concept Autofac in category dependency injection
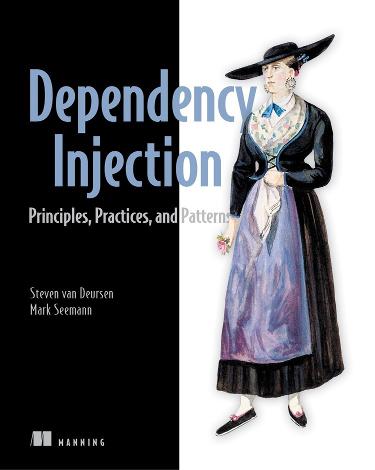
This is an excerpt from Manning's book Dependency Injection.
In the previous chapters, we discussed patterns and principles that apply to DI in general, but, apart from a few examples, we’ve yet to take a detailed look at how to apply them using any particular DI Container. In this chapter, you’ll see how these overall patterns map to Autofac. You’ll need to be familiar with the material from the previous chapters to fully benefit from this.
Autofac is a fairly comprehensive DI Container that offers a carefully designed and consistent API. It’s been around since late 2007 and is, at the time of writing, one of the most popular containers.1
In this section, you’ll learn where to get Autofac, what you get, and how you start using it. We’ll also look at common configuration options. Table 13.1 provides fundamental information that you’re likely to need to get started.
Table 13.1 Autofac at a glance (view table figure)
Question Answer Where do I get it? From Visual Studio, you can get it via NuGet. The package name is Autofac. Alternatively, the NuGet package can be downloaded from the GitHub repository (https://github.com/autofac/Autofac/releases). Which platforms are supported? .NET 4.5 (without a .NET Core SDK) and .NET Standard 1.1 (.NET Core 1.0, Mono 4.6, Xamarin.iOS 10.0, Xamarin.Mac 3.0, Xamarin.Android 7.0, UWP 10.0, Windows 8.0, Windows Phone 8.1). Older builds that support .NET 2.0 and Silverlight are available via NuGet history. How much does it cost? Nothing. It’s open source. How is it licensed? MIT License. Where can I get help? You can get commercial support from companies associated with the Autofac developers. Read more about the options at https://autofac.readthedocs.io/en/latest/support.html. Other than commercial support, Autofac is still open source software with a thriving ecosystem, so you’re also likely (but not guaranteed) to get help by posting on Stack Overflow at https://stackoverflow.com or by using the official forum at https://groups.google.com/group/autofac. On which version is this chapter based? 4.9.0-beta1 Using Autofac isn’t that different from using the other DI Containers that we’ll discuss in the following chapters. As with Simple Injector and Microsoft.Extensions.DependencyInjection, usage is a two-step process, as figure 13.1 illustrates. First, you configure a
ContainerBuilder
, and when you’re done with that, you use it to build a container to resolve components.Figure 13.1 The pattern for using Autofac is to first configure it, and then resolve components.
![]()
When you’re done with this section, you should have a good feeling for the overall usage pattern of Autofac, and you should be able to start using it in well-behaved scenarios — where all components follow proper DI patterns like Constructor Injection. Let’s start with the simplest scenario and see how you can resolve objects using an Autofac container.
Autofac’s configuration support is implemented in a separate assembly. To use this feature, you must add a reference to the
Autofac.Configuration
assembly (https://mng.bz/1Q4V).Once you have a reference to
Autofac.Configuration
, you can ask theContainerBuilder
to read component registrations from the standard .config file like this:var configuration = new ConfigurationBuilder() #1 .AddJsonFile("autofac.json") #1 .Build(); #1 builder.RegisterModule( #2 new ConfigurationModule(configuration)); #2 #1 Loads the autofac.json configuration file using .NET Core’s configuration system. By default, the configuration file will be located in the application’s root directory. #2 Wraps the created configuration in an Autofac module that processes the configuration file and maps file-based registrations in Autofac. That module is added to the builder using RegisterModule.
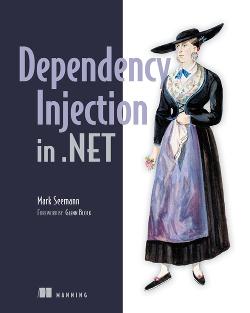
This is an excerpt from Manning's book Dependency Injection in .NET.
DI Container feature comparison chart
Feature Castle Windsor Structure-Map Spring .NET Autofac Unity MEF CODE AS CONFIGURATION X X X X AUTO-REGISTRATION X X X XML configuration X X X X X Modular configuration X X X X X X Custom lifetimes X X (X) X Decommissioning X X (X) X INTERCEPTION X X X (X) = partial support
Autofac is an example of what we could call second-generation DI CONTAINERS.
Autofac has a rather good understanding of sequences, so if we want to use all registered components of a given service, AUTO-WIRING just works. As an example, given the configured ICourse instances in listing 13.2, you can configure the IMeal service like this:
Notice that this is a completely standard mapping from a concrete type to an ABSTRACTION. Autofac will automatically understand the Meal constructor and determine that the correct course of action is to resolve all ICourse components. When you resolve IMeal, you get a Meal instance with the ICourse components from listing 13.2: Rillettes, CordonBleu, and MousseAuChocolat.
Autofac automatically handles sequences, and unless we specify otherwise, it does what we’d expect it to do: it resolves a sequence of DEPENDENCIES to all registered components of that type. Only when we need to explicitly pick only some components from a larger set do we need to do more. Let’s see how we can do that.
In this section, you’ll learn where to get Autofac, what you get, and how you start using it. We’ll also look at common configuration options, as well as how to package configuration settings into reusable components. Table 13.1 provides fundamental information that you’re likely to need to get started.
Table 13.1. Autofac at a glance
Answer
Where do I get it? Go to http://autofac.org and click the appropriate link under Featured Download. From Visual Studio 2010 you can also get it via NuGet. The package name is Autofac. What’s in the download? You can download a .zip file with precompiled binaries. You can also download the source code and compile it yourself, although it can be difficult to figure out which change set corresponds to a particular release. The last part of the build number (for example, 724 in this chapter) corresponds to the source code revision, but to find that you’ll need the Mercurial source control tools. Which platforms are supported? .NET 3.5 SP1, .NET 4, Silverlight 3, Silverlight 4. Older versions are available that support .NET 2.0, 3.0 and Silverlight 2 (select All Releases on the Download tab). How much does it cost? Nothing. It’s open source. Where can I get help? You can get commercial support from companies associated with the Autofac developers. Read more about the options at http://code.google.com/p/autofac/wiki/CommercialSupport. Other than commercial support, Autofac is still open source software with a thriving ecosystem, so you’re also likely (but not guaranteed) to get help in the official forum at http://groups.google.com/group/autofac. On which version is the chapter based? 2.4.5.724. Using Autofac is a little different from using other DI CONTAINERS. As figure 13.2 illustrates, it’s a more explicit two-step process: first we configure a ContainerBuilder, and when we’re done with that, we use it to build a container that can be used to resolve components.
Figure 13.2. With Autofac, we first create and configure a ContainerBuilder instance. When we’re done configuring the ContainerBuilder, we use it to create a Container that we can subsequently use to resolve components. Notice that the rhythm is pretty much similar to Castle Windsor or StructureMap: configure, then resolve. However, here the separation of concerns is much clearer. A ContainerBuilder can’t resolve components, and we can’t configure a Container.
![]()
When you’re done with this section, you should have a good feeling for the overall usage pattern of Autofac, and you should be able to start using it in well-behaved scenarios where all components follow proper DI patterns such as CONSTRUCTOR INJECTION. Let’s start with the simplest scenario and see how we can resolve objects using an Autofac container.