concept key in category python
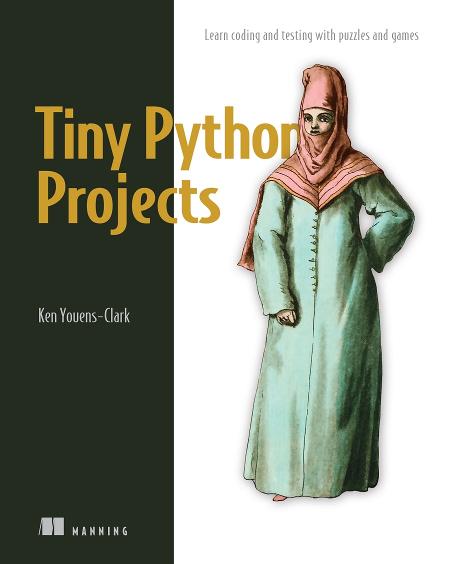
This is an excerpt from Manning's book Tiny Python Projects: Learn coding and testing with puzzles and games.
I’m using “favorite_color” (with an underscore) as the key, but I could use “favorite color” (with a space) or “FavoriteColor” or “Favorite color,” but each one of those would be a separate and distinct string, or key. I prefer to use the PEP 8 naming conventions for dictionary keys and variable and functions names. PEP 8, the “Style Guide for Python Code” (www.python.org/ dev/peps/pep-0008/), suggests using lowercase names with words separated by underscores.
>>> for key in answers.keys(): ... print(key, answers[key]) ... name Sir Lancelot quest To seek the Holy Grail favorite_color blue
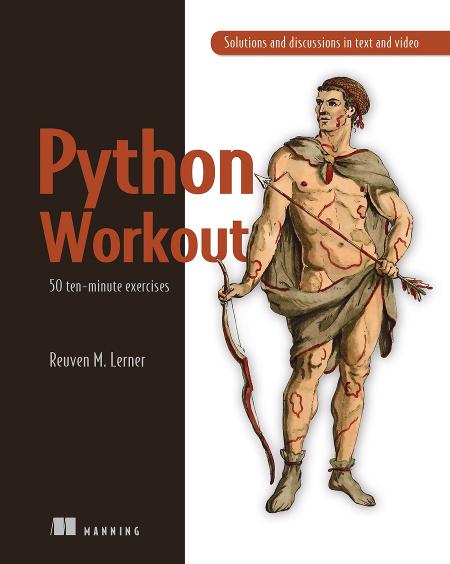
This is an excerpt from Manning's book Python Workout: 50 ten-minute exercises.
From what I’ve written so far, it might sound like any Python object can be used as the key or value in a dict. But that’s not true. While absolutely anything can be stored in a Python value, only hashable types, meaning those on which we can run the
hash
function, can be used as keys. This samehash
function ensures that a dict’s keys are unique, and that searching for a key can be quite fast.
One simple solution to this problem is to use the
dict.get
method with two arguments. With one argument,dict.get
either returns the value associated with the named key orNone
. But with two arguments,dict.get
returns either the value associated with the key or the second argument (figure 4.3).Thus, when we call
rainfall.get(city_name,
0)
, Python checks to see ifcity_name
already exists as a key inrainfall
. If so, then the call torainfall.get
will return the value associated with that key. Ifcity_name
is not inrainfall
, we get0
back.An alternative solution would use the
defaultdict
(http://mng.bz/pBy8), a class defined in thecollections
(http://mng.bz/6Qwy) module that allows you to define a dict that works just like a regular one--until you ask it for a key that doesn’t exist. In such cases,defaultdict
invokes the function with which it was defined; for example
def dictdiff(first, second): output = {} all_keys = first.keys() | second.keys() #1 for key in all_keys: if first.get(key) != second.get(key): output[key] = [first.get(key), second.get(key)] #2 return output d1 = {'a':1, 'b':2, 'c':3} d2 = {'a':1, 'b':2, 'd':4} print(dictdiff(d1, d2))
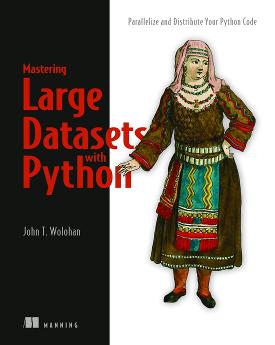
This is an excerpt from Manning's book Mastering Large Datasets with Python: Parallelize and Distribute Your Python Code.
The key with map is recognizing situations where we can apply this three-step pattern. Once we start looking for it, we’ll start to see it everywhere. Let’s take a look at another, and more complex, version of this pattern: web scraping.
CountBy— Get counts of keys resulting from a function.
To do this with mrjob, we’ll have to use a slightly different approach. mrjob keeps the mapper and reducer steps but wraps them up in a single worker class named mrjob. The methods of mrjob correspond directly to the steps we’re used to: there’s a .mapper method for the map step and a .reducer method for the reduce step. The required parameters for these two methods, though, are a little different from the map and reduce functions we’ve come to know. In mrjob, all the methods take a key and value parameter as input and return tuples of a key and a value as output (figure 8.6).
In Hadoop, map and reduce are implemented as two methods: .mapper and .reducer. Each method takes a sequence of key-value pairs and produces key-value pairs in return. The .mapper method produces intermediate key-value pairs. In other words, it takes in data as keys and values and outputs them for the .reducer. Because the .reducer is expecting a key-value pair, this is perfect. In fact, a hidden step between the map and reduce steps in Hadoop sorts the keys and values Hadoop consumes by key. This makes the .reducer job even easier.
Using keys allows Hadoop to make good use of our compute resources as it allocates work. Intermediate records output by map with like keys will tend to go to the same location for processing.
For our .mapper step in a standard MapReduce job, the key to our .mapper will be None, and the value will be the lines we consume. Because of this, our thinking about map and .mapper doesn’t have to change dramatically. We can ignore the key-value expectation of .mapper by simply ignoring the first parameter.
For the .reducer though, we will want to be aware of the key-value structure. Hadoop, through mrjob, does a lot of the organizing of keys and values for us. We can take advantage of that by considering the .mapper output not as a sequence but as a dict populated with keys and sequences. In our error analysis example, we’ll set these keys to the page URLs so we can quickly count the number of 404 errors associated with those pages. We can see this play out in listing 8.4.
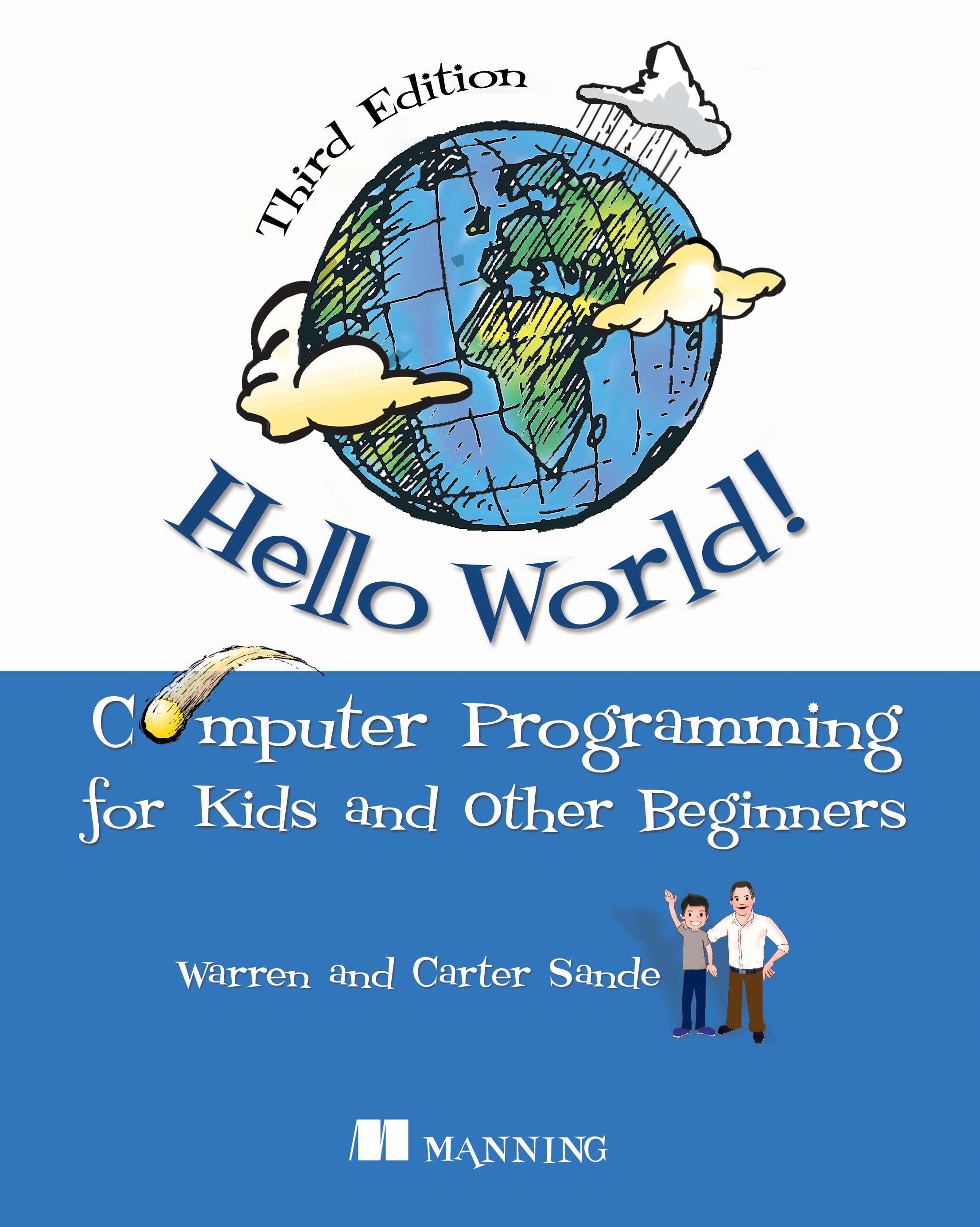
This is an excerpt from Manning's book Hello World! Third Edition.
By the way, the acronym GUI is usually pronounced “gooey,” instead of saying the letters, like “Gee You Eye.” It’s okay to have a GUI on your computer, but you should avoid getting anything gooey on your computer. It gets stuck in the keys and makes it hard to type!
All these events happen whenever somebody moves or clicks the mouse or presses a key. Where do they go? In the last section, I said that the event loop constantly scans part of the memory. The part of memory where events are stored is called the event queue.
You might have noticed that if you hold down the up or down arrow key, the ball only moves one step up or down. That’s because we didn’t tell our program what to do if a key was held down. When the user pressed the key, it generated a single KEYDOWN event, but there’s a setting in Pygame to make it generate multiple KEYDOWN events if a key is held down. This is known as key repeat. You tell it how long to wait before it starts repeating, and how often to repeat. The values are in milliseconds (thousandths of a second). It looks like this:
delay = 100 interval = 50 pygame.key.set_repeat(delay, interval)The delay value tells Pygame how long to wait before starting to repeat, and the interval value tells Pygame how fast the key should repeat—in other words, how long between each KEYDOWN event.
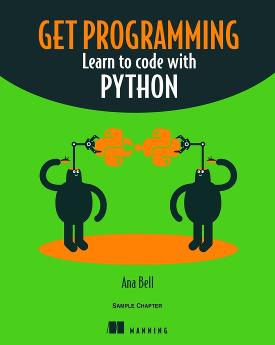
This is an excerpt from Manning's book Get Programming: Learn to code with Python.
Keys can’t be mutable objects.
This line creates a dictionary with three items, as shown in figure 27.2. Each item in a dictionary is separated by a comma. The keys and values for an item are separated by a colon. The key is to the left of the colon, and the value for that key is to the right of the colon.