concept main method in category java
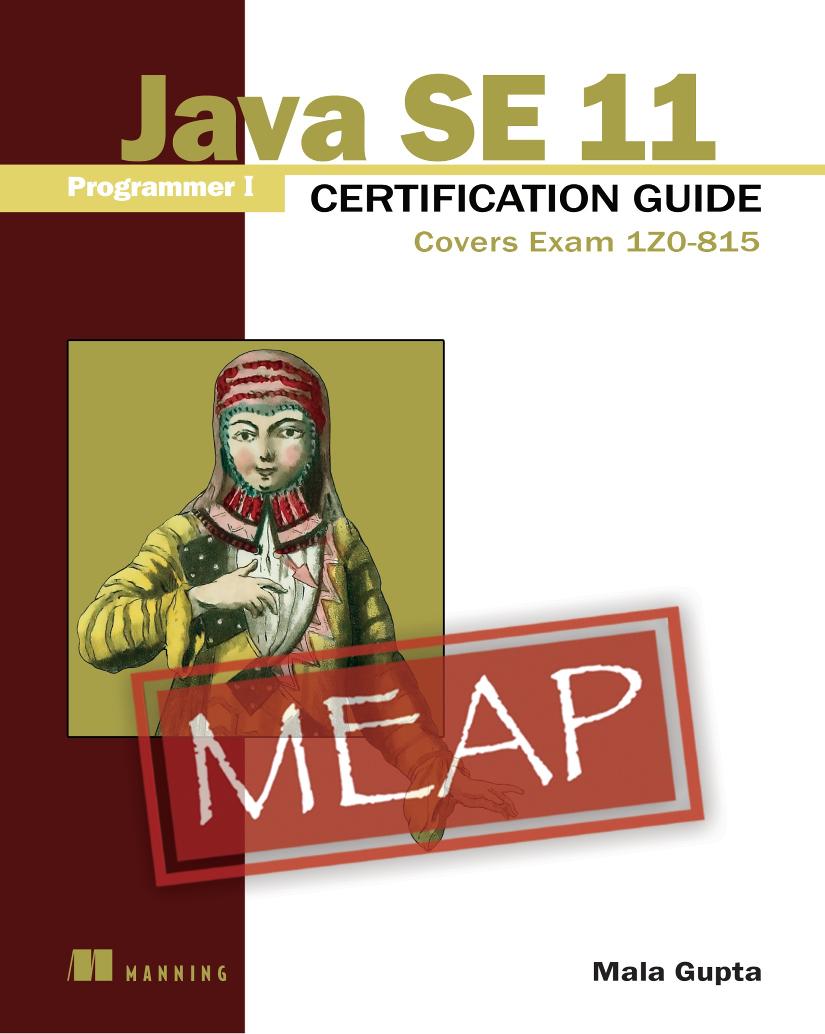
This is an excerpt from Manning's book Java SE 11 Programmer I Certification Guide MEAP V03.
Figure 1.7 Parts of a main method
![]()
Exam objectives covered in this chapter
What you need to know
[2.1] Create executable Java applications with a main method.
The right method signature for the main method to create an executable Java application.
The arguments that are passed to the main method.
[2.2] Compile and run a Java program from the command line.
[2.3] Import other Java packages to make them accessible in your code.
Compiling and executing a Java program using javac and java tools.
Understand packages and import statements. Get the right syntax and semantics to import classes and interfaces from packages in your own classes.
Figure 2.4 Passing command parameters to a main method
![]()
As you can see from the output shown in figure 2.4, the keyword java and the name of the class aren’t passed on as command parameters to the main method. The OCP Java SE 11 Programmer I exam will test you on your knowledge of whether the keyword java and the class name are passed on to the main method.
Exam Tip The method parameters that are passed on to the main method are also called command-line parameters or command-line values. As the name implies, these values are passed on to a method from the command line.
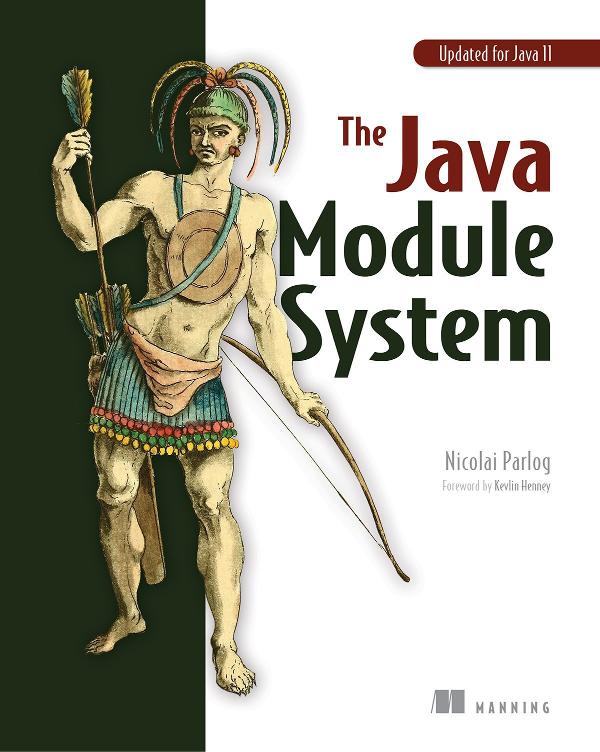
This is an excerpt from Manning's book The Java Module System.
Class that contains the application’s main method
Initial module —Application module where compilation starts (for javac
) or containing themain
method (forjava
). Section 5.1.1 shows how to specify it when launching the application with thejava
command. The compiler also has a use for the concept: as explained in section 4.3.5, it defines which module the compilation starts with.
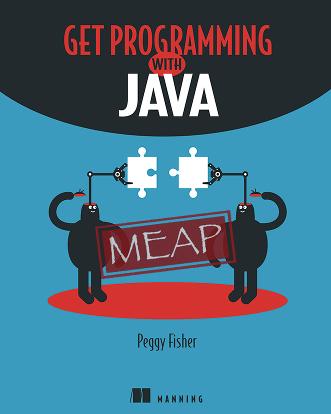
This is an excerpt from Manning's book Get Programming with Java MEAP V04 livebook.
Every Java project must include at least one file that contains a main method. In our first program, line 3 is the start of this method. The main method extends from line 3 where it starts with an open curly brace and ends on line 5 with a closing curly brace. In the next few lessons, we will talk more about exactly what each line of code is doing in this example.
As I’ve stated before, everyone programs differently. So, the order in which you create the classes for this application might vary from my approach. I have decided to start by creating an Author class, then a Book class. Finally, I’ll add an ACMEPublishing class which contains the main method. For this project, there is no need for an interface.
Listing 17.1 contains code for a Person class and a main method
1 package lesson17_examples; 2 public class Lesson17_Examples { 3 public static void main(String[] args) { 4 int x = 35; 5 int y = 5; 6 if(x == y) //#A 7 System.out.println("x is equal to y"); 8 9 Person p1 = new Person("Cy", "Young", 35); 10 Person p2 = new Person("cy", "young", 35); 11 if(p1 == p2) //#B 12 System.out.println("p1 is equal to p2"); 13 if(p1.age == p2.age) System.out.println("p1 age is equal to p2 age"); //#C 14 if(x == p1.age) System.out.println("x is equal to p1 age"); //#D 15 } 16 } 17 class Person { 18 String fname, lname; 19 int age; 20 public Person(String fname, String lname, int age) { 21 this.fname = fname; 22 this.lname = lname; 23 this.age = age; 24 } 25 }
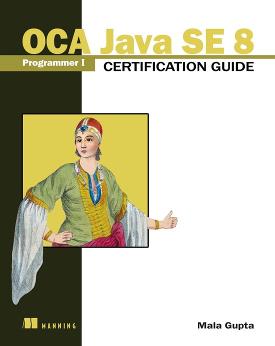
This is an excerpt from Manning's book OCA Java SE 8 Programmer I Certification Guide.
To output java one, the main method should output the first and either the second or third method parameters passed to it.
In the next section, you’ll create executable Java applications—classes that are used to define an entry point of execution for a Java application.
public class HelloExamWithParameters { public static void main(String args[]) { System.out.println(args[0]); System.out.println(args[1]); } }