’s particularly tailored for .NET developers with working knowledge of object-oriented programming and C# 2.0. Of course, you don’t need to know either of these for functional programming in general, or even F# in particular. In fact, it’s more difficult to learn functional programming if you’re already used to thinking in an object-oriented manner, because many of the functional ideas will appear unfamiliar to you. We’ve written the book with this concern in mind, so we’ll often refer to your intuition and use comparisons between OOP and functional programming to explain particular topics.
If you’re an existing object-oriented programmer using other languages and tools (for example, Java, Python, or Ruby) with the ability to understand languages quickly, you can also benefit from this book. We’re using examples in C#, but many of them would look similar in other object-oriented languages. The C# 3.0 features that aren’t available in other languages are briefly explained, so you don’t have to worry about getting lost.
This book doesn’t focus on academic aspects of functional programming, but if you’re a computer science student taking a course on the topic, you can read this book to learn about the real-world uses of functional concepts.