concept class in category javascript
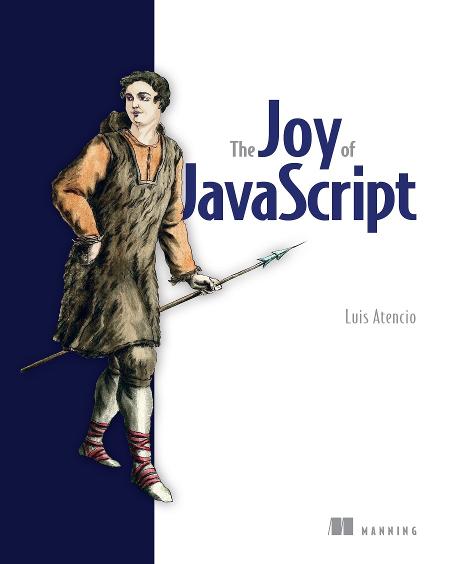
This is an excerpt from Manning's book The Joy of JavaScript MEAP V08.
The details of each technique will be explained in the chapters to come. As you progress through each one, the goal is to move the needle toward loosely coupled object composition whenever possible over tightly coupled object linkage. The reason for showing the spectrum of alternatives is not only to showcase the versatility of JavaScript’s object model, but also provide you different alternatives when classes are not desirable and you want finer-grained control to reuse behavior from multiple objects, rather than from a single monolithic parent class. Often, that’s the only thing you have, and it’s tempting to treat everything as it were a nail when all you have is a hammer. JavaScript gives you different types of hammers for different types of nails.
Figure 2.1 In this chapter, you’ll learn that function constructors are used to emulate a classical style of object-oriented programming. Classes act as the blueprints from which you can instantiate objects, which connect to their prototypes using an internal property resolution mechanism in the JavaScript engine.
![]()
Saving you from making the same mistake again. However, if you read the documentation, you’ll find that the Node.js community discourages this in favor of using class and extends indicating these are “semantically incompatible.” You don’t say! Previously I had briefly alluded to prototypes and classes being incompatible. The next section evaluates this in detail.
This idea of using a constructor function with new to create new instances is what we know today as the pseudo-classical model. With the advent of ECMAScript 2015, this has been largely replaced by a better, more familiar classical model that also addressed this awkward bit of syntax. In fact, with classes, forgetting to write new when invoking a constructor now generates a clear error. For example, for a Transaction class:
const tx = Transaction(...) TypeError: Class constructor Transaction cannot be invoked without 'new'Let’s explore the advantages of classes as well as some of its newer proposals.
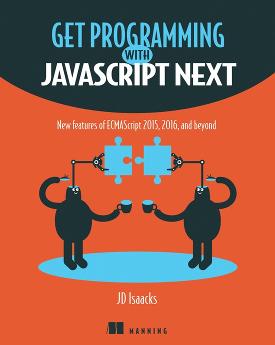
This is an excerpt from Manning's book Get Programming with JavaScript Next: New features of ECMAScript 2015, 2016, and beyond.
ECMAScript Fourth Edition (ES4) was intended to be a radical change. It introduced many new concepts, including classes and interfaces, and was statically typed. It also wasn’t backwards-compatible with ES3. This meant that if implemented, it had the potential to break existing JavaScript applications in the wild. Needless to say, ES4 was controversial and split the Ecma technical committee, resulting in a subcommittee formed to work on a much smaller update dubbed ECMAScript 3.1. ECMAScript Fourth Edition was eventually abandoned and ECMAScript 3.1 was renamed to ES5 (Fifth Edition) and published in 2009.
Classes are little more than syntactic sugar for declaring a constructor function and setting its prototype. Even with the introduction of classes, JavaScript isn’t statically or strongly typed. It remains a dynamically and weakly typed language. However, classes do create a simple self-contained syntax for defining constructors with prototypes. The main advantage over constructors, though, besides syntax, is when extending classes, which we’ll get to in the next lesson. Without having an easily extendable built-in construct such as classes, many libraries like Backbone.js, React.js, and several others had to continue to reinvent the wheel to allow extending their base objects. This library-specific form of extending objects will become a thing of the past as more and more libraries start to provide a base JavaScript class that can easily be extended. This means once you learn how to use and extend classes, you’ll have a jump start on many of today’s and tomorrow’s frameworks.
Static properties on classes are a special type of property that doesn’t set a property on the instance or even the prototype, but on the class object (the constructor) itself. Static properties make sense for properties that won’t change across instances. For example, your DataStore could potentially have a static property for what domain to use when connecting to APIs:
Static properties are just syntactic sugar for assigning them directly on the classes themselves, as shown in the next listing.
That sums up creating and using classes, but we aren’t done with classes yet. In the next lesson we’ll take a look at extending classes.
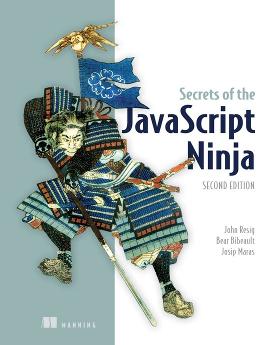
This is an excerpt from Manning's book Secrets of the JavaScript Ninja, Second Edition.
The function context is a notion that those coming from object-oriented languages such as Java might think that they understand. In such languages, this usually points to an instance of the class within which the method is defined.
ES6 introduces a new class keyword that provides a much more elegant way of creating objects and implementing inheritance than manually implementing it ourselves with prototypes. Using the class keyword is easy, as shown in the following listing.
Listing 7.13 shows that we can create a Ninja class by using the class keyword. When creating ES6 classes, we can explicitly define a constructor function that will be invoked when instantiating a Ninja instance. In the constructor’s body, we can access the newly created instance with the this keyword, and we can easily add new properties, such as the name property. Within the class body, we can also define methods that will be accessible to all Ninja instances. In this case, we’ve defined a swingSword method that returns true:
As mentioned earlier, even though ES6 has introduced the class keyword, under the hood we’re still dealing with good old prototypes; classes are syntactic sugar designed to make our lives a bit easier when mimicking classes in JavaScript.
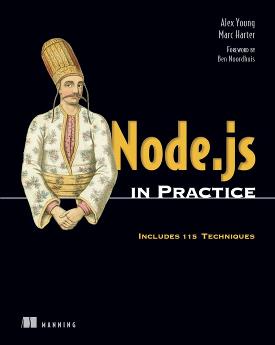
This is an excerpt from Manning's book Node.js in Practice.
You can inherit from EventEmitter to make your own event-based APIs. Let’s say you’re working on a PayPal payment-processing module. You could make it event-based, so instances of Payment objects emit events like paid and refund. By designing the class this way, you decouple it from your application logic, so you can reuse it in more than one project.
Now Node has reached a point where the core developers have seen the types of problems people are tackling with streams, so the new API is richer thanks to the new stream primitive classes. Table 5.1 shows a summary of the classes available from Node 0.10 onward.
Table 5.1. A summary of the classes available in streams2
Name
User methods
Description
stream.Readable _read(size) Used for I/O sources that generate data stream.Writable _write(chunk, encoding, callback) Used to write to an underlying output destination stream.Duplex _read(size), _write(chunk, encoding, callback) A readable and writable stream, like a network connection stream.Transform _flush(size), _transform(chunk, encoding, callback) A duplex stream that changes data in some way, with no limitation on matching input data size with the output
Built-in streams and the classes used to build custom streams allow the internal buffer size to be configured. It’s useful to know how to optimize this value to attain the desired performance characteristics.