concept reduce in category java
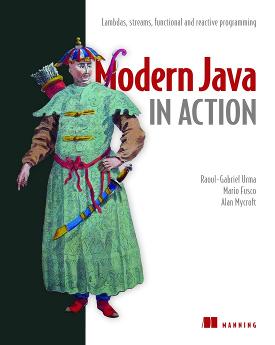
This is an excerpt from Manning's book Modern Java in Action: Lambdas, streams, reactive and functional programming.
But, for reasons centered on better exploiting parallelism, the designers didn’t do this. Java 8 instead contains a new Collection-like API called Stream, containing a comprehensive set of operations similar to the filter operation that functional programmers may be familiar with (for example, map and reduce), along with methods to convert between Collections and Streams, which we now investigate.
In this section, you’ll see how you can combine elements of a stream to express more complicated queries such as “Calculate the sum of all calories in the menu,” or “What is the highest calorie dish in the menu?” using the reduce operation. Such queries combine all the elements in the stream repeatedly to produce a single value such as an Integer. These queries can be classified as reduction operations (a stream is reduced to a value). In functional programming-language jargon, this is referred to as a fold because you can view this operation as repeatedly folding a long piece of paper (your stream) until it forms a small square, which is the result of the fold operation.
Before we investigate how to use the reduce method, it helps to first see how you’d sum the elements of a list of numbers using a for-each loop:
int sum = 0; for (int x : numbers) { sum += x; }Each element of numbers is combined iteratively with the addition operator to form a result. You reduce the list of numbers into one number by repeatedly using addition. There are two parameters in this code:
You could just as easily multiply all the elements by passing a different lambda, (a, b) -> a * b, to the reduce operation:
int product = numbers.stream().reduce(1, (a, b) -> a * b);Figure 5.7 illustrates how the reduce operation works on a stream: the lambda combines each element repeatedly until the stream containing the integers 4, 5, 3, 9, are reduced to a single value.
Let’s take an in-depth look into how the reduce operation happens to sum a stream of numbers. First, 0 is used as the first parameter of the lambda (a), and 4 is consumed from the stream and used as the second parameter (b). 0 + 4 produces 4, and it becomes the new accumulated value. Then the lambda is called again with the accumulated value and the next element of the stream, 5, which produces the new accumulated value, 9. Moving forward, the lambda is called again with the accumulated value and the next element, 3, which produces 12. Finally, the lambda is called with 12 and the last element of the stream, 9, which produces the final value, 21.
You can combine all elements of a stream iteratively to produce a result using the reduce method, for example, to calculate the sum or find the maximum of a stream. Some operations such as filter and map are stateless: they don’t store any state. Some operations such as reduce store state to calculate a value. Some operations such as sorted and distinct also store state because they need to buffer all the elements of a stream before returning a new stream. Such operations are called stateful operations.
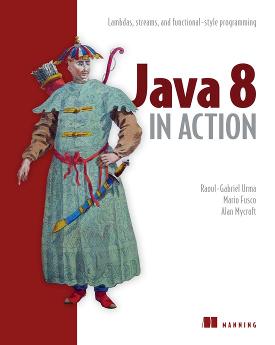
This is an excerpt from Manning's book Java 8 in Action: Lambdas, streams, and functional-style programming.
But, for reasons centered on better exploiting parallelism, the designers didn’t do this. Java 8 instead contains a whole new Collections-like API called Streams, containing a comprehensive set of operations similar to filter that functional programmers may be familiar with (for example, map, reduce), along with methods to convert between Collections and Streams, which we now investigate.
In this section, you’ll see how you can combine elements of a stream to express more complicated queries such as “Calculate the sum of all calories in the menu,” or “What is the highest calorie dish in the menu?” using the reduce operation. Such queries combine all the elements in the stream repeatedly to produce a single value such as an Integer. These queries can be classified as reduction operations (a stream is reduced to a value). In functional programming-language jargon, this is referred to as a fold because you can view this operation as repeatedly folding a long piece of paper (your stream) until it forms a small square, which is the result of the fold operation.
You could just as easily multiply all the elements by passing a different lambda, (a, b) -> a * b, to the reduce operation:
Figure 5.7 illustrates how the reduce operation works on a stream: the lambda combines each element repeatedly until the stream is reduced to a single value.
Let’s take an in-depth look into how the reduce operation happens to sum a stream of numbers. First, 0 is used as the first parameter of the lambda (a), and 4 is consumed from the stream and used as the second parameter (b). 0 + 4 produces 4, and it becomes the new accumulated value. Then the lambda is called again with the accumulated value and the next element of the stream, 5, which produces the new accumulated value, 9. Moving forward, the lambda is called again with the accumulated value and the next element, 3, which produces 12. Finally, the lambda is called with 12 and the last element of the stream, 9, which produces the final value, 21.
It turns out that reduction is all you need to compute maxima and minima as well! Let’s see how you can apply what you just learned about reduce to calculate the maximum or minimum element in a stream. As you saw, reduce takes two parameters:
You can solve this problem by mapping each element of a stream into the number 1 and then summing them using reduce! This is equivalent to counting in order the number of elements in the stream.
A chain of map and reduce is commonly known as the map-reduce pattern, made famous by Google’s use of it for web searching because it can be easily parallelized. Note that in chapter 4 you saw the built-in method count to count the number of elements in the stream: