concept Mockito in category java
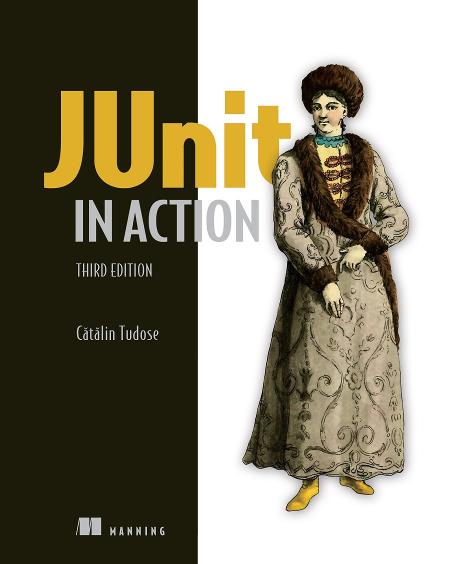
This is an excerpt from Manning's book JUnit in Action MEAP V06.
In practice, we can work with existing runners, such as the runners for the Spring framework or for mocking objects with Mockito (chapter 8). We consider as very useful to demonstrate the creation and usage of custom runners, as they reveal the principles of runners in general. We can extend the JUnit 4 abstract Runner class, override its methods and working with reflection. This is an approach that breaks the encapsulation but was the single possibility to add custom functionality to the JUnit 4 one. Revealing the shortcomings of working with custom runners in JUnit 4 opens the gate to understanding the capabilities and advantages of JUnit 5 extensions.
In this section, we will take a closer look at three of the most widely used mock frameworks: EasyMock, JMock, and Mockito.
8.6.3 Using Mockito
This section introduces Mockito (https://site.mockito.org), another popular mocking framework. The engineers at Tested Data Systems want to evaluate it and eventually introduce it into their projects.
To work with Mockito, you need to add to the pom.xml file the dependency shown in listing 8.24.
Listing 8.24 The Mockito dependency from the pom.xml configuration file
<dependency> <groupId>org.mockito</groupId> <artifactId>mockito-junit-jupiter</artifactId> <version>2.21.0</version> <scope>test</scope> </dependency>As with EasyMock and JMock, rework the example from listing 8.4 (testing money transfer with the help of a mock AccountManager), this time by means of Mockito, as shown in listing 8.25.
Listing 8.25 Reworking the TestAccountService test using Mockito
[...] import org.junit.jupiter.api.extension.ExtendWith; #A import org.mockito.Mock; #A import org.mockito.Mockito; #A import org.mockito.junit.jupiter.MockitoExtension; #A @ExtendWith(MockitoExtension.class) #B public class TestAccountServiceMockito { @Mock #C private AccountManager mockAccountManager; #C @Test public void testTransferOk() { Account senderAccount = new Account( "1", 200 ); #D Account beneficiaryAccount = new Account( "2", 100 ); #D Mockito.lenient() .when(mockAccountManager.findAccountForUser("1")) #E .thenReturn(senderAccount); #E Mockito.lenient() #E .when(mockAccountManager.findAccountForUser("2")) #E .thenReturn(beneficiaryAccount); #E AccountService accountService = new AccountService(); accountService.setAccountManager( mockAccountManager ); accountService.transfer( "1", "2", 50 ); #F assertEquals( 150, senderAccount.getBalance() ); #G assertEquals( 150, beneficiaryAccount.getBalance() ); #G } }
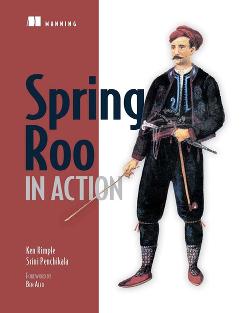
This is an excerpt from Manning's book Spring Roo in Action.
You should also pay close attention to mock object frameworks. They’re the best way to isolate your objects under test from other objects. Mockito is popular, but a lot of existing code is tested using EasyMock and JMock. Pick one mocking framework, as doing so makes it easy to read your tests without having to switch gears constantly.
For more information about the excellent Mockito mocking framework, visit the project website at http://mockito.org. You might also be interested in an API that layers on top of Mockito and its cousin EasyMock. Named PowerMock, this API allows you to mock static methods and implements a number of other helpful features. See the website at http://code.google.com/p/powermock.