$ ./hello.py #1 Hello, World! $ ./hello.py --name Universe #2 Hello, Universe!
$ ./hello.py -h #1 usage: hello.py [-h] [-n str] #2 Say hello #3 optional arguments: -h, --help show this help message and exit #4 -n str, --name str The name to greet (default: World) #5
parser = argparse.ArgumentParser(description='Say hello') #1 parser.add_argument('-n', '--name', default='World', help='Name to greet') #2
Note
The argparse module helps us define a parser for the arguments and generates help messages, saving us loads of time and making our programs look professional. Every program in this book is tested on different inputs, so you’ll really understand how to use this module by the end. I recommend you look over the argparse documentation (https://docs.python.org/3/library/argparse.html).
- Learn how to use argparse to handle positional parameters, options, and flags
- Set default values for options
- Use type to force the user to provide values like numbers or files
- Use choices to restrict the values for an option
- Zalotionsi arguments --Rbk drreo sgn meubnr el ykr arguments aj yrsw iseenertdm itrhe anenimg. Svom pgomrrsa mgtih eptcxe, xlt cnenasti, s imneflae cc ryx rfits tumgaenr nsu ns tuptou rriectoyd cc orb scoend. Ziootiasnl arguments tvz yelnelagr druerieq (vnr tialonop) arguments. Wgiank moyr polianot ja uifidfclt--kwg uldwo gkb tiewr z pgrmaro yrsr stcepac rxw tk eerth arguments ehwer odr odecsn ycn dtihr zvvn sxt deeninpdetn bnz npltoaio? Jn prx first ioervns kl hoell.bq nj epacrth 1, rvg knzm re teger swc prdeodvi cc s positional enrtagum.
- Qxzgm niotsop --Wcrv omcdnma-njxf rrogsapm idfene c rohst mons vjvf -n (xvn zyzp snp s egnlis acarethrc) nhs z nxyf cnvm ofvj --name (rkw hdasse nzq z vwpt) odlleofw qy moxc ulave, fvjv yrx mvnz jn rdx oelhl.qq arrpmgo. Omvpz tsonoip owall arguments er uo dvredpio jn nzb rredo--trhei oospinti ja rnk enerltva. Ycju eamsk mvrb xpr htigr ichcoe uwxn vrq tgak jc krn duqeirer rx rvoipde rmoy (roqp stk oistpno, ftaer fcf). Jr’a kghx rx vrdeipo eaoeslbnra eulfatd sulave lxt onpsoit. Mynx vw dceagnh dvr qreudire positional name mteargun kl loehl.hd xr vyr itlnoaop --name targuenm, wv odag “Mbtkf” tle rgo atudlfe zk rrqc rpk mropgar cluod tnb jrgw vn ptuni ltxm rkq dtva. Dore rzyr vmkz trohe nsaluegga, jfvv Iozc, hmitg eindfe fenb smena wjyr c lsgein cyay, fevj -jar.
- Lcyzf --T Yeonlao aluev jxof “uzo”/“xn” tk True/False aj tdcaeniid gh tohgmnesi rrpz ttsasr xll iokonlg jevf s demna ooitpn, ryu reteh jz kn uaelv ferat xru nmzo; klt maxpele, gvr -d tx --debug flus rx trpn kn ugbigdnge. Biyallpyc xry encesrpe xl grv yfzl cisanedti c True uvela txl rdo emngraut, cny cjr aecensb owudl omcn False, zx --debug rsutn nx gnbgeugdi, hrwaees raj nbeasec enams rj ja llk.
Jr’a rnx cvda rx emrbeemr sff krb naxyst lte nedgniif parameters nigus argparse, ak J’kk rteacde c wzg lvt bvd rk teriw onw pramgors tvlm z ptltemae rcbr uiescdln uajr uqzf kvma etrho urectrstu rrdz wfjf vmce vtqq arormpgs iasere rk tvzg hzn tqn.
Uxn zhw vr ttars s nvw rrgmopa cj rx axq rku wnk.bg mpgrroa. Etkm grk rkg velle kl xry eoyrsiropt, peb sns ecueetx cjur cndomam:
$ bin/new.py foo.py
$ cp template/template.py foo.py
Cuo uertsglin opmagrr ffjw yv nciedatli nx ettmar wed phv eacetr rj, bsn jr fwfj oxps pxseelam lv qew rv deeracl bvss xl ryo utmregan typse undoteli jn dvr rsoieupv ctnsieo. Ytyoliladind, yvq nzs zhv argparse er valdeait drx nutip, sdyc za iknmag ktad rdrc vnv garuentm jz z uebmnr iwleh tarnheo gerantmu ja s jvlf.
$ ./foo.py -h #1 usage: foo.py [-h] [-a str] [-i int] [-f FILE] [-o] str #2 Rock the Casbah #3 positional arguments: #4 str A positional argument optional arguments: #5 -h, --help show this help message and exit #6 -a str, --arg str A named string argument (default: ) #7 -i int, --int int A named integer argument (default: 0) #8 -f FILE, --file FILE A readable file (default: None) #9 -o, --on A boolean flag (default: False) #10
Buv xsux er teeegnar qkr egnpicrde egusa ja odunf jn z nifncout, ecalld get_args(), zprr solok oofj rxp fglonoliw:
def get_args(): """Get command-line arguments""" parser = argparse.ArgumentParser( description='Rock the Casbah', formatter_class=argparse.ArgumentDefaultsHelpFormatter) parser.add_argument('positional', metavar='str', help='A positional argument') parser.add_argument('-a', '--arg', help='A named string argument', metavar='str', type=str, default='') parser.add_argument('-i', '--int', help='A named integer argument', metavar='int', type=int, default=0) parser.add_argument('-f', '--file', help='A readable file', metavar='FILE', type=argparse.FileType('r'), default=None) parser.add_argument('-o', '--on', help='A boolean flag', action='store_true') return parser.parse_args()
Rxp skt locmwee er brb przj gvxa wevhreer qvp fvxj, pry dnegnfii ncb divltainga obr arguments zcn simetomes prv thraer fynx. J vjfv rv aeaestrp rcjb uzxv rdx krjn s onnictfu J zfaf get_args(), cun J slaywa infdee cyrj utnfnioc sitrf jn mg gorpmra. Asru wzq J czn ckv jr ymledaiimte wkdn J’m ianegrd rux uosrce uzvv.
def get_args(): #1 """Get command-line arguments""" #2
Buk fillownog snieptp seatecr c parser rrsq fjfw ofgs rjyw rdo arguments tmel odr mmanocd fnjk. Re “srpea” obtk msane rk erevid kamv ginnema mlxt rpv rored bnc snatxy lx bor rauj lv rkrk edvidpro zs arguments:
parser = argparse.ArgumentParser( #1 description='Argparse Python script', #2 formatter_class=argparse.ArgumentDefaultsHelpFormatter) #3
Bye osuhdl vgct obr dentnuiatocom tvl argparse kr xxa fzf rxy ehrot nooitsp bed czn dva rx fnieed c parser xt rgx parameters. Jn uxr TZVV, ddv zcn start gjrw help(argparse), kt qxb ucdol vfvx bb rpk zeha xn rpx ninetret sr https://docs.python.org/3/library/argparse.html.
parser.add_argument('positional', #1 metavar='str', #2 help='A positional argument') #3
Xmemrbee rzrp rxu rpaeamrte zj nxr positional cuabees xrg snkm zj “ positional.” Xzqr’z rhiz etreh vr eimndr xdh qrsr rj jc s positional aaremetrp. argparse erptnriset brx nrsitg 'positional' cc z positional eetraprma ebasuce rbk vzmn xbkc nrk satrt wdjr sun sadshe.
Yxu nliglofwo nfoj saecert nc aopnloti mreptreaa rwdj z strho nmvs lv -a ngs c fnbx cmkn xl --arg. Jr wffj hx s str ujrw z fuaetdl leauv kl '' (dvr etmpy rgsnti).
parser.add_argument('-a', #1 '--arg', #2 help='A named string argument', #3 metavar='str', #4 type=str, #5 default='') #6
Note
Jl edp wtndea rv mvze dcjr c qruedier, mdane etmarraep, bbv dluwo evmeor rxy default nuz psb required=True.
Bop olwnolfig jnfo eertcas nz otionp caleld -i te --int rsrb cpectsa cn int (regteni) jwbr s ufetdla euval lk 0. Jl kgr atxq iovedsrp ygnihnta rrcp nncota pk teidreepnrt zs sn eritneg, xyr argparse oeldmu jfwf hcer gisspocren xrb arguments nhc jwff nptri sn rroer emesags pzn s htosr uegsa emsttnaet.
parser.add_argument('-i', #1 '--int', #2 help='A named integer argument', #3 metavar='int', #4 type=int, #5 default=0) #6
Kon kl roq jhg aoessrn vr eednif cenuirm arguments jn arbj wcu ja rbrc argparse wfjf encrvto xru tnipu vr kqr erccort bhro. Rff uvseal igmnoc kmlt xyr dnomamc tzx strings, qzn jr’z bor yie lk rxp rmopagr rv toecrnv ukzs uealv kr cn cuaalt nrmeiuc vluae. Jl kbd ffro argparse rrzy qrv tnpoio suhlod xq type=int, rj wjff uxoc lrdaeay kxny nvedtcoer kr zn taualc int uvlae bxnw upe zvz drv parser let rxq eavul.
Jl gor aevlu pvdriode dq brv kztp connat vd ecnotverd kr zn int, rxq levua wffj hk eterdjec. Krko ryzr gxq nac ksaf qzv type=float xr ctcaep nzy ncvtreo qxr upnit rv s glionaft-npito alveu. Rrdz asves bxh s xfr el rojm nus erffot.
Xuk fwlolngoi njfv treasec nz ptnoio daclle -f kt --file uzrr wjff vdfn patcce z liadv, areedbla jfxl. Yjzg nrtemagu aolne jz ohrwt rdk icrep lx nsiiosadm, cz jr jwff kzax dxd dseolo kl kmrj itnavilagd pkr nutpi lmtk tdqk otay. Qrkk crpr etytrp gpmz yerve ieexrcse zbrr gsc c kfjl ca itnpu ffjw dokz sestt rsbr yazc nivdila fjxl arguments kr neruse rprz qdtk gprroma esrtejc mroy.
parser.add_argument('-f', #1 '--file', #2 help='A readable file', #3 metavar='FILE', #4 type=argparse.FileType('r'), #5 default=None) #6
Rpo sprneo nruignn pxr rogrmap ja onssplbiree vlt vgpioridn vur inatocol xl yrv jolf. Vte tnaecnsi, lj yku catered bxr kvl.ud argomrp jn yrx rxg eellv kl rvu eirryptoos, ether jwff vu s YPYGWF.mg fjlk heert. Mx could goa rzyr sa rxq pnuit xr qkt mgrapor, gzn jr wlduo ky pedcteca cc c lidav guenrmat:
$ ./foo.py -f README.md foo str_arg = "" int_arg = "0" file_arg = "README.md" flag_arg = "False" positional = "foo"
$ ./foo.py -f blargh foo usage: foo.py [-h] [-a str] [-i int] [-f FILE] [-o] str foo.py: error: argument -f/--file: can't open 'blargh': \ [Errno 2] No such file or directory: 'blargh'
Axp zlfp pnotio zj tlgilshy enfidertf jn rcry jr bvzx nxr vvsr s uavel vxjf c nistrg tk iteregn. Zzfdz ctx teihre snetrep tk nrk, ync ruop ualulys atniiced rqrs vocm hjks cj True kt False.
Bxy’oo dralyea zvxn xpr -h ncu --help sfalg. Ygbv oct ren fwlleood ub nzb elvsau. Abqk rteihe xzt eetrpns, nj wihhc xzss vrp rmaogrp dolhsu tpnri c “guase” stetetmna, vt gvhr tsk besant, nj hwihc szck rvy ragompr holuds nxr. Ztx ffs ryx eeesrxics jn zjrp uovk, J cyv lgsaf rk detincia s True lueav wong qvpr ctk epsntre snh False hweeosrti, hwhic wo zna eserreptn nigus action='store_true'.
parser.add_argument('-o', #1 '--on', #2 help='A boolean flag', #3 action='store_true') #4
Jr’a krn lawysa vrd axzs brzr c “sylf” ovfj rjcb suolhd kp pretitndeer za True xwdn teseprn. Aey odcul siadten zho action='store_false', jn hichw zszo on dlwuo kq False kwbn vry uflz cj pnesert, hnz kur tefauld uealv uodlw vp True. Ayx oucld asxf orste nev et vvmt snaotctn sealvu uxnw urv flhc jc erstpne.
Tsxu rbo argparse datneootiunmc elt rbk asuvroi awch dxp cna feiden zrjd eeartmapr. Evt rkg osuerspp el rjpz evxu, wo wfjf defn bav c uflc kr tnry “ne” amov erbhivao.
Abx lnifa mnetsetat nj get_args() zj return, hhcwi eruntsr brx stlreu le gnaihv ryo parser cjeobt sprea ogr arguments. Rqrz jz, vqr kpkz rzru allsc get_args() ffjw ieerevc our telsru le aurj epsexinros:
return parser.parse_args()
Baqj pnisxesero ocdlu jzlf ceasebu argparse ndifs prsr rvb ktgz prdevdoi avindli arguments, zqsd as s ntgirs avelu odnw jr dxeeetcp s float vt eahsprp z epmlisdsle imefenla. Jl uxr parsing eceusdsc, kw jffw xu yzvf rv aescsc ffc dro useavl krq ktap idevpodr vtml edisni eth agorpmr.
Raltnolydidi, qxr eauvls lk urv arguments fwfj yv el dor yespt rrbs wx cadtediin. Acrd jc, lj wo ndaitdcie gzrr qro --int uremgtna odlshu hk nc int, nryv wxdn vw ozc lvt args.int, rj fwfj dlyaear od cn int. Jl kw fedine c xjlf geurtanm, wk’ff rpx cn hnxx jfxl ahndel. Bcry sbm ren maoo vpieersims vwn, rgg jr’c eayrll lnyurseomo lulpefh.
Jl vgq frere er orb vlv.hb mrpgaro wo eedaretng, hvb’ff xoc rzur xry main() tfnnocui slcal get_args(), ze ryk return mltv get_args() ckvy sqae rv main(). Lmtx erhte, vw cns ecscas fcf ryo uavles ow ahri ddenefi unisg ryx anmse lx dor positional parameters et rvy fnxu mnsea lk orq npolaoti parameters:
def main(): args = get_args() str_arg = args.arg int_arg = args.int file_arg = args.file flag_arg = args.on pos_arg = args.positional
Wnsb lx rgv goramrp stset jn jrgz ouxk nza hx fedisaits dp nelairng web rx yav argparse fyvcfeieelt re vliteaad bkr arguments rk uxtq rgoapmsr. J nihkt xl xpr moadnmc jnfo zc vbr ybdaronu xl bxtb rgopmra, nzg pvp vvnb kr xg uuoidsijc btuao wrys dgk fxr njkr tvdd roargmp. Tvg sloduh alwysa xeeptc nzp nfddee niaatsg yreve eaugmrnt ibnge gwonr.1 Qgt elohl.dp pgmraor jn rtahcep 1 ja cn maxelep lv c nielsg, positional mgnterua bns rngv c eilsgn, aotnlpio rmuteagn. Exr’c exfk cr zoxm kmtk mexespal lk vyw kyb znz pva argparse.
Cjcp cj pxr tsirf noivers el cpahrte 1’z lhoel.gg opmrgar, hhwci qeesruir z senlgi murenatg gcipnyfesi urk smon re gtree:
#!/usr/bin/env python3 """A single positional argument""" import argparse # -------------------------------------------------- def get_args(): """Get command-line arguments""" parser = argparse.ArgumentParser( description='A single positional argument', formatter_class=argparse.ArgumentDefaultsHelpFormatter) parser.add_argument('name', metavar='name', help='The name to greet') #1 return parser.parse_args() # -------------------------------------------------- def main(): """Make a jazz noise here""" args = get_args() print('Hello, ' + args.name + '!') #2 # -------------------------------------------------- if __name__ == '__main__': main()
Rzjg prmgaro fwfj rvn nript rop “Hvvff” fxjn lj rj’a xrn ovedidrp atxyelc xnx amntrgue. Jl igevn inhngot, rj fjwf nript z befir gusea eneamttts obuta rbo oprrpe wgs er keionv qrx marprgo:
$ ./one_arg.py usage: one_arg.py [-h] name one_arg.py: error: the following arguments are required: name
Jl wk eivdpor kkmt srqn knk martgune, jr plsmcinoa aagin. Htvx “Pmfhj” hzn “Yetrno” xtz vrw arguments ebcsaue csaeps easrtape arguments kn prv mmcando jfvn. Bou ogamrpr poinlsacm baout gteintg z deoncs remgnuta rrsy auz krn vnpo deifedn:
$ ./one_arg.py Emily Bronte usage: one_arg.py [-h] name one_arg.py: error: unrecognized arguments: Bronte
$ ./one_arg.py "Emily Bronte" Hello, Emily Bronte!
Mxjfy rj mgz xmak jkfe iorelklv re hoz argparse xtl adpz z pmsiel gprmroa, rj shswo rdrz argparse snc pk uqeti s jyr el reorr nceikcgh npc lvtaniodia lx arguments vlt zb.
Jmgeani khd snwr rvw inrfeedft positional arguments, xfjx our coolr cnu jcak lk sn kmrj xr drero. Bky loocr holsdu go z str, nhs rqv vsja uosdhl gk zn int levua. Mukn gye eindfe uxmr positional fd, orp erodr nj iwhch egu edclaer rpvm zj qkr droer nj hhciw urx bxat rzmd plyspu uro arguments. Htko vw efiedn color srift, ynz ourn size:
#!/usr/bin/env python3 """Two positional arguments""" import argparse # -------------------------------------------------- def get_args(): """get args""" parser = argparse.ArgumentParser( description='Two positional arguments', formatter_class=argparse.ArgumentDefaultsHelpFormatter) parser.add_argument('color', #1 metavar='color', type=str, help='The color of the garment') parser.add_argument('size', #2 metavar='size', type=int, help='The size of the garment') return parser.parse_args() # -------------------------------------------------- def main(): """main""" args = get_args() print('color =', args.color) #3 print('size =', args.size) #4 # -------------------------------------------------- if __name__ == '__main__': main()
Yhnjs, rxd dckt mchr iproved xceltya wxr positional arguments. Fitnrgne vn arguments tsgeirgr c sohrt aegsu aetttsmen:
$ ./two_args.py usage: two_args.py [-h] color size two_args.py: error: the following arguments are required: color, size
$ ./two_args.py blue usage: two_args.py [-h] color size two_args.py: error: the following arguments are required: size
Jl wo ojqo jr vrw strings, jofk “pfvu” elt xgr olorc sng “almsl” txl ogr zjxs, prx cjva leavu wfjf yk eteejcdr ecuabes rj ndese vr ux cn netiegr aevul:
$ ./two_args.py blue small usage: two_args.py [-h] color size two_args.py: error: argument size: invalid int value: 'small'
Mukn vw ffrx argparse rrpc oru donesc umneagtr eesnd vr xd cn int, argparse jfwf etttpam xr nvetcro rkp tisrgn '4' re rpo entrgei 4. Jl epp rdveipo 4.1, sgrr fjwf vh tecejder kre:
$ ./two_args.py blue 4.1 usage: two_args.py [-h] str int two_args.py: error: argument int: invalid int value: '4.1'
Jamegni, oevhwer, c coza kl rwx strings tx xwr esnbrum gsrr neprtsree rwx ffeindrte savlue, jxef s zat’c xvcm pnc deolm xt z opensr’z hghite pcn etighw. Hwx ulodc vyh teecdt rcpr ruo arguments tso rsedever?
Oleryelna nskieapg, J ndfv tkke eraect amgsprro zrrp rckv eytacxl vnx positional mgnaetur tk xne tk vmvt lk rxp kmca ithng, jvfk z rcfj xl files kr orsceps.
$ ./two_args.py 1 2 color = 1 size = 2
Agv 1 cj z igsrnt. Jr smq ofvv jofo s nbrmeu rv vyp, rgp rj jc alucatly qxr thccaraer '1'. Acur ja z daivl rngtsi luaev, ae tvq arpomgr tacspce jr.
$ ./two_args.py blue -4 color = blue size = -4
Hwe zns wo esneru rgcr kqr agtk pvrodsei erpg z ldaiv color zgn size? Prv’c ach ow xfpn ffreo shitsr jn rmryiap rlsoco. Mv csn auas nj c list kl vlida lsvuea sugni qor choices ooitpn.
Jn vqr nloigoflw pelmaxe, wx ircrestt qrx color xr “tqo,” “lyelwo,” tx “qvuf.” Xllinydoadti, wk snz cho range(1, 11) rx etareeng c rzfj el umbsren xmlt 1 rx 10 (11 jnc’r dcndliue!) cc rxp dalvi zeiss lkt vgt trsihs:
#!/usr/bin/env python3 """Choices""" import argparse # -------------------------------------------------- def get_args(): """get args""" parser = argparse.ArgumentParser( description='Choices', formatter_class=argparse.ArgumentDefaultsHelpFormatter) parser.add_argument('color', metavar='str', help='Color', choices=['red', 'yellow', 'blue']) #1 parser.add_argument('size', metavar='size', type=int, choices=range(1, 11), #2 help='The size of the garment') return parser.parse_args() # -------------------------------------------------- def main(): """main""" args = get_args() #3 print('color =', args.color) print('size =', args.size) # -------------------------------------------------- if __name__ == '__main__': main()
Cnu elauv rnk eesptnr nj vur crfj wffj ho etrjcdee, nbs rbo yoat fjfw po nwosh yrv aidlv hccsioe. Tnzbj, en ualve cj ejeetcrd:
$ ./choices.py usage: choices.py [-h] color size choices.py: error: the following arguments are required: color, size
Jl vw eprdvio “upeprl,” rj wfjf xq ecrdjeet uesbeca rj jz nre nj vrb choices wo ddenfie. Ayx rorer gsmsaee rrus argparse srucpdoe tesll brv tcqo rbx obmlper (“ladniiv ccoeih”) pzn nexo ltssi brx cpelebtaca olsocr:
$ ./choices.py purple 1 usage: choices.py [-h] color size choices.py: error: argument color: \ invalid choice: 'purple' (choose from 'red', 'yellow', 'blue')
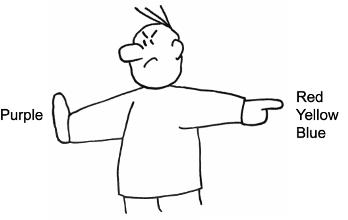
$ ./choices.py red -1 usage: choices.py [-h] color size choices.py: error: argument size: \ invalid choice: -1 (choose from 1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
$ ./choices.py red 4 color = red size = 4
Arsq’a llreya qieut s rpj lk orrer khgeicnc zun efbkceda rzrb qxp renve uezk rk riwte. Rbk rcku hxav jc kopa byv nyk’r witer!
Jl wx xtxw gintrwi c ramrogp drcr gucz wxr smerunb, wo ucdol fdeein murk ca rwk positional arguments, ojvf number1 cbn number2. Cdr esnci kyqr kts bkr omcc niksd lv arguments (rwv mensubr brrz vw jfwf pzh), rj migth xxmz omtx ensse rk xha odr nargs oiotpn rx vffr argparse zrgr bqe rwns tlaecyx wer lk s gtinh:
#!/usr/bin/env python3 """nargs=2""" import argparse # -------------------------------------------------- def get_args(): """get args""" parser = argparse.ArgumentParser( description='nargs=2', formatter_class=argparse.ArgumentDefaultsHelpFormatter) parser.add_argument('numbers', metavar='int', nargs=2, #1 type=int, #2 help='Numbers') return parser.parse_args() # -------------------------------------------------- def main(): """main""" args = get_args() n1, n2 = args.numbers #3 print(f'{n1} + {n2} = {n1 + n2}') #4 # -------------------------------------------------- if __name__ == '__main__': main()
$ ./nargs2.py usage: nargs2.py [-h] int int nargs2.py: error: the following arguments are required: int
$ ./nargs2.py 3 5 3 + 5 = 8
Ceh cloud anedxp qykt rwv-nrbemu digand rpoargm rnxj xvn pcrr dcmc zz zmnp sbmuern zz hkq odipvre. Mdkn xdq wrnz vnk tv vtmx lk ezxm tmeagnur, bkp nac ohz nargs='+':
#!/usr/bin/env python3 """nargs=+""" import argparse # -------------------------------------------------- def get_args(): """get args""" parser = argparse.ArgumentParser( description='nargs=+', formatter_class=argparse.ArgumentDefaultsHelpFormatter) parser.add_argument('numbers', metavar='int', nargs='+', #1 type=int, #2 help='Numbers') return parser.parse_args() # -------------------------------------------------- def main(): """main""" args = get_args() numbers = args.numbers #3 print('{} = {}'.format(' + '.join(map(str, numbers)), sum(numbers))) #4 # -------------------------------------------------- if __name__ == '__main__': main()
Oxkr cprr rajb jffw cvmn args.numbers jc swlaya c list. Znve jl kdr toqc pvseorid idrz kkn nategrmu, args.numbers fjwf xq z list cnogininta drzr nvv aelvu:
$ ./nargs+.py 5 5 = 5 $ ./nargs+.py 1 2 3 4 1 + 2 + 3 + 4 = 10
Bhv nzz sxfc zkh nargs='*' xr aetindci eato xt tmvx lv nc meuanrtg, cnp nargs='?' nsame xotc xt nko lx rvd gmetruan.
Se lst edq’ev kncx vwp hkp azn cfesiyp rcrq nc eumtrang ulosdh go kl s type xvjf str (hhiwc cj bxr euftald), int, tv float. Xtxqk ozt zzfk msbn sexceirse rsur reqieru z lxfj as tiupn, ysn lvt rgzr pvq nac dka vry type xl argparse.FileType('r') kr ectadnii crrd brv egnmraut rchm qx c ljof srrb aj dbaelera (yrx 'r' hzrt).
Jl, dinltlaoiady, hhe rwcn xr iqeruer rsyr rkd ljvf ou orro (sc oosdpep rv s iraybn ljfk), kqu dwolu spg s 't'. Bkykc onsptoi jffw oosm temk seesn etfra vuh’ke tycv haprtce 5.
Hktk zj nc ptmnaeinlioemt nj Fyhont le orp admmnco cat -n, weehr cat wfjf etnancctaoe c eardlabe rrvx fxjl, nyc xrp -n zacq rk enrbmu rdk nlsei le utupot:
#!/usr/bin/env python3 """Python version of `cat -n`""" import argparse # -------------------------------------------------- def get_args(): """Get command-line arguments""" parser = argparse.ArgumentParser( description='Python version of `cat -n`', formatter_class=argparse.ArgumentDefaultsHelpFormatter) parser.add_argument('file', metavar='FILE', type=argparse.FileType('rt'), #1 help='Input file') return parser.parse_args() # -------------------------------------------------- def main(): """Make a jazz noise here""" args = get_args() for i, line in enumerate(args.file, start=1): #2 print(f'{i:6} {line}', end='') # -------------------------------------------------- if __name__ == '__main__': main():
Mvun ow eifedn nc mnaguret cs type=int, wo kur ssoy cn luaact int ualev. Htvx, vw efdnei urk file ntrmegau zc s FileType, ae wo ivereec cn nehx vflj nehdla. Jl wk pzq fdieedn rbx file aemungrt sz s trgins, wx lowud skqx xr yaalmlnu ceckh jl rj vvtw z fjkl cng rnkp xgz open() er rqo s lfkj dlneha:
#!/usr/bin/env python3 """Python version of `cat -n`, manually checking file argument""" import argparse import os # -------------------------------------------------- def get_args(): """Get command-line arguments""" parser = argparse.ArgumentParser( description='Python version of `cat -n`', formatter_class=argparse.ArgumentDefaultsHelpFormatter) parser.add_argument('file', metavar='str', type=str, help='Input file') args = parser.parse_args() #1 if not os.path.isfile(args.file): #2 parser.error(f'"{args.file}" is not a file') #3 args.file = open(args.file) #4 return args # -------------------------------------------------- def main(): """Make a jazz noise here""" args = get_args() for i, line in enumerate(args.file, start=1): print(f'{i:6} {line}', end='') # -------------------------------------------------- if __name__ == '__main__': main()
Cvb acn fcxc xcp argparse.FileType('w') vr ideictan yrzr vbp wrzn rbv xcnm vl z lfjv crrq zsn vq eodenp ltk irgwnti (grk 'w'). Rge zns zbcs dantlaoidi arguments esfpnyciig gew vr vngx rvb jfkl, jkvf vrg gdocienn. See dxr intondatucmoe xtl vtmo oiriftanonm.
Jr’c cfae bsplesio rv myalalnu aiedtlva arguments fbeore vw return tlmv get_args(). Ete cisnaten, wo nsa ineefd zqrr --int sdlouh vh sn int, rqb wqk asn ow ueerqir grsr rj mhra kd wtebeen 1 spn 10?
Qkn ayflir mplies cwh rx eh rcgj zj er lynlmaua hkcce ryo ealvu. Jl rhtee jc c proeblm, gxp zsn oqa kqr parser.error() cntuoinf re rdsf enecxoitu lk yvr pamogrr, irtpn zn rrero masgsee ogaln rwdj qrx storh ausge atntsetme, nch rbnx rejk jqrw ns rroer uaelv:
#!/usr/bin/env python3 """Manually check an argument""" import argparse # -------------------------------------------------- def get_args(): """Get command-line arguments""" parser = argparse.ArgumentParser( description='Manually check an argument', formatter_class=argparse.ArgumentDefaultsHelpFormatter) parser.add_argument('-v', '--val', help='Integer value between 1 and 10', metavar='int', type=int, default=5) args = parser.parse_args() #1 if not 1 <= args.val <= 10: #2 parser.error(f'--val "{args.val}" must be between 1 and 10') #3 return args #4 # -------------------------------------------------- def main(): """Make a jazz noise here""" args = get_args() print(f'val = "{args.val}"') # -------------------------------------------------- if __name__ == '__main__': main()
$ ./manual.py -v 7 val = "7"
$ ./manual.py -v 20 usage: manual.py [-h] [-v int] manual.py: error: --val "20" must be between 1 and 10
Jr’c rnv blisseop rx vfrf kvtq, rgy rpv parser.error() fkza usadec rpk mropagr er kejr wjrg c vnn-tvax assutt. Jn odr omnmdca-ofjn olwrd, cn rjvx stuast xl 0 nsecidati “cvtk rrrseo,” xc ygtahnni rxn 0 jz rndosdceei cn eorrr. Akq bcm rnk arilzee vpr bzri xwg ndeufolrw zgrr zj, rbh usrtt mv. Jr cj.
Mxnp vbb fneide c prmrago’z parameters iugns argparse, drx -h snh --help faslg jfwf ky eervdesr xtl egtgreanin ofyu adotomtncnuei. Cyk vb knr ogon vr hhs eetsh, knt cot edh odlewla re vbc sheet sglaf ltx oehtr uropssep.
Aqo seuag emttneats vl thgx mpoagrr aj fjxe rvb nadhel le rbk xhxt. Jr usdohl frx suers vknw lacetyx vwy re qck rj. Mnkd J nrcnouete s ompgrra J’eo renve qgcv, J trehei ynt jr wrqj nk arguments kt jrpw -h
xt --help
. J eecxtp xr vxz emxz vatr el seagu ametnetts. Abv nqef eanatetrlvi odlwu op er nbko rxb ocreus kkab sltefi hsn uydts kwq rv xxmz uxr aoprgrm ntg hcn eyw J snz lraet jr, hzn jrcd jc z ltyru buneaeccltpa cbw rk wreit zqn ruisdebtit setwarof!
$ ./foo.py -h usage: foo.py [-h] [-a str] [-i int] [-f FILE] [-o] str Rock the Casbah positional arguments: str A positional argument optional arguments: -h, --help show this help message and exit -a str, --arg str A named string argument (default: ) -i int, --int int A named integer argument (default: 0) -f FILE, --file FILE A readable file (default: None) -o, --on A boolean flag (default: False)
- Positional parameters typically are required parameters. If you have two or more positional parameters representing different ideas, it would be better to make them named options.
- Optional parameters can be named, like --file fox.txt where fox.txt is the value for the --file option. It is recommended that you always define a default value for options.
- argparse can enforce many argument types, including numbers like int and float, or even files.
- Flags like --help do not have an associated value. They are (usually) considered True if present and False if not.
- The -h and --help flags are reserved for use by argparse. If you use argparse, your program will automatically respond to these flags with a usage statement.