concept derivative in category python
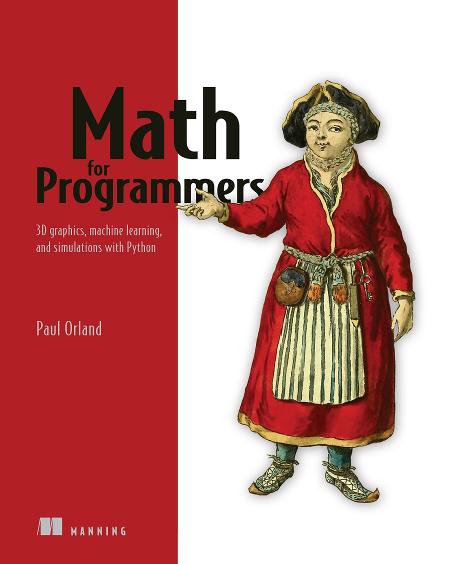
This is an excerpt from Manning's book Math for Programmers: 3D graphics, machine learning, and simulations with Python MEAP V11.
To have a tangent line, a function needs to be “smooth.” As a mini-project at the end of this section, you can try repeating this exercise with a function that’s not smooth, and you’ll see that there’s no line of best approximation. When we can find a tangent line to the graph of a function at a point, its slope is called the derivative of the function at the point. For instance, the derivative of the volume function at the point t = 1 is equal to 0.421875 (barrels per hour).
Figure 10.1: The derivative of the function f(x) = x3 has an exact formula, namely f ′(x) = 3x2.
![]()
There are infinitely many formulas you might want to know the derivative of, and you can’t memorize derivatives for all of them, so what you end up doing in a calculus class is learning a small set of rules and how to systematically apply them to transform a function into its derivative. By and large, this isn’t that useful of a skill for a programmer. If you want to know the exact formula for a derivative, you can use a specialized tool called a computer algebra system to compute it for you.
In the last chapter, you learned that the derivative of x3 is 3x2. By the time we’re done in this chapter, you’ll be able to write a Python function that takes an algebraic expression and gives you an expression for its derivative. Our data structure for an algebraic formula will be able to represent variables, numbers, sums, differences, products, quotients, powers, and special functions like sine and cosine. If you think about it, we can represent a huge variety of different formulas with that handful of building blocks, and our derivative will work on all of them (figure 10.5).
Figure 10.5: A goal is to write a derivative function in Python that takes an expression for a function and returns an expression for its derivative.
![]()
We’ll get started by modeling expressions as data structures instead of functions in Python code. Then, to warm up, we can do some simple computations with the data structures to do things like plugging in numbers for variables or expanding products of sums. After that, I’ll teach you some of the rules for taking derivatives of formulas, and we’ll write our own derivative function and perform them automatically on our symbolic data structures.
If we’re taking the derivative of f(x) = x, the result is f′(x) = 1, which is the slope of the line. Taking the derivative of f(x) = c should give us 0 as c represents a constant here, rather than the argument of the function f. For that reason, the derivative of a variable is 1 only if it’s the variable we’re taking the derivative with respect to; otherwise, the derivative is 0:
class Variable(Expression): ... def derivative(self, var): if self.symbol == var.symbol: return Number(1) else: return Number(0)The easiest combinator to take derivatives of is Sum: the derivative of a Sum function is just the sum of the derivatives of its terms: