concept plane in category python
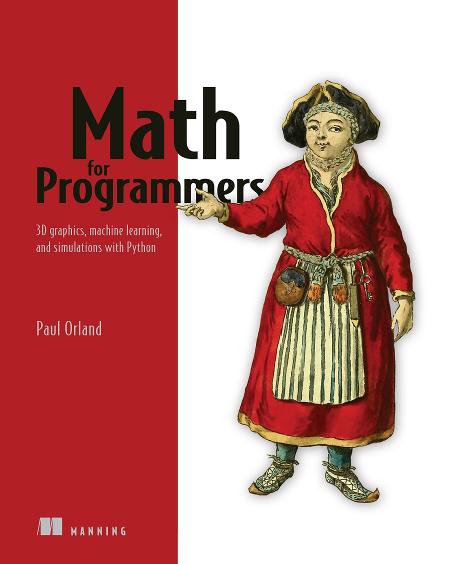
This is an excerpt from Manning's book Math for Programmers: 3D graphics, machine learning, and simulations with Python MEAP V11.
The 2D world is flat like a piece of paper or a computer screen. In the language of math, a flat, 2D space is referred to as a plane. An object living in a 2D plane has the two dimensions of height and width but no third dimension of depth. Likewise, you can describe locations in 2D by two pieces of information: their vertical and horizontal positions. To describe the location of points in the plane, you need a reference point. We call that special reference point the origin. Figure 2.1 shows this relationship.
Figure 2.1: Locating one of several points in the plane, relative to the origin
![]()
Given a point and a vector in 3D, there is a unique plane perpendicular to the vector and passing through that point. If the vector is (a, b, c) and the point is (x0, y0, z0), we can conclude that if a vector (x, y, z) lies in the plane, then (x−x0, y−y0, z−z0) is perpendicular to (a, b, c). Figure 7.22 shows this logic.
Figure 7.22: A plane parallel to the vector (a, b, c) passes through the point (x0, y0, z0).
![]()
Every point on the plane gives us such a perpendicular vector to (a, b, c), and every vector perpendicular to (a, b, c) leads us to a point in the plane. We can express this perpendicularity as a dot product of the two vectors, so the equation satisfied by every point (x, y, z) in the plane is
To be precise, a hyperplane in n dimensions is a solution to a linear equation in n unknown variables. A line is a 1D hyperplane living in 2D, and a plane is a 2D hyperplane living in 3D. As you might guess, a linear equation in standard form in 4D has the following form:
Mini-project 7.19: Write a Python function that takes three 3D points as inputs and returns the standard form equation of the plane that they lie in. For instance, if the standard form equation is ax + by + cz = d, the function could return the tuple (a, b, c, d).
Hint: Differences of any pairs of the three vectors are parallel to the plane, so cross products of the differences are perpendicular.
Solution: If the points given are p1, p2, and p3, then the vector differences like p3 − p1 and p2 − p1 are parallel to the plane. The cross product (p2 − p1) × (p3 − p1) is then perpendicular to the plane. (All is well as long as the points p1, p2, and p3 form a triangle, so the differences are not parallel.) With a point in the plane (for instance, p1) and a perpendicular vector, we can repeat the process of finding the standard form of the solution as in the previous two exercises:
from vectors import * def plane_equation(p1,p2,p3): parallel1 = subtract(p2,p1) parallel2 = subtract(p3,p1) a,b,c = cross(parallel1, parallel2) d = dot((a,b,c), p1) return a,b,c,dFor example, these are three points from the plane x + y + z = 3 from the preceding exercise: