concept Integer in category java
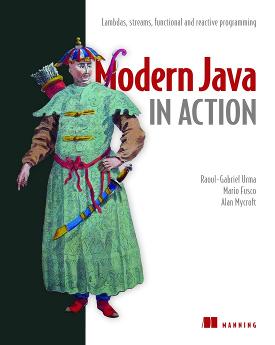
This is an excerpt from Manning's book Modern Java in Action: Lambdas, streams, reactive and functional programming.
Think about the possible values manipulated by Java programs. First, there are primitive values such as 42 (of type int) and 3.14 (of type double). Second, values can be objects (more strictly, references to objects). The only way to get one of these is by using new, perhaps via a factory method or a library function; object references point to instances of a class. Examples include "abc" (of type String), new Integer(1111) (of type Integer), and the result new HashMap<Integer, String>(100) of explicitly calling a constructor for HashMap. Even arrays are objects. What’s the problem?
Comparator<Apple> c1 = (Apple a1, Apple a2) -> a1.getWeight().compareTo(a2.getWeight()); ToIntBiFunction<Apple, Apple> c2 = (Apple a1, Apple a2) -> a1.getWeight().compareTo(a2.getWeight()); BiFunction<Apple, Apple, Integer> c3 = (Apple a1, Apple a2) -> a1.getWeight().compareTo(a2.getWeight());
In essence, Java 8 lambdas have enriched what you can write, but the type system hasn’t kept up with the flexibility of the code. In many functional languages, you can write, for example, the type (Integer, Double) => String, to represent what Java 8 calls BiFunction<Integer, Double, String>, along with Integer => String to represent Function<Integer, String>, and even () => String to represent Supplier<String>. You can understand => as an infix version of Function, BiFunction, Supplier, and the like. A simple extension to Java syntax for types to allow infix => would result in more readable types analogous to what Scala provides, as discussed in chapter 20.
Given all the implicit boxing and unboxing that Java has slowly acquired since Java 1.1, you might ask whether it’s time for Java to treat, for example, Integer and int identically and to rely on the Java compiler to optimize into the best form for the JVM.
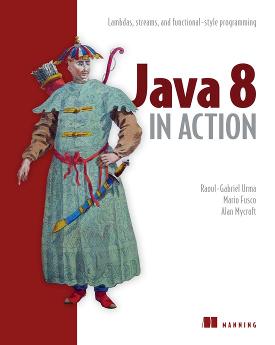
This is an excerpt from Manning's book Java 8 in Action: Lambdas, streams, and functional-style programming.
Think about the possible values manipulated by Java programs. First, there are primitive values such as 42 (of type int) and 3.14 (of type double). Second, values can be objects (more strictly, references to objects). The only way to get one of these is by using new, perhaps via a factory method or a library function; object references point to instances of a class. Examples include "abc" (of type String), new Integer(1111) (of type Integer), and the result new HashMap<Integer, String>(100) of explicitly calling a constructor for HashMap. Even arrays are objects. So what’s the problem?
As some level this seems harmless—if you get a primitive 42 from an ArrayList<int> and a String object “abc” from an ArrayList<String>, then why should you worry that the ArrayList containers are indistinguishable? Unfortunately, the answer is garbage collection, because the absence of run-time type information about the contents of the ArrayList would leave the JVM unable to determine whether element 13 of your ArrayList was an Integer reference (to be followed and marked as “in use” by GC) or an int primitive value (most definitely not to be followed).
Given all the implicit boxing and unboxing that Java has slowly acquired since Java 1.1, you might ask whether it’s time for Java to treat, for example, Integer and int identically and to rely on the Java compiler to optimize into the best form for the JVM.
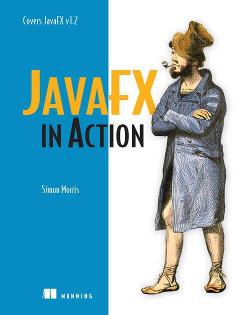
This is an excerpt from Manning's book JavaFX in Action.
Table 2.1. JavaFX Script value types
Type
Details
Java equivalent
True or false flag
java.lang.Boolean
Signed 8-bit integer. JFX 1.1+
java.lang.Byte
Unsigned 16-bit Unicode. JFX 1.1+
java.lang.Character
Signed 64-bit fraction. JFX 1.1+
java.lang.Double
Time interval
None
Signed 32-bit fraction. JFX 1.1+
java.lang.Float
Signed 32-bit integer
java.lang.Integer
Signed 64-bit integer. JFX 1.1+
java.lang.Long
Signed 32-bit fraction.
java.lang.Float
Signed 16-bit integer. JFX 1.1+
java.lang.Short
Unicode text string
java.lang.String
JavaFX Script variables are statically, not dynamically, typed. The language has no concept of primitives in the way Java does; everything is an object. Some types may be declared using a literal syntax. We call these the value types, and they are Boolean, Byte, Character, Double, Duration, Float, Integer, Long, Number, Short, and String. KeyFrame, an animation class, also has its own specific literal syntax (dealt with in the chapters dealing with animation timelines).