concept connection string in category entity framework
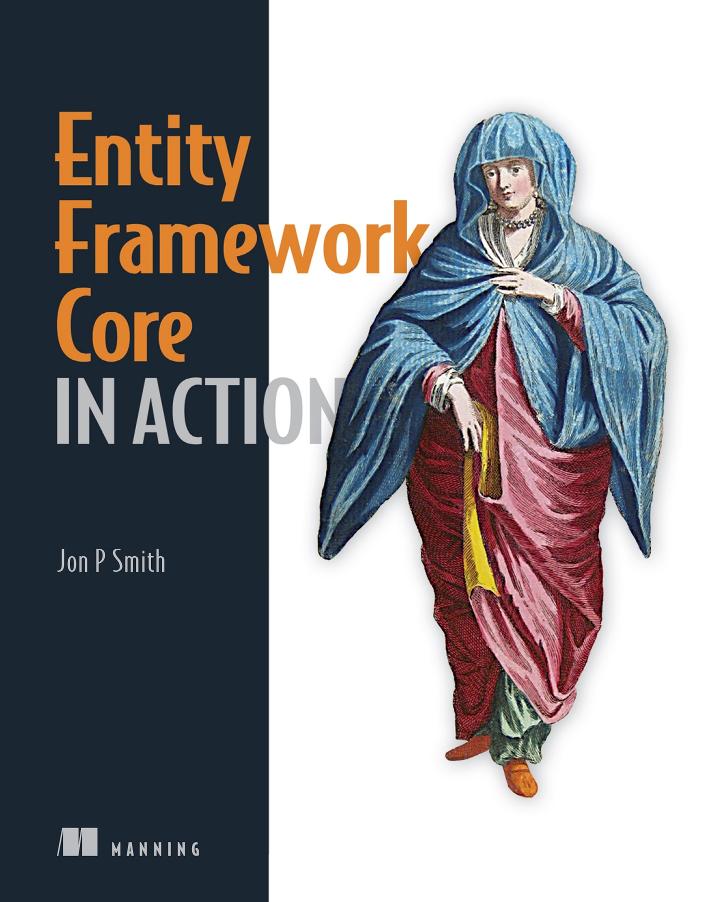
This is an excerpt from Manning's book Entity Framework Core in Action.
For web applications, the location of the database isn’t normally hardcoded into the application because it’ll change when the web application is moved to its host, where real users can access it. Therefore, the location and various database configuration settings are typically stored as a connection string. This string is stored in an application setting file that ASP.NET reads when it starts.
Listing 5.2 The
ConfigureServices
method in theStartup
class of ASP.NET Corepublic void ConfigureServices(IServiceCollection services) #1 { // Add framework services. services.AddMvc(); #2 var connection = Configuration #3 .GetConnectionString("DefaultConnection"); #3 services.AddDbContext<EfCoreContext>( #4 options => options.UseSqlServer(connection, #4 b => b.MigrationsAssembly("DataLayer"))); #5 //… other service defintions removed } #1 The method in ASP.NET to set up services #2 Sets up a series of services to use controllers, etc. #3 You get the connection string from the appsettings.json file, which can be changed when you deploy. #4 Configures the application’s DbContext to use SQL Server and provide the connection #5 You’re using EF Core’s Add-Migrations command, so you need to indicate which project your application’s DbContext is in.Your first step is to get the connection string from the application’s
Configuration
class. In ASP.NET Core, theConfiguration
class is set up during theStartup
class constructor, which reads theappsetting
files. Getting the connection string that way allows you to change the database connection string when you deploy the code to a host. Section 5.8.1, which is about deploying an ASP.NET Core application that uses a database, covers how this works.
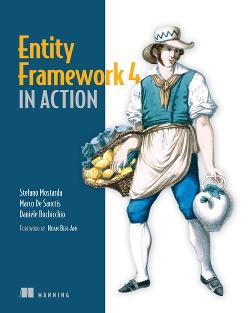
This is an excerpt from Manning's book Entity Framework 4 in Action.
When you’ve finished designing the entities, right-click the designer and select Generate Database Script from Model. A wizard appears, asking for the connection string. After you enter it, the wizard returns the script. Just launch the SQL script on the database, and you’re ready to go.
As you’ve seen, the connection string name is mandatory for the ObjectContext class. How you pass the connection string is slightly different in the Entity Framework compared to how you do it with other ADO.NET frameworks. In Entity Framework, you have to apply the (case-insensitive) prefix Name= to the connection string:
This isn’t the only difference from what you’re likely to be used to. The connection string itself is quite odd. This is how the connection string for OrderIT appears in the connectionString section of the configuration file.
The structure is always the same, but the data is organized in an unusual way. The attribute name represents the name of the connection string, and it’s the one used when passing the connection string name to the context constructor. So far, so good.
The connection string is only the first part of the game. We mentioned in chapter 1 that one of the key features of Entity Framework is that it lets you write queries against the object model and not against the database. In the next section, we’ll focus on this and look at what happens under the covers.