concept validation framework in category java
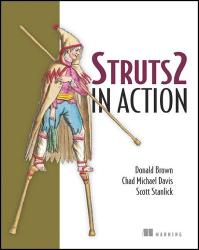
This is an excerpt from Manning's book Struts 2 in Action.
We’ve already shown one basic form of validation offered by Struts 2. To recap that technique, the Validateable interface, as we’ve seen, provides a programmatic validation mechanism; you put the validation code into your action’s validate() method and it’ll be executed by the workflow interceptor. The validation interceptor, on the other hand, is part of the Struts 2 validation framework and provides a declarative means to validate your data. Rather than writing validation code, the validation framework allows you to use both XML files and Java annotations to describe the validation rules for your data. Since the validation framework is such a rich topic, chapter 10 is dedicated to it.
For now, we should note that the validation interceptor, like the Validateable interface, works in tandem with the workflow interceptor. Recall that the workflow interceptor calls the validate() method of the Validateable interface to execute validation code before it checks for validation errors. In the case of the validation framework, the validation interceptor itself executes the validation logic. The validation interceptor is the entry point into the validation framework’s processing. When the validation framework does its work, it’ll store validation errors using the same ValidationAware methods that your handwritten validate() code does. When the workflow interceptor checks for error messages, it doesn’t know whether they were created by the validation framework or the validation code invoked through the Validateable interface. In fact, it doesn’t matter. The only thing that really matters is that the validation interceptor fires before the workflow interceptor, and this sequencing is handled by the defaultStack. You could even use both methods of validation if you liked. Either way, if errors are found, the workflow interceptor will divert workflow back to the input page.
The validation framework provides a more versatile and maintainable solution to validation than the Validateable interface. Throughout this chapter, we explore the components of the validation framework and learn how easy it is to work with this robust validation mechanism. We demonstrate this in code by migrating the Struts 2 Portfolio to use the validation framework instead of the Validateable interface mechanism. This example shows you how to wire up your actions for validation as well as demonstrates the use cases of the more common built-in validators. During this process, you’ll learn all you need to leverage this advanced validation tool in your own projects.
One of the stronger points of the validation framework is the Validator, a reusable component in which the logic of specific types of validations are implemented. Some of the built-in validators handle such validations as checking whether a given string represents a valid email address or whether a date falls within a given range. We also demonstrate the extensible nature of the Validators by implementing a custom Validator for use in the Struts 2 Portfolio. We even point out some advanced nuances and techniques before we wind things up.
But let’s get started by exploring the basic architecture of the validation framework. As you’ll soon see, the learning curve is gentle.
The only other thing you need to know about the built-in validators is the location of their declarations. The validation framework is actually a part of a low-level framework, upon which Struts 2 has been built, called Xwork. You don’t need to know much about Xwork to use Struts 2, but if you like you can visit the project home page at http://www.opensymphony.com/xwork. The only reason we mention it now is to show you where the validators of the validation framework are defined. If you look in the XWork JAR file, something like xwork-2.0.4.jar, you can find an XML file that declares all these built-in validators, located at /com/opensymphony/xwork2/ validator/validators/default.xml. When we build a custom validator in the next section, we see how to properly add new validators to this declaration.