concept guard in category python
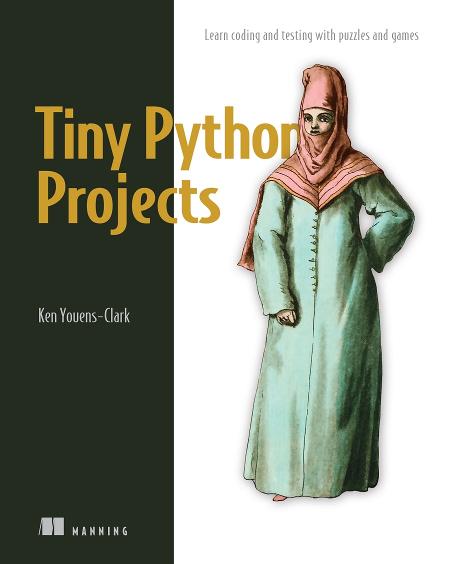
This is an excerpt from Manning's book Tiny Python Projects: Learn coding and testing with puzzles and games.
For the purposes of this program, the “stem” of a word is the part after any initial consonants, which I define using a list comprehension with a guard to take only the letters that are not vowels:
>>> vowels = 'aeiou' >>> consonants = ''.join([c for c in string.ascii_lowercase if c not in vowels])Throughout the chapters, I have shown how a list comprehension is a concise way to generate a list and is preferable to using a
for
loop to append to an existing list. Here we have added anif
statement to only include some characters if they are not vowels. This is called a guard statement, and only those elements that evaluate as “truthy” will be included in the resultinglist
.We’ve looked at
map()
several times now and talked about how it is a higher-order function (HOF) because it takes another function as the first argument and will apply it to all the elements from some iterable (something that can be iterated, like alist
). Here I’d like to introduce another HOF calledfilter()
, which also takes a function and an iterable (see figure 14.12). As with the list comprehension with the guard, only those elements that return a “truthy” value from the function are allowed in the resultinglist
.