concept method reference in category java
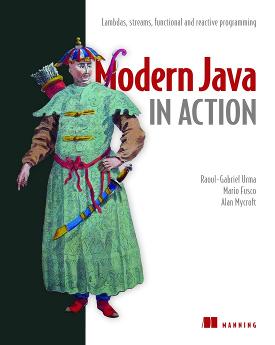
This is an excerpt from Manning's book Modern Java in Action: Lambdas, streams, reactive and functional programming.
The first new Java 8 feature we introduce is that of method references. Suppose you want to filter all the hidden files in a directory. You need to start writing a method that, given a File, will tell you whether it’s hidden. Fortunately, there’s such a method in the File class called isHidden. It can be viewed as a function that takes a File and returns a boolean. But to use it for filtering, you need to wrap it into a FileFilter object that you then pass to the File.listFiles method, as follows:
A method reference to an instance method of an existing object or expression (for example, suppose you have a local variable expensiveTransaction that holds an object of type Transaction, which supports an instance method getValue; you can write expensiveTransaction::getValue) The second and third kinds of method references may be a bit overwhelming at first. The idea with the second kind of method references, such as String::length, is that you’re referring to a method to an object that will be supplied as one of the parameters of the lambda. For example, the lambda expression (String s) -> s.toUpperCase() can be rewritten as String::toUpperCase. But the third kind of method reference refers to a situation when you’re calling a method in a lambda to an external object that already exists. For example, the lambda expression () -> expensiveTransaction.getValue() can be rewritten as expensiveTransaction::getValue. This third kind of method reference is particularly useful when you need to pass around a method defined as a private helper. For example, say you defined a helper method isValidName:
private boolean isValidName(String string) { return Character.isUpperCase(string.charAt(0)); }You can now pass this method around in the context of a Predicate<String> using a method reference:
Figure 3.5. Recipes for constructing method references for three different types of lambda expressions
![]()
Note that there are also special forms of method references for constructors, array constructors, and super-calls. Let’s apply method references in a concrete example. Say you’d like to sort a List of strings, ignoring case differences. The sort method on a List expects a Comparator as parameter. You saw earlier that Comparator describes a function descriptor with the signature (T, T) -> int. You can define a lambda expression that uses the method compareToIgnoreCase in the String class as follows (note that compareToIgnoreCase is predefined in the String class):
Note that the compiler goes through a similar type-checking process as for lambda expressions to figure out whether a method reference is valid with a given functional interface. The signature of the method reference has to match the type of the context.
To check your understanding of method references, do try quiz 3.6!
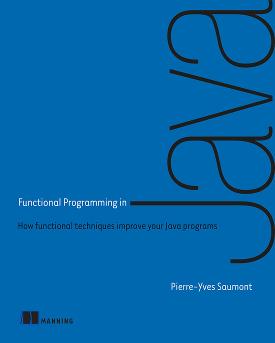
This is an excerpt from Manning's book Functional Programming in Java: How functional techniques improve your Java programs.
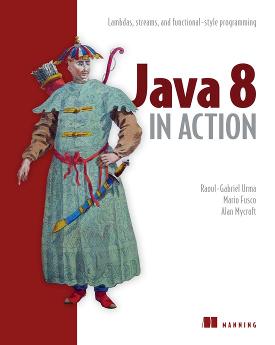
This is an excerpt from Manning's book Java 8 in Action: Lambdas, streams, and functional-style programming.
The first new Java 8 feature we introduce is that of method references. Suppose you want to filter all the hidden files in a directory. You need to start writing a method that given a File will tell you whether it’s hidden or not. Thankfully there’s such a method inside the File class called isHidden. It can be viewed as a function that takes a File and returns a boolean. But to use it for filtering you need to wrap it into a FileFilter object that you then pass to the File.listFiles method, as follows:
Why should you care about method references? Method references can be seen as shorthand for lambdas calling only a specific method. The basic idea is that if a lambda represents “call this method directly,” it’s best to refer to the method by name rather than by a description of how to call it. Indeed, a method reference lets you create a lambda expression from an existing method implementation. But by referring to a method name explicitly, your code can gain better readability. How does it work? When you need a method reference, the target reference is placed before the delimiter :: and the name of the method is provided after it. For example, Apple::getWeight is a method reference to the method getWeight defined in the Apple class. Remember that no brackets are needed because you’re not actually calling the method. The method reference is shorthand for the lambda expression (Apple a) -> a.getWeight(). Table 3.4 gives a couple more examples of possible method references in Java 8.
Table 3.4. Examples of lambdas and method reference equivalents
Lambda
Method reference equivalent
(Apple a) -> a.getWeight() Apple::getWeight () -> Thread.currentThread().dumpStack() Thread.currentThread()::dumpStack (str, i) -> str.substring(i) String::substring (String s) -> System.out.println(s) System.out::println You can think of method references as syntactic sugar for lambdas that refer only to a single method because you write less to express the same thing.
Figure 3.5. Recipes for constructing method references for three different types of lambda expressions
![]()
Note that there are also special forms of method references for constructors, array constructors, and super-calls. Let’s apply method references in a concrete example. Say you’d like to sort a List of strings, ignoring case differences. The sort method on a List expects a Comparator as parameter. You saw earlier that Comparator describes a function descriptor with the signature (T, T) -> int. You can define a lambda expression that leverages the method compareToIgnoreCase in the String class as follows (note that compareToIgnoreCase is predefined in the String class):