concept parameter list in category java
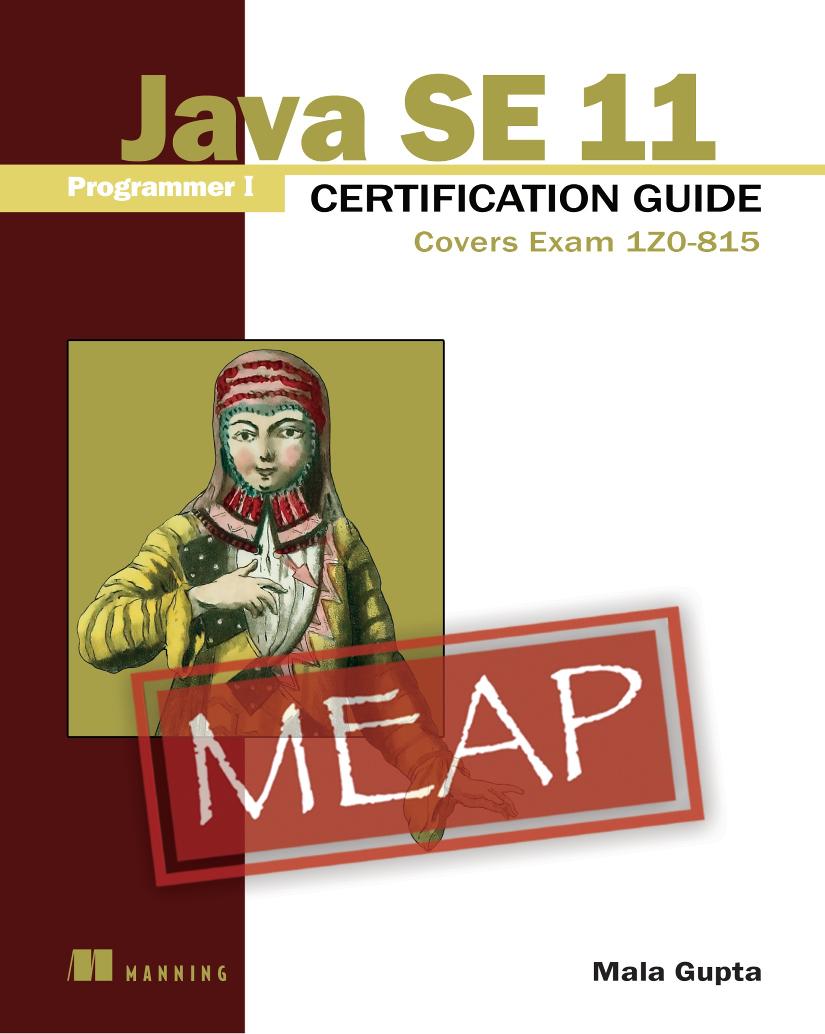
This is an excerpt from Manning's book Java SE 11 Programmer I Certification Guide MEAP V03.
If the parameter list of the called method defines a variable argument at the rightmost position, you can call the method with a variable number of arguments. Let’s add a method daysOffWork in the class Employee that accepts a variable list of arguments (modifications in bold):
You can define only one variable argument in a parameter list, and it must be the last variable in the parameter list. If you don’t comply with these two rules, your code won’t compile:
class Employee { public int daysOffWork(String... months, int... days) { #A int daysOff = 0; for (int i = 0; i < days.length; i++) daysOff += days[i]; return daysOff; } }If your method defines multiple method parameters, the variable that accepts variable arguments must be the last one in the parameter list:
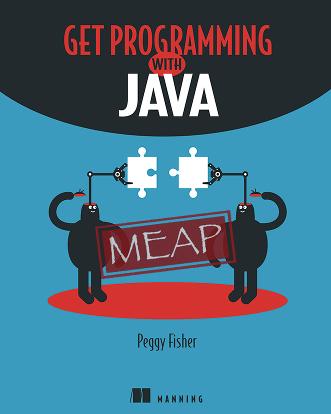
This is an excerpt from Manning's book Get Programming with Java MEAP V04 livebook.
In Java, a method signature paints a picture about how to use the method. The only required elements of a method signature are the method’s return type, the method name, parentheses for the parameter list (which can be empty), and the method body inside curly braces (which can also be empty).
In addition to these required elements, methods often include a visibility modifier, and a parameter list inside the parentheses. The keyword static is included when the method is not associated with a particular object, like the main method. Static methods are addressed later in the book. Figure 4.1 describes each part of a method signature, including required and optional components.
Figure 4.4 Diagram showing how the argument is passed to the method through the parameter list
![]()
This lesson reviewed how to write overloaded methods in Java. An overloaded method is useful when your class requires a method that can have different arguments in the parameter list. For code readability, it is helpful to use the same method name, but the parameter lists must be different. For example, a method used to calculate the average of three numbers might have one version that accepts all integer values and a second version that has all double values as arguments. In the next lesson, I introduce another topic related to methods, overriding methods.