concept foreach in category powershell
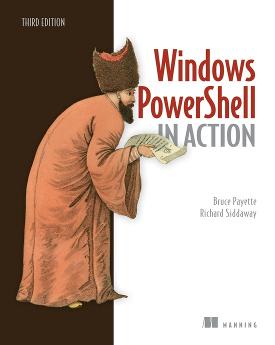
This is an excerpt from Manning's book Windows PowerShell in Action, Third Edition.
Pipelines are great, but sometimes you need more control over the flow of your script. PowerShell has the usual flow-control statements found in most programming languages. These include the basic if statements, a powerful switch statement, and loops like while, for and foreach, and so on. Here’s an example showing the while and if statements:
This example uses the while loop to count through a range of numbers, printing only the odd numbers. In the body of the while loop is an if statement that tests to see whether the current number is odd, and then writes a message if it is. You can do the same thing using the foreach statement and the range operator (..), but much more succinctly:
The foreach statement iterates over a collection of objects, and the range operator is a way to generate a sequence of numbers. The two combine to make looping over a sequence of numbers a very clean operation.
When foreach is the first word in a statement, it’s a keyword; otherwise it’s the name of a command.
Now let’s look at the second alias. Even though foreach is significantly shorter than ForEach-Object, there have still been times when users wanted it to be even shorter.
The whole point of using a scripting language for automation is so you can operate on more than one object at a time. PowerShell provides many ways of operating on collections. Perhaps the most straightforward of these mechanisms is the foreach loop.
To reiterate, when the word “foreach” is used at the beginning of a statement, it’s recognized as the foreach keyword. When it appears in the middle of a pipeline, it’s treated as the name of a command (ForEach-Object). When used as a method name, it’s treated as the fallback .foreach() method. Of all the looping constructs, the foreach construct will be the one you use most often.
This statement is syntactically identical to the C# foreach loop except that you don’t, and can’t, declare the type of the loop variable. This example loops over all the text files (.txt extension) in the current directory, calculating the total size of all the files:
First you set the variable that will hold the total length to 0. Then, in the foreach loop, you use the Get-ChildItem command to get a list of the text files in the current directory. The foreach statement assigns elements from this list one at a time to the loop variable $f and then executes the statement list with this variable set. At the end of the statement, $f will retain the last value that was assigned to it, which is the last value in the list. Compare this example to the for loop examples in the previous section. Because you don’t have to manually deal with the loop counter and explicit indexing, this example is significantly simpler.
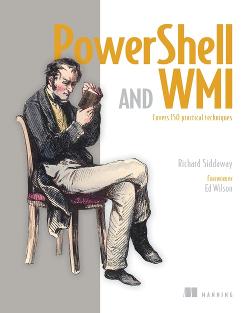
This is an excerpt from Manning's book PowerShell and WMI.
ForEach-Object aliased as foreach but never as %
The code snippet in the previous section showed an example of using select, sort, and group. Their purposes are obvious from their names. Of the other utility cmdlets, where and foreach will be used the most.
You use a loop when you want to perform the same action a number of times. You’ve already seen one type of loop, in the shape of the ForEach-Object cmdlet, in section 2.2.1. There’s also a foreach loop using a PowerShell keyword, and there are the usual for, do, and while types of loop structures. Foreach is the most confusing, so we’ll get that one settled first.
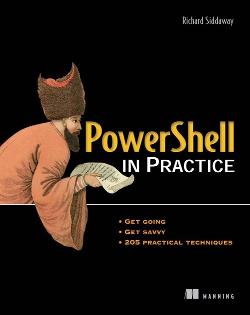
This is an excerpt from Manning's book PowerShell in Practice.
I don’t particularly like % and ? as aliases of foreach and where, respectively. They make scripts harder to read for people new to PowerShell, so I tend to avoid using them. In this chapter, I’ll mix and match the full name and alias for the *-Object cmdlets and only use the alias in subsequent chapters.
The output of Get-ChildItem (a listing of the files in the c:\temp directory) is stored in the variable $files
. $files is a collection of objects (array), so we can iterate through them using a foreach loop
. The switch statement uses a variable, in this case the file length property
, as its test. We define a number of tests in this case:
They serve slightly different purposes; I’ll explain when it’s best to use each one as we go along. When using the foreach, do, for, or while loop, you can use the break command to jump out of the loop and the continue command to skip processing the script block and return to the start of the loop. Details can be found in the about_break and about_continue help files.
One important difference is that Foreach-Object will process objects as they come along the pipeline, but a foreach loop needs the objects to be already available in a collection. Testing has suggested that in PowerShell v1 a foreach loop is faster than using the cmdlet version, though the gap has narrowed in v2. If you have a huge number of objects, the cmdlet’s ability to process the objects as presented will be a bonus. Listing 2.8 shows how to use a foreach statement to iterate through a set of files and remove files older than a certain date. This is a common technique for cleaning up sets of data that are created periodically.