concept tuple in category functional programming
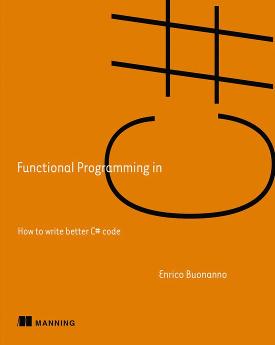
This is an excerpt from Manning's book Functional Programming in C#: How to write better C# code.
Better syntax for tuples is the most important feature of C# 7. It allows you to easily create and consume tuples, and, most importantly, to assign meaningful names to their elements. For example, the Stats property returns a tuple of type (double, double), and specifies meaningful names by which its elements can be accessed:
Tuples are important in FP because of the tendency to break tasks down into very small functions. You may end up with a data type whose only purpose is to capture the information returned by one function, and that’s expected as input by another function. It’s impractical to define dedicated types for such structures, which don’t correspond to meaningful domain abstractions. That’s where tuples come in.
The last example in table 3.1 shows multiple input arguments: we’ll just group them with parentheses (parentheses are used to indicate tuples; that is, we’re notating a binary function as a unary function whose input argument is a binary tuple).
How many possible input values are there? Well, there are two possible values for Gender and 120 for Age, so in total there are 2 * 120 = 240 possible inputs. Notice that if you define a tuple of Age and Gender, 240 tuples are possible. The same is true if you define a custom object to hold that same data, like this:
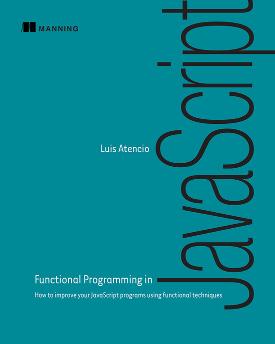
This is an excerpt from Manning's book Functional Programming in JavaScript.
What you need is a stricter policy for immutability; and encapsulation is a good strategy to protect against mutations. For simple object structures, a good alternative is to adopt the Value Object pattern. A value object is one whose equality doesn’t depend on identity or reference, just on its value; once declared, its state may not change. In addition to numbers and strings, some examples of value objects are types like tuple, pair, point, zipCode, coordinate, money, date, and others. Here’s an implementation for zipCode:
But how can you return two different values? Functional languages have support for a structure called a tuple. It’s a finite, ordered list of elements, usually grouping two or three values at a time, and written (a, b, c). Based on this concept, you can use a tuple as a return value from isValid that groups a status with a possible error message, to be returned as a single entity and subsequently passed to another function if need be. Let’s explore tuples in more detail.
Tuples are immutable structures that pack together items of different types so that they can be passed into other functions. There are other ways of returning ad hoc data, such as object literals or arrays:
return { status : false, or return [false, 'Input is too long!']; message: 'Input is too long!' }; !@%STYLE%@! {"css":"{\"css\": \"font-weight: bold;\"}","target":"[[{\"line\":1,\"ch\":34},{\"line\":1,\"ch\":36}]]"} !@%STYLE%@!But when it comes to transferring data between functions, tuples offer more advantages:
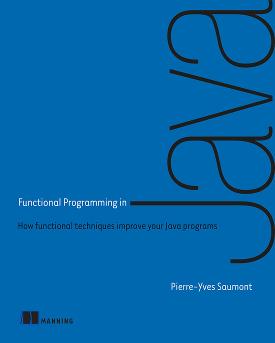
This is an excerpt from Manning's book Functional Programming in Java: How functional techniques improve your Java programs.
Rather than creating a specific Purchase class, you’ll use a generic one that you’ll call Tuple. This class will be parameterized by the two types it will contain (Donut and Payment). The following listing shows its implementation, as well as the way it’s used in the DonutShop class.
N x N is the set of all possible pairs of integers. An element of this set is a pair of integers, and a pair is a special case of the more general tuple concept used to represent combinations of several elements. A pair is a tuple of two elements.
It’s important to note that, in this case, addTax is not a function of price, because it won’t always give the same result for the same argument. It may, however, be seen as a function of the tuple (price, taxRate).
One way to make programs more modular is to use functions of tuples of arguments:
But using tuples is cumbersome, because Java doesn’t offer a simple syntax for this, except for function arguments, where the parentheses notation can be used. You’d have to define a special interface for a function of tuples, such as this: