concept default method in category java
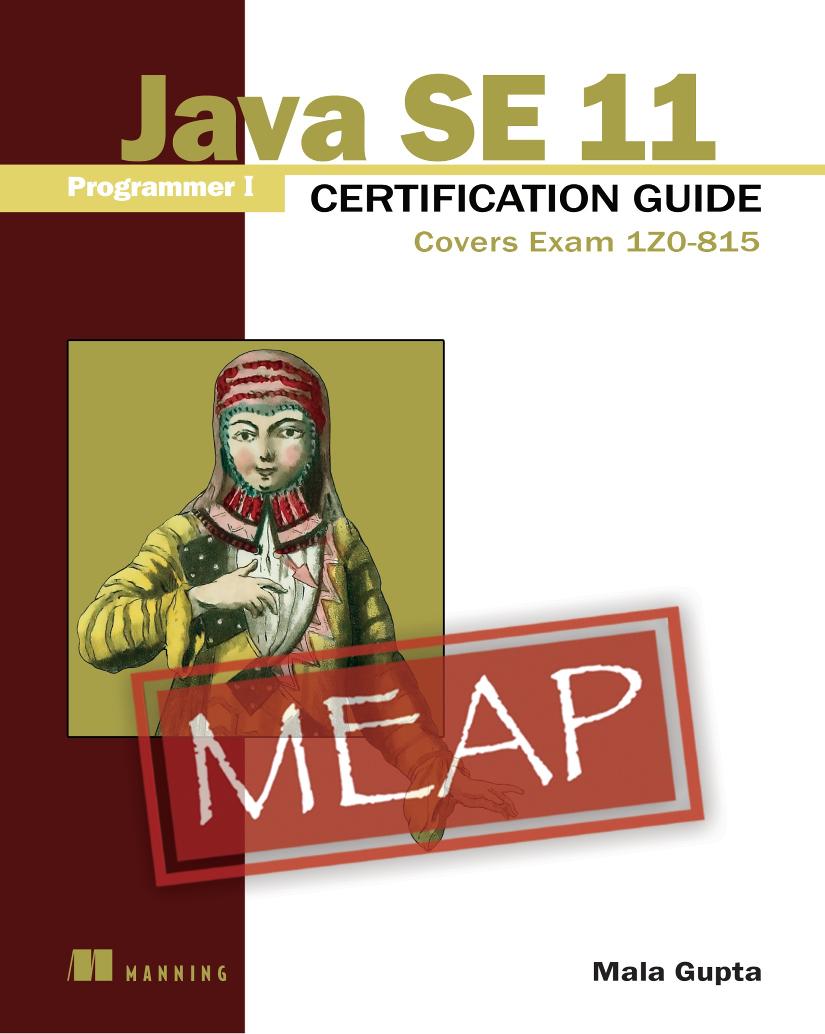
This is an excerpt from Manning's book Java SE 11 Programmer I Certification Guide MEAP V03.
With Java 8, you can use the keyword this in an interface’s default method to access its constants and other default and abstract methods. In the following example, the interface Interviewer defines a default method submitInterviewStatus. This method uses this to access itself and its constants or methods:
Exam objectives covered in this chapter
What you need to know
[10.1] Create and implement interfaces
How to create interfaces, extend and implement them. The role of interfaces in implementing polymorphism.
The types of methods that can be defined in an interface – abstract, static and default.
Implicit properties of constants and methods defined in an interface.
Challenges with inheriting multiple interfaces.
Implications of modifying methods of an interface – their signature or implementations of default methods.
[10.2] Distinguish class inheritance from interface inheritance including abstract classes
Differences and similarities in inheriting abstract or concrete classes and implementing interfaces.
[10.3] Declare and use List and ArrayList instances
How to declare, create, and use List and ArrayList. Advantages of using an ArrayList over arrays.
Use of methods that add, modify, and delete elements of an ArrayList.
[10.4] Understanding lambda expressions
Syntax and usage of lambda expressions. Usage of Predicate class.
Imagine you need to add a behavior—submit interview status—to the interface Interviewer, after its publication. This wouldn’t have been possible with Java 7 and its earlier versions without implying the need to provide an implementation for each existing concrete class (either directly or through a superclass). Default methods can rescue you here. Starting with Java 8, interfaces can be augmented by adding methods with default implementation. Implementing classes might choose to override these methods to define their own specific behavior. If they don’t choose to override them, the default implementation from the interface is used. The definition of a default method must include the keyword default:
interface Interviewer { abstract void conductInterview(); default void submitInterviewStatus() { #A System.out.println("Accept"); } }I deliberately oversimplified submitInterviewStatus() in the preceding code so that the code focuses on the definition of default methods and not on its implementation details.
New in Java 8 Interface methods can define an implementation by using default methods.
Changing a default method to abstract or static
If you modify an interface and change its default method to an abstract method, a class that implements it might fail to compile. The implementing class will fail to compile, if it doesn’t override the default method of the interface. If you modify the default method to a static method in an interface, the code that calls the method won’t compile. The code, changes, and results are shown in figure 11.12.
Figure 11.12 What happens when you change a default method in an interface to an abstract or static method
![]()
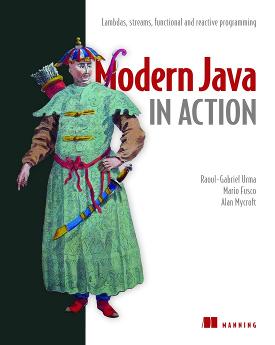
This is an excerpt from Manning's book Modern Java in Action: Lambdas, streams, reactive and functional programming.
Large-scale component-based programming, and evolving a system’s interfaces, weren’t historically well served by Java. You can now specify modules to structure systems in Java 9 and use default methods to allow an interface to be enhanced without changing all the classes that implement it.
Wow! Are interfaces like abstract classes now? Yes and no; there are fundamental differences, which we explain in this chapter. More important, why should you care about default methods? The main users of default methods are library designers. As we explain later, default methods were introduced to evolve libraries such as the Java API in a compatible way, as illustrated in figure 13.1.
In a nutshell, adding a method to an interface is the source of many problems; existing classes implementing the interface need to be changed to provide an implementation for the method. If you’re in control of the interface and all its implementations, the situation isn’t too bad. But this is often not the case—and it provides the motivation for default methods, which let classes inherit a default implementation from an interface automatically.
If you’re a library designer, this chapter is important because default methods provide a means of evolving interfaces without modifying existing implementations. Also, as we explain later in the chapter, default methods can help structure your programs by providing a flexible mechanism for multiple inheritance of behavior; a class can inherit default methods from several interfaces. Therefore, you may still be interested in finding out about default methods even if you’re not a library designer.
The chapter is structured as follows. First, we walk you through a use case of evolving an API and the problems that can arise. Then we explain what default methods are and discuss how you can use them to tackle the problems in the use case. Next, we show how you can create your own default methods to achieve a form of multiple inheritance in Java. We conclude with some more technical information about how the Java compiler resolves possible ambiguities when a class inherits several default methods with the same signature.
You’ve seen that default methods can be useful for evolving a library in a compatible way. Can you do anything else with them? You can create your own interfaces that have default methods too. You may want to do this for two use cases that we explore in the following sections: optional methods and multiple inheritance of behavior.
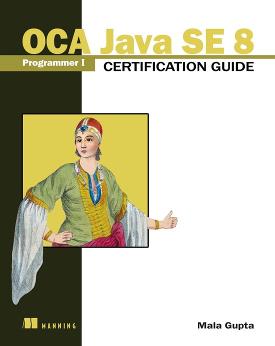
This is an excerpt from Manning's book OCA Java SE 8 Programmer I Certification Guide.
The default methods are also referred to as defender or virtual extension methods. But the most popular term to refer them is default methods because the default keyword is used to identify them.
Manager@19e0bfd 9999 I am Manager@19e0bfd
In this section, you’ll see what happens when you modify an interface by changing the type of its method (abstract, default, or static). This change can affect the classes that implement the interface or the code that calls the modified methods.
Figure 6.15. What happens when you change a static method in an interface to a default or abstract method
![]()
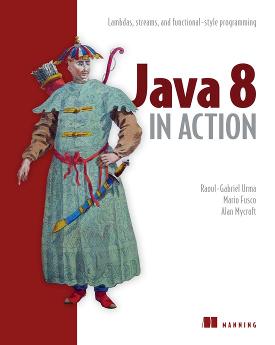
This is an excerpt from Manning's book Java 8 in Action: Lambdas, streams, and functional-style programming.
From a slightly revisionist viewpoint, the addition of Streams in Java 8 can be seen as a direct cause of the two other additions to Java 8: concise techniques to pass code to methods (method references, lambdas) and default methods in interfaces.
Wow! Are interfaces like abstract classes now? Yes and no; there are fundamental differences, which we explain in this chapter. But more important, why should you care about default methods? The main users of default methods are library designers. As we explain later, default methods were introduced to evolve libraries such as the Java API in a compatible way, as illustrated in figure 9.1.
In a nutshell, adding a method to an interface is the source of many problems; existing classes implementing the interface need to be changed to provide an implementation for the method. If you’re in control of the interface and all the implementations, then it’s not too bad. But this is often not the case. This is the motivation for default methods: they let classes automatically inherit a default implementation from an interface.
So if you’re a library designer, this chapter is important because default methods provide a means to evolve interfaces without causing modifications to existing implementations. Also, as we explain later in the chapter, default methods can help structure your programs by providing a flexible mechanism for multiple inheritance of behavior: a class can inherit default methods from several interfaces. Therefore, you may still be interested in finding out about default methods even if you’re not a library designer.
The chapter is structured as follows. We first walk you through a use case of evolving an API and the problems that can arise. We then explain what default methods are and how they can tackle the problems faced in the use case. Next, we show how you can create your own default methods to achieve a form of multiple inheritance in Java. We conclude with some more technical information about how the Java compiler resolves possible ambiguities when a class inherits several default methods with the same signature.
You’ve seen how default methods can be useful to evolve a library in a compatible way. Is there anything else you can do with them? You can create your own interfaces that have default methods too. You may want to do this for two use cases that we explore in this section: optional methods and multiple inheritance of behavior.