concept operator in category dart
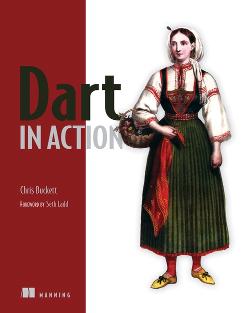
This is an excerpt from Manning's book Dart in Action.
Finally, we’ll cover operator overloading. Overloading happens when you provide a new implementation, customized to your particular class, which allows you to overload the common operators such as > (greater than) and < (less than). This function provides your classes with greater readability when they’re in use. We also revisit Map and look at overloading the [] and []= indexer operators, which let your own classes implement the Map interface and be converted to JSON by the built-in JSON library.
Fortunately, Dart allows this functionality with operator overloading, which means you can take the standard operators and let your own classes provide meaning for them. In this instance, you want to provide meaning for the greater-than and less-than operators in the context of the Role class. Dart lets you do this in the same way you created a new version of the toString() method in chapter 7, by providing your own version of the operators’ implementation. The operator keyword lets Dart know that your class is defining an operator implementation, as shown in the next listing.
When you overload an operator, provide a method containing your implementation of the operator. The operator’s method usually takes a single parameter containing another instance of the same class. Table 8.3 shows some common comparison operators that you can overload.
Table 8.3. Some common comparison operators
Operator method
Description
bool operator >(var other) {...} This instance is greater than the other. bool operator <(var other) {...} This instance is less than the other. bool operator >=(var other) {...} This instance is greater than or equal to the other. bool operator <=(var other) {...} This instance is less than or equal to the other. bool operator equals(var other) {...}
bool operator ==(var other) {...}This instance is equal to the other. Note that there are two different versions of this method. At the time of writing, the language spec defines the word equals as the operator, but the implementations are currently using a double equal sign == to represent the equals operator.
When you’re overloading operators, the other value should be the same class, but there’s no requirement that it must be the same class. This situation provides for some interesting, if slightly unorthodox, syntax. For example, to add a role to a user, you could overload the Users + operator, allowing you to write the code shown in the following listing.