concept static variable in category java
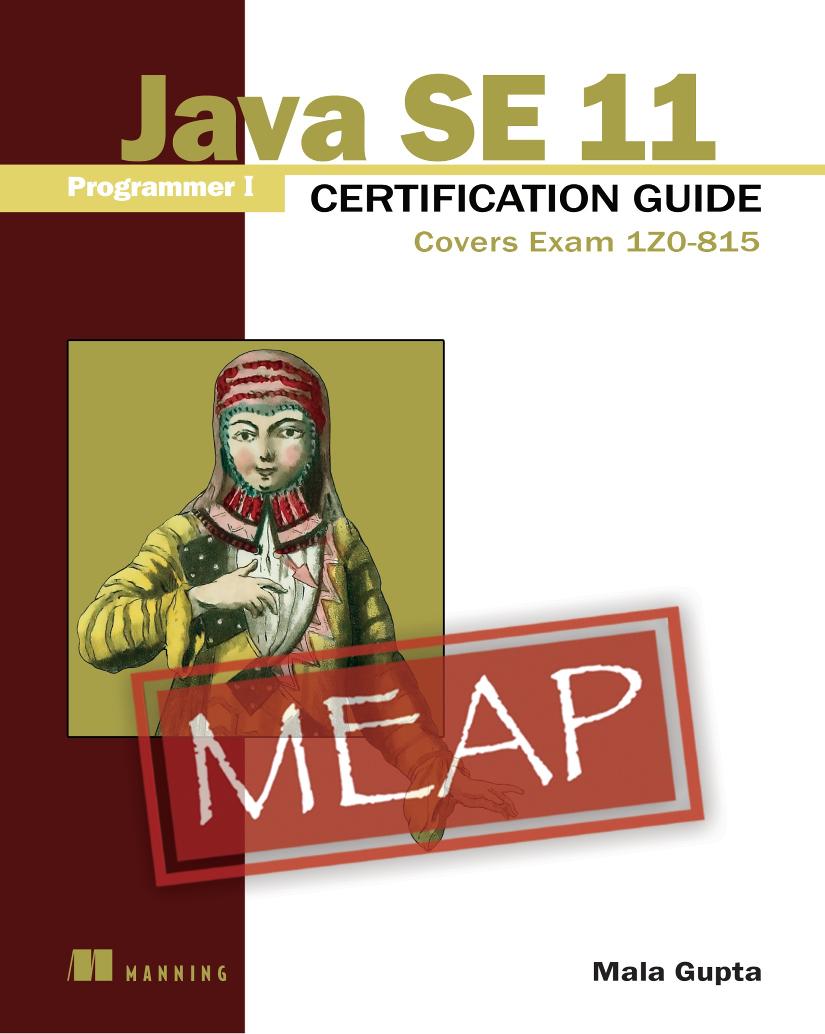
This is an excerpt from Manning's book Java SE 11 Programmer I Certification Guide MEAP V03.
A single copy of a class variable (also referred to as a static variable) is shared by all the objects of a class. The static variables are covered in chapter 8 with a detailed discussion of the nonaccess modifier static.
A lot of action can happen within an initializer block: It can create local variables. It can access and assign values to instance and static variables. It can call methods and define loops, conditional statements, and try-catch-finally blocks. Unlike constructors, an initializer block can’t accept method parameters.
static variables belong to a class. They’re common to all instances of a class and aren’t unique to any instance of a class. static attributes exist independently of any instances of a class and may be accessed even when no instances of the class have been created. You can compare a static variable with a shared variable. A static variable is shared by all the instances of a class.
Note A class and an interface can declare static variables. This section covers declaration and usage of static variables that are defined in a class. Chapter 6 covers interfaces and their static variables in detail.
Think of a static variable as being like a common bank vault that’s shared by the employees of an organization. Each of the employees accesses the same bank vault, so any change made by one employee is visible to all the other employees, as illustrated in figure 8.10.
Figure 8.10 Comparing a shared bank vault with a static variable
![]()
Because static variables and methods belong to a class and not to an instance, you can access them using variables, which are initialized to null. Watch out for such questions in the exam. Such code won’t throw a runtime exception (NullPointerException to be precise). In the following example, the reference variable emp is initialized to null:
class Emp { String name; static int bankVault; static int getBankVaultValue() { #A return bankVault; #A } #A } class Office { public static void main(String[] args) { Emp emp = null; System.out.println(emp.bankVault); #A System.out.println(emp.getBankVaultValue()); #A } }
Exam Tip You can access static variables and methods using a null reference.
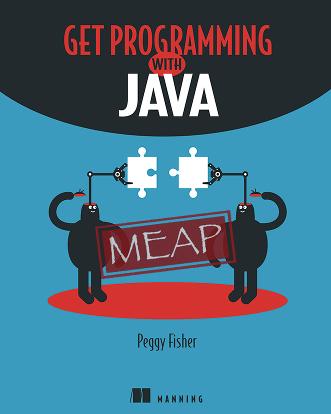
This is an excerpt from Manning's book Get Programming with Java MEAP V04 livebook.
Static variables are useful when the information is the same for all instances of a class. For example, if we had an Employee class and all employees worked for Linkedin, we could create a static variable for the company name in the Employee class that allocates space for that variable once, even if we have 1,000 employees. A static variable is shared across all instances of a class. Here is an example:
class Employee { String name; static String company = "Linkedin"; //other demographic information }Let’s revisit the TShirt class from the prior section to review the static variable nextItemNumber.
10.2 Example of a class with both static and non-static methods
1. public class TShirt { 2. static int nextItemNumber = 100; //#A 3. int itemNumber; 4. String size, color, graphic; 5. double price = 14.99; 6. static int assignNextNumber() { return nextItemNumber++; } //#B 7. void print() {System.out.println("TShirt number: "+itemNumber);} 8. public static void main(String[] args) { 9. TShirt tee1 = new TShirt(); 10. tee1.color="pink"; 11. tee1.graphic="Duke"; 12. tee1.itemNumber = assignNextNumber(); //#C 13. tee1.print(); 14. } 15. }Static variables are often used to either keep a running total of all objects or to provide values that are not dependent on a specific object. In our TShirt class, a variable is defined to hold the next item number. Each object has a unique item number. On line 2, I have declared a static variable called nextItemNumber and assigned it a starting value of 100. Since this variable is considered static, there is only one copy of this variable that is shared by all the TShirt objects. This number gets updated every time the static method assignNextNumber() is called. Notice that this method first returns the current number so that the calling TShirt object is assigned the next number, then the ++ is executed and one is added to the number so it is ready for the next TShirt.
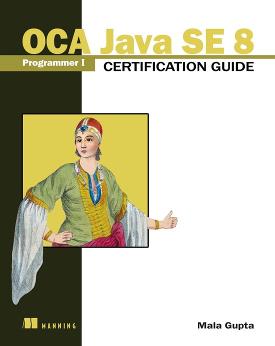
This is an excerpt from Manning's book OCA Java SE 8 Programmer I Certification Guide.
Revisit the definition of the class Phone in the previous example. Because the variables model, company, and weight are used to store the state of an object (also called an instance), they’re called instance variables or instance attributes. Each object has its own copy of the instance variables. If you change the value of an instance variable for an object, the value for the same named instance variable won’t change for another object. The instance variables are defined within a class but outside all methods in a class.
static variables
static variables belong to a class. They’re common to all instances of a class and aren’t unique to any instance of a class. static attributes exist independently of any instances of a class and may be accessed even when no instances of the class have been created. You can compare a static variable with a shared variable. A static variable is shared by all the objects of a class.
Note