concept Map in category java
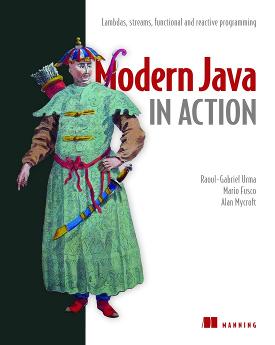
This is an excerpt from Manning's book Modern Java in Action: Lambdas, streams, reactive and functional programming.
The capability of referring to a constructor without instantiating it enables interesting applications. For example, you can use a Map to associate constructors with a string value. You can then create a method giveMeFruit that, given a String and an Integer, can create different types of fruits with different weights, as follows:
How about Map? There’s no elegant way of creating small maps, but don’t worry; Java 9 added factory methods to make your life simpler when you need to create small lists, sets, and maps.
This method is convenient if you want to create a small map of up to ten keys and values. To go beyond this, use the alternative factory method called Map.ofEntries, which takes Map.Entry<K, V> objects but is implemented with varargs. This method requires additional object allocations to wrap up a key and a value:
import static java.util.Map.entry; Map<String, Integer> ageOfFriends = Map.ofEntries(entry("Raphael", 30), entry("Olivia", 25), entry("Thibaut", 26)); System.out.println(ageOfFriends); #1Map.entry is a new factory method to create Map.Entry objects.
Java 8 introduced several default methods supported by the Map interface. (Default methods are covered in detail in chapter 13, but here you can think of them as being preimplemented methods in an interface.) The purpose of these new operations is to help you write more concise code by using a readily available idiomatic pattern instead of implementing it yourself. We look at these operations in the following sections, starting with the shiny new forEach.
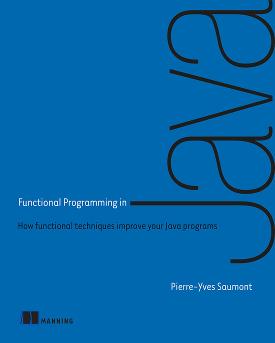
This is an excerpt from Manning's book Functional Programming in Java: How functional techniques improve your Java programs.
This is an instance method of Map that returns a list of all the values in the map:
Functional trees, like the red-black tree you’ve developed, have the advantage of immutability, which allows you to use them in multithreaded environments without bothering about locks and synchronization. The next listing shows the interface of a Map that can be implemented using the red-black tree.
Not all tree operations have been delegated because some operations don’t make much sense in the current conditions. But you may need additional operations in some special use cases. Implementing these operations is easy: extend the Map class and add delegating methods. For example, you might need to find the object with the maximal or minimal key. Another possible need is to fold the map, perhaps to get a list of the contained values. Here’s an example of delegating the foldLeft method:
Generally, folding maps occur in very specific use cases that deserve to be abstracted inside the Map class.
The Map class is useful and relatively efficient, but it has a big disadvantage compared to the maps you may be used to: the keys must be comparable. The types used for keys are usually comparable, such as integers or strings, but what if you need to use a noncomparable type for the keys?
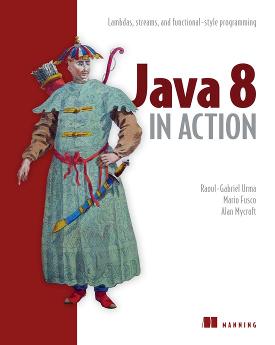
This is an excerpt from Manning's book Java 8 in Action: Lambdas, streams, and functional-style programming.
The capability of referring to a constructor without instantiating it enables interesting applications. For example, you can use a Map to associate constructors with a string value. You can then create a method giveMeFruit that, given a String and an Integer, can create different types of fruits with different weights:
This section briefly introduces basic Scala features so you can get a feel for simple Scala programs. We start with a slightly modified “Hello world” example written in an imperative style and a functional style. We then look at some data structures that Scala supports—List, Set, Map, Stream, Tuple, and Option—and compare them to Java 8. Finally, we present traits, Scala’s replacement for Java’s interfaces, which also support inheritance of methods at object-instantiation time.
Creating collections in Scala is simple, thanks to its emphasis on conciseness. To exemplify, here’s how to create a Map:
Several things are new with this line of code. First, it’s pretty awesome that you can create a Map and associate a key to a value directly, using the syntax ->. There’s no need to add elements manually like in Java:
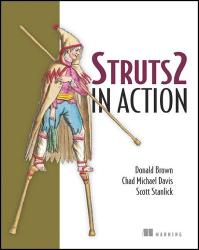
This is an excerpt from Manning's book Struts 2 in Action.
We’ve already learned how to take advantage of the automatic data transfer for simple cases. In this chapter, we’ll learn how to take advantage of more complex forms of automatic data transfer. Most of the increased complexity comes when transferring data onto more complex Java-side types, such as Maps and Lists. We haven’t mentioned it much so far, but when the framework transfers data from string-based request parameters to strictly typed Java properties, it must also convert from string to Java type. The framework comes with a strong set of built-in type converters that support all common conversions, including complex types such as Maps and Lists. The central focus of this chapter will be explaining how to take advantage of the framework’s ability to automatically transfer and convert all manner of data. At the end of the chapter, we’ll also show you how to extend the type conversion mechanism by developing custom converters that can handle any types, including user-defined types.
The last out-of-the-box conversion we’ll cover is conversion to Maps. Similar to its support for Lists, Struts 2 also supports automatic conversion of a set of values from the HTTP request into a Map property. Maps associate their values with keys rather than indexes. This keyed nature has a couple of implications for the Struts 2 type conversion process. First, the OGNL expression syntax for referencing them is different than for Lists because it has to provide a key rather than a numeric index. The second implication involves specifying types for the Map properties. We’ve already seen that we can specify a type for our List elements. You can do this with Maps also. With Maps, however, you can also specify a type for the key object. As you might expect, both the Map element and key will default to a String if you don’t specify a type. We’ll explore all of this in the examples of this section. Again, the examples can be seen in action on the chapter 5 home page.
We’ll start with a simple version of using a Map property to receive your data from the request. Here’s the form markup from MapsDataTransferTest.jsp:
![]()
Again, the main difference between this and the List property version is that we now need to specify a key value, a string in this case. We’re using first names as keys to the incoming maiden names. As you can see, OGNL provides a couple of syntax options for specifying the key. First, you can use a simple, if somewhat misleading, property notation. Second, you can use a bracketed syntax that makes the fact that the property is a Map more evident. It doesn’t matter which you use, though you’ll probably find one or the other more flexible in certain situations. Just to prove that the syntax doesn’t matter, all of our fields in this example will submit to the same property, a Map going by the name of maidenNames. Since we haven’t specified a type for this Map with a type conversion properties file, all of the values will be converted into elements of type String. Similarly, our keys will also be treated as Strings.
With the OGNL expressions in place in the form elements, we just need a property on the Java side to receive the data. In this case, we have a Map-backed property implemented on the DataTransferTest.java action. Here’s the property that receives the data from the form: