concept module in category node
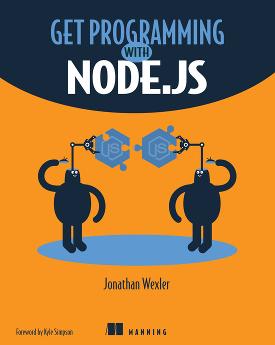
This is an excerpt from Manning's book Get Programming with Node.js.
In the REPL environment, you have access to all the core modules that come with Node.js. Core modules are JavaScript files that come with your Node.js installation. I talk more about modules in unit 1. You’ll soon see in your own custom applications that you need to import some modules to use them in REPL. For a short list of commands to use in REPL, see table 1.1.
The exports object is a property of the module object. module is both the name of the code files in Node.js and one of its global objects. exports is shorthand for module .exports.
The module is ready to be required (imported) by another JavaScript file. You can test this module by creating another file called printMessages.js, the purpose of which is to loop through the messages and log them to your console with the code shown in the next listing. First, require the local module by using the require object and the module’s filename (with or without the .js extension). Then refer to the module’s array by the variable set up in printMessages.js, as shown in the next listing.
Listing 3.1. Log messages to console in printMessages.js
const messageModule = require("./messages"); #1 messageModule.messages.forEach(m => console.log(m)); #2require is another Node.js global object used to locally introduce methods and objects from other modules. Node.js interprets require(“./messages”) to look for a module called messages.js within your project directory and allows code within printMessages.js to use any properties on the exports object in messages.js.
Every Node.js application or module contains a package.json file to define the properties of that project. This file lives at the root level of your project. Typically, this file is where you specify the version of your current release, the name of your application, and the main application file. This file is important for npm to save any packages to the node community online.
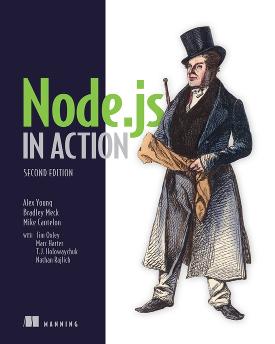
This is an excerpt from Manning's book Node.js in Action, Second Edition.
If you open package.json, you’ll see a simple JSON file that describes your project. If you now install a module from www.npmjs.com and use the --save option, npm will automatically update your package.json file. Try it out by typing npm install, or npm i for short:
If you open your package.json file, you should see express added under the dependencies property. Also, if you look inside the node_modules folder, you’ll see an express directory. This contains the version of Express that you just installed. You can also install modules globally by using the --global option. You should use local modules as much as possible, but global modules can be useful for command-line tools that you want to use outside Node JavaScript code. An example of a command-line tool that’s installable with npm is ESLint (http://eslint.org/).
Modules can be either single files or directories containing one or more files, as you can see in figure 2.3. If a module is a directory, the file in the module directory that will be evaluated is typically named index.js (although this can be overridden: see section 2.5).
Requiring a module that begins with ./ means that if you were to create your application script named test-currency.js in a directory named currency_app, then your currency.js module file, as represented in figure 2.4, would also need to exist in the currency_app directory. When requiring, the .js extension is assumed, so you can omit it if desired. If you don’t include .js, Node will also check for a .json file. JSON files are loaded as JavaScript objects.
Figure 2.4. When you put ./ at the beginning of a module require, Node will look in the same directory as the program file being executed.
![]()
After Node has located and evaluated your module, the require function returns the contents of the exports object defined in the module. You’re then able to use the two functions returned by the module to perform currency conversion.
If you want to organize related modules, you can put modules into subdirectories. If, for example, you want to put the currency module in a folder called lib/, you can do so by changing the line with require to the following:
Populating the exports object of a module gives you a simple way to group reusable code in separate files.
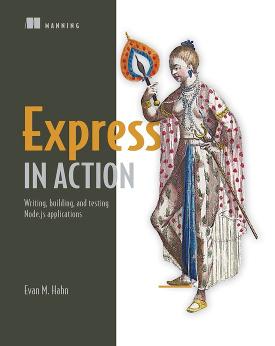
This is an excerpt from Manning's book Express in Action: Writing, building, and testing Node.js applications.
Node has a number of built-in modules, ranging from filesystem access in a module called fs to utility functions in a built-in module called util.
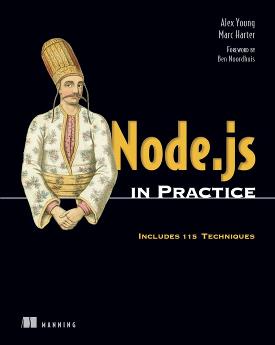
This is an excerpt from Manning's book Node.js in Practice.
In figure 1.1, a new HTTP request has been received and parsed by Node’s http module
. The ad server’s application code then makes a database query, using an asynchronous API—a callback passed to a database read function
. While Node waits for this to finish, the ad server is able to read a template file from the disk
. This template will be used to display a suitable web page. Once the database request has finished, the template and database results are used to render the response
.
Node’s require system (based on CommonJS; http://wiki.commonjs.org/wiki/Modules/1.1) manages those dependencies in a way that avoids dependency hell. It’s perfectly fine for modules to depend on different versions of the same module, as shown in figure 13.1.