concept constructor in category angular
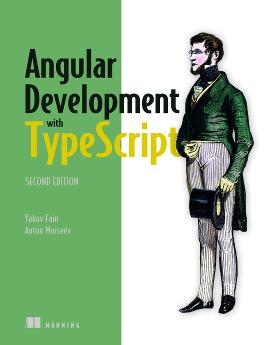
This is an excerpt from Manning's book Angular Development with Typescript, Second Edition.
The class in listing 2.1 has an empty constructor and one method, ngOnInit(), which is one of the component lifecycle methods. If implemented, ngOnInit() is invoked after the code in the constructor. OnInit is one of the lifecycle interfaces that require the implementation of ngOnInit(). We’ll cover a component lifecycle in section 9.2 in chapter 9.
Listing 11.11. Adding the error description in a custom validator
function ssnValidator(control: FormControl): {[key: string]: any} { const value: string = control.value || ''; const valid = value.match(/^\d{9}$/); return valid ? null : {ssn: {description: 'SSN is invalid'}}; #1 } @Component({ selector: 'app', template: ` <form [formGroup]="myForm"> SSN: <input type="text" formControlName="socialSecurity"> <span [hidden]="!myForm.hasError('ssn', 'socialSecurity')"> #2 {{myForm.getError('ssn', 'socialSecurity')?.description}} #3 </span> </form> ` }) class AppComponent { myForm: FormGroup; constructor() { this.form = new FormGroup({ 'socialSecurity': new FormControl('', ssnValidator) }); } }
When you use a constructor with access modifiers, the TypeScript compiler takes it as an instruction to create and retain class properties matching the constructor arguments. You don’t need to explicitly declare and initialize them. Both the short and long versions of the Person class generate the same JavaScript, but we recommend using the shorter syntax as shown in figure B.3.
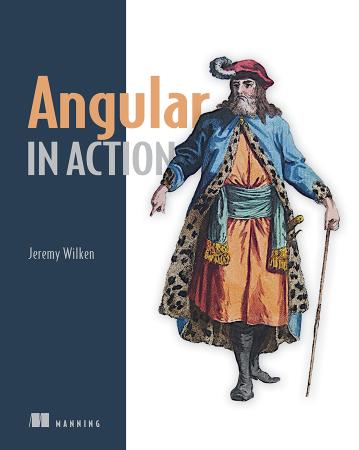
This is an excerpt from Manning's book Angular in Action.
The
constructor
method runs as soon as the component is created. It will import the Stocks service onto theservice
property and then request the current list of stock symbols from it. This works because this is a synchronous action that loads a value directly from memory.But we don’t load data from the service in the constructor for a number of reasons. We’ll dig into the complexities later in the book, but the primary reason is due to the way that components are rendered. The constructor fires early in the rendering of a component, which means that often, values are not yet ready to be consumed. Components expose a number of lifecycle hooks that allow you to execute commands at various stages of rendering, giving you greater control over when things occur.
In our code, we use the
ngOnInit
lifecycle hook to call the service to load the stock data. It uses the list of stock symbols that was loaded in the constructor. We then subscribe to wait for the results to return and store them in thestocks
property. This uses the observable approach to handling asynchronous requests. We’ll look at observables in depth later as well. Here we are using them because the HttpClient returns an observable for us to receive the response. This is exposed as a stream of data, even though it is a single event.
Here we import the NgbModal service that will allow us to create a new modal. We saw the NgbActiveModal service in the Nodes Detail component, which, once the modal and its component has been created, will allow the Nodes Detail component to reference the active modal instance. We need to import the Nodes Detail component as well. The constructor also sets the
modalService
property with an instance of the NgbModal service.
Listing 7.1 Forum component getting access to route parameters
export class ForumComponent implements OnInit { forum: Forum; constructor( private route: ActivatedRoute, #A private router: Router, #A private forumsService: ForumsService) { } ngOnInit() { this.route.params.subscribe((params: Params) => { #B this.forum = this.forumsService.forum(params['forum_alias']); #C if (!this.forum) this.router.navigate(['/not-found']); #D }); } }