concept stub in category java
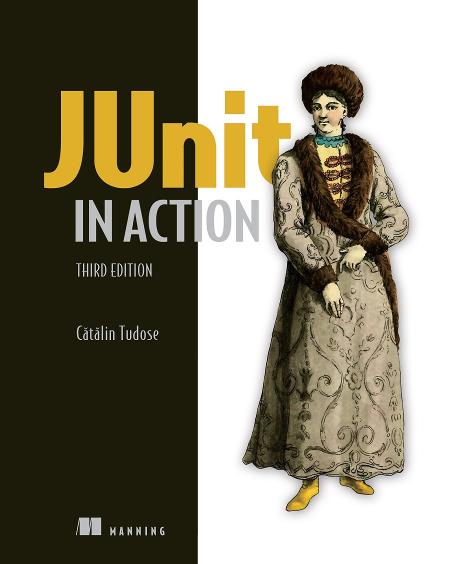
This is an excerpt from Manning's book JUnit in Action MEAP V06.
Chapter 7 discusses test granularity and introduces stubs: programs that simulate the behavior of software components that a module under test depends on.
Stubs are mechanisms for faking the behavior of real code or code that is not ready yet. In other words, stubs allow you to test a portion of a system when the other part is not available. Stubs usually do not change the code you are testing; instead, they adapt to provide seamless integration.
To demonstrate what stubs can do, we’ll explain how the engineers at Tested Data Systems Inc. build stubs for an application that opens an HTTP connection to a URL and reads its content. Figure 7.1 shows the sample application (limited to a WebClient.getContent method) opening an HTTP connection to a remote web resource. The remote web resource is a servlet, which generates an HTML response. The web resource in figure 7.2 is what we call the "real code" in the stub definition.
Figure 7.2 The sample application opens an HTTP connection to a remote web resource. The web resource is the real code in the stub definition.
![]()
The goal of the engineers at Tested Data Systems Inc. is to unit-test the getContent method by stubbing the remote web resource, as demonstrated in figure 7.3. The remote web resource is not yet available, and the engineers need to progress with their part of the work in the absence of that resource. They replace the servlet web resource with the stub—a simple HTML page that returns whatever they need for the TestWebClient test case. This approach allows them to test the getContent method independently of the implementation of the web resource (which in turn could call several other objects down the execution chain, possibly down to a database).
Figure 7.3 Adding a test case and replacing the real web resource with a stub
![]()
The important point to notice with stubbing is that getContent is not modified to accept the stub. The change is transparent to the application under test. For stubbing to be possible, the target code needs to have a well-defined interface and to allow different implementations (a stub, in this case) to be plugged in. In figure 7.1, the interface is actually the public abstract class java.net.URLConnection, which cleanly isolates the implementation of the page from its caller.
Following is an example of a stub in action, using the simple HTTP connection example. Listing 7.1 demonstrates a code snippet opening an HTTP connection to a given URL and reading the content at that URL. The method is one part of a bigger application to be unit-tested.
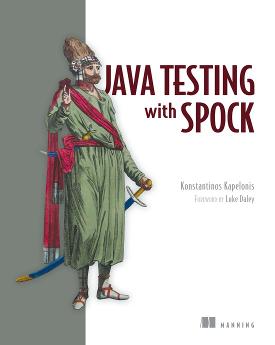
This is an excerpt from Manning's book Java Testing with Spock.
Mocks and stubs are a further subdivision of fake objects. Here are the definitions I first introduced in chapter 3:
A stub is a fake class that comes with preprogrammed return values. It’s injected into the class under test so that you have absolute control over what’s being tested as input. In figure 6.1, the fake sensor is a stub so that you can re-create any radiation levels you want.
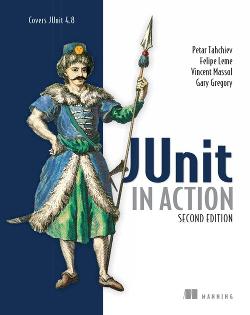
This is an excerpt from Manning's book JUnit in Action, Second Edition.
Beyond eliminating clutter, a separate-but-equal directory structure yields several other benefits. Right now, the only test class has the convenient Test prefix. Later you may need other helper classes to create more sophisticated tests. These might include stubs, mock objects, and other helpers. It may not be convenient to prefix all of these classes with Test, and it becomes harder to tell the domain classes from the test classes.
There are two strategies for providing these fake objects: stubbing and using mock objects. Stubs, the original solution, are still very popular, mostly because they allow you to test code without changing it to make it testable. This isn’t the case with mock objects. This chapter is dedicated to stubbing, whereas chapter 7 covers mock objects.
Our goal in this chapter is to unit test the getContent method by stubbing the remote web resource, as demonstrated in figure 6.2. You replace the servlet web resource with the stub, a simple HTML page returning whatever you need for the TestWebClient test case. This approach allows you to test the getContent method independently of the implementation of the web resource (which in turn could call several other objects down the execution chain, possibly down to a database).
The important point to notice with stubbing is that we didn’t modify getContent to accept the stub. The change is transparent to the application under test. In order to allow stubbing, the target code needs to have a well-defined interface and allow plugging in of different implementations (a stub, in our case). In the figure 6.1 example, the interface is the public abstract class java.net.URLConnection, which cleanly isolates the implementation of the page from its caller.
Let’s look at a stub in action using the simple HTTP connection example. Listing 6.1 from the example application demonstrates a code snippet opening an HTTP connection to a given URL and reading the content found at that URL. Imagine the method is one part of a bigger application that you want to unit test.
Stubs are a mechanism for faking the behavior of real code or code that isn’t ready yet. Stubs allow you to test a portion of a system even if the other part isn’t available. Stubs usually don’t change the code you’re testing but instead adapt to provide seamless integration.