concept XML Script in category .net
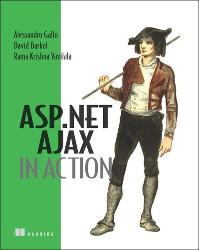
This is an excerpt from Manning's book ASP.NET AJAX in Action.
ASP.NET AJAX-enabled web pages are written in HTML, JavaScript, and a new XML-based, declarative syntax called XML Script. This provides you with more than one option for authoring client-side code—you can code declaratively with XML Script and imperatively with JavaScript. Elements declared in XML Script are contained in a new script tag:
XML Script is a declarative language for creating instances of JavaScript objects at runtime, setting their properties, and specifying their behavior, using an XML-like syntax similar to the ASP.NET markup code.
In an HTML page, you can separate content (the markup code) from style by embedding the style information in a CSS file. Similarly, in an ASP.NET page, you usually define the page layout using declarative markup code in an ASPX page. Then, you can use a separate code-behind file to specify the behavior of server controls and how they’re wired together, using the classic imperative syntax. XML Script lets you achieve this kind of separation and instantiate JavaScript components using a declarative script language embedded in a web page.
XML Script, like declarative languages, has a number of advantages over the imperative syntax. Building designers for markup is easier than building them for code. Great visual tools, like the Visual Studio Designer, take care of generating markup code for you. If a client can parse declarative markup, you can make server controls render the markup more easily than rendering imperative code. In addition, declarative markup carries semantics. For example, an application that parses a TextBox tag knows that it has to instantiate a text field, but it’s up to the application to decide to instantiate a simple text field rather than a more complex auto-complete text box—for example, based on browser capabilities. Finally, declarative code can be more expressive and less verbose than imperative code. Features like bindings help keep the values exposed by object properties synchronized, without the need to deal with multiple event handlers.
This chapter illustrates these aspects of XML Script, beginning with the basics of the language and moving to advanced features like actions, bindings, and transformers. Keep in mind that because they’re part of the ASP.NET Futures package, the features illustrated in this chapter aren’t currently documented or supported by Microsoft.
Before you begin using XML Script, you need to enable it in a web page. This turns out to be an easy job, because you have to reference the PreviewScript.js file in the ScriptManager control, as shown in listing 11.1. This file is embedded as a web resource in the Microsoft.Web.Preview assembly, which is shipped with the ASP.NET Futures package. You can find more information on how to install this package in appendix A.
If the class exists, the parser checks whether it exposes a static method called parseFromMarkup. This method must be defined in a class in order to be used in XML Script. It receives the markup code and is responsible for parsing it and creating a new instance of the class. This process is repeated for each XML Script block and for each tag extracted from the components node. When all the markup has been processed, all the client objects have been instantiated and can be safely accessed in the application code. This process is illustrated in figure 11.2.
Figure 11.2. The XML Script parser extracts component declarations from XML Script code blocks. Then, it parses the declarative code and creates instances of the corresponding JavaScript objects.
![]()
Luckily, you can avoid writing the logic needed to parse the markup code and create an instance of a client class. The Sys.Component class exposes and implements the parseFromMarkup method; it’s a good choice to derive the custom classes from the base Sys.Component class. This way, you can take advantage of the features offered by the client component model and also use the custom class in XML Script with little effort. The only requirement is that every component must expose a type descriptor in order to be used in XML Script.