concept Fabric8 Java client in category kubernetes
appears as: Fabric8 Java client
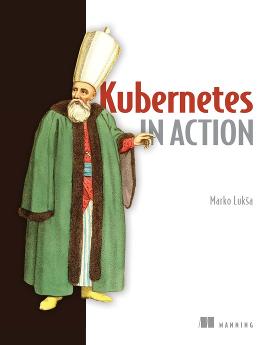
This is an excerpt from Manning's book Kubernetes in Action.
Listing 8.17. Listing, creating, updating, and deleting pods with the Fabric8 Java client
import java.util.Arrays; import io.fabric8.kubernetes.api.model.Pod; import io.fabric8.kubernetes.api.model.PodList; import io.fabric8.kubernetes.client.DefaultKubernetesClient; import io.fabric8.kubernetes.client.KubernetesClient; public class Test { public static void main(String[] args) throws Exception { KubernetesClient client = new DefaultKubernetesClient(); // list pods in the default namespace PodList pods = client.pods().inNamespace("default").list(); pods.getItems().stream() .forEach(s -> System.out.println("Found pod: " + s.getMetadata().getName())); // create a pod System.out.println("Creating a pod"); Pod pod = client.pods().inNamespace("default") .createNew() .withNewMetadata() .withName("programmatically-created-pod") .endMetadata() .withNewSpec() .addNewContainer() .withName("main") .withImage("busybox") .withCommand(Arrays.asList("sleep", "99999")) .endContainer() .endSpec() .done(); System.out.println("Created pod: " + pod); // edit the pod (add a label to it) client.pods().inNamespace("default") .withName("programmatically-created-pod") .edit() .editMetadata() .addToLabels("foo", "bar") .endMetadata() .done(); System.out.println("Added label foo=bar to pod"); System.out.println("Waiting 1 minute before deleting pod..."); Thread.sleep(60000); // delete the pod client.pods().inNamespace("default") .withName("programmatically-created-pod") .delete(); System.out.println("Deleted the pod"); } }