concept reduce in category functional programming
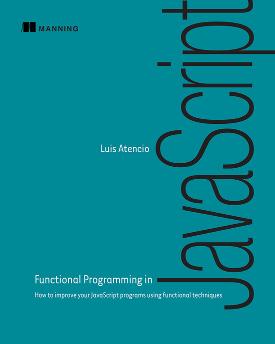
This is an excerpt from Manning's book Functional Programming in JavaScript.
Compared with the previous example, you see that this code frees you from the responsibility of properly managing a loop counter and array index access; put simply, the more code you have, the more places there are for bugs to occur. Also, standard loops aren’t reusable artifacts unless they’re abstracted with functions. And that’s precisely what we’ll do. In chapter 3, I demonstrate how to remove manual loops completely from your code in favor of first-class, higher-order functions like map, reduce, and filter, which accept functions as parameters so that your code is more reusable, extensible, and declarative. This is what I did with the magical run function in listings 1.1 and 1.2.
In this chapter, I’ll introduce you to a few useful and practical operations like map, reduce, and filter that allow you to traverse and transform data structures in a sequential manner. These operations are so important that virtually all functional programs use them in one way or another. They also facilitate removing manual loops from your code, because most loops are just specific cases handled by these functions.
You know how to transform your data, but how do you gather meaningful results from it? Suppose you want to compute the country with the largest count from a collection of Person objects. You can use the reduce function to accomplish this.
reduce is a higher-order function that compresses an array of elements down to a single value. This value is computed from the accumulated result of invoking a function with an accumulator value against each element. This is easier to visualize by looking at the diagram in figure 3.5.