concept sum in category java
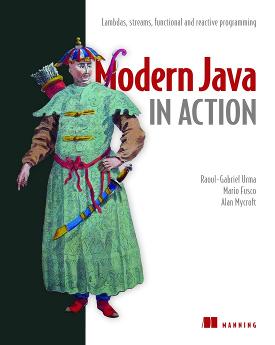
This is an excerpt from Manning's book Modern Java in Action: Lambdas, streams, reactive and functional programming.
The problem is that exploiting parallelism by writing multithreaded code (using the Threads API from previous versions of Java) is difficult. You have to think differently: threads can access and update shared variables at the same time. As a result, data could change unexpectedly if not coordinated[6] properly. This model is harder to think about[7] than a step-by-step sequential model. For example, figure 1.5 shows a possible problem with two threads trying to add a number to a shared variable sum if they’re not synchronized properly.
Why does it return an Optional<Integer>? Consider the case when the stream contains no elements. The reduce operation can’t return a sum because it doesn’t have an initial value. This is why the result is wrapped in an Optional object to indicate that the sum may be absent. Now see what else you can do with reduce.
You saw earlier that you could use the reduce method to calculate the sum of the elements of a stream. For example, you can calculate the number of calories in the menu as follows:
int calories = menu.stream() .map(Dish::getCalories) .reduce(0, Integer::sum);The problem with this code is that there’s an insidious boxing cost. Behind the scenes each Integer needs to be unboxed to a primitive before performing the summation. In addition, wouldn’t it be nicer if you could call a sum method directly as follows?
int calories = menu.stream() .map(Dish::getCalories) .sum(); !@%STYLE%@! {"css":"{\"css\": \"font-weight: bold;\"}","target":"[[{\"line\":2,\"ch\":19},{\"line\":2,\"ch\":26}]]"} !@%STYLE%@!But this isn’t possible. The problem is that the method map generates a Stream<T>. Even though the elements of the stream are of type Integer, the streams interface doesn’t define a sum method. Why not? Say you had only a Stream<Dish> like the menu; it wouldn’t make any sense to be able to sum dishes. But don’t worry; the Streams API also supplies primitive stream specializations that support specialized methods to work with streams of numbers.
You can combine all elements of a stream iteratively to produce a result using the reduce method, for example, to calculate the sum or find the maximum of a stream.
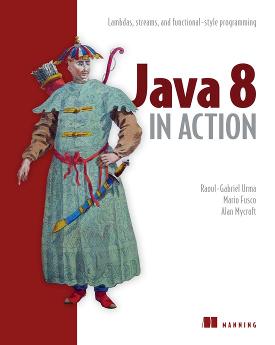
This is an excerpt from Manning's book Java 8 in Action: Lambdas, streams, and functional-style programming.
The problem is that exploiting parallelism by writing multithreaded code (using the Threads API from previous versions of Java) is difficult. You have to think differently: threads can access and update shared variables at the same time. As a result, data could change unexpectedly if not coordinated[6] properly. This model is harder to think about[7] than a step-by-step sequential model. For example, figure 1.5 shows a possible problem with two Threads trying to add a number to a shared variable sum if they’re not synchronized properly.
Why does it return an Optional<Integer>? Consider the case when the stream contains no elements. The reduce operation can’t return a sum because it doesn’t have an initial value. This is why the result is wrapped in an Optional object to indicate that the sum may be absent. Now see what else you can do with reduce.
You saw earlier that you could use the reduce method to calculate the sum of the elements of a stream. For example, you can calculate the number of calories in the menu as follows:
The problem with this code is that there’s an insidious boxing cost. Behind the scenes each Integer needs to be unboxed to a primitive before performing the summation. In addition, wouldn’t it be nicer if you could call a sum method directly as follows?
But this isn’t possible. The problem is that the method map generates a Stream<T>. Even though the elements of the stream are of type Integer, the Streams interface doesn’t define a sum method. Why not? Say you had only a Stream<Dish> like the menu; it wouldn’t make any sense to be able to sum dishes. But don’t worry; the Streams API also supplies primitive stream specializations that support specialized methods to work with streams of numbers.
The benefit of using reduce compared to the step-by-step iteration summation that you wrote earlier is that the iteration is abstracted using internal iteration, which enables the internal implementation to choose to perform the reduce operation in parallel. The iterative summation example involves shared updates to a sum variable, which doesn’t parallelize gracefully. If you add in the needed synchronization, you’ll likely discover that thread contention robs you of all the performance that parallelism was supposed to give you! Parallelizing this computation requires a different approach: partition the input, sum the partitions, and combine the sums. But now the code is starting to look really different. You’ll see what this looks like in chapter 7 using the fork/join framework. But for now it’s important to realize that the mutable accumulator pattern is a dead end for parallelization. You need a new pattern, and this is what reduce provides you. You’ll also see in chapter 7 that to sum all the elements in parallel using streams, there’s almost no modification to your code: stream() becomes parallelStream():