concept mutable state in category functional programming
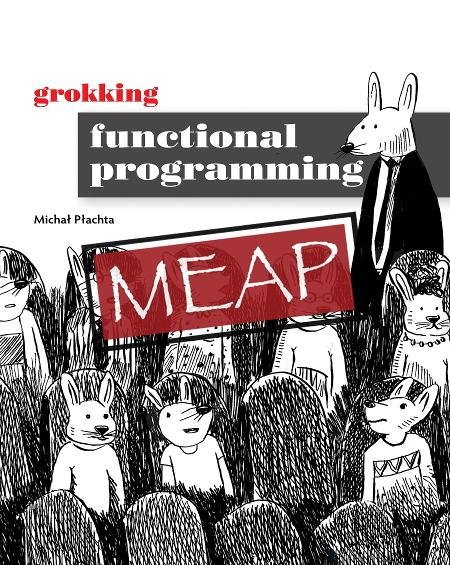
This is an excerpt from Manning's book Grokking Functional Programming MEAP V11.
In the last chapter we met a pure function, which is going to be our best friend throughout the rest of the book. We introduced and briefly discussed some caveats regarding values that may change—mutable states. This chapter focuses on problems with mutable states and explains why pure functions can’t use them in majority of cases. We are going to learn about immutable values, which are used extensively in functional programming. The relation between a pure function and an immutable value is so strong, that we can define the functional programming using only those two concepts:
A state is one instance of a value that is stored in one place and can be accessed from the code. If this value can be modified, we have a mutable state. Furthermore, if this mutable state can be accessed from different parts of the code, it’s a shared mutable state.
![]()
Let’s go through our problematic examples and define which parts could be categorized as shared mutable state and caused us headaches:
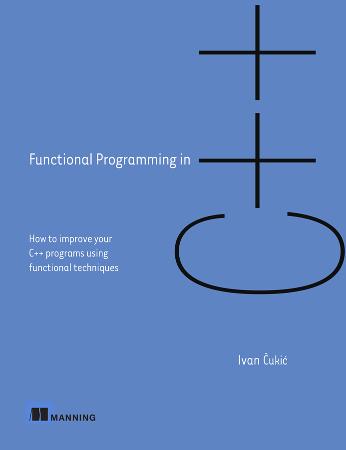
This is an excerpt from Manning's book Functional Programming in C++.
This issue wouldn’t exist if the saving function had its own immutable copy of the data that it should write (see figure 1.3). This is the biggest problem of mutable state: it creates dependencies between parts of the program that don’t need to have anything in common. This example involves two clearly separate user actions: saving the typed text and typing the text. These should be able to be performed independently of one another. Having multiple actions that might be executed at the same time and that share a mutable state creates a dependency between them, opening you to issues like the ones just described.
This is a perfect example of when mutable state is useful. You have a maze and a player, and you’re moving the player around the maze, thus changing its state. Let’s see how to model this in a world of immutable state.
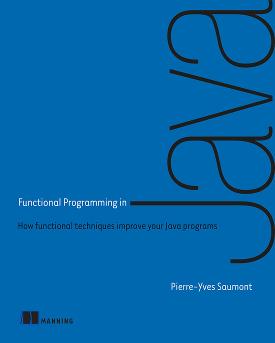
This is an excerpt from Manning's book Functional Programming in Java: How functional techniques improve your Java programs.
Let’s think about what’s happening. If the method takes no argument and returns a value, this value must come from somewhere. Of course, you’d guess that this somewhere is inside the random number generator. The fact that the value changes on each call means that the generator changes between each call; it has a mutable state. So the question is whether the value returned by the nextInt method depends only on the state of the generator, or whether it depends on something else.
The benefit of this approach is that it relieves you from caring about synchronization and locking when accessing resources. But this security is obtained by preventing data sharing. This is good because it forces you to find other, safer ways of doing things. Using immutable lists doesn’t automatically add safety to operations involving sharing those lists. It just prevents you from sharing mutable state. It allows you to fake a list mutation in a way that more or less corresponds to making defensive copies, but without the performance penalty. This is useful, but sometimes it’s not what you need.