concept coordinate in category python
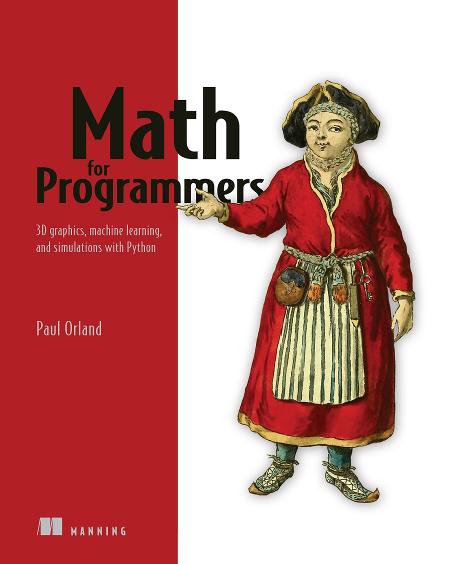
This is an excerpt from Manning's book Math for Programmers: 3D graphics, machine learning, and simulations with Python MEAP V11.
The coordinates of v − w are the differences of the coordinates v and w. In figure 2.21, v = (-1, 3) and w = (2, 2). The difference for v − w has the coordinates (-1 − 2, 3 − 2) = (-3, 1).
In 3D, vector addition can still be accomplished by adding coordinates. The vectors (2, 1, 1) and (1, 2, 2) sum to (2+1, 1+2, 1+2) = (3, 3, 3). We can start at the origin and place the two input vectors tip-to-tail in either order to get to the sum point (3, 3, 3) (figure 3.13).
Figure 3.13: Two visual examples of vector addition in 3D
![]()
As in 2D, we can add any number of 3D vectors together by summing all of their x-coordinates, all of their y-coordinates, and all of their z-coordinates. These three sums give us the coordinates of the new vector. For instance, in the sum (1, 1, 3) + (2, 4, -4) + (4, 2, -2), the respective x-coordinates are 1, 2, and 4, which sum to 7. The y-coordinates sum to 7 as well, and the z-coordinates sum to -3; therefore, the vector sum is (7, 7, -3). Tip-to-tail, the three vectors look like those in figure 3.14.
Exercise 3.22: What are the coordinates of the cross product of (1, -2, 1) and (-6, 12, -6)?
from abc import abstractproperty from vectors import add, scale class CoordinateVector(Vector): @abstractproperty def dimension(self): pass def __init__(self,*coordinates): self.coordinates = tuple(x for x in coordinates) def add(self,other): return self.__class__(*add(self.coordinates, other.coordinates)) def scale(self,scalar): return self.__class__(*scale(scalar, self.coordinates)) def __repr__(self): return "{}{}".format(self.__class__.__qualname__, self.coordinates)
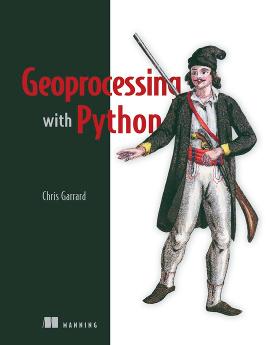
This is an excerpt from Manning's book Geoprocessing with Python.
You can imagine many other examples of geographic data that lend themselves to being represented this way. Anything that can be described with a single set of coordinates, such as latitude and longitude, can be represented as a point. This includes cities, restaurants, mountain peaks, weather stations, and geocache locations. In addition to their x and y coordinates (such as latitude and longitude), points can have a third z coordinate that represents elevation.
Most people are familiar with the concept of using latitude and longitude to specify a location on the earth’s surface. Would you be surprised to learn that many other coordinate systems are also used, and that these different spatial reference systems are used for different purposes? To make things even more complicated, the earth isn’t a perfect sphere, and multiple models, called datums, are used to represent the planet’s shape. Given this, coordinates from any system, including latitude and longitude, aren’t absolute—a set of coordinates can specify a slightly different location depending on the datum used.
There are a couple of different ways to convert coordinates between spatial reference systems using pyproj. You can use the Proj class to convert between geographic and projected coordinates or the module-level transform function to convert between two spatial reference systems. Let’s start with converting the Eiffel Tower coordinates from latitude and longitude to UTM Zone 31N. The first thing to do is initialize a Proj object with the UTM spatial reference system using a PROJ.4 string, and then use that to transform the coordinates. The syntax might look a bit odd to you, because you don’t need to call a specific function on the Proj object to do the conversion: